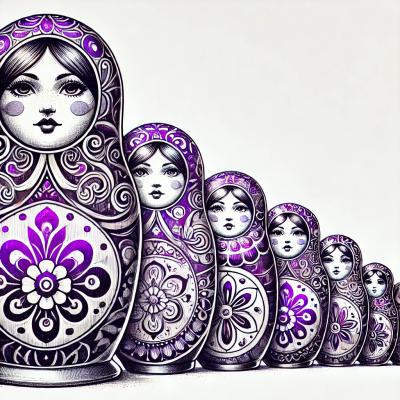
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
aws-sdk-wrap
Advanced tools
Wrapper around aws-sdk.
When dealing with the aws-sdk a lot, some calls become very repetitive and achieving code coverage becomes tiresome. This wrapper abstracts some of the repetitive logic.
Some examples of repetitive logic are:
.promise()
Install with npm:
$ npm install --save aws-sdk-wrap
Ensure required peer dependencies are available.
const aws = require('aws-sdk-wrap')();
aws
.call("s3", "putObject", { /* ... */ })
.then(/* ... */)
.catch(/* ... */);
where the first parameter is the service, the second parameter is the method and the third parameter are the "params" passed into the call.
Services are lazily initialized on first access.
Type: Logger
Default: null
Provide logger. E.g. logplease or lambda-rollbar.
When an unexpected error is risen, information is logged using .error(...)
.
Type: Object
Default: {}
AWS Config object used to initialize the service.
Type: list
Default: []
Provide string list of expected AWS error codes. Promise succeeds on expected error with error code as string.
FAQs
Wrapper around aws-sdk
The npm package aws-sdk-wrap receives a total of 231 weekly downloads. As such, aws-sdk-wrap popularity was classified as not popular.
We found that aws-sdk-wrap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.