Comparing version 0.7.0 to 0.8.0
@@ -1,2 +0,2 @@ | ||
!function(t){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=t();else if("function"==typeof define&&define.amd)define([],t);else{var e;"undefined"!=typeof window?e=window:"undefined"!=typeof global?e=global:"undefined"!=typeof self&&(e=self),e.babble=t()}}(function(){var t;return function e(t,n,r){function i(u,s){if(!n[u]){if(!t[u]){var a="function"==typeof require&&require;if(!s&&a)return a(u,!0);if(o)return o(u,!0);var c=new Error("Cannot find module '"+u+"'");throw c.code="MODULE_NOT_FOUND",c}var f=n[u]={exports:{}};t[u][0].call(f.exports,function(e){var n=t[u][1][e];return i(n?n:e)},f,f.exports,e,t,n,r)}return n[u].exports}for(var o="function"==typeof require&&require,u=0;u<r.length;u++)i(r[u]);return i}({1:[function(t,e){"use strict";e.exports=t("./lib/babble")},{"./lib/babble":3}],2:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if(!t)throw new Error("id required");this.id=t,this.listeners={},this.conversations={},this.connect()}var r=t("node-uuid"),i=t("es6-promise").Promise,o=t("./messagers"),u=t("./block/Block"),s=t("./block/Then"),a=t("./block/Tell"),c=t("./block/Listen");n.prototype.connect=function(t){if(this.disconnect(),t||(t=o["default"]()),"function"!=typeof t.connect)throw new Error("messager must contain a function connect(params: {id: string, callback: function}) : string");if("function"!=typeof t.disconnect)throw new Error("messager must contain a function disconnect(token: string)");if("function"!=typeof t.send)throw new Error("messager must contain a function send(params: {id: string, message: *})");var e,n=new i(function(t){e=t}),r=t.connect({id:this.id,message:this._onMessage.bind(this),connect:e});return this.disconnect=function(){t.disconnect(r)},this.send=t.send,n},n.prototype._onMessage=function(t){var e,n,r=t.message;return e=this.conversations[t.id],e?(this._run(e,r),!0):(n=this.listeners[r])?(e={id:t.id,peer:t.from,next:n,context:{from:t.from}},this._run(e,r),!0):!1},n.prototype.disconnect=function(){},n.prototype.send=function(){throw new Error("Cannot send: not connected")},n.prototype.listen=function(t,e){if("string"!=typeof t)throw new TypeError("Parameter message must be a string");var n=new c(e);return this.listeners[t]=n,n},n.prototype.tell=function(t,e){var n=r.v4(),i=new a,o={id:n,peer:t,next:i,context:{from:t}},f=this;return i.then=function l(t){if("function"==typeof t&&(t=new s(t)),!(t instanceof u))throw new TypeError("Parameter next must be a Block or function");return o.next=t,t instanceof c?f.conversations[n]=o:(e=f._run(o,e),t.then=l),t},e=this._run(o,e),i},n.prototype.ask=function(t,e,n){return this.tell(t,e).listen(n)},n.prototype._run=function(t,e){delete this.conversations[t.id];var n=t.next;do{var r=n.execute(e,t.context);e=r.result,n.next instanceof c&&(t.next=n.next,this.conversations[t.id]=t),n instanceof a&&this.send(t.peer,{id:t.id,from:this.id,to:t.peer,message:e}),n=r.block}while(n&&!(n instanceof c));return e},e.exports=n},{"./block/Block":4,"./block/Listen":6,"./block/Tell":7,"./block/Then":8,"./messagers":9,"es6-promise":30,"node-uuid":40}],3:[function(t,e,n){"use strict";var r=t("./Babbler"),i=t("./block/Tell"),o=t("./block/Listen"),u=t("./block/Then"),s=t("./block/Decision");n.babbler=function(t){return new r(t)},n.tell=function(t){var e="function"==typeof t?t:function(){return t};return new i(e)},n.ask=function(t,e){return n.tell(t).listen(e)},n.decide=function(t,e){return new s(t,e)},n.listen=function(t){return new o(t)},n.then=function(t){return new u(t)},n.Babbler=r,n.block={Block:t("./block/Block"),Then:t("./block/Then"),Decision:t("./block/Decision"),Listen:t("./block/Listen"),Tell:t("./block/Tell")},n.messagers=t("./messagers"),n.babblify=function(t,e){var r;if(e&&void 0!==e.id)r=e.id;else{if(void 0===t.id)throw new Error('Id missing. Ensure that either actor has a property "id", or provide an id as a property in second argument params');r=t.id}["ask","tell","listen"].forEach(function(e){if(void 0!==t[e])throw new Error('Conflict: actor already has a property "'+e+'"')});var i=e&&e.send||"send";if("function"!=typeof t[i])throw new Error('Missing function. Function "'+i+'(to, message)" expected on actor or on params');var o=n.babbler(r),u=e&&e.onMessage||"onMessage",s=t.hasOwnProperty(u)?t[u]:null;return t[u]=function(e,n){var r=o._onMessage(n);r||s.call(t,e,n)},o.send=function(e,n){setTimeout(function(){t[i](e,n)},0)},t.__babbler__={babbler:o,onMessage:s,onMessageName:u},t.ask=o.ask.bind(o),t.tell=o.tell.bind(o),t.listen=o.listen.bind(o),t},n.unbabblify=function(t){var e=t.__babbler__;return e&&(delete t.__babbler__,delete t.ask,delete t.tell,delete t.listen,delete t[e.onMessageName],e.onMessage&&(t[e.onMessageName]=e.onMessage)),t}},{"./Babbler":2,"./block/Block":4,"./block/Decision":5,"./block/Listen":6,"./block/Tell":7,"./block/Then":8,"./messagers":9}],4:[function(t,e){"use strict";function n(){this.next=null,this.previous=null}n.prototype.execute=function(){throw new Error("Cannot run an abstract Block")},e.exports=n},{}],5:[function(t,e){"use strict";function n(t,e){var r,i;if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if("function"==typeof t?(r=t,i=e):(r=null,i=t),r){if("function"!=typeof r)throw new TypeError("Parameter decision must be a function")}else r=function(t){return t};if(i&&i instanceof Function)throw new TypeError("Parameter choices must be an object");if(this.decision=r,this.choices={},i){var o=this;Object.keys(i).forEach(function(t){o.addChoice(t,i[t])})}}var r=t("./Block");n.prototype=Object.create(r.prototype),n.prototype.execute=function(t,e){var n=this.decision(t,e);if("string"!=typeof n)throw new TypeError("Decision function must return a string containing the id of the next block");var r=this.choices[n];if(!r)throw new Error('Block with id "'+n+'" not found');return{result:t,block:r}},n.prototype.addChoice=function(t,e){if("string"!=typeof t)throw new TypeError("String expected as choice id");if(!(e instanceof r))throw new TypeError("Block expected as choice");if(t in this.choices)throw new Error('Choice with id "'+t+'" already exists');return this.choices[t]=e,this},r.prototype.decide=function(t,e){var r=new n(t,e);return this.then(r)},e.exports=n},{"./Block":4}],6:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if(t&&"function"!=typeof t)throw new TypeError("Parameter callback must be a Function");this.callback=t||function(t){return t}}var r=t("./Block");n.prototype=Object.create(r.prototype),n.prototype.execute=function(t,e){var n=this.callback(t,e);return{result:n,block:this.next}},r.prototype.listen=function(t){var e=new n(t);return this.then(e)},e.exports=n},{"./Block":4}],7:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if(t&&"function"!=typeof t)throw new TypeError("Parameter callback must be a Function");this.callback=t||function(t){return t}}var r=t("./Block");n.prototype=Object.create(r.prototype),n.prototype.execute=function(t,e){var n=this.callback(t,e);return{result:n,block:this.next}},r.prototype.tell=function(t){var e="function"==typeof t?t:function(){return t},r=new n(e);return this.then(r)},r.prototype.ask=function(t,e){return this.tell(t).listen(e)},e.exports=n},{"./Block":4}],8:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if("function"!=typeof t)throw new TypeError("Parameter callback must be a Function");this.callback=t}var r=t("./Block");n.prototype=Object.create(r.prototype),n.prototype.execute=function(t,e){var n=this.callback(t,e);return{result:n,block:this.next}},r.prototype.then=function(t){if("function"==typeof t&&(t=new n(t)),!(t instanceof r))throw new TypeError("Parameter next must be a Block or function");for(var e=this;e.next;)e=e.next;for(var i=this;i.previous;)i=i.previous;return t.previous=this,e.next=t,i},e.exports=n},{"./Block":4}],9:[function(t,e,n){"use strict";n["pubsub-js"]=function(){var e=t("pubsub-js");return{connect:function(t){var n=e.subscribe(t.id,function(e,n){t.message(n)});return"function"==typeof t.connect&&t.connect(),n},disconnect:function(t){e.unsubscribe(t)},send:function(t,n){e.publish(t,n)}}},n.pubnub=function(e){var n;if("undefined"!=typeof window){if("undefined"==typeof window.PUBNUB)throw new Error("Please load pubnub first in the browser");n=window.PUBNUB}else n=t("pubnub");var r=n.init(e);return{connect:function(t){return r.subscribe({channel:t.id,message:t.message,connect:t.connect}),t.id},disconnect:function(t){r.unsubscribe(t)},send:function(t,e){r.publish({channel:t,message:e})}}},n["default"]=n["pubsub-js"]},{pubnub:void 0,"pubsub-js":41}],10:[function(t,e,n){function r(t,e,n){if(!(this instanceof r))return new r(t,e,n);var i,o=typeof t;if("number"===o)i=t>0?t>>>0:0;else if("string"===o)"base64"===e&&(t=A(t)),i=r.byteLength(t,e);else{if("object"!==o||null===t)throw new Error("First argument needs to be a number, array or string.");"Buffer"===t.type&&j(t.data)&&(t=t.data),i=+t.length>0?Math.floor(+t.length):0}var u;V?u=r._augment(new Uint8Array(i)):(u=this,u.length=i,u._isBuffer=!0);var s;if(V&&"number"==typeof t.byteLength)u._set(t);else if(T(t))if(r.isBuffer(t))for(s=0;i>s;s++)u[s]=t.readUInt8(s);else for(s=0;i>s;s++)u[s]=(t[s]%256+256)%256;else if("string"===o)u.write(t,0,e);else if("number"===o&&!V&&!n)for(s=0;i>s;s++)u[s]=0;return u}function i(t,e,n,r){n=Number(n)||0;var i=t.length-n;r?(r=Number(r),r>i&&(r=i)):r=i;var o=e.length;H(o%2===0,"Invalid hex string"),r>o/2&&(r=o/2);for(var u=0;r>u;u++){var s=parseInt(e.substr(2*u,2),16);H(!isNaN(s),"Invalid hex string"),t[n+u]=s}return u}function o(t,e,n,r){var i=D(O(e),t,n,r);return i}function u(t,e,n,r){var i=D(C(e),t,n,r);return i}function s(t,e,n,r){return u(t,e,n,r)}function a(t,e,n,r){var i=D(P(e),t,n,r);return i}function c(t,e,n,r){var i=D(M(e),t,n,r);return i}function f(t,e,n){return R.fromByteArray(0===e&&n===t.length?t:t.slice(e,n))}function l(t,e,n){var r="",i="";n=Math.min(t.length,n);for(var o=e;n>o;o++)t[o]<=127?(r+=N(i)+String.fromCharCode(t[o]),i=""):i+="%"+t[o].toString(16);return r+N(i)}function h(t,e,n){var r="";n=Math.min(t.length,n);for(var i=e;n>i;i++)r+=String.fromCharCode(t[i]);return r}function p(t,e,n){return h(t,e,n)}function d(t,e,n){var r=t.length;(!e||0>e)&&(e=0),(!n||0>n||n>r)&&(n=r);for(var i="",o=e;n>o;o++)i+=U(t[o]);return i}function g(t,e,n){for(var r=t.slice(e,n),i="",o=0;o<r.length;o+=2)i+=String.fromCharCode(r[o]+256*r[o+1]);return i}function y(t,e,n,r){r||(H("boolean"==typeof n,"missing or invalid endian"),H(void 0!==e&&null!==e,"missing offset"),H(e+1<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o;return n?(o=t[e],i>e+1&&(o|=t[e+1]<<8)):(o=t[e]<<8,i>e+1&&(o|=t[e+1])),o}}function b(t,e,n,r){r||(H("boolean"==typeof n,"missing or invalid endian"),H(void 0!==e&&null!==e,"missing offset"),H(e+3<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o;return n?(i>e+2&&(o=t[e+2]<<16),i>e+1&&(o|=t[e+1]<<8),o|=t[e],i>e+3&&(o+=t[e+3]<<24>>>0)):(i>e+1&&(o=t[e+1]<<16),i>e+2&&(o|=t[e+2]<<8),i>e+3&&(o|=t[e+3]),o+=t[e]<<24>>>0),o}}function v(t,e,n,r){r||(H("boolean"==typeof n,"missing or invalid endian"),H(void 0!==e&&null!==e,"missing offset"),H(e+1<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o=y(t,e,n,!0),u=32768&o;return u?-1*(65535-o+1):o}}function w(t,e,n,r){r||(H("boolean"==typeof n,"missing or invalid endian"),H(void 0!==e&&null!==e,"missing offset"),H(e+3<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o=b(t,e,n,!0),u=2147483648&o;return u?-1*(4294967295-o+1):o}}function m(t,e,n,r){return r||(H("boolean"==typeof n,"missing or invalid endian"),H(e+3<t.length,"Trying to read beyond buffer length")),J.read(t,e,n,23,4)}function _(t,e,n,r){return r||(H("boolean"==typeof n,"missing or invalid endian"),H(e+7<t.length,"Trying to read beyond buffer length")),J.read(t,e,n,52,8)}function E(t,e,n,r,i){i||(H(void 0!