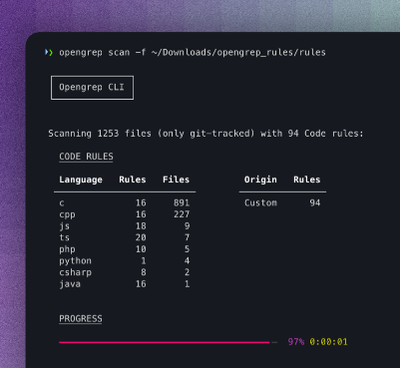
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
awesome-notifications
Advanced tools
It's a lightweight, fully customizable JavaScript library for showing notifications.
Advantages: no dependencies, advanced async support, fully customizable, >95% test coverage.
Demo: https://f3oall.github.io/awesome-notifications/
Attention! This library uses FontAwesome icons, so you either need to make sure that FontAwesome is connected to your project or disable icons, passing the
icons: {enabled: false}
property to options. Also you can preserve icons setting up a custom template for them via editingoptions.icons.prefix
andoptions.icons.suffix
Via NPM
npm install --save awesome-notifications
In browser
Download index.var.js and style.css, then put them in your html:
<head>
<link rel="stylesheet" href="path/to/style.css"></link>
<script src="path/to/index.var.js"></script>
</head>
Vue.js version
You can learn more in the Vue.js version repository: https://github.com/f3oall/vue-awesome-notifications
Node.js
import AWN from "awesome-notifications"
let notifier = new AWN(options)
In browser
<script>
var notifier = new AWN(options);
</script>
<button onclick="notifier.success('Success message');">Show Success</button>
Available functions
You can pass any valid HTML to html
functions params.
Function | Params | Description | Example |
---|---|---|---|
tip(html) | html - String , required | shows a gray toast with specified html | tip('First line text<br>Second line text') |
info(html) | html - String , required | shows a blue toast with specified html | info('<b>You can put any HTML here</b>') |
success(html) | html - String , required | shows a green toast with specified html | success('Simple none-HTML message') |
warning(html) | html - String , required | shows an orange toast with specified html | warning('Simple none HTML message') |
alert(html) | html - String , required | shows a red toast with specified html | alert('Simple none HTML message') |
async(promise, onResolve, onReject, html) | promise - Promise , required; onResolve - Function , String , optional, either callback or message; onReject - Function , String , optional, either callback or message; html - String , optional, html for async toast | shows an async gray toast untill promise will be completed, then run a callback or show new toast | async(somePromise, 'success toast msg', rejectCallback , 'Custom async msg') |
asyncBlock(promise, onResolve, onReject, html) | promise - Promise , required; onResolve - Function , String , optional, either callback or message; onReject - Function , String , optional, either callback or message; html - String , optional, html for async toast | shows loader and blocks the screen untill promise will be completed, then run a callback or show new toast | asyncBlock(somePromise, resolveCallback, 'custom message for alert toast' , 'Custom async msg') |
confirm(html, okFunc, cancelFunc) | html - String , required okFunc - Function , optional cancelFunc - Function , optional | shows a modal dialog, which is waiting for users confirmation | confirm('Are you sure?', runIfOkClicked, runIfCancelClicked) |
modal(html, className) | html - String , required className - String , required | shows a custom modal dialog with which contains only value of html | modal('<h2>Your custom title</h2><p>Your custom text</p>', 'custom-class-name') |
How to use async
There are two types of async functions in this library: async
and asyncBlock
. They differ in appearance but work with promises identically.
If your promise was rejected, alert toast will be shown to the user. New toast message will contain error returned by promise. Make sure that error returned by promise has type of string
Take a look on function behaviour after promise rejection:
notifier.async(Promise.reject("some error")) // will show a new alert toast with message "some error"
notifier.async(Promise.reject({message: "some error")) // will throw an error, because returned value is Object.
notifier.async(Promise.reject("some error"), null, "custom error") // you can pass a string as `onResolve`, it will be used as message for alert toast
notifier.async(Promise.reject("some error"), null, err => console.log(err)) // you can pass a function as `onResolve`, no new toast will be added
notifier.async(Promise.reject("some error")).then(() => {},err => console.log(err)) // run same function with preserving new alert toast
If most of your promises returns similar objects, you can set handleReject
function in options, to transform your objects to the strings:
let notifier = new AWN({
handleReject(value) {
// value is returned value of your promises reject
if (typeof value === "string") return value // optional check to preserve values which are already strings
// any code which should be applied to all reject values
return value.errors.message //choose string property of object that should be shown as error
}
})
notifer.async(Promise.reject({ errors: { message: "custom message" } })) // there is no error anymore, alert toast will be shown.
