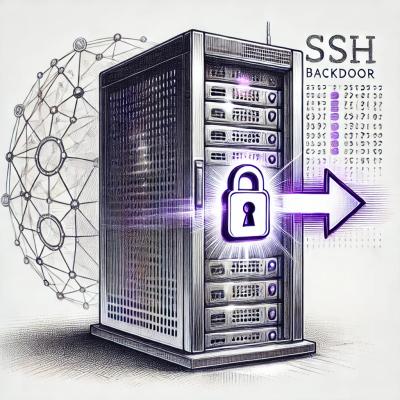
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
aws-serverless-express
Advanced tools
This library enables you to utilize AWS Lambda and Amazon API Gateway to respond to web and API requests using your existing Node.js application framework.
The aws-serverless-express package allows you to easily run Node.js web applications, such as those built with Express, on AWS Lambda and Amazon API Gateway. It simplifies the process of creating serverless applications by handling the integration between Lambda and API Gateway, allowing you to focus on your application logic.
Create a Serverless Express Application
This feature allows you to create a serverless Express application that can be deployed on AWS Lambda. The code sample demonstrates how to set up a simple Express app with a single route and export a Lambda handler that uses aws-serverless-express to handle incoming requests.
const awsServerlessExpress = require('aws-serverless-express');
const app = require('express')();
const server = awsServerlessExpress.createServer(app);
app.get('/hello', (req, res) => {
res.send('Hello, world!');
});
exports.handler = (event, context) => awsServerlessExpress.proxy(server, event, context);
Custom Lambda Proxy Integration
This feature allows you to customize the Lambda proxy integration, such as specifying custom binary MIME types. The code sample shows how to create a server with custom binary MIME types and handle requests with a custom route.
const awsServerlessExpress = require('aws-serverless-express');
const app = require('express')();
const server = awsServerlessExpress.createServer(app, null, ['*/*']);
app.get('/custom', (req, res) => {
res.json({ message: 'Custom integration' });
});
exports.handler = (event, context) => awsServerlessExpress.proxy(server, event, context);
Middleware Support
This feature demonstrates how to use middleware in your serverless Express application. The code sample shows how to log incoming requests using middleware and handle a route that responds with a message.
const awsServerlessExpress = require('aws-serverless-express');
const app = require('express')();
const server = awsServerlessExpress.createServer(app);
app.use((req, res, next) => {
console.log('Request received');
next();
});
app.get('/middleware', (req, res) => {
res.send('Middleware example');
});
exports.handler = (event, context) => awsServerlessExpress.proxy(server, event, context);
The serverless-http package is a similar tool that allows you to run web applications on AWS Lambda. It supports various frameworks like Express, Koa, and Hapi. Compared to aws-serverless-express, serverless-http offers broader framework support and a simpler API for converting your web application into a Lambda-compatible handler.
The lambda-api package is a lightweight web framework designed specifically for AWS Lambda. It provides a similar experience to Express but is optimized for serverless environments. Compared to aws-serverless-express, lambda-api is more lightweight and tailored for Lambda, offering better performance and lower cold start times.
While not a direct competitor, the express package is the core framework that aws-serverless-express is designed to work with. Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. aws-serverless-express leverages Express to create serverless applications, whereas Express itself is not inherently serverless.
This library enables you to utilize AWS Lambda and Amazon API Gateway to respond to web and API requests using your existing Node.js application framework. The sample provided allows you to easily build serverless web applications/services and RESTful APIs using the Express framework.
npm install aws-serverless-express
// lambda.js
'use strict'
const awsServerlessExpress = require('aws-serverless-express')
const app = require('./app')
const server = awsServerlessExpress.createServer(app)
exports.handler = (event, context) => awsServerlessExpress.proxy(server, event, context)
Package and create your Lambda function, then configure a simple proxy API using Amazon API Gateway and integrate it with your Lambda function.
In addition to a basic Lambda function and Express server, the example
directory includes a Swagger file, CloudFormation template, and helper scripts to help you set up and manage your AWS assets.
Windows users must have 7-Zip CLI installed http://www.7-zip.org/download.html and added to their path (setx path "%path%;C:\Program Files\7-Zip"
) to run the commands. You must also use the win-
prefixed commands, eg. npm run win-setup
. If you do not want to install 7-Zip, you can instead zip the necessary files using the Windows UI and modify the commands accordingly.
