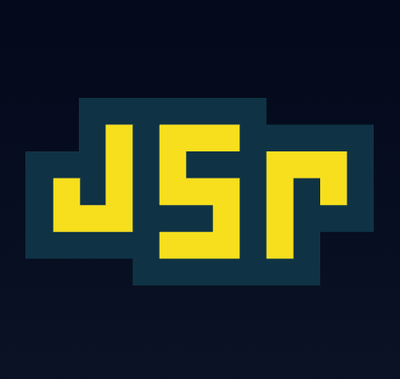
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Utilities for AST transformers.
$ npm install [--save] ast-util
# callArraySlice(scope, node[, begin, end])
Returns a call to Array.prototype.slice
with node
as the context and
begin
and end
as the arguments to slice
.
# callFunctionBind(scope, fn, context[, args])
Returns a call to Function.prototype.bind
using either call
or apply
depending on what the value of args
is. If args
is an expression then
apply
is used. If args
is an array of expressions, then call
.
# callGet(scope, object, property, receiver)
The [[Get]] internal method on objects would look something like helpers/get.js.
# callGetOwnPropertyDescriptor(scope, object, property)
Returns a call to Object.getOwnPropertyDescriptor
with the given object
and
property
.
# callGetPrototypeOf(scope, object)
Returns a call to Object.getPrototypeOf
with the given object
.
# callHasOwnProperty(scope, node, property)
Returns a call to hasOwnProperty
with node
as the context and property
as
the property to check.
# callSharedMethod(scope, callee, args)
Returns a call to the given callee
with args
as the arguments. If callee
is a string then it is treated as a globally-accessible function such as
Object.defineProperty
which will be stored in a unique temporary variable.
Subsequent calls to this function will re-use the same temporary variable.
# callSharedMethodWithContext(scope, callee, context, args)
Returns a call to the given callee
with context
as the method context and
args
as the arguments. If callee
is a string then it is treated as a
globally-accessible function such as Array.prototype.slice
which will be
stored in a unique temporary variable. Subsequent calls to this function will
re-use the same temporary variable.
# getGlobals(ast)
Gets a list of identifiers referencing global variables anywhere within the
given ast
. Assuming the ast is for this code:
var a;
function b(){ return c; }
b(d);
Then getGlobals
will return two identifiers, c
and a
.
# identifierForString(string)
Generate a safe JavaScript identifier for the given string.
# injectShared(scope, name, expression)
Injects a shared variable with a unique identifier. Only the first call with
the same scope
and name
will result in a variable declaration being
created. The expression
passed in can either be an AST node or a function to
generate one. This function is generally used to inject repeatedly-used values
and prevent repeated execution.
# injectVariable(scope, identifier[, init])
Injects a variable with the given identifier
into the given scope
as a
var
declaration with an optional initial value.
# isReference(path)
Determines whether the given path
is a value reference. For example, a
and
b
are references, but c
is not:
a(b.c);
Only identifiers count as references.
# isUsed(scope, name)
Determines whether the given name
should be considered "used" in the given
scope
. For a name to be used, it should either:
For example, a
, b
, and d
are used in the global scope of this example
while c
is not:
var a;
function b() {}
try {
a = b(d);
} catch (c) {
}
# sharedFor(scope, name)
Injects a shared variable by getting the named value from a dotted path. For example, this will return an identifier that can be used in place of the named expression:
sharedFor(scope, 'Object.defineProperty')
Subsequent calls to sharedFor
in the same scope will return the same
identifier.
# uniqueIdentifier(scope[, name])
Generates an identifier guaranteed not to collide with any others in the given
scope
. This function will also never generate the same identifier twice for
any scope
whose global scope already got that identifier.
Called in a scope with no global references and no variables, the first time
this function is called it will return an identifier named $__0
.
When called with a name that name will be used with a prefix, "$__", if possible. If that name is already used then it will append incrementing numbers until it finds a name that isn't used.
These methods are useful to source transforms, such as transpilers or macros.
Such transforms often have to insert variables into scopes and replace
expressions. Using injectVariable
and injectShared
are specifically for
that purpose. In conjunction with ast-types
, here's how you'd write a simple
version of a swap
macro:
// var tmp;
var tmp = util.injectVariable(
this.scope,
util.uniqueIdentifier(this.scope)
);
this.replace(
b.sequenceExpression([
// tmp = left
b.assignmentExpression(
'=',
tmp,
left
),
// left = right
b.assignmentExpression(
'=',
left,
right
),
// right = tmp
b.assignmentExpression(
'=',
right,
tmp
)
])
);
See examples/swap-macro.js for a more complete example.
First, install the development dependencies:
$ npm install
Then, try running the tests:
$ make test
If you're adding or editing code that injects helpers into a scope, you'll need to edit and run the Makefile to have it generate the files in lib/helpers from the files in helpers.
git checkout -b my-new-feature
)git commit -am 'Add some feature'
)git push origin my-new-feature
)Any contributors to the master ast-util repository must sign the Individual Contributor License Agreement (CLA). It's a short form that covers our bases and makes sure you're eligible to contribute.
When you have a change you'd like to see in the master repository, send a pull request. Before we merge your request, we'll make sure you're in the list of people who have signed a CLA.
Huge thanks to Ben Newman for ast-types, on which much of this library depends.
FAQs
Utilities for AST transformers.
The npm package ast-util receives a total of 285 weekly downloads. As such, ast-util popularity was classified as not popular.
We found that ast-util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.