==e&&null!==e,"missing value"),H("boolean"==typeof r,"missing or invalid endian"),H(void 0!==n&&null!==n,"missing offset"),H(n+1<t.length,"trying to write beyond buffer length"),F(e,65535));var o=t.length;if(!(n>=o)){for(var u=0,s=Math.min(o-n,2);s>u;u++)t[n+u]=(e&255<<8*(r?u:1-u))>>>8*(r?u:1-u);return n+2}}function B(t,e,n,r,i){i||(H(void 0!==e&&null!==e,"missing value"),H("boolean"==typeof r,"missing or invalid endian"),H(void 0!==n&&null!==n,"missing offset"),H(n+3<t.length,"trying to write beyond buffer length"),F(e,4294967295));var o=t.length;if(!(n>=o)){for(var u=0,s=Math.min(o-n,4);s>u;u++)t[n+u]=e>>>8*(r?u:3-u)&255;return n+4}}function k(t,e,n,r,i){i||(H(void 0!==e&&null!==e,"missing value"),H("boolean"==typeof r,"missing or invalid endian"),H(void 0!==n&&null!==n,"missing offset"),H(n+1<t.length,"Trying to write beyond buffer length"),z(e,32767,-32768));var o=t.length;if(!(n>=o))return e>=0?E(t,e,n,r,i):E(t,65535+e+1,n,r,i),n+2}function I(t,e,n,r,i){i||(H(void 0!==e&&null!==e,"missing value"),H("boolean"==typeof r,"missing or invalid endian"),H(void 0!==n&&null!==n,"missing offset"),H(n+3<t.length,"Trying to write beyond buffer length"),z(e,2147483647,-2147483648));var o=t.length;if(!(n>=o))return e>=0?B(t,e,n,r,i):B(t,4294967295+e+1,n,r,i),n+4}function x(t,e,n,r,i){i||(H(void 0!==e&&null!==e,"missing value"),H("boolean"==typeof r,"missing or invalid endian"),H(void 0!==n&&null!==n,"missing offset"),H(n+3<t.length,"Trying to write beyond buffer length"),q(e,3.4028234663852886e38,-3.4028234663852886e38));var o=t.length;if(!(n>=o))return J.write(t,e,n,r,23,4),n+4}function S(t,e,n,r,i){i||(H(void 0!==e&&null!==e,"missing value"),H("boolean"==typeof r,"missing or invalid endian"),H(void 0!==n&&null!==n,"missing offset"),H(n+7<t.length,"Trying to write beyond buffer length"),q(e,1.7976931348623157e308,-1.7976931348623157e308));var o=t.length;if(!(n>=o))return J.write(t,e,n,r,52,8),n+8}function A(t){for(t=L(t).replace($,"");t.length%4!==0;)t+="=";return t}function L(t){return t.trim?t.trim():t.replace(/^\s+|\s+$/g,"")}function j(t){return(Array.isArray||function(t){return"[object Array]"===Object.prototype.toString.call(t)})(t)}function T(t){return j(t)||r.isBuffer(t)||t&&"object"==typeof t&&"number"==typeof t.length}function U(t){return 16>t?"0"+t.toString(16):t.toString(16)}function O(t){for(var e=[],n=0;n<t.length;n++){var r=t.charCodeAt(n);if(127>=r)e.push(r);else{var i=n;r>=55296&&57343>=r&&n++;for(var o=encodeURIComponent(t.slice(i,n+1)).substr(1).split("%"),u=0;u<o.length;u++)e.push(parseInt(o[u],16))}}return e}function C(t){for(var e=[],n=0;n<t.length;n++)e.push(255&t.charCodeAt(n));return e}function M(t){for(var e,n,r,i=[],o=0;o<t.length;o++)e=t.charCodeAt(o),n=e>>8,r=e%256,i.push(r),i.push(n);return i}function P(t){return R.toByteArray(t)}function D(t,e,n,r){for(var i=0;r>i&&!(i+n>=e.length||i>=t.length);i++)e[i+n]=t[i];return i}function N(t){try{return decodeURIComponent(t)}catch(e){return String.fromCharCode(65533)}}function F(t,e){H("number"==typeof t,"cannot write a non-number as a number"),H(t>=0,"specified a negative value for writing an unsigned value"),H(e>=t,"value is larger than maximum value for type"),H(Math.floor(t)===t,"value has a fractional component")}function z(t,e,n){H("number"==typeof t,"cannot write a non-number as a number"),H(e>=t,"value larger than maximum allowed value"),H(t>=n,"value smaller than minimum allowed value"),H(Math.floor(t)===t,"value has a fractional component")}function q(t,e,n){H("number"==typeof t,"cannot write a non-number as a number"),H(e>=t,"value larger than maximum allowed value"),H(t>=n,"value smaller than minimum allowed value")}function H(t,e){if(!t)throw new Error(e||"Failed assertion")}/*! | ||
!function(t){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=t();else if("function"==typeof define&&define.amd)define([],t);else{var e;"undefined"!=typeof window?e=window:"undefined"!=typeof global?e=global:"undefined"!=typeof self&&(e=self),e.babble=t()}}(function(){var t;return function e(t,n,r){function i(s,u){if(!n[s]){if(!t[s]){var a="function"==typeof require&&require;if(!u&&a)return a(s,!0);if(o)return o(s,!0);var c=new Error("Cannot find module '"+s+"'");throw c.code="MODULE_NOT_FOUND",c}var f=n[s]={exports:{}};t[s][0].call(f.exports,function(e){var n=t[s][1][e];return i(n?n:e)},f,f.exports,e,t,n,r)}return n[s].exports}for(var o="function"==typeof require&&require,s=0;s<r.length;s++)i(r[s]);return i}({1:[function(t,e){"use strict";e.exports=t("./lib/babble")},{"./lib/babble":4}],2:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if(!t)throw new Error("id required");this.id=t,this.listeners=[],this.conversations={},this.connect()}var r=t("node-uuid"),i=t("es6-promise").Promise,o=t("./messagers"),s=t("./Conversation"),u=(t("./block/Block"),t("./block/Then"),t("./block/Tell")),a=t("./block/Listen");t("./block/IIf"),n.prototype.connect=function(t){if(this.disconnect(),t||(t=o["default"]()),"function"!=typeof t.connect)throw new Error("messager must contain a function connect(params: {id: string, callback: function}) : string");if("function"!=typeof t.disconnect)throw new Error("messager must contain a function disconnect(token: string)");if("function"!=typeof t.send)throw new Error("messager must contain a function send(params: {id: string, message: *})");var e=null,n=new i(function(t){e=t}),r=t.connect({id:this.id,message:this._onMessage.bind(this),connect:e});return this.disconnect=function(){t.disconnect(r)},this.send=t.send,n},n.prototype._onMessage=function(t){if(t&&"id"in t&&"message"in t){var e=this,n=t.id,r=this.conversations[n];r&&r.length?r.forEach(function(e){e.deliver(t)}):(r||(r=[]),this.conversations[n]=r,this.listeners.forEach(function(i){var o=new s({id:n,self:e.id,other:t.from,context:{from:t.from},send:e.send});return r.push(o),o.deliver(t),e._process(i,o).then(function(){var t=r.indexOf(o);-1!==t&&r.splice(t,1),0===r.length&&delete e.conversations[n]})}))}},n.prototype.disconnect=function(){},n.prototype.send=function(){throw new Error("Cannot send: not connected")},n.prototype.listen=function(t,e){var n=new a;this.listeners.push(n);var r=n;return t&&(r=n.iif(t)),e&&(r=n.then(e)),r},n.prototype.tell=function(t,e){var n=this,i=r.v4(),o=new s({id:i,self:this.id,other:t,context:{from:t},send:n.send});this.conversations[i]=[o];var a=new u(e);return setTimeout(function(){n._process(a,o).then(function(){delete n.conversations[i]})},0),a},n.prototype.ask=function(t,e,n){return this.tell(t,e).listen(n)},n.prototype._process=function(t,e){return new i(function(n){function r(t,i){t.execute(e,i).then(function(t){t.block?r(t.block,t.result):n(e)})}r(t)})},e.exports=n},{"./Conversation":3,"./block/Block":5,"./block/IIf":7,"./block/Listen":8,"./block/Tell":9,"./block/Then":10,"./messagers":11,"es6-promise":33,"node-uuid":43}],3:[function(t,e){function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");this.id=t&&t.id||r.v4(),this.self=t&&t.self||null,this.other=t&&t.other||null,this.context=t&&t.context||{},this._send=t&&t.send||null,this._inbox=[],this._receivers=[]}var r=t("node-uuid"),i=t("es6-promise").Promise;n.prototype.send=function(t){return this._send(this.other,{id:this.id,from:this.self,to:this.other,message:t})},n.prototype.deliver=function(t){if(this._receivers.length){var e=this._receivers.shift();e(t.message)}else this._inbox.push(t.message)},n.prototype.receive=function(){var t=this;return this._inbox.length?i.resolve(this._inbox.shift()):new i(function(e){t._receivers.push(e)})},e.exports=n},{"es6-promise":33,"node-uuid":43}],4:[function(t,e,n){"use strict";var r=t("./Babbler"),i=t("./block/Tell"),o=t("./block/Listen"),s=t("./block/Then"),u=t("./block/Decision"),a=t("./block/IIf");n.babbler=function(t){return new r(t)},n.tell=function(t){return new i(t)},n.ask=function(t,e){return n.tell(t).listen(e)},n.decide=function(t,e){return new u(t,e)},n.listen=function(t){var e=new o;return t?e.then(t):e},n.then=function(t){return new s(t)},n.iif=function(t,e,n){return new a(t,e,n)},n.Babbler=r,n.block={Block:t("./block/Block"),Then:t("./block/Then"),Decision:t("./block/Decision"),IIf:t("./block/IIf"),Listen:t("./block/Listen"),Tell:t("./block/Tell")},n.messagers=t("./messagers"),n.babblify=function(t,e){var r;if(e&&void 0!==e.id)r=e.id;else{if(void 0===t.id)throw new Error('Id missing. Ensure that either actor has a property "id", or provide an id as a property in second argument params');r=t.id}["ask","tell","listen"].forEach(function(e){if(void 0!==t[e])throw new Error('Conflict: actor already has a property "'+e+'"')});var i=e&&e.send||"send";if("function"!=typeof t[i])throw new Error('Missing function. Function "'+i+'(to, message)" expected on actor or on params');var o=n.babbler(r),s=e&&e.onMessage||"onMessage",u=t.hasOwnProperty(s)?t[s]:null;return t[s]=u?function(e,n){o._onMessage(n),u.call(t,e,n)}:function(t,e){o._onMessage(e)},o.send=function(e,n){setTimeout(function(){t[i](e,n)},0)},t.__babbler__={babbler:o,onMessage:u,onMessageName:s},t.ask=o.ask.bind(o),t.tell=o.tell.bind(o),t.listen=o.listen.bind(o),t},n.unbabblify=function(t){var e=t.__babbler__;return e&&(delete t.__babbler__,delete t.ask,delete t.tell,delete t.listen,delete t[e.onMessageName],e.onMessage&&(t[e.onMessageName]=e.onMessage)),t}},{"./Babbler":2,"./block/Block":5,"./block/Decision":6,"./block/IIf":7,"./block/Listen":8,"./block/Tell":9,"./block/Then":10,"./messagers":11}],5:[function(t,e){"use strict";function n(){this.next=null,this.previous=null}n.prototype.execute=function(){throw new Error("Cannot run an abstract Block")},e.exports=n},{}],6:[function(t,e){"use strict";function n(t,e){var r,i;if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if("function"==typeof t?(r=t,i=e):(r=null,i=t),r){if("function"!=typeof r)throw new TypeError("Parameter decision must be a function")}else r=function(t){return t};if(i&&i instanceof Function)throw new TypeError("Parameter choices must be an object");if(this.decision=r,this.choices={},i){var o=this;Object.keys(i).forEach(function(t){o.addChoice(t,i[t])})}}var r=t("es6-promise").Promise,i=t("./Block"),o=t("../util").isPromise;t("./Then"),n.prototype=Object.create(i.prototype),n.prototype.execute=function(t,e){var n=this,i=this.decision(e,t.context),s=o(i)?i:r.resolve(i);return s.then(function(t){var r=n.choices[t];if(!r)throw new Error('Block with id "'+t+'" not found');return{result:e,block:r}})},n.prototype.addChoice=function(t,e){if("string"!=typeof t)throw new TypeError("String expected as choice id");if(!(e instanceof i))throw new TypeError("Block expected as choice");if(t in this.choices)throw new Error('Choice with id "'+t+'" already exists');return this.choices[t]=e,this},i.prototype.decide=function(t,e){var r=new n(t,e);return this.then(r)},e.exports=n},{"../util":12,"./Block":5,"./Then":10,"es6-promise":33}],7:[function(t,e){"use strict";function n(t,e,r){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if(this.condition=t instanceof Function?t:t instanceof RegExp?function(e){return t.test(e)}:function(e){return e==t},e&&!(e instanceof i))throw new TypeError("Parameter trueBlock must be a Block");if(r&&!