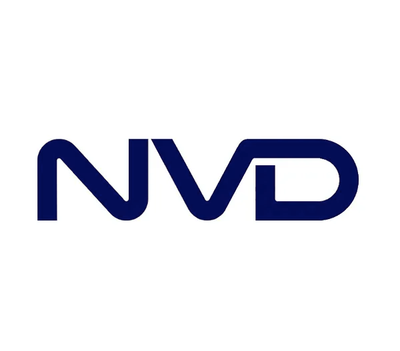
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
azure-arm-resource
Advanced tools
The azure-arm-resource npm package is a client library for managing Azure resources. It provides functionalities to interact with Azure Resource Manager (ARM) APIs, allowing you to create, update, delete, and manage Azure resources programmatically.
Create Resource Group
This code sample demonstrates how to create a new resource group in Azure using the azure-arm-resource package. It logs in using a service principal and then creates a resource group in the specified location.
const msRestAzure = require('ms-rest-azure');
const ResourceManagementClient = require('azure-arm-resource').ResourceManagementClient;
msRestAzure.loginWithServicePrincipalSecret(clientId, secret, domain, (err, credentials) => {
if (err) throw err;
const client = new ResourceManagementClient(credentials, subscriptionId);
const groupParameters = { location: 'westus' };
client.resourceGroups.createOrUpdate('myResourceGroup', groupParameters, (err, result) => {
if (err) throw err;
console.log('Resource group created:', result);
});
});
List Resource Groups
This code sample demonstrates how to list all resource groups in a subscription using the azure-arm-resource package. It logs in using a service principal and then retrieves and prints the list of resource groups.
const msRestAzure = require('ms-rest-azure');
const ResourceManagementClient = require('azure-arm-resource').ResourceManagementClient;
msRestAzure.loginWithServicePrincipalSecret(clientId, secret, domain, (err, credentials) => {
if (err) throw err;
const client = new ResourceManagementClient(credentials, subscriptionId);
client.resourceGroups.list((err, result) => {
if (err) throw err;
console.log('List of resource groups:', result);
});
});
Delete Resource Group
This code sample demonstrates how to delete a resource group in Azure using the azure-arm-resource package. It logs in using a service principal and then deletes the specified resource group.
const msRestAzure = require('ms-rest-azure');
const ResourceManagementClient = require('azure-arm-resource').ResourceManagementClient;
msRestAzure.loginWithServicePrincipalSecret(clientId, secret, domain, (err, credentials) => {
if (err) throw err;
const client = new ResourceManagementClient(credentials, subscriptionId);
client.resourceGroups.deleteMethod('myResourceGroup', (err, result) => {
if (err) throw err;
console.log('Resource group deleted:', result);
});
});
The azure-arm-compute package is used for managing Azure compute resources, such as virtual machines, VM scale sets, and availability sets. It provides functionalities specific to compute resources, whereas azure-arm-resource is more general and can manage a wider range of Azure resources.
The azure-arm-network package is used for managing Azure networking resources, such as virtual networks, subnets, and network interfaces. It focuses on networking-specific functionalities, while azure-arm-resource provides a broader scope for managing various types of Azure resources.
The azure-arm-storage package is used for managing Azure storage resources, such as storage accounts and blobs. It is specialized for storage-related operations, whereas azure-arm-resource offers a more comprehensive set of functionalities for managing different types of Azure resources.
This project provides a Node.js package that makes it easy to manage Azure resources. Right now it supports:
npm install azure-arm-resource
var msRestAzure = require('ms-rest-azure');
var resourceManagement = require("azure-arm-resource");
// Interactive Login
msRestAzure.interactiveLogin(function(err, credentials) {
var client = new resourceManagement.ResourceManagementClient(credentials, 'your-subscription-id');
client.resources.list(function(err, result) {
if (err) console.log(err);
console.log(result);
});
});
var util = require('util');
var groupParameters = {
location: 'West US',
tags: {
tag1: 'val1',
tag2: 'val2'
}
};
var groupName = 'testGroup1';
client.resourceGroups.createOrUpdate(groupName, groupParameters, function (err, result, request, response) {
if (err) {
console.log(err);
/*err has reference to the actual request and response, so you can see what was sent and received on the wire.
The structure of err looks like this:
err: {
code: 'Error Code',
message: 'Error Message',
body: 'The response body if any',
request: reference to a stripped version of http request
response: reference to a stripped version of the response
}
*/
} else {
console.log('result is: ' + util.inspect(result, {depth: null}));
}
});
var groupName = 'testGroup1';
var resourceName = 'autorestsite102';
var params = { 'location': 'West US', 'properties' : { 'SiteMode': 'Limited', 'ComputeMode': 'Shared' }, 'Name': resourceName };
var resourceType = 'sites';
var parentResourcePath = '';
var resourceProviderNamespace = 'Microsoft.Web';
var apiVersion = '2014-04-01';
client.resources.createOrUpdate(groupName, resourceProviderNamespace, parentResourcePath,
resourceType, resourceName , apiVersion, params, function (err, result, request, response) {
if (err) {
console.log(err);
} else {
console.log(result);
}
});
var groupName = 'testGroup1';
var resourceName = 'autorestsite102';
var resourceType = 'sites';
var parentResourcePath = '';
var resourceProviderNamespace = 'Microsoft.Web';
var apiVersion = '2014-04-01';
client.resources.get(groupName, resourceProviderNamespace, parentResourcePath,
resourceType, resourceName, apiVersion, function (err, result, request, response) {
if (err) {
console.log(err);
} else {
console.log(result);
}
});
client.resources.list(function (err, result, request, response) {
if (err) {
console.log(err);
} else {
console.log(result);
}
});
var groupName = 'testGroup1';
var resourceName = 'autorestsite102';
var resourceType = 'sites';
var parentResourcePath = '';
var resourceProviderNamespace = 'Microsoft.Web';
var apiVersion = '2014-04-01';
client.resources.deleteMethod(groupName, resourceProviderNamespace, parentResourcePath,
resourceType, resourceName, apiVersion, function (err, result, request, response) {
if (err) {
console.log(err);
} else {
console.log(result);
}
});
var groupName = 'testGroup1';
client.resourceGroups.deleteMethod(groupName, function (err, result, request, response) {
if (err) {
console.log(err);
} else {
console.log(result);
}
});
Please take a look at the tests over here for more examples.
2017.04.03 version 2.0.0-preview
FAQs
Microsoft Azure Resource Management Client Library for node
The npm package azure-arm-resource receives a total of 202,328 weekly downloads. As such, azure-arm-resource popularity was classified as popular.
We found that azure-arm-resource demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.