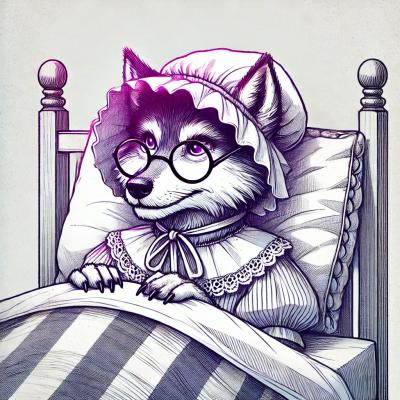
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Run a command in your terminal. Tiny exec() with promises and trim() for Node.js:
import cmd from 'atocha';
(async () => {
// Any basic command will work
console.log(await cmd('ls'));
// Using a better Promise interface, see the lib `swear`
console.log(await cmd('ls').split('\n'));
// Can pipe commands as normal
console.log(await cmd('sort record.txt | uniq'));
})();
Terminal Atocha; Madrid's train station.
exec()
.trim()
so you don't have to do it manually.stderr
will reject the promise with an error instance. Can be caught as normal with .catch()
or try {} catch (error) {}
.Install it in your project:
npm install atocha
Import it to be able to use it in your code:
const cmd = require('atocha'); // Old school
import cmd from 'atocha'; // New wave
Parsing this package's information:
const out = await cmd(`npm info atocha --json`);
const info = JSON.parse(out);
console.log(info.name + '@' + info.version);
// atocha@1.1.1
FAQs
Tiny exec() with Promises and trim()
We found that atocha demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.