(r instanceof i))throw new TypeError("Parameter falseBlock must be a Block");this.trueBlock=e||null,this.falseBlock=r||null}var r=t("es6-promise").Promise,i=t("./Block"),o=t("../util").isPromise;t("./Then"),n.prototype=Object.create(i.prototype),n.prototype.constructor=n,n.prototype.execute=function(t,e){var n=this,i=this.condition(e,t.context),s=o(i)?i:r.resolve(i);return s.then(function(t){var r=t?n.trueBlock||n.next:n.falseBlock;return{result:e,block:r}})},i.prototype.iif=function(t,e,r){var i=new n(t,e,r);return this.then(i)},e.exports=n},{"../util":12,"./Block":5,"./Then":10,"es6-promise":33}],8:[function(t,e){"use strict";function n(){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator")}{var r=(t("es6-promise").Promise,t("./Block"));t("./Then")}n.prototype=Object.create(r.prototype),n.prototype.constructor=n,n.prototype.execute=function(t){var e=this;return t.receive().then(function(t){return{result:t,block:e.next}})},r.prototype.listen=function(t){var e=new n,r=this.then(e);return t&&(r=r.then(t)),r},e.exports=n},{"./Block":5,"./Then":10,"es6-promise":33}],9:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");this.message=t}var r=t("es6-promise").Promise,i=t("./Block"),o=t("../util").isPromise;t("./Then"),t("./Listen"),n.prototype=Object.create(i.prototype),n.prototype.constructor=n,n.prototype.execute=function(t,e){var n,i=this;if("function"==typeof this.message){var s=this.message(e,t.context);n=o(s)?s:r.resolve(s)}else n=r.resolve(this.message);return n.then(function(e){var n=t.send(e),s=o(n)?n:r.resolve(n);return s.then(function(){return{result:e,block:i.next}})})},i.prototype.tell=function(t){var e=new n(t);return this.then(e)},i.prototype.ask=function(t,e){return this.tell(t).listen(e)},e.exports=n},{"../util":12,"./Block":5,"./Listen":8,"./Then":10,"es6-promise":33}],10:[function(t,e){"use strict";function n(t){if(!(this instanceof n))throw new SyntaxError("Constructor must be called with the new operator");if("function"!=typeof t)throw new TypeError("Parameter callback must be a Function");this.callback=t}var r=t("es6-promise").Promise,i=t("./Block"),o=t("../util").isPromise;n.prototype=Object.create(i.prototype),n.prototype.constructor=n,n.prototype.execute=function(t,e){var n=this,i=this.callback(e,t.context),s=o(i)?i:r.resolve(i);return s.then(function(t){return{result:t,block:n.next}})},i.prototype.then=function(t){if("function"==typeof t&&(t=new n(t)),!(t instanceof i))throw new TypeError("Parameter next must be a Block or function");for(var e=this;e.next;)e=e.next;for(var r=this;r.previous;)r=r.previous;return t.previous=this,e.next=t,r},e.exports=n},{"../util":12,"./Block":5,"es6-promise":33}],11:[function(t,e,n){"use strict";var r=t("es6-promise").Promise;n["pubsub-js"]=function(){var e=t("pubsub-js");return{connect:function(t){var n=e.subscribe(t.id,function(e,n){t.message(n)});return"function"==typeof t.connect&&t.connect(),n},disconnect:function(t){e.unsubscribe(t)},send:function(t,n){e.publish(t,n)}}},n.pubnub=function(e){var n;if("undefined"!=typeof window){if("undefined"==typeof window.PUBNUB)throw new Error("Please load pubnub first in the browser");n=window.PUBNUB}else n=t("pubnub");var i=n.init(e);return{connect:function(t){return i.subscribe({channel:t.id,message:t.message,connect:t.connect}),t.id},disconnect:function(t){i.unsubscribe(t)},send:function(t,e){return new r(function(n){i.publish({channel:t,message:e,callback:n})})}}},n["default"]=n["pubsub-js"]},{"es6-promise":33,pubnub:void 0,"pubsub-js":44}],12:[function(t,e,n){n.isPromise=function(t){return t&&"function"==typeof t.then&&"function"==typeof t["catch"]}},{}],13:[function(t,e,n){function r(t,e,n){if(!(this instanceof r))return new r(t,e,n);var i,o=typeof t;if("number"===o)i=t>0?t>>>0:0;else if("string"===o)"base64"===e&&(t=A(t)),i=r.byteLength(t,e);else{if("object"!==o||null===t)throw new Error("First argument needs to be a number, array or string.");"Buffer"===t.type&&L(t.data)&&(t=t.data),i=+t.length>0?Math.floor(+t.length):0}var s;V?s=r._augment(new Uint8Array(i)):(s=this,s.length=i,s._isBuffer=!0);var u;if(V&&"number"==typeof t.byteLength)s._set(t);else if(j(t))if(r.isBuffer(t))for(u=0;i>u;u++)s[u]=t.readUInt8(u);else for(u=0;i>u;u++)s[u]=(t[u]%256+256)%256;else if("string"===o)s.write(t,0,e);else if("number"===o&&!V&&!n)for(u=0;i>u;u++)s[u]=0;return s}function i(t,e,n,r){n=Number(n)||0;var i=t.length-n;r?(r=Number(r),r>i&&(r=i)):r=i;var o=e.length;R(o%2===0,"Invalid hex string"),r>o/2&&(r=o/2);for(var s=0;r>s;s++){var u=parseInt(e.substr(2*s,2),16);R(!isNaN(u),"Invalid hex string"),t[n+s]=u}return s}function o(t,e,n,r){var i=D(O(e),t,n,r);return i}function s(t,e,n,r){var i=D(P(e),t,n,r);return i}function u(t,e,n,r){return s(t,e,n,r)}function a(t,e,n,r){var i=D(M(e),t,n,r);return i}function c(t,e,n,r){var i=D(C(e),t,n,r);return i}function f(t,e,n){return H.fromByteArray(0===e&&n===t.length?t:t.slice(e,n))}function l(t,e,n){var r="",i="";n=Math.min(t.length,n);for(var o=e;n>o;o++)t[o]<=127?(r+=N(i)+String.fromCharCode(t[o]),i=""):i+="%"+t[o].toString(16);return r+N(i)}function h(t,e,n){var r="";n=Math.min(t.length,n);for(var i=e;n>i;i++)r+=String.fromCharCode(t[i]);return r}function p(t,e,n){return h(t,e,n)}function d(t,e,n){var r=t.length;(!e||0>e)&&(e=0),(!n||0>n||n>r)&&(n=r);for(var i="",o=e;n>o;o++)i+=U(t[o]);return i}function g(t,e,n){for(var r=t.slice(e,n),i="",o=0;o<r.length;o+=2)i+=String.fromCharCode(r[o]+256*r[o+1]);return i}function y(t,e,n,r){r||(R("boolean"==typeof n,"missing or invalid endian"),R(void 0!==e&&null!==e,"missing offset"),R(e+1<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o;return n?(o=t[e],i>e+1&&(o|=t[e+1]<<8)):(o=t[e]<<8,i>e+1&&(o|=t[e+1])),o}}function b(t,e,n,r){r||(R("boolean"==typeof n,"missing or invalid endian"),R(void 0!==e&&null!==e,"missing offset"),R(e+3<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o;return n?(i>e+2&&(o=t[e+2]<<16),i>e+1&&(o|=t[e+1]<<8),o|=t[e],i>e+3&&(o+=t[e+3]<<24>>>0)):(i>e+1&&(o=t[e+1]<<16),i>e+2&&(o|=t[e+2]<<8),i>e+3&&(o|=t[e+3]),o+=t[e]<<24>>>0),o}}function v(t,e,n,r){r||(R("boolean"==typeof n,"missing or invalid endian"),R(void 0!==e&&null!==e,"missing offset"),R(e+1<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o=y(t,e,n,!0),s=32768&o;return s?-1*(65535-o+1):o}}function w(t,e,n,r){r||(R("boolean"==typeof n,"missing or invalid endian"),R(void 0!==e&&null!==e,"missing offset"),R(e+3<t.length,"Trying to read beyond buffer length"));var i=t.length;if(!(e>=i)){var o=b(t,e,n,!0),s=2147483648&o;return s?-1*(4294967295-o+1):o}}function m(t,e,n,r){return r||(R("boolean"==typeof n,"missing or invalid endian"),R(e+3<t.length,"Trying to read beyond buffer length")),J.read(t,e,n,23,4)}function _(t,e,n,r){return r||(R("boolean"==typeof n,"missing or invalid endian"),R(e+7<t.length,"Trying to read beyond buffer length")),J.read(t,e,n,52,8)}function E(t,e,n,r,i){i||(R(void 0!==e&&null!==e,"missing value"),R("boolean"==typeof r,"missing or invalid endian"),R(void 0!==n&&null!==n,"missing offset"),R(n+1<t.length,"trying to write beyond buffer length"),F(e,65535));var o=t.length;if(!(n>=o)){for(var s=0,u=Math.min(o-n,2);u>s;s++)t[n+s]=(e&255<<8*(r?s:1-s))>>>8*(r?s:1-s);return n+2}}function B(t,e,n,r,i){i||(R(void 0!==e&&null!==e,"missing value"),R("boolean"==typeof r,"missing or invalid endian"),R(void 0!==n&&null!==n,"missing offset"),R(n+3<t.length,"trying to write beyond buffer length"),F(e,4294967295));var o=t.length;if(!(n>=o)){for(var s=0,u=Math.min(o-n,4);u>s;s++)t[n+s]=e>>>8*(r?s:3-s)&255;return n+4}}function k(t,e,n,r,i){i||(R(void 0!==e&&null!==e,"missing value"),R("boolean"==typeof r,"missing or invalid endian"),R(void 0!==n&&null!==n,"missing offset"),R(n+1<t.length,"Trying to write beyond buffer length"),z(e,32767,-32768));var o=t.length;if(!(n>=o))return e>=0?E(t,e,n,r,i):E(t,65535+e+1,n,r,i),n+2}function I(t,e,n,r,i){i||(R(void 0!==e&&null!==e,"missing value"),R("boolean"==typeof r,"missing or invalid endian"),R(void 0!==n&&null!==n,"missing offset"),R(n+3<t.length,"Trying to write beyond buffer length"),z(e,2147483647,-2147483648));var o=t.length;if(!(n>=o))return e>=0?B(t,e,n,r,i):B(t,4294967295+e+1,n,r,i),n+4}function x(t,e,n,r,i){i||(R(void 0!==e&&null!==e,"missing value"),R("boolean"==typeof r,"missing or invalid endian"),R(void 0!==n&&null!==n,"missing offset"),R(n+3<t.length,"Trying to write beyond buffer length"),q(e,3.4028234663852886e38,-3.4028234663852886e38));var o=t.length;if(!(n>=o))return J.write(t,e,n,r,23,4),n+4}function S(t,e,n,r,i){i||(R(void 0!==e&&null!==e,"missing value"),R("boolean"==typeof r,"missing or invalid endian"),R(void 0!==n&&null!==n,"missing offset"),R(n+7<t.length,"Trying to write beyond buffer length"),q(e,1.7976931348623157e308,-1.7976931348623157e308));var o=t.length;if(!(n>=o))return J.write(t,e,n,r,52,8),n+8}function A(t){for(t=T(t).replace($,"");t.length%4!==0;)t+="=";return t}function T(t){return t.trim?t.trim():t.replace(/^\s+|\s+$/g,"")}function L(t){return(Array.isArray||function(t){return"[object Array]"===Object.prototype.toString.call(t)})(t)}function j(t){return L(t)||r.isBuffer(t)||t&&"object"==typeof t&&"number"==typeof t.length}function U(t){return 16>t?"0"+t.toString(16):t.toString(16)}function O(t){for(var e=[],n=0;n<t.length;n++){var r=t.charCodeAt(n);if(127>=r)e.push(r);else{var i=n;r>=55296&&57343>=r&&n++;for(var o=encodeURIComponent(t.slice(i,n+1)).substr(1).split("%"),s=0;s<o.length;s++)e.push(parseInt(o[s],16))}}return e}function P(t){for(var e=[],n=0;n<t.length;n++)e.push(255&t.charCodeAt(n));return e}function C(t){for(var e,n,r,i=[],o=0;o<t.length;o++)e=t.charCodeAt(o),n=e>>8,r=e%256,i.push(r),i.push(n);return i}function M(t){return H.toByteArray(t)}function D(t,e,n,r){for(var i=0;r>i&&!(i+n>=e.length||i>=t.length);i++)e[i+n]=t[i];return i}function N(t){try{return decodeURIComponent(t)}catch(e){return String.fromCharCode(65533)}}function F(t,e){R("number"==typeof t,"cannot write a non-number as a number"),R(t>=0,"specified a negative value for writing an unsigned value"),R(e>=t,"value is larger than maximum value for type"),R(Math.floor(t)===t,"value has a fractional component")}function z(t,e,n){R("number"==typeof t,"cannot write a non-number as a number"),R(e>=t,"value larger than maximum allowed value"),R(t>=n,"value smaller than minimum allowed value"),R(Math.floor(t)===t,"value has a fractional component")}function q(t,e,n){R("number"==typeof t,"cannot write a non-number as a number"),R(e>=t,"value larger than maximum allowed value"),R(t>=n,"value smaller than minimum allowed value")}function R(t,e){if(!t)throw new Error(e||"Failed assertion")}/*! | ||
* The buffer module from node.js, for the browser. | ||
@@ -7,3 +7,3 @@ * | ||
*/ | ||
