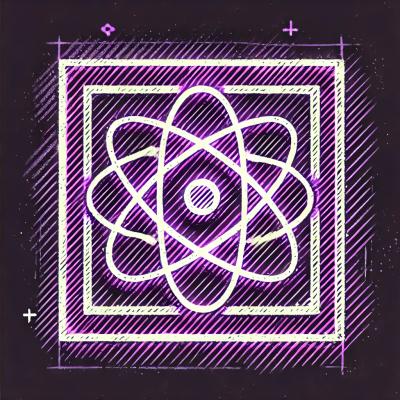
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
axios-sugar
Advanced tools
An axios wrapper, which supports resending, storage, cancel repeat request automatically; and so on.
import axios from 'axios'
import AxiosSugar from 'axios-sugar'
const axiosSugar = new AxiosSugar(axios);
// or
const ins = axios.create();
const axiosSugar = new AxiosSugar(ins);
// now you can use axios as before
axios.get();
// or
ins.get();
// ...
import axios from 'axios'
import AxiosSugar, { AxiosSugarConfig } from 'axios-sugar'
// AxiosSugarConfigOptions
let options = {
isResend: true,
isSave: true
}
const axiosSugar = new AxiosSugar(axios, {
config: new AxiosSugarConfig(options)
});
{
isResend?: boolean; // default: false
resendDelay?: number; // default: 1000 (ms)
resendTimes?: number; // default: 3
isSave?: boolean; // default: false
prop?: string; // default: custom to override the options above in a special request
}
prop is a property injected in the config of axios:
import axios from 'axios'
import AxiosSugar, { AxiosSugarConfig } from 'axios-sugar'
// AxiosSugarConfigOptions
let options = {
isResend: true,
isSave: true // default to use innerStorage
}
const axiosSugar = new AxiosSugar(axios, {
config: new AxiosSugarConfig(options)
});
axios.get('/path', {
// prop
custom: {
isSave: false // Now, this request will not be saved.
}
})
axios.get('/path') // This request will be saved
This library supports two way to save the response data(res.data in axios.then)
.
This storage will be save the data in a object variable.
import axios from 'axios'
import AxiosSugar, { AxiosSugarInnerStorage } from 'axios-sugar'
let options = {
isSave: true // default to use innerStorage
}
const axiosSugar = new AxiosSugar(axios, {
config: new AxiosSugarConfig(options),
storage: new AxiosSugarInnerStorage() // storage
});
Unlike InnerStorage, it can automatically free up memory.
import axios from 'axios'
import AxiosSugar, { AxiosSugarInnerReleaseStorage } from 'axios-sugar'
let options = {
isSave: true // default to use innerStorage
}
const axiosSugar = new AxiosSugar(axios, {
config: new AxiosSugarConfig(options),
storage: new AxiosSugarInnerReleaseStorage() // storage
});
// the constructor of AxiosSugarInnerReleaseStorage is:
/**
* duration: storage time (ms) default is 5 * 60 * 1000 (i.e. 5 minutes)
* limit: memory limit (Bytes) default is 15 * 1024 * 1024 (i.e. 15MB)
* constructor (duration: number, limit: number);
* /
This storage will be save the data in localStorage.
import axios from 'axios'
import AxiosSugar, { AxiosSugarLocalStorage } from 'axios-sugar'
let options = {
isSave: true // default to use innerStorage
}
const axiosSugar = new AxiosSugar(axios, {
config: new AxiosSugarConfig(options),
storage: new AxiosSugarLocalStorage() // storage
});
You can implements the interface AxiosSugarStorage to customize your storage. // The interface is:
interface AxiosSugarStorage {
set (symbol: string, res: any): void;
get (symbol: string): any;
contains (symbol: string): boolean;
}
// custom:
import axios from 'axios'
import AxiosSugar from 'axios-sugar'
let options = {
isSave: true // default to use innerStorage
}
// override set, get and contains methods
class CustomStorage {
// symbol is a string to identify a request, res is `res.data in axios.then`
set (symbol: string, res: any);
get (symbol: string);
contains (symbol: string);
}
const axiosSugar = new AxiosSugar(axios, {
config: new AxiosSugarConfig(options),
storage: new CustomStorage() // storage
});
The lifecycle is some callback.
import axios from 'axios'
import AxiosSugar, { AxiosSugarLifeCycle } from 'axios-sugar'
let cycle = new AxiosSugarLifeCycle();
// now, you can override some callback
cycle.beforeRequest = (conf) => {
return false // This will break the request
}
const axiosSugar = new AxiosSugar(axios, {
lifecycle: cycle
})
The callback of lifecycle is:
beforeRequest(conf: AxiosRequestConfig): AxiosSugarLifeCycleResult;
// carefully, this res is the `res` not `res.data`
beforeResponse(res: AxiosResponse): AxiosSugarLifeCycleResult;
All callback should return an AxiosSugarLifeCycleResult, it is a object:
{
state: false, // will break execution and throw an AxiosSugarError if state is false
message: '', // error message
}
The AxiosSugarError is:
{
reason: string; // error reason
message?: any;
data?: any;
}
Node test:
npm test
Some browser test(e.g. AxiosSugarLocalStorage)
:
open the corresponding index.html
File in /test
which built by mocha.
FAQs
A wrapper for axios
The npm package axios-sugar receives a total of 3 weekly downloads. As such, axios-sugar popularity was classified as not popular.
We found that axios-sugar demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.