This guide assumes you have already set up an AWS account and have the AWS CLI installed.
git clone https://github.com/awslabs/aws-serverless-express.git && cd aws-serverless-express/example
.npm run config <accountId> <bucketName> [region]
to configure the example, eg. npm run config 123456789012 my-bucket us-west-2
. This modifies package.json
and simple-proxy-api.yaml
with your account ID, bucket, and region (region defaults to us-east-1
). If the bucket you specify does not yet exist, the next step will create it for you. This step modifies the existing files in-place; if you wish to make changes to these settings, you will need to modify package.json
and simple-proxy-api.yaml
manually.npm run setup
(Windows users: npm run win-setup
) - this installs the node dependencies, creates the S3 bucket (if it does not already exist), packages and uploads your serverless Express application assets to S3, uploads the API Swagger file to S3, and finally spins up a CloudFormation stack, which creates your API Gateway API and Lambda Function.AwsServerlessExpressStack
stack, and wait several minutes for the status to change to CREATE_COMPLETE
, then click the ApiUrl
value under the Outputs section - this will open a new page with your running API. The API index lists the resources available in the example Express server (app.js
), along with example curl
commands.See the sections below for details on how to migrate an existing (or create a new) Node.js project based on this example. If you would prefer to delete AWS assets that were just created, simply run npm run delete-stack
to delete the CloudFormation Stack, including the API and Lambda Function. If you specified a new bucket in the config
command for step 1 and want to delete that bucket, run npm run delete-bucket
.
To use this example as a base for a new Node.js project:
example
directory into a new project directory (cp -r ./example ~/projects/my-new-node-project
). If you have not already done so, follow the steps for running the example (you may want to first modify some of the resource names to something more project-specific, eg. the CloudFormation stack, Lambda function, and API Gateway API).app.js
, simply run npm run package-upload-update-function
(Windows users: npm run win-package-upload-update-function
). This will compress lambda.js
, app.js
, index.html
, and your node_modules
directory into lambda-function.zip
, upload that zip to S3, and update your Lambda function.To migrate an existing Node server:
example
directory: api-gateway-event.json
, cloudformation.json
, lambda.js
, and simple-proxy-api.yaml
. Additionally, copy the scripts
and config
sections of example/package.json
into your existing package.json
- this includes many helpful commands to manage your AWS serverless assets and perform basic local simulation of API Gateway and Lambda. If you have not already done so, follow the steps for running the example (be sure to copy over configure.js
. You may want to first modify some of the resource names to something more project-specific, eg. the CloudFormation stack, Lambda function, and API Gateway API).npm install --save aws-serverless-express
.lambda.js
to import your own server configuration (eg. change require('./app')
to require('./app')
). You will need to ensure you export your app configuration from the necessary file (eg. module.exports = app
). This library takes your app configuration and listens on a Unix Domain Socket for you, so you can remove your call to app.listen()
(if you have a server.listen
callback, you can provide it as the second parameter in the awsServerlessExpress.createServer
method).package-function
script (win-package-function
for Windows users) in package.json
to include all files necessary to run your application. If everything you need is in a single child directory, this is as simple as changing app.js
to my-app-dir
(also remove index.html
from that command). If you are using a build tool, you will instead want to add your build output directory to this command.npm run package-upload-update-function
(Windows users: npm run win-package-upload-update-function
) to package (zip), upload (to S3), and update your Lambda function.To perform a basic, local simulation of API Gateway and Lambda with your Node server, update api-gateway-event.json
with some values that are valid for your server (httpMethod
, path
, body
etc.) and run npm run local
. AWS Lambda uses NodeJS 4.3 LTS, and it is recommended to use the same version for testing purposes.
If you need to make modifications to your API Gateway API, modify simple-proxy-api.yaml
and run npm run upload-api-gateway-swagger && npm run update stack
. If your API requires CORS, be sure to modify the two options
methods defined in the Swagger file, otherwise you can safely remove them. Note: there is currently an issue with updating CloudFormation when it's not obvious that one of its resources has been modified; eg. the Swagger file is an external file hosted on S3. To work around this, simply update one of the resource's properties, such as the Description
on the ApiGatewayApi
resource. To modify your other AWS assets, make your changes to cloudformation.json
and run npm run update-stack
. Alternatively, you can manage these assets via the AWS console.
This package includes middleware to easily get the event object Lambda receives from API Gateway
const awsServerlessExpressMiddleware = require('aws-serverless-express/middleware')
app.use(awsServerlessExpressMiddleware.eventContext())
FAQs
This library enables you to utilize AWS Lambda and Amazon API Gateway to respond to web and API requests using your existing Node.js application framework.
The npm package aws-serverless-express receives a total of 141,704 weekly downloads. As such, aws-serverless-express popularity was classified as popular.
We found that aws-serverless-express demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.