var R=t("base64-js"),J=t("ieee754");n.Buffer=r,n.SlowBuffer=r,n.INSPECT_MAX_BYTES=50,r.poolSize=8192;var V=function(){try{var t=new ArrayBuffer(0),e=new Uint8Array(t);return e.foo=function(){return 42},42===e.foo()&&"function"==typeof e.subarray&&0===new Uint8Array(1).subarray(1,1).byteLength}catch(n){return!1}}();r.isEncoding=function(t){switch(String(t).toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"raw":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return!0;default:return!1}},r.isBuffer=function(t){return!(null==t||!t._isBuffer)},r.byteLength=function(t,e){var n;switch(t=t.toString(),e||"utf8"){case"hex":n=t.length/2;break;case"utf8":case"utf-8":n=O(t).length;break;case"ascii":case"binary":case"raw":n=t.length;break;case"base64":n=P(t).length;break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":n=2*t.length;break;default:throw new Error("Unknown encoding")}return n},r.concat=function(t,e){if(H(j(t),"Usage: Buffer.concat(list[, length])"),0===t.length)return new r(0);if(1===t.length)return t[0];var n;if(void 0===e)for(e=0,n=0;n<t.length;n++)e+=t[n].length;var i=new r(e),o=0;for(n=0;n<t.length;n++){var u=t[n];u.copy(i,o),o+=u.length}return i},r.compare=function(t,e){H(r.isBuffer(t)&&r.isBuffer(e),"Arguments must be Buffers");for(var n=t.length,i=e.length,o=0,u=Math.min(n,i);u>o&&t[o]===e[o];o++);return o!==u&&(n=t[o],i=e[o]),i>n?-1:n>i?1:0},r.prototype.write=function(t,e,n,r){if(isFinite(e))isFinite(n)||(r=n,n=void 0);else{var f=r;r=e,e=n,n=f}e=Number(e)||0;var l=this.length-e;n?(n=Number(n),n>l&&(n=l)):n=l,r=String(r||"utf8").toLowerCase();var h;switch(r){case"hex":h=i(this,t,e,n);break;case"utf8":case"utf-8":h=o(this,t,e,n);break;case"ascii":h=u(this,t,e,n);break;case"binary":h=s(this,t,e,n);break;case"base64":h=a(this,t,e,n);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":h=c(this,t,e,n);break;default:throw new Error("Unknown encoding")}return h},r.prototype.toString=function(t,e,n){var r=this;if(t=String(t||"utf8").toLowerCase(),e=Number(e)||0,n=void 0===n?r.length:Number(n),n===e)return"";var i;switch(t){case"hex":i=d(r,e,n);break;case"utf8":case"utf-8":i=l(r,e,n);break;case"ascii":i=h(r,e,n);break;case"binary":i=p(r,e,n);break;case"base64":i=f(r,e,n);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":i=g(r,e,n);break;default:throw new Error("Unknown encoding")}return i},r.prototype.toJSON=function(){return{type:"Buffer",data:Array.prototype.slice.call(this._arr||this,0)}},r.prototype.equals=function(t){return H(r.isBuffer(t),"Argument must be a Buffer"),0===r.compare(this,t)},r.prototype.compare=function(t){return H(r.isBuffer(t),"Argument must be a Buffer"),r.compare(this,t)},r.prototype.copy=function(t,e,n,r){var i=this;if(n||(n=0),r||0===r||(r=this.length),e||(e=0),r!==n&&0!==t.length&&0!==i.length){H(r>=n,"sourceEnd < sourceStart"),H(e>=0&&e<t.length,"targetStart out of bounds"),H(n>=0&&n<i.length,"sourceStart out of bounds"),H(r>=0&&r<=i.length,"sourceEnd out of bounds"),r>this.length&&(r=this.length),t.length-e<r-n&&(r=t.length-e+n);var o=r-n;if(100>o||!V)for(var u=0;o>u;u++)t[u+e]=this[u+n];else t._set(this.subarray(n,n+o),e)}},r.prototype.slice=function(t,e){var n=this.length;if(t=~~t,e=void 0===e?n:~~e,0>t?(t+=n,0>t&&(t=0)):t>n&&(t=n),0>e?(e+=n,0>e&&(e=0)):e>n&&(e=n),t>e&&(e=t),V)return r._augment(this.subarray(t,e));for(var i=e-t,o=new r(i,void 0,!0),u=0;i>u;u++)o[u]=this[u+t];return o},r.prototype.get=function(t){return console.log(".get() is deprecated. Access using array indexes instead."),this.readUInt8(t)},r.prototype.set=function(t,e){return console.log(".set() is deprecated. Access using array indexes instead."),this.writeUInt8(t,e)},r.prototype.readUInt8=function(t,e){return e||(H(void 0!==t&&null!==t,"missing offset"),H(t<this.length,"Trying to read beyond buffer length")),t>=this.length?void 0:this[t]},r.prototype.readUInt16LE=function(t,e){return y(this,t,!0,e)},r.prototype.readUInt16BE=function(t,e){return y(this,t,!1,e)},r.prototype.readUInt32LE=function(t,e){return b(this,t,!0,e)},r.prototype.readUInt32BE=function(t,e){return b(this,t,!1,e)},r.prototype.readInt8=function(t,e){if(e||(H(void 0!==t&&null!==t,"missing offset"),H(t<this.length,"Trying to read beyond buffer length")),!(t>=this.length)){var n=128&this[t];return n?-1*(255-this[t]+1):this[t]}},r.prototype.readInt16LE=function(t,e){return v(this,t,!0,e)},r.prototype.readInt16BE=function(t,e){return v(this,t,!1,e)},r.prototype.readInt32LE=function(t,e){return w(this,t,!0,e)},r.prototype.readInt32BE=function(t,e){return w(this,t,!1,e)},r.prototype.readFloatLE=function(t,e){return m(this,t,!0,e)},r.prototype.readFloatBE=function(t,e){return m(this,t,!1,e)},r.prototype.readDoubleLE=function(t,e){return _(this,t,!0,e)},r.prototype.readDoubleBE=function(t,e){return _(this,t,!1,e)},r.prototype.writeUInt8=function(t,e,n){return n||(H(void 0!==t&&null!==t,"missing value"),H(void 0!==e&&null!==e,"missing offset"),H(e<this.length,"trying to write beyond buffer length"),F(t,255)),e>=this.length?void 0:(this[e]=t,e+1)},r.prototype.writeUInt16LE=function(t,e,n){return E(this,t,e,!0,n)},r.prototype.writeUInt16BE=function(t,e,n){return E(this,t,e,!1,n)},r.prototype.writeUInt32LE=function(t,e,n){return B(this,t,e,!0,n)},r.prototype.writeUInt32BE=function(t,e,n){return B(this,t,e,!1,n)},r.prototype.writeInt8=function(t,e,n){return n||(H(void 0!==t&&null!==t,"missing value"),H(void 0!==e&&null!==e,"missing offset"),H(e<this.length,"Trying to write beyond buffer length"),z(t,127,-128)),e>=this.length?void 0:(t>=0?this.writeUInt8(t,e,n):this.writeUInt8(255+t+1,e,n),e+1)},r.prototype.writeInt16LE=function(t,e,n){return k(this,t,e,!0,n)},r.prototype.writeInt16BE=function(t,e,n){return k(this,t,e,!1,n)},r.prototype.writeInt32LE=function(t,e,n){return I(this,t,e,!0,n)},r.prototype.writeInt32BE=function(t,e,n){return I(this,t,e,!1,n)},r.prototype.writeFloatLE=function(t,e,n){return x(this,t,e,!0,n)},r.prototype.writeFloatBE=function(t,e,n){return x(this,t,e,!1,n)},r.prototype.writeDoubleLE=function(t,e,n){return S(this,t,e,!0,n)},r.prototype.writeDoubleBE=function(t,e,n){return S(this,t,e,!1,n)},r.prototype.fill=function(t,e,n){if(t||(t=0),e||(e=0),n||(n=this.length),H(n>=e,"end < start"),n!==e&&0!==this.length){H(e>=0&&e<this.length,"start out of bounds"),H(n>=0&&n<=this.length,"end out of bounds");var r;if("number"==typeof t)for(r=e;n>r;r++)this[r]=t;else{var i=O(t.toString()),o=i.length;for(r=e;n>r;r++)this[r]=i[r%o]}return this}},r.prototype.inspect=function(){for(var t=[],e=this.length,r=0;e>r;r++)if(t[r]=U(this[r]),r===n.INSPECT_MAX_BYTES){t[r+1]="...";break}return"<Buffer "+t.join(" ")+">"},r.prototype.toArrayBuffer=function(){if("undefined"!=typeof Uint8Array){if(V)return new r(this).buffer;for(var t=new Uint8Array(this.length),e=0,n=t.length;n>e;e+=1)t[e]=this[e];return t.buffer}throw new Error("Buffer.toArrayBuffer not supported in this browser")};var Y=r.prototype;r._augment=function(t){return t._isBuffer=!0,t._get=t.get,t._set=t.set,t.get=Y.get,t.set=Y.set,t.write=Y.write,t.toString=Y.toString,t.toLocaleString=Y.toString,t.toJSON=Y.toJSON,t.equals=Y.equals,t.compare=Y.compare,t.copy=Y.copy,t.slice=Y.slice,t.readUInt8=Y.readUInt8,t.readUInt16LE=Y.readUInt16LE,t.readUInt16BE=Y.readUInt16BE,t.readUInt32LE=Y.readUInt32LE,t.readUInt32BE=Y.readUInt32BE,t.readInt8=Y.readInt8,t.readInt16LE=Y.readInt16LE,t.readInt16BE=Y.readInt16BE,t.readInt32LE=Y.readInt32LE,t.readInt32BE=Y.readInt32BE,t.readFloatLE=Y.readFloatLE,t.readFloatBE=Y.readFloatBE,t.readDoubleLE=Y.readDoubleLE,t.readDoubleBE=Y.readDoubleBE,t.writeUInt8=Y.writeUInt8,t.writeUInt16LE=Y.writeUInt16LE,t.writeUInt16BE=Y.writeUInt16BE,t.writeUInt32LE=Y.writeUInt32LE,t.writeUInt32BE=Y.writeUInt32BE,t.writeInt8=Y.writeInt8,t.writeInt16LE=Y.writeInt16LE,t.writeInt16BE=Y.writeInt16BE,t.writeInt32LE=Y.writeInt32LE,t.writeInt32BE=Y.writeInt32BE,t.writeFloatLE=Y.writeFloatLE,t.writeFloatBE=Y.writeFloatBE,t.writeDoubleLE=Y.writeDoubleLE,t.writeDoubleBE=Y.writeDoubleBE,t.fill=Y.fill,t.inspect=Y.inspect,t.toArrayBuffer=Y.toArrayBuffer,t};var $=/[^+\/0-9A-z]/g},{"base64-js":11,ieee754:12}],11:[function(t,e,n){var r="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";!function(t){"use strict";function e(t){var e=t.charCodeAt(0);return e===u?62:e===s?63:a>e?-1:a+10>e?e-a+26+26:f+26>e?e-f:c+26>e?e-c+26:void 0}function n(t){function n(t){c[l++]=t}var r,i,u,s,a,c;if(t.length%4>0)throw new Error("Invalid string. Length must be a multiple of 4");var f=t.length;a="="===t.charAt(f-2)?2:"="===t.charAt(f-1)?1:0,c=new o(3*t.length/4-a),u=a>0?t.length-4:t.length;var l=0;for(r=0,i=0;u>r;r+=4,i+=3)s=e(t.charAt(r))<<18|e(t.charAt(r+1))<<12|e(t.charAt(r+2))<<6|e(t.charAt(r+3)),n((16711680&s)>>16),n((65280&s)>>8),n(255&s);return 2===a?(s=e(t.charAt(r))<<2|e(t.charAt(r+1))>>4,n(255&s)):1===a&&(s=e(t.charAt(r))<<10|e(t.charAt(r+1))<<4|e(t.charAt(r+2))>>2,n(s>>8&255),n(255&s)),c}function i(t){function e(t){return r.charAt(t)}function n(t){return e(t>>18&63)+e(t>>12&63)+e(t>>6&63)+e(63&t)}var i,o,u,s=t.length%3,a="";for(i=0,u=t.length-s;u>i;i+=3)o=(t[i]<<16)+(t[i+1]<<8)+t[i+2],a+=n(o);switch(s){case 1:o=t[t.length-1],a+=e(o>>2),a+=e(o<<4&63),a+="==";break;case 2:o=(t[t.length-2]<<8)+t[t.length-1],a+=e(o>>10),a+=e(o>>4&63),a+=e(o<<2&63),a+="="}return a}var o="undefined"!=typeof Uint8Array?Uint8Array:Array,u="+".charCodeAt(0),s="/".charCodeAt(0),a="0".charCodeAt(0),c="a".charCodeAt(0),f="A".charCodeAt(0);t.toByteArray=n,t.fromByteArray=i}("undefined"==typeof n?this.base64js={}:n)},{}],12:[function(t,e,n){n.read=function(t,e,n,r,i){var o,u,s=8*i-r-1,a=(1<<s)-1,c=a>>1,f=-7,l=n?i-1:0,h=n?-1:1,p=t[e+l];for(l+=h,o=p&(1<<-f)-1,p>>=-f,f+=s;f>0;o=256*o+t[e+l],l+=h,f-=8);for(u=o&(1<<-f)-1,o>>=-f,f+=r;f>0;u=256*u+t[e+l],l+=h,f-=8);if(0===o)o=1-c;else{if(o===a)return u?0/0:1/0*(p?-1:1);u+=Math.pow(2,r),o-=c}return(p?-1:1)*u*Math.pow(2,o-r)},n.write=function(t,e,n,r,i,o){var u,s,a,c=8*o-i-1,f=(1<<c)-1,l=f>>1,h=23===i?Math.pow(2,-24)-Math.pow(2,-77):0,p=r?0:o-1,d=r?1:-1,g=0>e||0===e&&0>1/e?1:0;for(e=Math.abs(e),isNaN(e)||1/0===e?(s=isNaN(e)?1:0,u=f):(u=Math.floor(Math.log(e)/Math.LN2),e*(a=Math.pow(2,-u))<1&&(u--,a*=2),e+=u+l>=1?h/a:h*Math.pow(2,1-l),e*a>=2&&(u++,a/=2),u+l>=f?(s=0,u=f):u+l>=1?(s=(e*a-1)*Math.pow(2,i),u+=l):(s=e*Math.pow(2,l-1)*Math.pow(2,i),u=0));i>=8;t[n+p]=255&s,p+=d,s/=256,i-=8);for(u=u<<i|s,c+=i;c>0;t[n+p]=255&u,p+=d,u/=256,c-=8);t[n+p-d]|=128*g}},{}],13:[function(t,e){(function(n){function r(t){return function(){var e=[],r={update:function(t,r){return n.isBuffer(t)||(t=new n(t,r)),e.push(t),this},digest:function(r){var i=n.concat(e),o=t(i);return e=null,r?o.toString(r):o}};return r}}var i=t("sha.js"),o=r(t("./md5")),u=r(t("ripemd160"));e.exports=function(t){return"md5"===t?new o:"rmd160"===t?new u:i(t)}}).call(this,t("buffer").Buffer)},{"./md5":17,buffer:10,ripemd160:18,"sha.js":20}],14:[function(t,e){(function(n){function r(t,e){if(!(this instanceof r))return new r(t,e);this._opad=a,this._alg=t,e=this._key=n.isBuffer(e)?e:new n(e),e.length>o?e=i(t).update(e).digest():e.length<o&&(e=n.concat([e,u],o));for(var s=this._ipad=new n(o),a=this._opad=new n(o),c=0;o>c;c++)s[c]=54^e[c],a[c]=92^e[c];this._hash=i(t).update(s)}var i=t("./create-hash"),o=64,u=new n(o);u.fill(0),e.exports=r,r.prototype.update=function(t,e){return this._hash.update(t,e),this},r.prototype.digest=function(t){var e=this._hash.digest();return i(this._alg).update(this._opad).update(e).digest(t)}}).call(this,t("buffer").Buffer)},{"./create-hash":13,buffer:10}],15:[function(t,e){(function(t){function n(e,n){if(e.length%o!