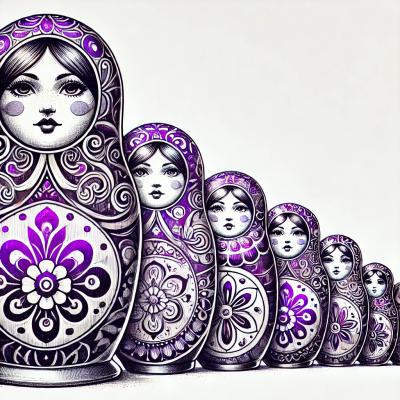
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Assume is an expect
inspired assertion library who's sole purpose is to create
a working and human readable assert library for browsers and node. The library
is designed to work with different assertion styles.
I've been trying out a lot of libraries over the last couple of years and none
of the assertion libraries that I found "tickled my fancy". They either only
worked in node or had really bad browser support. I wanted something that I can
use starting from Internet Explorer 5 to the latest version while maintaining
the expect
like API that we all know an love. Writing tests should be dead
simple and not cause any annoyances. This library attempts to achieve all of
this.
The module is written with browserify and Node.js in mind and can be installed using:
npm install --save-dev assume
The --save-dev
flag tells npm
to automatically add this package and it's
installed version to the devDependencies
of your module. It's not advised to
install this as an regular dependency as it was only designed to run in test and
throw
errors when assertions fail.
We support a lot of different syntaxes and assertion styles. The only thing we
will no (read never) support is the should
syntax as we will never extend
build-in objects/primitives of JavaScript.
The default syntax that we support is modeled after expect
so you can replace
any assertion library which implements this API by simply changing the require
to:
var expect = require('assume');
expect('foo').equals('foo');
expect('foo').is.a('string');
As you can see in the example above the is
property is used to make the
assertion more readable. We support the following aliases which allow these kind
of chains:
to
be
been
is
and
has
with
that
at
of
some
does
itself
which
So you can just write:
assume(100).is.at.most(100);
But do note that these aliases are optionally so the above example can also be written as:
assume(100).most(1000);
The module can be configured globally be changing the properties on the config
object:
var assume = require('assume');
assume.config.includeStack = false;
Or locally for each assertions by supplying the assume
function with an
optional configuration object:
assume('foo', { includeStack: false }).is.a('buffer');
The following options can be configured:
includeStack
Should we output a stack trace. Defaults to true
.showDIff
Show difference between the given and expected values. Defaults
to true
.There are various of assertions available. If you want the failed assertion to include a custom message or reason you can always add this as last argument of the assertion function.
assume(true).is.false('how odd, true is not a false');
The following assertions are available:
Asserts if the given value is the correct type. We need to use Object.toString
here because there are some implementation bugs the typeof
operator:
function
As well as all common flaws like Arrays being seen as Objects etc. This eliminates all these edge cases.
assume([]).is.a('array');
Asserts that the value is instanceof the given constructor.
function Classy() {}
var classes = new Classy();
assume(classes).is.an.instanceOf(Classy);
Assert that value includes a given value. I know this sounds vague but an example might be more useful here. It can check this for strings, objects and arrays.
assume({foo: 'bar'}).contains('foo');
assume('hello world').includes('world');
assume([1,3,4]).contains(1);
Assert that the value is truthy.
assume(1).is.ok();
assume(0).is.not.ok();
assume(true).is.ok();
Assert that the value is falsey.
assume(0).is.falsely();
assume(true).is.not.falsey();
assume(null).is.falsely;
Explicitly check that the value is the boolean true
.
assume(true).true();
Explicitly check that the value is the boolean false
.
assume(true).true();
Check if the value not not null
.
assume('hello').exists();
assume(undefined).exists(); // throws
Assert if the given value has the given length. It accepts arrays, strings,
functions, object and anything else that has a .length
property.
assume({ foo: 'bar' }).has.length(1);
assume([1,2,3,4,5,6]).is.size(6)
Short hand function for assume(val).length(0)
so it can check if objects,
arrays, strings are empty.
assume([]).empty();
assume('').empty();
assume({}).empty();
//
// Also works against everything that has a `.length` property
//
localStorage.clear();
assume(localStorage).is.empty();
Assert if the value is above the given value. If you need greater or equal check
out the least
method. If value to assert is not a number we automatically
extract the length out of it so you can use it check the length of arrays etc.
assume(100).is.above(10);
Assert if the value is above or equal to the given value. If you just need
greater check out the above
method. If value to assert is not a number we
automatically extract the length out of it so you can use it check the length of
arrays etc.
assume(100).is.least(10);
assume(100).is.least(100);
Assert if the value is less than the given value. If you need less or equal
check out the most
method. If value to assert is not a number we automatically
extract the length out of it so you can use it check the length of arrays etc.
assume(10).is.below(100);
Assert if the value is less or equal to the given value. If you just need less,
check out the less
method. If value to assert is not a number we automatically
extract the length out of it so you can use it check the length of arrays etc.
assume(10).is.most(100);
assume(100).is.most(100);
Check if the value is between or equal to a given range. If value to assert is not a number we automatically extract the length out of it so you can use it check the length of arrays etc.
assume(100).is.between(90, 100);
assume([1, 213, 13, 94, 5, 6, 7]).is.between(2, 10);
Assert that the value has the specified property.
assume({foo: bar}).owns('foo');
Matches the value against a given Regular Expression. If a string is given
instead of an actual Regular Expression we automatically transform it to an new RegExp
.
assume('hello world').matches(/world$/);
Assert that given value is strictly (===) equal to the supplied value.
assume('foo').equals('foo');
assume(13424).equals(13424);
Assert that the given value deeply equals the supplied value.
assume([1,2]).equals([1,2]);
Assert that the value is either one of the given values. It can be prefixed with
.deep
for deep assertions.
assume('foo').is.either('bar', 'banana', 'foo');
assume({ foo: 'bar' }).is.either('bar', 'banana', { foo: 'bar' });
Assert that the given function throws an error. The error can match a string, regexp or function instance.
function arrow() { throw new Error('you have failed this city'); }
assume(arrow).throws(/failed this city/);
assume(arrow).throws('failed this city');
assume(arrow).does.not.throw('your mom');
assume(function(){}).does.not.throw();
Assert that the value starts with the given string.
assume('foobar').startWith('foo');
Assert that the value ends with the given string.
assume('foor bar, banana').endsWith('ana');
Assert a float point number is near a given value within a delta margin.
assume(1.5).is.approximately(1.4, 0.2);
The asserts we write are assumptions that we receive a given value. While you're
writing tests you hope that they all pass. We could write these tests using an
i.hope.that(value)
syntax:
var i = require('assume');
i.hope.that('foo').is.a('string');
i.expect.that('foo').is.a('string');
i.assume.that('foo').equals('bar');
i.sincerely.hope.that('foo').is.a('string');
MIT
FAQs
Expect-like assertions that works seamlessly in node and browsers
The npm package assume receives a total of 0 weekly downloads. As such, assume popularity was classified as not popular.
We found that assume demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.