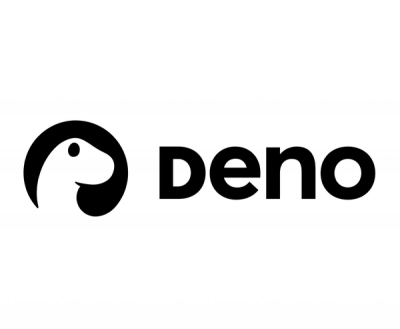
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
aws-deployer
Advanced tools
Should be considered very beta at the moment.
Provides a method to easily deploy/update code from a git repo to all servers within an AWS Autoscaling group. Similar to how AWS CodeDeploy works without the requirement of the CodeDeploy agent on the actual server.
var Deploy = require('aws-deployer')(config);
var opts = {
"parallel_groups": "availability_zone",
"auto_scaling_groups": "My-ASG",
"command" : "sudo su; pwd;"
};
Deploy.run(opts, function (err, groups) {
console.log(arguments);
});
{
aws : 'The sandard configuration options for the aws-sdk',
pem : 'String value of the AWS pem that is required to connect to the servers.'
}
##Public Functions
Runs a deployment.
Parameters:
##Events Emitted
Deployer will emit some helpful events:
When the instance has been fully setup and ready for use.
Deploy.on("ready", function(config){ });
When the instance has been fully setup and ready for use.
Deploy.on("start-deploy", function(config){ });
When the deployment has been started for a specific group.
Deploy.on("start-deploy-to-group", function(key){ });
When the deployment has been completed for a specific group.
Deploy.on("complete-deploy-to-group", function(key){ });
When the deployment process has fully completed.
Deploy.on("end-deploy", function(options){ });
When the instance has been fully setup and ready for use.
Deploy.on("start-deploy-to-instance", function(instance){ });
When the instance has been fully setup and ready for use.
Deploy.on("command-complete", function(data){ });
When the deployment has been completed on a specific instance.
Deploy.on("complete-deploy-to-instance", function(instance){ });
If an error is thrown.
Deploy.on("error", function(error){ });
var config = {
aws : {
accessKeyId: process.env.AWS_KEY ,
secretAccessKey: process.env.AWS_SECRET,
region: "us-east-1",
maxRetries: 2,
sslEnabled: true,
convertResponseTypes: true,
apiVersion: "2014-11-11"
},
pem : process.env.AWS_PEM
};
var Deploy = require('aws-deployer')(config);
var opts = {
"parallel_groups": "availability_zone",
"auto_scaling_groups": "SQL-Proxy-ASG",
"command" : "sudo su;cd /data/application;git reset --hard;git pull;npm install;pm2 reload all;pm2 jlist;"
};
Deploy.run(opts, function (err, ASGroups) {
console.log(arguments);
});
Deploy.on("ready", function(config){
console.dir("..-->ready---->", config)
});
Deploy.on("start-deploy", function(options){
console.log(".....Start Deploy---->", options)
});
Deploy.on("start-deploy-to-group", function(key){
console.log("..........start-deploy-to-group---->", key)
});
Deploy.on("complete-deploy-to-group", function(key){
console.log("...............complete-deploy-to-group---->", key)
});
Deploy.on("end-deploy", function(options){
console.log("end-deploy---->", options)
});
Deploy.on("start-deploy-to-instance", function(instance){
console.log("..............start-deploy-to-instance---->", instance)
});
Deploy.on("command-complete", function(data){
if(data.command === 'pm2 jlist'){
var response_parts = data.response.split('\n');
var json = JSON.parse(response_parts[1]);
var revision = json[0].pm2_env.versioning.revision
console.log('..............command-complete---->",', revision);
}
//console.log("............command-complete---->", details)
});
Deploy.on("complete-deploy-to-instance", function(instance){
console.log("-complete-deploy-to-instance---->", instance)
});
Deploy.on("error", function(err){
console.log("-ERROR---->", err)
});
FAQs
Handles automated deployment to all servers in an AWS autoscaling group.
The npm package aws-deployer receives a total of 1 weekly downloads. As such, aws-deployer popularity was classified as not popular.
We found that aws-deployer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.