==0){var r=e.length+(o-e.length%o);e=t.concat([e,u],r)}for(var i=[],s=n?e.readInt32BE:e.readInt32LE,a=0;a<e.length;a+=o)i.push(s.call(e,a));return i}function r(e,n,r){for(var i=new t(n),o=r?i.writeInt32BE:i.writeInt32LE,u=0;u<e.length;u++)o.call(i,e[u],4*u,!0);return i}function i(e,i,o,u){t.isBuffer(e)||(e=new t(e));var a=i(n(e,u),e.length*s);return r(a,o,u)}var o=4,u=new t(o);u.fill(0);var s=8;e.exports={hash:i}}).call(this,t("buffer").Buffer)},{buffer:10}],16:[function(t,e,n){(function(e){function r(){var t=[].slice.call(arguments).join(" ");throw new Error([t,"we accept pull requests","http://github.com/dominictarr/crypto-browserify"].join("\n"))}function i(t,e){for(var n in t)e(t[n],n)}var o=t("./rng");n.createHash=t("./create-hash"),n.createHmac=t("./create-hmac"),n.randomBytes=function(t,n){if(!n||!n.call)return new e(o(t));try{n.call(this,void 0,new e(o(t)))}catch(r){n(r)}},n.getHashes=function(){return["sha1","sha256","md5","rmd160"]};var u=t("./pbkdf2")(n.createHmac);n.pbkdf2=u.pbkdf2,n.pbkdf2Sync=u.pbkdf2Sync,i(["createCredentials","createCipher","createCipheriv","createDecipher","createDecipheriv","createSign","createVerify","createDiffieHellman"],function(t){n[t]=function(){r("sorry,",t,"is not implemented yet")}})}).call(this,t("buffer").Buffer)},{"./create-hash":13,"./create-hmac":14,"./pbkdf2":24,"./rng":25,buffer:10}],17:[function(t,e){function n(t,e){t[e>>5]|=128<<e%32,t[(e+64>>>9<<4)+14]=e;for(var n=1732584193,r=-271733879,c=-1732584194,f=271733878,l=0;l<t.length;l+=16){var h=n,p=r,d=c,g=f;n=i(n,r,c,f,t[l+0],7,-680876936),f=i(f,n,r,c,t[l+1],12,-389564586),c=i(c,f,n,r,t[l+2],17,606105819),r=i(r,c,f,n,t[l+3],22,-1044525330),n=i(n,r,c,f,t[l+4],7,-176418897),f=i(f,n,r,c,t[l+5],12,1200080426),c=i(c,f,n,r,t[l+6],17,-1473231341),r=i(r,c,f,n,t[l+7],22,-45705983),n=i(n,r,c,f,t[l+8],7,1770035416),f=i(f,n,r,c,t[l+9],12,-1958414417),c=i(c,f,n,r,t[l+10],17,-42063),r=i(r,c,f,n,t[l+11],22,-1990404162),n=i(n,r,c,f,t[l+12],7,1804603682),f=i(f,n,r,c,t[l+13],12,-40341101),c=i(c,f,n,r,t[l+14],17,-1502002290),r=i(r,c,f,n,t[l+15],22,1236535329),n=o(n,r,c,f,t[l+1],5,-165796510),f=o(f,n,r,c,t[l+6],9,-1069501632),c=o(c,f,n,r,t[l+11],14,643717713),r=o(r,c,f,n,t[l+0],20,-373897302),n=o(n,r,c,f,t[l+5],5,-701558691),f=o(f,n,r,c,t[l+10],9,38016083),c=o(c,f,n,r,t[l+15],14,-660478335),r=o(r,c,f,n,t[l+4],20,-405537848),n=o(n,r,c,f,t[l+9],5,568446438),f=o(f,n,r,c,t[l+14],9,-1019803690),c=o(c,f,n,r,t[l+3],14,-187363961),r=o(r,c,f,n,t[l+8],20,1163531501),n=o(n,r,c,f,t[l+13],5,-1444681467),f=o(f,n,r,c,t[l+2],9,-51403784),c=o(c,f,n,r,t[l+7],14,1735328473),r=o(r,c,f,n,t[l+12],20,-1926607734),n=u(n,r,c,f,t[l+5],4,-378558),f=u(f,n,r,c,t[l+8],11,-2022574463),c=u(c,f,n,r,t[l+11],16,1839030562),r=u(r,c,f,n,t[l+14],23,-35309556),n=u(n,r,c,f,t[l+1],4,-1530992060),f=u(f,n,r,c,t[l+4],11,1272893353),c=u(c,f,n,r,t[l+7],16,-155497632),r=u(r,c,f,n,t[l+10],23,-1094730640),n=u(n,r,c,f,t[l+13],4,681279174),f=u(f,n,r,c,t[l+0],11,-358537222),c=u(c,f,n,r,t[l+3],16,-722521979),r=u(r,c,f,n,t[l+6],23,76029189),n=u(n,r,c,f,t[l+9],4,-640364487),f=u(f,n,r,c,t[l+12],11,-421815835),c=u(c,f,n,r,t[l+15],16,530742520),r=u(r,c,f,n,t[l+2],23,-995338651),n=s(n,r,c,f,t[l+0],6,-198630844),f=s(f,n,r,c,t[l+7],10,1126891415),c=s(c,f,n,r,t[l+14],15,-1416354905),r=s(r,c,f,n,t[l+5],21,-57434055),n=s(n,r,c,f,t[l+12],6,1700485571),f=s(f,n,r,c,t[l+3],10,-1894986606),c=s(c,f,n,r,t[l+10],15,-1051523),r=s(r,c,f,n,t[l+1],21,-2054922799),n=s(n,r,c,f,t[l+8],6,1873313359),f=s(f,n,r,c,t[l+15],10,-30611744),c=s(c,f,n,r,t[l+6],15,-1560198380),r=s(r,c,f,n,t[l+13],21,1309151649),n=s(n,r,c,f,t[l+4],6,-145523070),f=s(f,n,r,c,t[l+11],10,-1120210379),c=s(c,f,n,r,t[l+2],15,718787259),r=s(r,c,f,n,t[l+9],21,-343485551),n=a(n,h),r=a(r,p),c=a(c,d),f=a(f,g)}return Array(n,r,c,f)}function r(t,e,n,r,i,o){return a(c(a(a(e,t),a(r,o)),i),n)}function i(t,e,n,i,o,u,s){return r(e&n|~e&i,t,e,o,u,s)}function o(t,e,n,i,o,u,s){return r(e&i|n&~i,t,e,o,u,s)}function u(t,e,n,i,o,u,s){return r(e^n^i,t,e,o,u,s)}function s(t,e,n,i,o,u,s){return r(n^(e|~i),t,e,o,u,s)}function a(t,e){var n=(65535&t)+(65535&e),r=(t>>16)+(e>>16)+(n>>16);return r<<16|65535&n}function c(t,e){return t<<e|t>>>32-e}var f=t("./helpers");e.exports=function(t){return f.hash(t,n,16)}},{"./helpers":15}],18:[function(t,e){(function(t){function n(t,e,n){return t^e^n}function r(t,e,n){return t&e|~t&n}function i(t,e,n){return(t|~e)^n}function o(t,e,n){return t&n|e&~n}function u(t,e,n){return t^(e|~n)}function s(t,e){return t<<e|t>>>32-e}function a(e){var n=[1732584193,4023233417,2562383102,271733878,3285377520];"string"==typeof e&&(e=new t(e,"utf8"));var r=g(e),i=8*e.length,o=8*e.length;r[i>>>5]|=128<<24-i%32,r[(i+64>>>9<<4)+14]=16711935&(o<<8|o>>>24)|4278255360&(o<<24|o>>>8);for(var u=0;u<r.length;u+=16)b(n,r,u);for(var u=0;5>u;u++){var s=n[u];n[u]=16711935&(s<<8|s>>>24)|4278255360&(s<<24|s>>>8)}var a=y(n);return new t(a)}e.exports=a;/** @preserve | ||
var H=t("base64-js"),J=t("ieee754");n.Buffer=r,n.SlowBuffer=r,n.INSPECT_MAX_BYTES=50,r.poolSize=8192;var V=function(){try{var t=new ArrayBuffer(0),e=new Uint8Array(t);return e.foo=function(){return 42},42===e.foo()&&"function"==typeof e.subarray&&0===new Uint8Array(1).subarray(1,1).byteLength}catch(n){return!1}}();r.isEncoding=function(t){switch(String(t).toLowerCase()){case"hex":case"utf8":case"utf-8":case"ascii":case"binary":case"base64":case"raw":case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":return!0;default:return!1}},r.isBuffer=function(t){return!(null==t||!t._isBuffer)},r.byteLength=function(t,e){var n;switch(t=t.toString(),e||"utf8"){case"hex":n=t.length/2;break;case"utf8":case"utf-8":n=O(t).length;break;case"ascii":case"binary":case"raw":n=t.length;break;case"base64":n=M(t).length;break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":n=2*t.length;break;default:throw new Error("Unknown encoding")}return n},r.concat=function(t,e){if(R(L(t),"Usage: Buffer.concat(list[, length])"),0===t.length)return new r(0);if(1===t.length)return t[0];var n;if(void 0===e)for(e=0,n=0;n<t.length;n++)e+=t[n].length;var i=new r(e),o=0;for(n=0;n<t.length;n++){var s=t[n];s.copy(i,o),o+=s.length}return i},r.compare=function(t,e){R(r.isBuffer(t)&&r.isBuffer(e),"Arguments must be Buffers");for(var n=t.length,i=e.length,o=0,s=Math.min(n,i);s>o&&t[o]===e[o];o++);return o!==s&&(n=t[o],i=e[o]),i>n?-1:n>i?1:0},r.prototype.write=function(t,e,n,r){if(isFinite(e))isFinite(n)||(r=n,n=void 0);else{var f=r;r=e,e=n,n=f}e=Number(e)||0;var l=this.length-e;n?(n=Number(n),n>l&&(n=l)):n=l,r=String(r||"utf8").toLowerCase();var h;switch(r){case"hex":h=i(this,t,e,n);break;case"utf8":case"utf-8":h=o(this,t,e,n);break;case"ascii":h=s(this,t,e,n);break;case"binary":h=u(this,t,e,n);break;case"base64":h=a(this,t,e,n);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":h=c(this,t,e,n);break;default:throw new Error("Unknown encoding")}return h},r.prototype.toString=function(t,e,n){var r=this;if(t=String(t||"utf8").toLowerCase(),e=Number(e)||0,n=void 0===n?r.length:Number(n),n===e)return"";var i;switch(t){case"hex":i=d(r,e,n);break;case"utf8":case"utf-8":i=l(r,e,n);break;case"ascii":i=h(r,e,n);break;case"binary":i=p(r,e,n);break;case"base64":i=f(r,e,n);break;case"ucs2":case"ucs-2":case"utf16le":case"utf-16le":i=g(r,e,n);break;default:throw new Error("Unknown encoding")}return i},r.prototype.toJSON=function(){return{type:"Buffer",data:Array.prototype.slice.call(this._arr||this,0)}},r.prototype.equals=function(t){return R(r.isBuffer(t),"Argument must be a Buffer"),0===r.compare(this,t)},r.prototype.compare=function(t){return R(r.isBuffer(t),"Argument must be a Buffer"),r.compare(this,t)},r.prototype.copy=function(t,e,n,r){var i=this;if(n||(n=0),r||0===r||(r=this.length),e||(e=0),r!==n&&0!==t.length&&0!==i.length){R(r>=n,"sourceEnd < sourceStart"),R(e>=0&&e<t.length,"targetStart out of bounds"),R(n>=0&&n<i.length,"sourceStart out of bounds"),R(r>=0&&r<=i.length,"sourceEnd out of bounds"),r>this.length&&(r=this.length),t.length-e<r-n&&(r=t.length-e+n);var o=r-n;if(100>o||!V)for(var s=0;o>s;s++)t[s+e]=this[s+n];else t._set(this.subarray(n,n+o),e)}},r.prototype.slice=function(t,e){var n=this.length;if(t=~~t,e=void 0===e?n:~~e,0>t?(t+=n,0>t&&(t=0)):t>n&&(t=n),0>e?(e+=n,0>e&&(e=0)):e>n&&(e=n),t>e&&(e=t),V)return r._augment(this.subarray(t,e));for(var i=e-t,o=new r(i,void 0,!0),s=0;i>s;s++)o[s]=this[s+t];return o},r.prototype.get=function(t){return console.log(".get() is deprecated. Access using array indexes instead."),this.readUInt8(t)},r.prototype.set=function(t,e){return console.log(".set() is deprecated. Access using array indexes instead."),this.writeUInt8(t,e)},r.prototype.readUInt8=function(t,e){return e||(R(void 0!==t&&null!==t,"missing offset"),R(t<this.length,"Trying to read beyond buffer length")),t>=this.length?void 0:this[t]},r.prototype.readUInt16LE=function(t,e){return y(this,t,!0,e)},r.prototype.readUInt16BE=function(t,e){return y(this,t,!1,e)},r.prototype.readUInt32LE=function(t,e){return b(this,t,!0,e)},r.prototype.readUInt32BE=function(t,e){return b(this,t,!1,e)},r.prototype.readInt8=function(t,e){if(e||(R(void 0!==t&&null!==t,"missing offset"),R(t<this.length,"Trying to read beyond buffer length")),!(t>=this.length)){var n=128&this[t];return n?-1*(255-this[t]+1):this[t]}},r.prototype.readInt16LE=function(t,e){return v(this,t,!0,e)},r.prototype.readInt16BE=function(t,e){return v(this,t,!1,e)},r.prototype.readInt32LE=function(t,e){return w(this,t,!0,e)},r.prototype.readInt32BE=function(t,e){return w(this,t,!1,e)},r.prototype.readFloatLE=function(t,e){return m(this,t,!0,e)},r.prototype.readFloatBE=function(t,e){return m(this,t,!1,e)},r.prototype.readDoubleLE=function(t,e){return _(this,t,!0,e)},r.prototype.readDoubleBE=function(t,e){return _(this,t,!1,e)},r.prototype.writeUInt8=function(t,e,n){return n||(R(void 0!==t&&null!==t,"missing value"),R(void 0!==e&&null!==e,"missing offset"),R(e<this.length,"trying to write beyond buffer length"),F(t,255)),e>=this.length?void 0:(this[e]=t,e+1)},r.prototype.writeUInt16LE=function(t,e,n){return E(this,t,e,!0,n)},r.prototype.writeUInt16BE=function(t,e,n){return E(this,t,e,!1,n)},r.prototype.writeUInt32LE=function(t,e,n){return B(this,t,e,!0,n)},r.prototype.writeUInt32BE=function(t,e,n){return B(this,t,e,!1,n)},r.prototype.writeInt8=function(t,e,n){return n||(R(void 0!==t&&null!==t,"missing value"),R(void 0!==e&&null!==e,"missing offset"),R(e<this.length,"Trying to write beyond buffer length"),z(t,127,-128)),e>=this.length?void 0:(t>=0?this.writeUInt8(t,e,n):this.writeUInt8(255+t+1,e,n),e+1)},r.prototype.writeInt16LE=function(t,e,n){return k(this,t,e,!0,n)},r.prototype.writeInt16BE=function(t,e,n){return k(this,t,e,!1,n)},r.prototype.writeInt32LE=function(t,e,n){return I(this,t,e,!0,n)},r.prototype.writeInt32BE=function(t,e,n){return I(this,t,e,!1,n)},r.prototype.writeFloatLE=function(t,e,n){return x(this,t,e,!0,n)},r.prototype.writeFloatBE=function(t,e,n){return x(this,t,e,!1,n)},r.prototype.writeDoubleLE=function(t,e,n){return S(this,t,e,!0,n)},r.prototype.writeDoubleBE=function(t,e,n){return S(this,t,e,!1,n)},r.prototype.fill=function(t,e,n){if(t||(t=0),e||(e=0),n||(n=this.length),R(n>=e,"end < start"),n!==e&&0!==this.length){R(e>=0&&e<this.length,"start out of bounds"),R(n>=0&&n<=this.length,"end out of bounds");var r;if("number"==typeof t)for(r=e;n>r;r++)this[r]=t;else{var i=O(t.toString()),o=i.length;for(r=e;n>r;r++)this[r]=i[r%o]}return this}},r.prototype.inspect=function(){for(var t=[],e=this.length,r=0;e>r;r++)if(t[r]=U(this[r]),r===n.INSPECT_MAX_BYTES){t[r+1]="...";break}return"<Buffer "+t.join(" ")+">"},r.prototype.toArrayBuffer=function(){if("undefined"!=typeof Uint8Array){if(V)return new r(this).buffer;for(var t=new Uint8Array(this.length),e=0,n=t.length;n>e;e+=1)t[e]=this[e];return t.buffer}throw new Error("Buffer.toArrayBuffer not supported in this browser")};var Y=r.prototype;r._augment=function(t){return t._isBuffer=!0,t._get=t.get,t._set=t.set,t.get=Y.get,t.set=Y.set,t.write=Y.write,t.toString=Y.toString,t.toLocaleString=Y.toString,t.toJSON=Y.toJSON,t.equals=Y.equals,t.compare=Y.compare,t.copy=Y.copy,t.slice=Y.slice,t.readUInt8=Y.readUInt8,t.readUInt16LE=Y.readUInt16LE,t.readUInt16BE=Y.readUInt16BE,t.readUInt32LE=Y.readUInt32LE,t.readUInt32BE=Y.readUInt32BE,t.readInt8=Y.readInt8,t.readInt16LE=Y.readInt16LE,t.readInt16BE=Y.readInt16BE,t.readInt32LE=Y.readInt32LE,t.readInt32BE=Y.readInt32BE,t.readFloatLE=Y.readFloatLE,t.readFloatBE=Y.readFloatBE,t.readDoubleLE=Y.readDoubleLE,t.readDoubleBE=Y.readDoubleBE,t.writeUInt8=Y.writeUInt8,t.writeUInt16LE=Y.writeUInt16LE,t.writeUInt16BE=Y.writeUInt16BE,t.writeUInt32LE=Y.writeUInt32LE,t.writeUInt32BE=Y.writeUInt32BE,t.writeInt8=Y.writeInt8,t.writeInt16LE=Y.writeInt16LE,t.writeInt16BE=Y.writeInt16BE,t.writeInt32LE=Y.writeInt32LE,t.writeInt32BE=Y.writeInt32BE,t.writeFloatLE=Y.writeFloatLE,t.writeFloatBE=Y.writeFloatBE,t.writeDoubleLE=Y.writeDoubleLE,t.writeDoubleBE=Y.writeDoubleBE,t.fill=Y.fill,t.inspect=Y.inspect,t.toArrayBuffer=Y.toArrayBuffer,t};var $=/[^+\/0-9A-z]/g},{"base64-js":14,ieee754:15}],14:[function(t,e,n){var r="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";!function(t){"use strict";function e(t){var e=t.charCodeAt(0);return e===s?62:e===u?63:a>e?