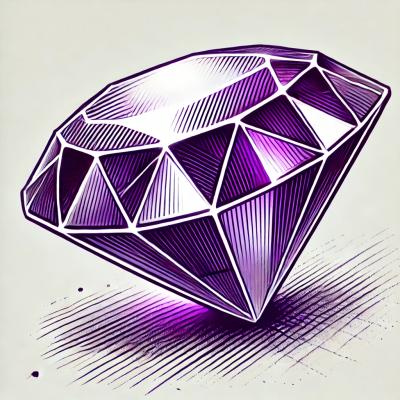
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
autobind-decorator
Advanced tools
The `autobind-decorator` npm package provides a decorator for automatically binding methods to their class instance. This is particularly useful in JavaScript and TypeScript classes where you want to ensure that methods retain the correct `this` context, especially when passing them as callbacks.
Automatic Method Binding
This feature allows you to automatically bind class methods to the instance of the class. In the example, the `getValue` method is bound to the instance of `MyClass`, ensuring that `this.value` correctly refers to the instance's `value` property even when the method is called as a standalone function.
class MyClass {
constructor() {
this.value = 42;
}
@autobind
getValue() {
return this.value;
}
}
const instance = new MyClass();
const getValue = instance.getValue;
console.log(getValue()); // 42
The `core-decorators` package provides a collection of decorators, including an `autobind` decorator. It offers a broader set of decorators for various use cases, such as `@debounce`, `@memoize`, and `@readonly`, making it a more versatile choice if you need multiple types of decorators.
The `lodash-decorators` package offers a set of decorators based on lodash functions, including an `autobind` decorator. It is useful if you are already using lodash in your project and want to leverage its utility functions in a decorator form.
The `typescript-decorators` package provides a set of decorators specifically designed for TypeScript. It includes an `autobind` decorator and other useful decorators for TypeScript development, making it a good choice for TypeScript projects.
A class or method decorator which binds methods to the instance so this
is always correct, even when the method is detached.
This is particularly useful for situations like React components, where you often pass methods as event handlers and would otherwise need to .bind(this)
.
// Before:
<button onClick={ this.handleClick.bind(this) }></button>
// After:
<button onClick={ this.handleClick }></button>
As decorators are a part of future ECMAScript standard they can only be used with transpilers such as Babel.
npm install autobind-decorator
We target IE11+ browsers (see out browserslist) with the following caveats:
main
: ES5
module
: ES5 + ES modules to enable tree shaking
es
: modern JS
On consuming modern JS, you can transpile the script to your target environment (@babel/preset-env is recommended) to minimise the cost. For more details, please read https://babeljs.io/blog/2018/06/26/on-consuming-and-publishing-es2015+-packages.
node 8.10+ with latest npm
The implementation of the decorator transform is currently on hold as the syntax is not final. If you would like to use this project with Babel 6, you may use babel-plugin-transform-decorators-legacy which implement Babel 5 decorator transform for Babel 6.
Babel 7's @babel/plugin-proposal-decorators
officially supports the same logic that babel-plugin-transform-decorators-legacy has, but integrates better with Babel 7's other plugins. You can enable this with
{
"plugins": [
["@babel/plugin-proposal-decorators", { "legacy": true }],
]
}
in your Babel configuration. Note that legacy: true
is specifically needed if you
want to get the same behavior as transform-decorators-legacy
because there
are newer versions of the decorator specification coming out, and they do not
behave the same way.
For now, you'll have to use one of the solutions in https://github.com/nicolo-ribaudo/legacy-decorators-migration-utility. We are trying to keep this module up-to-date with the latest spec. For more details, please read https://babeljs.io/blog/2018/09/17/decorators.
This package will work out of the box with TypeScript (no Babel needed) and includes the .d.ts
typings along with it.
Use @boundMethod
on a method
import {boundMethod} from 'autobind-decorator'
class Component {
constructor(value) {
this.value = value
}
@boundMethod
method() {
return this.value
}
}
let component = new Component(42)
let method = component.method // .bind(component) isn't needed!
method() // returns 42
@boundMethod
makes method
into an auto-bound method, replacing the explicit bind call later.
Magical @autobind
that can be used on both classes and methods
import autobind from 'autobind-decorator'
class Component {
constructor(value) {
this.value = value
}
@autobind
method() {
return this.value
}
}
let component = new Component(42)
let method = component.method // .bind(component) isn't needed!
method() // returns 42
// Also usable on the class to bind all methods
// Please see performance section below if you decide to autobind your class
@autobind
class Component { }
Use @boundClass
on a class
Please see performance section below if you decide to autobind your class
import {boundClass} from 'autobind-decorator'
@boundClass
class Component {
constructor(value) {
this.value = value
}
method() {
return this.value
}
}
let component = new Component(42)
let method = component.method // .bind(component) isn't needed!
method() // returns 42
autobind
(boundMethod
) on a method is lazy and is only bound once. :thumbsup:
However,
It is unnecessary to do that to every function. This is just as bad as autobinding (on a class). You only need to bind functions that you pass around. e.g.
onClick={this.doSomething}
. Orfetch.then(this.handleDone)
-- Dan Abramov
You should avoid using autobind
(boundClass
) on a class. :thumbsdown:
I was the guy who came up with autobinding in older Reacts and I'm glad to see it gone. It might save you a few keystrokes but it allocates functions that'll never be called in 90% of cases and has noticeable performance degradation. Getting rid of autobinding is a good thing -- Peter Hunt
FAQs
Decorator for binding method to an object
The npm package autobind-decorator receives a total of 152,624 weekly downloads. As such, autobind-decorator popularity was classified as popular.
We found that autobind-decorator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.