If your promise was resolved, nothing will happen by default. Customization here is similar:
notifier.async(Promise.resolve("all done")) // won't show anything after promise resolved
notifier.async(Promise.resolve("all done"), "your custom message") // will show a new success toast with message "your custom message"
notifier.async(Promise.resolve("all done"), result => {
notifier.success(`${result} and your custom message`)
}) // will show a new success toast with message "all done and your custom message"
notifier.async(Promise.resolve("all done")).then(result => {
notifier.success(`${result} and your custom message`)
}) // same as above, written without callback
*How to use modal
modal
function will create a new modal window with HTML that you provided. All your HTML will be put into the div
with class awn-modal-${className}
, where className
is the second parameter in modal
function.
Options
You can pass your own options when you're initializing a library, e.g.
var options = {
labels: {
tip: "Your custom tip box label"
}
}
var notifier = new AWN.default(options)
Available options
All labels
properties support HTML.
Name | Type | Default | Description |
---|---|---|---|
position | String | "bottom-right" | position of notifications |
duration | Number | 5000 | determines how long notification exists, ms |
animationDuration | Number | 300 | determines speed of animation, ms |
asyncBlockMinDuration | Number | 500 | minimal time to show asyncBlock modal window, prevents blinking, when async function completes too fast |
maxNotifications | Number | 10 | max amount of notifications |
handleReject | Function | see in source code | handles returned value of promise in async functions if it was rejected; By default will throw an error, if value type isn't a String |
labels | Object | See properties below | default labels for notifications |
labels.tip | String | "Tip" | default label for tip toast |
labels.info | String | "Info" | default label for info toast |
labels.success | String | "Success" | default label for success toast |
labels.warning | String | "Attention" | default label for warning toast |
labels.alert | String | "Error" | default label for alert toast |
labels.async | String | "Loading" | default label for async toast |
labels.confirm | String | "Confirmation required" | confrim window title |
icons | Object | See properties below | default Font Awesome icons for notifications |
icons.tip | String | "question-circle" | FontAwesome icon classes for tip toast, first one without fa- |
icons.info | String | "info-circle" | FontAwesome icon classes for info toast, first one without fa- |
icons.success | String | "check-circle" | FontAwesome icon classes for success toast, first one without fa- |
icons.warning | String | "exclamation-circle" | FontAwesome icon classes for warning toast, first one without fa- |
icons.alert | String | "warning" | FontAwesome icon classes for alert toast, first one without fa- |
icons.async | String | "cof fa-spin" | FontAwesome icon classes for async toast, first one without fa- |
icons.confirm | String | "warning" | FontAwesome icon classes for confirm window, first one without fa- |
icons.enabled | Boolean | false | Determines icons existence |
icons.prefix | String | "<i class='fa fa-fw fa" | HTML before any icons[value] (e.g. icons.tip ) |
icons.suffix | String | "'></i>" | HTML after any icons[value] (e.g. icons.tip ) |
modal | Object | See properties below | modal windows settings |
modal.okLabel | String | "OK" | confirm window success button label |
modal.cancelLabel | String | "Cancel" | confirm window cancel button label |
modal.maxWidth | String | "500px" | confirm window max-width CSS property |
messages | Object | See properties below | default messages |
messages.async | String | "Please, wait..." | default async toast message, supports HTML |
messages["async-block"] | String | "Loading" | default asyncBlock modal message, supports HTML |
replacements | Object | See properties below | contains rules of replacement for html each rule is Object where keys are first param for replace function and values are second param. |
replacements.general | Object | { "<script>": "", "</script>": "" } | rules for all event types |
replacements.tip | Object | "" | rules for tip events |
replacements.info | Object | "" | rules for info events |
replacements.success | Object | "" | rules for success events |
replacements.warning | Object | "" | rules for warning events |
replacements.alert | Object | "" | rules for alert events |
replacements.async | Object | "" | rules for async events |
replacements.asyncBlock | Object | "" | rules for asyncBlock modal window |
replacements.modal | Object | "" | rules for custom modal window |
replacements.confirm | Object | "" | rules for confirm window |
Styles
The most convinient and quick way to change styles is dowload styles folder which contains .scss
files. Then you have to edit variables.scss, compile your scss
to css
and add new css
file to your project.
Also, you can just add default style.css
to your project and override it in your styles file. To learn more about default styles, look at styles folder.
Make sure that you pass safe HTML to msg
param. Sending data which can be directly or indirectly edited by user (e.g. name of account), provides a possibility for HTML Injections. You can set up replacements
in options to filter msg
variable.
This project is licensed under the terms of the MIT license.
FAQs
Lightweight library for beautifull and smooth notifications
The npm package awesome-notifications receives a total of 1,566 weekly downloads. As such, awesome-notifications popularity was classified as popular.
We found that awesome-notifications demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.