-1:a+10>e?e-a+26+26:f+26>e?e-f:c+26>e?e-c+26:void 0}function n(t){function n(t){c[l++]=t}var r,i,s,u,a,c;if(t.length%4>0)throw new Error("Invalid string. Length must be a multiple of 4");var f=t.length;a="="===t.charAt(f-2)?2:"="===t.charAt(f-1)?1:0,c=new o(3*t.length/4-a),s=a>0?t.length-4:t.length;var l=0;for(r=0,i=0;s>r;r+=4,i+=3)u=e(t.charAt(r))<<18|e(t.charAt(r+1))<<12|e(t.charAt(r+2))<<6|e(t.charAt(r+3)),n((16711680&u)>>16),n((65280&u)>>8),n(255&u);return 2===a?(u=e(t.charAt(r))<<2|e(t.charAt(r+1))>>4,n(255&u)):1===a&&(u=e(t.charAt(r))<<10|e(t.charAt(r+1))<<4|e(t.charAt(r+2))>>2,n(u>>8&255),n(255&u)),c}function i(t){function e(t){return r.charAt(t)}function n(t){return e(t>>18&63)+e(t>>12&63)+e(t>>6&63)+e(63&t)}var i,o,s,u=t.length%3,a="";for(i=0,s=t.length-u;s>i;i+=3)o=(t[i]<<16)+(t[i+1]<<8)+t[i+2],a+=n(o);switch(u){case 1:o=t[t.length-1],a+=e(o>>2),a+=e(o<<4&63),a+="==";break;case 2:o=(t[t.length-2]<<8)+t[t.length-1],a+=e(o>>10),a+=e(o>>4&63),a+=e(o<<2&63),a+="="}return a}var o="undefined"!=typeof Uint8Array?Uint8Array:Array,s="+".charCodeAt(0),u="/".charCodeAt(0),a="0".charCodeAt(0),c="a".charCodeAt(0),f="A".charCodeAt(0);t.toByteArray=n,t.fromByteArray=i}("undefined"==typeof n?this.base64js={}:n)},{}],15:[function(t,e,n){n.read=function(t,e,n,r,i){var o,s,u=8*i-r-1,a=(1<<u)-1,c=a>>1,f=-7,l=n?i-1:0,h=n?-1:1,p=t[e+l];for(l+=h,o=p&(1<<-f)-1,p>>=-f,f+=u;f>0;o=256*o+t[e+l],l+=h,f-=8);for(s=o&(1<<-f)-1,o>>=-f,f+=r;f>0;s=256*s+t[e+l],l+=h,f-=8);if(0===o)o=1-c;else{if(o===a)return s?0/0:1/0*(p?-1:1);s+=Math.pow(2,r),o-=c}return(p?-1:1)*s*Math.pow(2,o-r)},n.write=function(t,e,n,r,i,o){var s,u,a,c=8*o-i-1,f=(1<<c)-1,l=f>>1,h=23===i?Math.pow(2,-24)-Math.pow(2,-77):0,p=r?0:o-1,d=r?1:-1,g=0>e||0===e&&0>1/e?1:0;for(e=Math.abs(e),isNaN(e)||1/0===e?(u=isNaN(e)?1:0,s=f):(s=Math.floor(Math.log(e)/Math.LN2),e*(a=Math.pow(2,-s))<1&&(s--,a*=2),e+=s+l>=1?h/a:h*Math.pow(2,1-l),e*a>=2&&(s++,a/=2),s+l>=f?(u=0,s=f):s+l>=1?(u=(e*a-1)*Math.pow(2,i),s+=l):(u=e*Math.pow(2,l-1)*Math.pow(2,i),s=0));i>=8;t[n+p]=255&u,p+=d,u/=256,i-=8);for(s=s<<i|u,c+=i;c>0;t[n+p]=255&s,p+=d,s/=256,c-=8);t[n+p-d]|=128*g}},{}],16:[function(t,e){(function(n){function r(t){return function(){var e=[],r={update:function(t,r){return n.isBuffer(t)||(t=new n(t,r)),e.push(t),this},digest:function(r){var i=n.concat(e),o=t(i);return e=null,r?o.toString(r):o}};return r}}var i=t("sha.js"),o=r(t("./md5")),s=r(t("ripemd160"));e.exports=function(t){return"md5"===t?new o:"rmd160"===t?new s:i(t)}}).call(this,t("buffer").Buffer)},{"./md5":20,buffer:13,ripemd160:21,"sha.js":23}],17:[function(t,e){(function(n){function r(t,e){if(!(this instanceof r))return new r(t,e);this._opad=a,this._alg=t,e=this._key=n.isBuffer(e)?e:new n(e),e.length>o?e=i(t).update(e).digest():e.length<o&&(e=n.concat([e,s],o));for(var u=this._ipad=new n(o),a=this._opad=new n(o),c=0;o>c;c++)u[c]=54^e[c],a[c]=92^e[c];this._hash=i(t).update(u)}var i=t("./create-hash"),o=64,s=new n(o);s.fill(0),e.exports=r,r.prototype.update=function(t,e){return this._hash.update(t,e),this},r.prototype.digest=function(t){var e=this._hash.digest();return i(this._alg).update(this._opad).update(e).digest(t)}}).call(this,t("buffer").Buffer)},{"./create-hash":16,buffer:13}],18:[function(t,e){(function(t){function n(e,n){if(e.length%o!==0){var r=e.length+(o-e.length%o);e=t.concat([e,s],r)}for(var i=[],u=n?e.readInt32BE:e.readInt32LE,a=0;a<e.length;a+=o)i.push(u.call(e,a));return i}function r(e,n,r){for(var i=new t(n),o=r?i.writeInt32BE:i.writeInt32LE,s=0;s<e.length;s++)o.call(i,e[s],4*s,!0);return i}function i(e,i,o,s){t.isBuffer(e)||(e=new t(e));var a=i(n(e,s),e.length*u);return r(a,o,s)}var o=4,s=new t(o);s.fill(0);var u=8;e.exports={hash:i}}).call(this,t("buffer").Buffer)},{buffer:13}],19:[function(t,e,n){(function(e){function r(){var t=[].slice.call(arguments).join(" ");throw new Error([t,"we accept pull requests","http://github.com/dominictarr/crypto-browserify"].join("\n"))}function i(t,e){for(var n in t)e(t[n],n)}var o=t("./rng");n.createHash=t("./create-hash"),n.createHmac=t("./create-hmac"),n.randomBytes=function(t,n){if(!n||!n.call)return new e(o(t));try{n.call(this,void 0,new e(o(t)))}catch(r){n(r)}},n.getHashes=function(){return["sha1","sha256","md5","rmd160"]};var s=t("./pbkdf2")(n.createHmac);n.pbkdf2=s.pbkdf2,n.pbkdf2Sync=s.pbkdf2Sync,i(["createCredentials","createCipher","createCipheriv","createDecipher","createDecipheriv","createSign","createVerify","createDiffieHellman"],function(t){n[t]=function(){r("sorry,",t,"is not implemented yet")}})}).call(this,t("buffer").Buffer)},{"./create-hash":16,"./create-hmac":17,"./pbkdf2":27,"./rng":28,buffer:13}],20:[function(t,e){function n(t,e){t[e>>5]|=128<<e%32,t[(e+64>>>9<<4)+14]=e;for(var n=1732584193,r=-271733879,c=-1732584194,f=271733878,l=0;l<t.length;l+=16){var h=n,p=r,d=c,g=f;n=i(n,r,c,f,t[l+0],7,-680876936),f=i(f,n,r,c,t[l+1],12,-389564586),c=i(c,f,n,r,t[l+2],17,606105819),r=i(r,c,f,n,t[l+3],22,-1044525330),n=i(n,r,c,f,t[l+4],7,-176418897),f=i(f,n,r,c,t[l+5],12,1200080426),c=i(c,f,n,r,t[l+6],17,-1473231341),r=i(r,c,f,n,t[l+7],22,-45705983),n=i(n,r,c,f,t[l+8],7,1770035416),f=i(f,n,r,c,t[l+9],12,-1958414417),c=i(c,f,n,r,t[l+10],17,-42063),r=i(r,c,f,n,t[l+11],22,-1990404162),n=i(n,r,c,f,t[l+12],7,1804603682),f=i(f,n,r,c,t[l+13],12,-40341101),c=i(c,f,n,r,t[l+14],17,-1502002290),r=i(r,c,f,n,t[l+15],22,1236535329),n=o(n,r,c,f,t[l+1],5,-165796510),f=o(f,n,r,c,t[l+6],9,-1069501632),c=o(c,f,n,r,t[l+11],14,643717713),r=o(r,c,f,n,t[l+0],20,-373897302),n=o(n,r,c,f,t[l+5],5,-701558691),f=o(f,n,r,c,t[l+10],9,38016083),c=o(c,f,n,r,t[l+15],14,-660478335),r=o(r,c,f,n,t[l+4],20,-405537848),n=o(n,r,c,f,t[l+9],5,568446438),f=o(f,n,r,c,t[l+14],9,-1019803690),c=o(c,f,n,r,t[l+3],14,-187363961),r=o(r,c,f,n,t[l+8],20,1163531501),n=o(n,r,c,f,t[l+13],5,-1444681467),f=o(f,n,r,c,t[l+2],9,-51403784),c=o(c,f,n,r,t[l+7],14,1735328473),r=o(r,c,f,n,t[l+12],20,-1926607734),n=s(n,r,c,f,t[l+5],4,-378558),f=s(f,n,r,c,t[l+8],11,-2022574463),c=s(c,f,n,r,t[l+11],16,1839030562),r=s(r,c,f,n,t[l+14],23,-35309556),n=s(n,r,c,f,t[l+1],4,-1530992060),f=s(f,n,r,c,t[l+4],11,1272893353),c=s(c,f,n,r,t[l+7],16,-155497632),r=s(r,c,f,n,t[l+10],23,-1094730640),n=s(n,r,c,f,t[l+13],4,681279174),f=s(f,n,r,c,t[l+0],11,-358537222),c=s(c,f,n,r,t[l+3],16,-722521979),r=s(r,c,f,n,t[l+6],23,76029189),n=s(n,r,c,f,t[l+9],4,-640364487),f=s(f,n,r,c,t[l+12],11,-421815835),c=s(c,f,n,r,t[l+15],16,530742520),r=s(r,c,f,n,t[l+2],23,-995338651),n=u(n,r,c,f,t[l+0],6,-198630844),f=u(f,n,r,c,t[l+7],10,1126891415),c=u(c,f,n,r,t[l+14],15,-1416354905),r=u(r,c,f,n,t[l+5],21,-57434055),n=u(n,r,c,f,t[l+12],6,1700485571),f=u(f,n,r,c,t[l+3],10,-1894986606),c=u(c,f,n,r,t[l+10],15,-1051523),r=u(r,c,f,n,t[l+1],21,-2054922799),n=u(n,r,c,f,t[l+8],6,1873313359),f=u(f,n,r,c,t[l+15],10,-30611744),c=u(c,f,n,r,t[l+6],15,-1560198380),r=u(r,c,f,n,t[l+13],21,1309151649),n=u(n,r,c,f,t[l+4],6,-145523070),f=u(f,n,r,c,t[l+11],10,-1120210379),c=u(c,f,n,r,t[l+2],15,718787259),r=u(r,c,f,n,t[l+9],21,-343485551),n=a(n,h),r=a(r,p),c=a(c,d),f=a(f,g)}return Array(n,r,c,f)}function r(t,e,n,r,i,o){return a(c(a(a(e,t),a(r,o)),i),n)}function i(t,e,n,i,o,s,u){return r(e&n|~e&i,t,e,o,s,u)}function o(t,e,n,i,o,s,u){return r(e&i|n&~i,t,e,o,s,u)}function s(t,e,n,i,o,s,u){return r(e^n^i,t,e,o,s,u)}function u(t,e,n,i,o,s,u){return r(n^(e|~i),t,e,o,s,u)}function a(t,e){var n=(65535&t)+(65535&e),r=(t>>16)+(e>>16)+(n>>16);return r<<16|65535&n}function c(t,e){return t<<e|t>>>32-e}var f=t("./helpers");e.exports=function(t){return f.hash(t,n,16)}},{"./helpers":18}],21:[function(t,e){(function(t){function n(t,e,n){return t^e^n}function r(t,e,n){return t&e|~t&n}function i(t,e,n){return(t|~e)^n}function o(t,e,n){return t&n|e&~n}function s(t,e,n){return t^(e|~n)}function u(t,e){return t<<e|t>>>32-e}function a(e){var n=[1732584193,4023233417,2562383102,271733878,3285377520];"string"==typeof e&&(e=new t(e,"utf8"));var r=g(e),i=8*e.length,o=8*e.length;r[i>>>5]|=128<<24-i%32,r[(i+64>>>9<<4)+14]=16711935&(o<<8|o>>>24)|4278255360&(o<<24|o>>>8);for(var s=0;s<r.length;s+=16)b(n,r,s);for(var s=0;5>s;s++){var u=n[s];n[s]=16711935&(u<<8|u>>>24)|4278255360&(u<<24|u>>>8)}var a=y(n);return new t(a)}e.exports=a;/** @preserve | ||
(c) 2012 by Cédric Mesnil. All rights reserved. | ||
@@ -18,3 +18,3 @@ | ||
*/ | ||
var c=[0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,7,4,13,1,10,6,15,3,12,0,9,5,2,14,11,8,3,10,14,4,9,15,8,1,2,7,0,6,13,11,5,12,1,9,11,10,0,8,12,4,13,3,7,15,14,5,6,2,4,0,5,9,7,12,2,10,14,1,3,8,11,6,15,13],f=[5,14,7,0,9,2,11,4,13,6,15,8,1,10,3,12,6,11,3,7,0,13,5,10,14,15,8,12,4,9,1,2,15,5,1,3,7,14,6,9,11,8,12,2,10,0,4,13,8,6,4,1,3,11,15,0,5,12,2,13,9,7,10,14,12,15,10,4,1,5,8,7,6,2,13,14,0,3,9,11],l=[11,14,15,12,5,8,7,9,11,13,14,15,6,7,9,8,7,6,8,13,11,9,7,15,7,12,15,9,11,7,13,12,11,13,6,7,14,9,13,15,14,8,13,6,5,12,7,5,11,12,14,15,14,15,9,8,9,14,5,6,8,6,5,12,9,15,5,11,6,8,13,12,5,12,13,14,11,8,5,6],h=[8,9,9,11,13,15,15,5,7,7,8,11,14,14,12,6,9,13,15,7,12,8,9,11,7,7,12,7,6,15,13,11,9,7,15,11,8,6,6,14,12,13,5,14,13,13,7,5,15,5,8,11,14,14,6,14,6,9,12,9,12,5,15,8,8,5,12,9,12,5,14,6,8,13,6,5,15,13,11,11],p=[0,1518500249,1859775393,2400959708,2840853838],d=[1352829926,1548603684,1836072691,2053994217,0],g=function(t){for(var e=[],n=0,r=0;n<t.length;n++,r+=8)e[r>>>5]|=t[n]<<24-r%32;return e},y=function(t){for(var e=[],n=0;n<32*t.length;n+=8)e.push(t[n>>>5]>>>24-n%32&255);return e},b=function(t,e,a){for(var g=0;16>g;g++){var y=a+g,b=e[y];e[y]=16711935&(b<<8|b>>>24)|4278255360&(b<<24|b>>>8)}var v,w,m,_,E,B,k,I,x,S;B=v=t[0],k=w=t[1],I=m=t[2],x=_=t[3],S=E=t[4];for(var A,g=0;80>g;g+=1)A=v+e[a+c[g]]|0,A+=16>g?n(w,m,_)+p[0]:32>g?r(w,m,_)+p[1]:48>g?i(w,m,_)+p[2]:64>g?o(w,m,_)+p[3]:u(w,m,_)+p[4],A=0|A,A=s(A,l[g]),A=A+E|0,v=E,E=_,_=s(m,10),m=w,w=A,A=B+e[a+f[g]]|0,A+=16>g?u(k,I,x)+d[0]:32>g?o(k,I,x)+d[1]:48>g?i(k,I,x)+d[2]:64>g?r(k,I,x)+d[3]:n(k,I,x)+d[4],A=0|A,A=s(A,h[g]),A=A+S|0,B=S,S=x,x=s(I,10),I=k,k=A;A=t[1]+m+x|0,t[1]=t[2]+_+S|0,t[2]=t[3]+E+B|0,t[3]=t[4]+v+k|0,t[4]=t[0]+w+I|0,t[0]=A}}).call(this,t("buffer").Buffer)},{buffer:10}],19:[function(t,e){var n=t("./util"),r=n.write,i=n.zeroFill;e.exports=function(t){function e(e,n){this._block=new t(e),this._finalSize=n,this._blockSize=e,this._len=0,this._s=0}function o(t,e){return null==e?t.byteLength||t.length:"ascii"==e||"binary"==e?t.length:"hex"==e?t.length/2:"base64"==e?t.length/3:void 0}return e.prototype.init=function(){this._s=0,this._len=0},e.prototype.update=function(e,n){var i,u=this._blockSize;n||"string"!=typeof e||(n="utf8"),n?("utf-8"===n&&(n="utf8"),("base64"===n||"utf8"===n)&&(e=new t(e,n),n=null),i=o(e,n)):i=e.byteLength||e.length;for(var s=this._len+=i,a=this._s=this._s||0,c=0,f=this._block;s>a;){var l=Math.min(i,c+u-a%u);r(f,e,n,a%u,c,l);var h=l-c;a+=h,c+=h,a%u||this._update(f)}return this._s=a,this},e.prototype.digest=function(t){var e=this._blockSize,r=this._finalSize,o=8*this._len,u=this._block,s=o%(8*e);u[this._len%e]=128,i(this._block,this._len%e+1),s>=8*r&&(this._update(this._block),n.zeroFill(this._block,0)),u.writeInt32BE(o,r+4);var a=this._update(this._block)||this._hash();return null==t?a:a.toString(t)},e.prototype._update=function(){throw new Error("_update must be implemented by subclass")},e}},{"./util":23}],20:[function(t,e,n){var n=e.exports=function(t){var e=n[t];if(!e)throw new Error(t+" is not supported (we accept pull requests)");return new e},r=t("buffer").Buffer,i=t("./hash")(r);n.sha=n.sha1=t("./sha1")(r,i),n.sha256=t("./sha256")(r,i)},{"./hash":19,"./sha1":21,"./sha256":22,buffer:10}],21:[function(t,e){e.exports=function(e,n){function r(){return g.length?g.pop().init():this instanceof r?