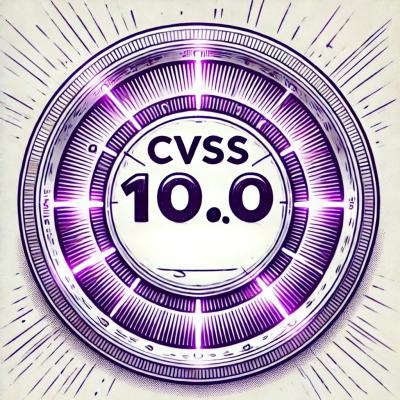
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
async-validate
Advanced tools
Asynchronous validation for node.
npm install async-validate
npm test
Basic usage involves defining a descriptor, assigning it to a schema and passing the object to be validated and a callback function to the validate
method of the schema:
var schema = require('async-validate');
var descriptor = {
name: {type: "string", required: true}
}
var validator = new schema(descriptor);
validator.validate({name: "muji"}, function(errors, fields) {
if(errors) {
// validation failed, errors is an array of all errors
// fields is an object keyed by field name with an array of
// errors per field
return handleErrors(errors, fields);
}
// validation passed
});
Rules may be functions that perform validation. The signature for a validation function is:
function(rule, value, callback, values)
rule
: The validation rule in the source descriptor that corresponds to the field name being validated. It is always assigned a field
property with the name of the field being validated.value
: The value of the source object property being validated.callback
: A callback function to invoke once validation is complete. It expects to be passed an array of Error
instances to indicate validation failure.values
: The source object that was passed to the validate
method.var schema = require('async-validate');
var ValidationError = schema.error;
var descriptor = {
name: function(rule, value, callback, values) {
var errors = [];
if(!/^[a-z0-9]+$/.test(value)) {
errors.push(
new ValidationError(
util.format("Field %s must be lowercase alphanumeric characters",
rule.field)));
}
callback(errors);
}
}
var validator = new schema(descriptor);
validator.validate({name: "Firstname"}, function(errors, fields) {
if(errors) {
return handleErrors(errors, fields);
}
// validation passed
});
It is often useful to test against multiple validation rules for a single field, to do so make the rule an array of objects, for example:
var descriptor = {
email: [
{type: "string", required: true, pattern: schema.pattern.email},
function(rule, value, callback, values) {
var errors = [];
// test if email address already exists in a database
// and add a validation error to the errors array if it does
callback(errors);
}
]
}
The validate method has the signature:
function(source, [options], callback)
source
: The object to validate - required.options
: An object describing processing options for the validation.callback
: A callback function to invoke when validation completes.first
: Invoke callback
when the first validation rule generates an error, no more validation rules are processed. If your validation involves multiple asynchronous calls (for example, database queries) and you only need the first error use this option.single
: Only ever return a single error typically used in conjunction with first
when a validation rule could generate multiple errors.keys
: Specifies the keys on the source object to be validated. Use this option to validate fields in a determinate order or to validate a subset of the rules assigned to a schema.Consider the rule:
{type: "string", required: true, min: 10, pattern: /^[^-].*$/}
When supplied with a source object such as {name: "-name"}
the validation rule would generate two errors, as the pattern does not match and the string length is less then the required minimum length for the field.
In this instance when you only want the first error encountered use the single
option.
Add a required
field to the rule to validate that the property exists.
Add a type
field to a rule to indicate that the field must be of the specified type. Recognised type values are:
string
number
boolean
regexp
integer
float
array
The pattern
field should be a valid RegExp
to test the value against.
When testing with a type
of string
the min
property determines the minimum number of characters for the string.
When testing with a type
of number
the min
property indicates the number may not be less than min
.
When testing with a type
of string
the max
property determines the maximum number of characters for the string.
When testing with a type
of number
the max
property indicates the number may not be greater than max
.
Combine the min
and max
properties to define a validation range.
It is typical to treat required fields that only contain whitespace as errors. To add an additional test for a string that consists solely of whitespace add a whitespace
property to a rule with a value of true
. The rule must be a string
type.
Some standard rules for common validation requirements are accessible via schema.rules.std
. You may wish to reference these rules or copy and modify them.
A typical required field:
{type: "string", required: true, whitespace: true}
A basic email validation rule using a pattern:
{type: "string", required: true, pattern: pattern.email}
Note validating email addresses by regular expressions is fraught with pitfalls, use this with caution.
A simple http(s) URL rule:
{type: "string", required: true, pattern: pattern.url}
A rule for hexadecimal color values with optional leading hash:
{type: "string", required: true, pattern: pattern.hex}
FAQs
Asynchronous validation for node and the browser
The npm package async-validate receives a total of 238 weekly downloads. As such, async-validate popularity was classified as not popular.
We found that async-validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.