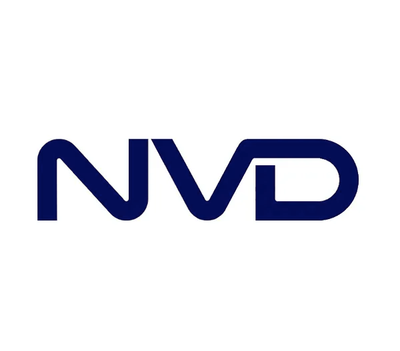
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
import { HttpApp, HttpKoa, HttpCors } from 'astad';
import Koa from 'koa';
import koaLogger from 'koa-logger';
import { koaBody } from 'koa-body';
// if required otherwise remove it
import ejs from 'ejs';
// config file
import { Config } from './config.js';
// main files
import web from './http/web/index.js';
import api from './http/api/index.js';
// koa instance
const app = new Koa();
// add middleware directly to koa
app.use(koaLogger());
app.use(koaBody({ multipart: true, formidable: { keepExtensions: true } }));
// create http app
const httpApp = new HttpApp({
use: new HttpKoa(app),
conf: Config,
});
// cors middleware
httpApp.use(new HttpCors());
// view engine if required
httpApp.viewEngine(new class {
async render(template: string, data: Record<any, any> = {}) {
return await ejs.renderFile(`./resources/views/${template}.ejs.html`, data);
}
});
// register routers
httpApp.router(web); // web router
httpApp.router('/api', api); // api router
// start server
httpApp.listen();
import { config } from 'dotenv';
import { Conf } from 'astad/conf';
config({
path: '.env',
});
export const Config = new Conf();
Web router file
import { HttpRouter } from 'astad/http';
const web = new HttpRouter();
web.get('/', async ctx => {
await ctx.view('welcome');
});
export default web;
Below is api router file
import { HTTP_KEY_REQ_ID, HttpRouter } from 'astad/http';
const api = new HttpRouter();
api.get('/', async ctx => {
ctx.json({
name: 'Astad Example',
ver: '0.1.0',
reqid: ctx.value(HTTP_KEY_REQ_ID),
});
});
export default api;
import { Astad, AstadContext, AstadCompose } from 'astad';
class TestCommand {
// note: signature is important, as register command will use this property
signature: string = "test";
description: string = "This is test command!";
compose() {
const command = new AstadCompose(this.signature);
return command;
}
async handle(ctx: AstadContext) {
ctx.info('This is test command!');
}
}
const cmd = new Astad();
cmd.register(new TestCommand);
await cmd.handle();
import { Container, ContainerScope } from 'astad/container';
const container = new Container('default');
container.register({
id: 'static value',
scope: ContainerScope.Value,
value: 'Any static value',
});
container.register({
id: 'static date',
scope: ContainerScope.Singleton,
value: () => new Date(),
});
container.register({
id: 'current date',
scope: ContainerScope.Transient,
value: () => new Date(),
});
// only available within context
container.register({
id: 'context date',
scope: ContainerScope.Contextual,
value: () => new Date(),
});
console.log(container.resolve('static value')); // will print "Any static value"
console.log(container.resolve('static date')); // will print same date not matter how many times you resolve
console.log(container.resolve('static date')); // will print same date not matter how many times you resolve
console.log(container.resolve('current date')); // always give current data
console.log(container.resolve('current date')); // always give current data
Testing routes, can use with any testing tool
import t from 'tap';
import { TestHttpRouter } from 'astad/testing';
import routes from './api.js';
const testroute = TestHttpRouter(routes);
function createRequest(
method: string,
path: string,
body?: Record<string, any>,
) {
const req: any = {
method: method,
path: `/api${path}`,
body,
headers: { accept: 'application/json' },
};
if (body) {
req.headers['content-type'] = 'application/json';
}
return testroute.req(req);
}
t.test('route', async t => {
const res = await createRequest('GET', '/').exec();
t.equal(res.status, 200);
const data = res.json();
t.hasOwnProp(data, 'name');
});
Testing services
import t from 'tap';
import { TestMinimalContext } from 'astad/testing';
import Service from './service.js';
// depends on service, if your service need context or your are using contextual container scope
const ctx = new TestMinimalContext();
t.test('service', async t => {
await ctx.run(async () => {
const service = new Service();
const data = await service.test();
t.ok(data);
})
});
FAQs
astad is a nodejs framework for api and web development
We found that astad demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.