(this._w=d,n.call(this,64,56),this._h=null,void this.init()):new r}function i(t,e,n,r){return 20>t?e&n|~e&r:40>t?e^n^r:60>t?e&n|e&r|n&r:e^n^r}function o(t){return 20>t?1518500249:40>t?1859775393:60>t?-1894007588:-899497514}function u(t,e){return t+e|0}function s(t,e){return t<<e|t>>>32-e}var a=t("util").inherits;a(r,n);var c=0,f=4,l=8,h=12,p=16,d=new Int32Array(80),g=[];r.prototype.init=function(){return this._a=1732584193,this._b=4023233417,this._c=2562383102,this._d=271733878,this._e=3285377520,n.prototype.init.call(this),this},r.prototype._POOL=g;new e(1)instanceof DataView;return r.prototype._update=function(){{var t,e,n,r,a,c,f,l,h,p,d=this._block;this._h}t=c=this._a,e=f=this._b,n=l=this._c,r=h=this._d,a=p=this._e;for(var g=this._w,y=0;80>y;y++){var b=g[y]=16>y?d.readInt32BE(4*y):s(g[y-3]^g[y-8]^g[y-14]^g[y-16],1),v=u(u(s(t,5),i(y,e,n,r)),u(u(a,b),o(y)));a=r,r=n,n=s(e,30),e=t,t=v}this._a=u(t,c),this._b=u(e,f),this._c=u(n,l),this._d=u(r,h),this._e=u(a,p)},r.prototype._hash=function(){g.length<100&&g.push(this);var t=new e(20);return t.writeInt32BE(0|this._a,c),t.writeInt32BE(0|this._b,f),t.writeInt32BE(0|this._c,l),t.writeInt32BE(0|this._d,h),t.writeInt32BE(0|this._e,p),t},r}},{util:29}],22:[function(t,e){{var n=t("util").inherits;t("./util")}e.exports=function(t,e){function r(){d.length,this.init(),this._w=p,e.call(this,64,56)}function i(t,e){return t>>>e|t<<32-e}function o(t,e){return t>>>e}function u(t,e,n){return t&e^~t&n}function s(t,e,n){return t&e^t&n^e&n}function a(t){return i(t,2)^i(t,13)^i(t,22)}function c(t){return i(t,6)^i(t,11)^i(t,25)}function f(t){return i(t,7)^i(t,18)^o(t,3)}function l(t){return i(t,17)^i(t,19)^o(t,10)}var h=[1116352408,1899447441,3049323471,3921009573,961987163,1508970993,2453635748,2870763221,3624381080,310598401,607225278,1426881987,1925078388,2162078206,2614888103,3248222580,3835390401,4022224774,264347078,604807628,770255983,1249150122,1555081692,1996064986,2554220882,2821834349,2952996808,3210313671,3336571891,3584528711,113926993,338241895,666307205,773529912,1294757372,1396182291,1695183700,1986661051,2177026350,2456956037,2730485921,2820302411,3259730800,3345764771,3516065817,3600352804,4094571909,275423344,430227734,506948616,659060556,883997877,958139571,1322822218,1537002063,1747873779,1955562222,2024104815,2227730452,2361852424,2428436474,2756734187,3204031479,3329325298];n(r,e);var p=new Array(64),d=[];r.prototype.init=function(){return this._a=1779033703,this._b=-1150833019,this._c=1013904242,this._d=-1521486534,this._e=1359893119,this._f=-1694144372,this._g=528734635,this._h=1541459225,this._len=this._s=0,this};return r.prototype._update=function(){var t,e,n,r,i,o,p,d,g,y,b=this._block,v=this._w;t=0|this._a,e=0|this._b,n=0|this._c,r=0|this._d,i=0|this._e,o=0|this._f,p=0|this._g,d=0|this._h;for(var w=0;64>w;w++){var m=v[w]=16>w?b.readInt32BE(4*w):l(v[w-2])+v[w-7]+f(v[w-15])+v[w-16];g=d+c(i)+u(i,o,p)+h[w]+m,y=a(t)+s(t,e,n),d=p,p=o,o=i,i=r+g,r=n,n=e,e=t,t=g+y}this._a=t+this._a|0,this._b=e+this._b|0,this._c=n+this._c|0,this._d=r+this._d|0,this._e=i+this._e|0,this._f=o+this._f|0,this._g=p+this._g|0,this._h=d+this._h|0},r.prototype._hash=function(){d.length<10&&d.push(this);var e=new t(32);return e.writeInt32BE(this._a,0),e.writeInt32BE(this._b,4),e.writeInt32BE(this._c,8),e.writeInt32BE(this._d,12),e.writeInt32BE(this._e,16),e.writeInt32BE(this._f,20),e.writeInt32BE(this._g,24),e.writeInt32BE(this._h,28),e},r}},{"./util":23,util:29}],23:[function(t,e,n){function r(t,e,n,r,i,o){var u=o-i;if("ascii"===n||"binary"===n)for(var s=0;u>s;s++)t[r+s]=e.charCodeAt(s+i);else if(null==n)for(var s=0;u>s;s++)t[r+s]=e[s+i];else{if("hex"!==n)throw new Error("base64"===n?"base64 encoding not yet supported":n+" encoding not yet supported");for(var s=0;u>s;s++){var a=i+s;t[r+s]=parseInt(e[2*a]+e[2*a+1],16)}}}function i(t,e){for(var n=e;n<t.length;n++)t[n]=0}n.write=r,n.zeroFill=i,n.toString=toString},{}],24:[function(t,e){(function(t){var n=64,r=new t(n);r.fill(0),e.exports=function(e,i){return i=i||{},i.pbkdf2=function(t,e,n,r,o){if("function"!=typeof o)throw new Error("No callback provided to pbkdf2");setTimeout(function(){o(null,i.pbkdf2Sync(t,e,n,r))})},i.pbkdf2Sync=function(i,o,u,s){if("number"!=typeof u)throw new TypeError("Iterations not a number");if(0>u)throw new TypeError("Bad iterations");if("number"!=typeof s)throw new TypeError("Key length not a number");if(0>s)throw new TypeError("Bad key length");var i=t.isBuffer(i)?i:new t(i);i.length>n?i=createHash(alg).update(i).digest():i.length<n&&(i=t.concat([i,r],n));var a,c,f,l=0,h=1,p=new t(4),d=new t(s);for(d.fill(0);s;){c=s>20?20:s,p[0]=h>>24&255,p[1]=h>>16&255,p[2]=h>>8&255,p[3]=255&h,a=e("sha1",i),a.update(o),a.update(p),f=a.digest(),f.copy(d,l,0,c);for(var g=1;u>g;g++){a=e("sha1",i),a.update(f),f=a.digest();for(var y=0;c>y;y++)d[y]^=f[y]}s-=c,h++,l+=c}return d},i}}).call(this,t("buffer").Buffer)},{buffer:10}],25:[function(t,e){(function(t){!function(){e.exports=function(e){var n=new t(e);return crypto.getRandomValues(n),n}}()}).call(this,t("buffer").Buffer)},{buffer:10}],26:[function(t,e){e.exports="function"==typeof Object.create?function(t,e){t.super_=e,t.prototype=Object.create(e.prototype,{constructor:{value:t,enumerable:!1,writable:!0,configurable:!0}})}:function(t,e){t.super_=e;var n=function(){};n.prototype=e.prototype,t.prototype=new n,t.prototype.constructor=t}},{}],27:[function(t,e){function n(){}var r=e.exports={};r.nextTick=function(){var t="undefined"!=typeof window&&window.setImmediate,e="undefined"!=typeof window&&window.postMessage&&window.addEventListener;if(t)return function(t){return window.setImmediate(t)};if(e){var n=[];return window.addEventListener("message",function(t){var e=t.source;if((e===window||null===e)&&"process-tick"===t.data&&(t.stopPropagation(),n.length>0)){var r=n.shift();r()}},!0),function(t){n.push(t),window.postMessage("process-tick","*")}}return function(t){setTimeout(t,0)}}(),r.title="browser",r.browser=!0,r.env={},r.argv=[],r.on=n,r.addListener=n,r.once=n,r.off=n,r.removeListener=n,r.removeAllListeners=n,r.emit=n,r.binding=function(){throw new Error("process.binding is not supported")},r.cwd=function(){return"/"},r.chdir=function(){throw new Error("process.chdir is not supported")}},{}],28:[function(t,e){e.exports=function(t){return t&&"object"==typeof t&&"function"==typeof t.copy&&"function"==typeof t.fill&&"function"==typeof t.readUInt8}},{}],29:[function(t,e,n){(function(e,r){function i(t,e){var r={seen:[],stylize:u};return arguments.length>=3&&(r.depth=arguments[2]),arguments.length>=4&&(r.colors=arguments[3]),g(e)?r.showHidden=e:e&&n._extend(r,e),_(r.showHidden)&&(r.showHidden=!1),_(r.depth)&&(r.depth=2),_(r.colors)&&(r.colors=!1),_(r.customInspect)&&(r.customInspect=!0),r.colors&&(r.stylize=o),a(r,t,r.depth)}function o(t,e){var n=i.styles[e];return n?"["+i.colors[n][0]+"m"+t+"["+i.colors[n][1]+"m":t}function u(t){return t}function s(t){var e={};return t.forEach(function(t){e[t]=!0}),e}function a(t,e,r){if(t.customInspect&&e&&x(e.inspect)&&e.inspect!==n.inspect&&(!e.constructor||e.constructor.prototype!==e)){var i=e.inspect(r,t);return w(i)||(i=a(t,i,r)),i}var o=c(t,e);if(o)return o;var u=Object.keys(e),g=s(u);if(t.showHidden&&(u=Object.getOwnPropertyNames(e)),I(e)&&(u.indexOf("message")>=0||u.indexOf("description")>=0))return f(e);if(0===u.length){if(x(e)){var y=e.name?": "+e.name:"";return t.stylize("[Function"+y+"]","special")}if(E(e))return t.stylize(RegExp.prototype.toString.call(e),"regexp");if(k(e))return t.stylize(Date.prototype.toString.call(e),"date");if(I(e))return f(e)}var b="",v=!1,m=["{","}"];if(d(e)&&(v=!0,m=["[","]"]),x(e)){var _=e.name?": "+e.name:"";b=" [Function"+_+"]"}if(E(e)&&(b=" "+RegExp.prototype.toString.call(e)),k(e)&&(b=" "+Date.prototype.toUTCString.call(e)),I(e)&&(b=" "+f(e)),0===u.length&&(!v||0==e.length))return m[0]+b+m[1];if(0>r)return E(e)?t.stylize(RegExp.prototype.toString.call(e),"regexp"):t.stylize("[Object]","special");t.seen.push(e);var B;return B=v?l(t,e,r,g,u):u.map(function(n){return h(t,e,r,g,n,v)}),t.seen.pop(),p(B,b,m)}function c(t,e){if(_(e))return t.stylize("undefined","undefined");if(w(e)){var n="'"+JSON.stringify(e).replace(/^"|"$/g,"").replace(/'/g,"\\'").replace(/\\"/g,'"')+"'";return t.stylize(n,"string")}return v(e)?t.stylize(""+e,"number"):g(e)?t.stylize(""+e,"boolean"):y(e)?t.stylize("null","null"):void 0}function f(t){return"["+Error.prototype.toString.call(t)+"]"}function l(t,e,n,r,i){for(var o=[],u=0,s=e.length;s>u;++u)o.push(T(e,String(u))?h(t,e,n,r,String(u),!0):"");return i.forEach(function(i){i.match(/^\d+$/)||o.push(h(t,e,n,r,i,!0))}),o}function h(t,e,n,r,i,o){var u,s,c;if(c=Object.getOwnPropertyDescriptor(e,i)||{value:e[i]},c.get?s=c.set?t.stylize("[Getter/Setter]","special"):t.stylize("[Getter]","special"):c.set&&(s=t.stylize("[Setter]","special")),T(r,i)||(u="["+i+"]"),s||(t.seen.indexOf(c.value)<0?(s=y(n)?a(t,c.value,null):a(t,c.value,n-1),s.indexOf("\n")>-1&&(s=o?s.split("\n").map(function(t){return" "+t}).join("\n").substr(2):"\n"+s.split("\n").map(function(t){return" "+t}).join("\n"))):s=t.stylize("[Circular]","special")),_(u)){if(o&&i.match(/^\d+$/))return s;u=JSON.stringify(""+i),u.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)?(u=u.substr(1,u.length-2),u=t.stylize(u,"name")):(u=u.replace(/'/g,"\\'").replace(/\\"/g,'"').replace(/(^"|"$)/g,"'"),u=t.stylize(u,"string"))}return u+": "+s}function p(t,e,n){var r=0,i=t.reduce(function(t,e){return r++,e.indexOf("\n")>=0&&r++,t+e.replace(/\u001b\[\d\d?m/g,"").length+1},0);return i>60?n[0]+(""===e?"":e+"\n ")+" "+t.join(",\n ")+" "+n[1]:n[0]+e+" "+t.join(", ")+" "+n[1]}function d(t){return Array.isArray(t)}function g(t){return"boolean"==typeof t}function y(t){return null===t}function b(t){return null==t}function v(t){return"number"==typeof t}function w(t){return"string"==typeof t}function m(t){return"symbol"==typeof t}function _(t){return void 0===t}function E(t){return B(t)&&"[object RegExp]"===A(t)}function B(t){return"object"==typeof t&&null!