Asuha
Asuha: the Webhook server for online git hosting services.

WIP
Currently only support for Bitbucket. There are other great packages for Github (e.g. probot) that you can have a try ;)
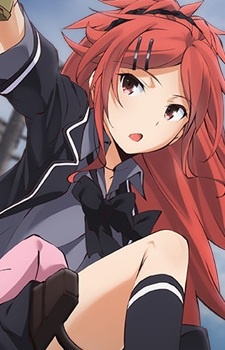
Installation
- Install from Github with npm,
npm i asuha
- For CLI usage,
npm i asuha -g
- Set up dependencies,
npm install
- Optional dependencies are
commander.js
and express
, if in your use case, none of those above is required (i.e. you are not using as CLI command and you prefer builtin http
/https
server), you can install dependencies without them through
npm install --no-optional
CLI
Document is WIP
API
Instance Method
set(configObj)
set(configKey, configValue)
See Configuration for available configs.
- Parameters
configObj
objectconfigKey
stringconfigValue
any
- Return
listen(...args)
It is an alias for net.Server#listen(). Read the document from Node official for information about parameters.
close(cb)
claim(repoPath)
Claim the existing repository at the local path repoPath
. This will fire claim
and init
events in order.
disclaim(repoPath)
disclaim(repoHost, repoFullname)
Disclaim a repository specified by the repoPath
or [ repoHost
, repoFullname
] pair.
- Parameters
repoPath
stringrepoHost
stringrepoFullname
string
on(event, listener)
once(event, listener)
See Events for available events and listener parameters.
Note that listener can be either a normal function or an async function (or a function with Promise
return type). Asuha adopts await-event-emitter
as her event handling system; therefore, listeners are executed one by one in the order they were defined.
- Parameters
event
objectlistener
Function
- Return
off(event)
off(event, listener)
Just like EventEmitter API, you can call this method with one parameter event
to remove all listeners related to this event or specified the listener as the second parameter.
MUST be called after Asuha#listen()
has been called; otherwise, nothing happens.
- Parameters
event
objectlistener
Function
- Return
Static Method
http()
Creating a http server with Asuha as the request handler.
https(httpsOptions)
Creating a https server with Asuha as the request handler.
express()
express(app)
Optional dependencies express
is required!
Creating an express server with Asuha as an express middleware. You can pass your custom express app as the first argument.
Configuration
This is the default public configuration for Asuha. Use Asuha#set()
to change the settings.
{
cwd: process.cwd(),
autoScan: true,
match: /^[^.#].*$/,
repoMappings: {},
subscribeEvents: [ 'push' ],
actions: [
'git pull'
],
stdio: {
stdout: process.stdout,
stderr: process.stderr
}
}
Events
The following events are listed in the order which they are fired when a remote event is received.
Note that action.pre
and action.post
can be fired multiple times in pair according to the number of configured actions.
init
fired only once after Asuha#init() called
repoPath
stringrepoFullname
stringhost
string
claim
fired when a repository is claimed before an init
event is fired
repoPath
stringrepoFullname
stringhost
string
disclaim
fired when a repository is disclaimed
repoPath
stringrepoFullname
stringhost
string
remote
actions.pre
repo
RepoMetaactions
string[]repoPath
string
action.pre
repo
RepoMetaaction
stringrepoPath
string
action.post
repo
RepoMetaaction
stringrepoPath
string
actions.post
repo
RepoMetaactions
string[]repoPath
string
done
RepoMeta
For all remote events, see Github or Bitbucket webhook doc for more info.
{
host: string,
name: string,
fullname: string,
owner: string,
event: string,
commits: {
hash: string,
message: string,
author: string,
timestamp: Date
}[]
}
Example
- Using
http
server with Asuha
@see Asuha.test.js
const asuha = Asuha.http()
.set('actions', [ 'git pull --rebase' ])
.set('cwd', __dirname)
.on('debug', console.debug.bind(console))
.listen( function () {
const { port, address } = asuha.server.address()
console.debug('Asuha is listening at %s:%d', address, port)
})
- Using async listeners for Asuha
const ret = []
const start = Date.now()
asuha.on('init', async function () {
ret.push(await setTimeout(() => 1, 2000))
})
.on('init', function () {
ret.push(2)
})
.on('init', async function () {
ret.push(await setTimeout(() => 3, 3000))
})
.listen(function () {
})