==t}function k(t){return B(t)&&"[object Date]"===A(t)}function I(t){return B(t)&&("[object Error]"===A(t)||t instanceof Error)}function x(t){return"function"==typeof t}function S(t){return null===t||"boolean"==typeof t||"number"==typeof t||"string"==typeof t||"symbol"==typeof t||"undefined"==typeof t}function A(t){return Object.prototype.toString.call(t)}function L(t){return 10>t?"0"+t.toString(10):t.toString(10)}function j(){var t=new Date,e=[L(t.getHours()),L(t.getMinutes()),L(t.getSeconds())].join(":");return[t.getDate(),M[t.getMonth()],e].join(" ")}function T(t,e){return Object.prototype.hasOwnProperty.call(t,e)}var U=/%[sdj%]/g;n.format=function(t){if(!w(t)){for(var e=[],n=0;n<arguments.length;n++)e.push(i(arguments[n]));return e.join(" ")}for(var n=1,r=arguments,o=r.length,u=String(t).replace(U,function(t){if("%%"===t)return"%";if(n>=o)return t;switch(t){case"%s":return String(r[n++]);case"%d":return Number(r[n++]);case"%j":try{return JSON.stringify(r[n++])}catch(e){return"[Circular]"}default:return t}}),s=r[n];o>n;s=r[++n])u+=y(s)||!B(s)?" "+s:" "+i(s);return u},n.deprecate=function(t,i){function o(){if(!u){if(e.throwDeprecation)throw new Error(i);e.traceDeprecation?console.trace(i):console.error(i),u=!0}return t.apply(this,arguments)}if(_(r.process))return function(){return n.deprecate(t,i).apply(this,arguments)};if(e.noDeprecation===!0)return t;var u=!1;return o};var O,C={};n.debuglog=function(t){if(_(O)&&(O=e.env.NODE_DEBUG||""),t=t.toUpperCase(),!C[t])if(new RegExp("\\b"+t+"\\b","i").test(O)){var r=e.pid;C[t]=function(){var e=n.format.apply(n,arguments);console.error("%s %d: %s",t,r,e)}}else C[t]=function(){};return C[t]},n.inspect=i,i.colors={bold:[1,22],italic:[3,23],underline:[4,24],inverse:[7,27],white:[37,39],grey:[90,39],black:[30,39],blue:[34,39],cyan:[36,39],green:[32,39],magenta:[35,39],red:[31,39],yellow:[33,39]},i.styles={special:"cyan",number:"yellow","boolean":"yellow",undefined:"grey","null":"bold",string:"green",date:"magenta",regexp:"red"},n.isArray=d,n.isBoolean=g,n.isNull=y,n.isNullOrUndefined=b,n.isNumber=v,n.isString=w,n.isSymbol=m,n.isUndefined=_,n.isRegExp=E,n.isObject=B,n.isDate=k,n.isError=I,n.isFunction=x,n.isPrimitive=S,n.isBuffer=t("./support/isBuffer");var M=["Jan","Feb","Mar","Apr","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec"];n.log=function(){console.log("%s - %s",j(),n.format.apply(n,arguments))},n.inherits=t("inherits"),n._extend=function(t,e){if(!e||!B(e))return t;for(var n=Object.keys(e),r=n.length;r--;)t[n[r]]=e[n[r]];return t}}).call(this,t("_process"),"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{"./support/isBuffer":28,_process:27,inherits:26}],30:[function(t,e,n){"use strict";var r=t("./promise/promise").Promise,i=t("./promise/polyfill").polyfill;n.Promise=r,n.polyfill=i},{"./promise/polyfill":34,"./promise/promise":35}],31:[function(t,e,n){"use strict";function r(t){var e=this;if(!i(t))throw new TypeError("You must pass an array to all.");return new e(function(e,n){function r(t){return function(e){i(t,e)}}function i(t,n){s[t]=n,0===--a&&e(s)}var u,s=[],a=t.length;0===a&&e([]);for(var c=0;c<t.length;c++)u=t[c],u&&o(u.then)?u.then(r(c),n):i(c,u)})}var i=t("./utils").isArray,o=t("./utils").isFunction;n.all=r},{"./utils":39}],32:[function(t,e,n){(function(t,e){"use strict";function r(){return function(){t.nextTick(u)}}function i(){var t=0,e=new f(u),n=document.createTextNode("");return e.observe(n,{characterData:!0}),function(){n.data=t=++t%2}}function o(){return function(){l.setTimeout(u,1)}}function u(){for(var t=0;t<h.length;t++){var e=h[t],n=e[0],r=e[1];n(r)}h=[]}function s(t,e){var n=h.push([t,e]);1===n&&a()}var a,c="undefined"!=typeof window?window:{},f=c.MutationObserver||c.WebKitMutationObserver,l="undefined"!=typeof e?e:void 0===this?window:this,h=[];a="undefined"!=typeof t&&"[object process]"==={}.toString.call(t)?r():f?i():o(),n.asap=s}).call(this,t("_process"),"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{_process:27}],33:[function(t,e,n){"use strict";function r(t,e){return 2!==arguments.length?i[t]:void(i[t]=e)}var i={instrument:!1};n.config=i,n.configure=r},{}],34:[function(t,e,n){(function(e){"use strict";function r(){var t;t="undefined"!=typeof e?e:"undefined"!=typeof window&&window.document?window:self;var n="Promise"in t&&"resolve"in t.Promise&&"reject"in t.Promise&&"all"in t.Promise&&"race"in t.Promise&&function(){var e;return new t.Promise(function(t){e=t}),o(e)}();n||(t.Promise=i)}var i=t("./promise").Promise,o=t("./utils").isFunction;n.polyfill=r}).call(this,"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{"./promise":35,"./utils":39}],35:[function(t,e,n){"use strict";function r(t){if(!y(t))throw new TypeError("You must pass a resolver function as the first argument to the promise constructor");if(!(this instanceof r))throw new TypeError("Failed to construct 'Promise': Please use the 'new' operator, this object constructor cannot be called as a function.");this._subscribers=[],i(t,this)}function i(t,e){function n(t){c(e,t)}function r(t){l(e,t)}try{t(n,r)}catch(i){r(i)}}function o(t,e,n,r){var i,o,u,s,f=y(n);if(f)try{i=n(r),u=!0}catch(h){s=!0,o=h}else i=r,u=!0;a(e,i)||(f&&u?c(e,i):s?l(e,o):t===k?c(e,i):t===I&&l(e,i))}function u(t,e,n,r){var i=t._subscribers,o=i.length;i[o]=e,i[o+k]=n,i[o+I]=r}function s(t,e){for(var n,r,i=t._subscribers,u=t._detail,s=0;s<i.length;s+=3)n=i[s],r=i[s+e],o(e,n,r,u);t._subscribers=null}function a(t,e){var n,r=null;try{if(t===e)throw new TypeError("A promises callback cannot return that same promise.");if(g(e)&&(r=e.then,y(r)))return r.call(e,function(r){return n?!0:(n=!0,void(e!==r?c(t,r):f(t,r)))},function(e){return n?!0:(n=!0,void l(t,e))}),!0}catch(i){return n?!0:(l(t,i),!0)}return!1}function c(t,e){t===e?f(t,e):a(t,e)||f(t,e)}function f(t,e){t._state===E&&(t._state=B,t._detail=e,d.async(h,t))}function l(t,e){t._state===E&&(t._state=B,t._detail=e,d.async(p,t))}function h(t){s(t,t._state=k)}function p(t){s(t,t._state=I)}var d=t("./config").config,g=(t("./config").configure,t("./utils").objectOrFunction),y=t("./utils").isFunction,b=(t("./utils").now,t("./all").all),v=t("./race").race,w=t("./resolve").resolve,m=t("./reject").reject,_=t("./asap").asap;d.async=_;var E=void 0,B=0,k=1,I=2;r.prototype={constructor:r,_state:void 0,_detail:void 0,_subscribers:void 0,then:function(t,e){var n=this,r=new this.constructor(function(){});if(this._state){var i=arguments;d.async(function(){o(n._state,r,i[n._state-1],n._detail)})}else u(this,r,t,e);return r},"catch":function(t){return this.then(null,t)}},r.all=b,r.race=v,r.resolve=w,r.reject=m,n.Promise=r},{"./all":31,"./asap":32,"./config":33,"./race":36,"./reject":37,"./resolve":38,"./utils":39}],36:[function(t,e,n){"use strict";function r(t){var e=this;if(!i(t))throw new TypeError("You must pass an array to race.");return new e(function(e,n){for(var r,i=0;i<t.length;i++)r=t[i],r&&"function"==typeof r.then?r.then(e,n):e(r)})}var i=t("./utils").isArray;n.race=r},{"./utils":39}],37:[function(t,e,n){"use strict";function r(t){var e=this;return new e(function(e,n){n(t)})}n.reject=r},{}],38:[function(t,e,n){"use strict";function r(t){if(t&&"object"==typeof t&&t.constructor===this)return t;var e=this;return new e(function(e){e(t)})}n.resolve=r},{}],39:[function(t,e,n){"use strict";function r(t){return i(t)||"object"==typeof t&&null!==t}function i(t){return"function"==typeof t}function o(t){return"[object Array]"===Object.prototype.toString.call(t)}var u=Date.now||function(){return(new Date).getTime()};n.objectOrFunction=r,n.isFunction=i,n.isArray=o,n.now=u},{}],40:[function(e,n){(function(r){(function(){function i(t,e,n){var r=e&&n||0,i=0;for(e=e||[],t.toLowerCase().replace(/[0-9a-f]{2}/g,function(t){16>i&&(e[r+i++]=y[t])});16>i;)e[r+i++]=0;return e}function o(t,e){var n=e||0,r=g;return r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]}function u(t,e,n){var r=e&&n||0,i=e||[];t=t||{};var u=null!=t.clockseq?t.clockseq:m,s=null!=t.msecs?t.msecs:(new Date).getTime(),a=null!=t.nsecs?t.nsecs:E+1,c=s-_+(a-E)/1e4;if(0>c&&null==t.clockseq&&(u=u+1&16383),(0>c||s>_)&&null==t.nsecs&&(a=0),a>=1e4)throw new Error("uuid.v1(): Can't create more than 10M uuids/sec");_=s,E=a,m=u,s+=122192928e5;var f=(1e4*(268435455&s)+a)%4294967296;i[r++]=f>>>24&255,i[r++]=f>>>16&255,i[r++]=f>>>8&255,i[r++]=255&f;var l=s/4294967296*1e4&268435455;i[r++]=l>>>8&255,i[r++]=255&l,i[r++]=l>>>24&15|16,i[r++]=l>>>16&255,i[r++]=u>>>8|128,i[r++]=255&u;for(var h=t.node||w,p=0;6>p;p++)i[r+p]=h[p];return e?e:o(i)}function s(t,e,n){var r=e&&n||0;"string"==typeof t&&(e="binary"==t?new d(16):null,t=null),t=t||{};var i=t.random||(t.rng||a)();if(i[6]=15&i[6]|64,i[8]=63&i[8]|128,e)for(var u=0;16>u;u++)e[r+u]=i[u];return e||o(i)}var a,c=this;if("function"==typeof e)try{var f=e("crypto").randomBytes;a=f&&function(){return f(16)}}catch(l){}if(!a&&c.crypto&&crypto.getRandomValues){var h=new Uint8Array(16);a=function(){return crypto.getRandomValues(h),h}}if(!a){var p=new Array(16);a=function(){for(var t,e=0;16>e;e++)0===(3&e)&&(t=4294967296*Math.random()),p[e]=t>>>((3&e)<<3)&255;return p}}for(var d="function"==typeof r?r:Array,g=[],y={},b=0;256>b;b++)g[b]=(b+256).toString(16).substr(1),y[g[b]]=b;var v=a(),w=[1|v[0],v[1],v[2],v[3],v[4],v[5]],m=16383&(v[6]<<8|v[7]),_=0,E=0,B=s;if(B.v1=u,B.v4=s,B.parse=i,B.unparse=o,B.BufferClass=d,"function"==typeof t&&t.amd)t(function(){return B});else if("undefined"!=typeof n&&n.exports)n.exports=B;else{var k=c.uuid;B.noConflict=function(){return c.uuid=k,B},c.uuid=B}}).call(this)}).call(this,e("buffer").Buffer)},{buffer:10,crypto:16}],41:[function(e,n,r){!function(e,i){"use strict";"object"==typeof r&&n?n.exports=i():"function"==typeof t&&t.amd?t(i):e.PubSub=i()}("object"==typeof window&&window||this,function(){"use strict";function t(t){var e;for(e in t)if(t.hasOwnProperty(e))return!0;return!1}function e(t){return function(){throw t}}function n(t,n,r){try{t(n,r)}catch(i){setTimeout(e(i),0)}}function r(t,e,n){t(e,n)}function i(t,e,i,o){var u,s=c[e],a=o?r:n;if(c.hasOwnProperty(e))for(u in s)s.hasOwnProperty(u)&&a(s[u],t,i)}function o(t,e,n){return function(){var r=String(t),o=r.lastIndexOf(".");for(i(t,t,e,n);-1!==o;)r=r.substr(0,o),o=r.lastIndexOf("."),i(t,r,e)}}function u(e){for(var n=String(e),r=Boolean(c.hasOwnProperty(n)&&t(c[n])),i=n.lastIndexOf(".");!r&&-1!==i;)n=n.substr(0,i),i=n.lastIndexOf("."),r=Boolean(c.hasOwnProperty(n)&&t(c[n]));return r}function s(t,e,n,r){var i=o(t,e,r),s=u(t);return s?(n===!0?i():setTimeout(i,0),!0):!1}var a={},c={},f=-1;return a.publish=function(t,e){return s(t,e,!1,a.immediateExceptions)},a.publishSync=function(t,e){return s(t,e,!0,a.immediateExceptions)},a.subscribe=function(t,e){if("function"!=typeof e)return!1;c.hasOwnProperty(t)||(c[t]={});var n="uid_"+String(++f);return c[t][n]=e,n},a.unsubscribe=function(t){var e,n,r,i="string"==typeof t,o=!1;for(e in c)if(c.hasOwnProperty(e)){if(n=c[e],i&&n[t]){delete n[t],o=t;break}if(!i)for(r in n)n.hasOwnProperty(r)&&n[r]===t&&(delete n[r],o=!0)}return o},a})},{}]},{},[1])(1)}); | ||
var c=[0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,7,4,13,1,10,6,15,3,12,0,9,5,2,14,11,8,3,10,14,4,9,15,8,1,2,7,0,6,13,11,5,12,1,9,11,10,0,8,12,4,13,3,7,15,14,5,6,2,4,0,5,9,7,12,2,10,14,1,3,8,11,6,15,13],f=[5,14,7,0,9,2,11,4,13,6,15,8,1,10,3,12,6,11,3,7,0,13,5,10,14,15,8,12,4,9,1,2,15,5,1,3,7,14,6,9,11,8,12,2,10,0,4,13,8,6,4,1,3,11,15,0,5,12,2,13,9,7,10,14,12,15,10,4,1,5,8,7,6,2,13,14,0,3,9,11],l=[11,14,15,12,5,8,7,9,11,13,14,15,6,7,9,8,7,6,8,13,11,9,7,15,7,12,15,9,11,7,13,12,11,13,6,7,14,9,13,15,14,8,13,6,5,12,7,5,11,12,14,15,14,15,9,8,9,14,5,6,8,6,5,12,9,15,5,11,6,8,13,12,5,12,13,14,11,8,5,6],h=[8,9,9,11,13,15,15,5,7,7,8,11,14,14,12,6,9,13,15,7,12,8,9,11,7,7,12,7,6,15,13,11,9,7,15,11,8,6,6,14,12,13,5,14,13,13,7,5,15,5,8,11,14,14,6,14,6,9,12,9,12,5,15,8,8,5,12,9,12,5,14,6,8,13,6,5,15,13,11,11],p=[0,1518500249,1859775393,2400959708,2840853838],d=[1352829926,1548603684,1836072691,2053994217,0],g=function(t){for(var e=[],n=0,r=0;n<t.length;n++,r+=8)e[r>>>5]|=t[n]<<24-r%32;return e},y=function(t){for(var e=[],n=0;n<32*t.length;n+=8)e.push(t[n>>>5]>>>24-n%32&255);return e},b=function(t,e,a){for(var g=0;16>g;g++){var y=a+g,b=e[y];e[y]=16711935&(b<<8|b>>>24)|4278255360&(b<<24|b>>>8)}var v,w,m,_,E,B,k,I,x,S;B=v=t[0],k=w=t[1],I=m=t[2],x=_=t[3],S=E=t[4];for(var A,g=0;80>g;g+=1)A=v+e[a+c[g]]|0,A+=16>g?n(w,m,_)+p[0]:32>g?r(w,m,_)+p[1]:48>g?i(w,m,_)+p[2]:64>g?o(w,m,_)+p[3]:s(w,m,_)+p[4],A=0|A,A=u(A,l[g]),A=A+E|0,v=E,E=_,_=u(m,10),m=w,w=A,A=B+e[a+f[g]]|0,A+=16>g?s(k,I,x)+d[0]:32>g?o(k,I,x)+d[1]:48>g?i(k,I,x)+d[2]:64>g?r(k,I,x)+d[3]:n(k,I,x)+d[4],A=0|A,A=u(A,h[g]),A=A+S|0,B=S,S=x,x=u(I,10),I=k,k=A;A=t[1]+m+x|0,t[1]=t[2]+_+S|0,t[2]=t[3]+E+B|0,t[3]=t[4]+v+k|0,t[4]=t[0]+w+I|0,t[0]=A}}).call(this,t("buffer").Buffer)},{buffer:13}],22:[function(t,e){var n=t("./util"),r=n.write,i=n.zeroFill;e.exports=function(t){function e(e,n){this._block=new t(e),this._finalSize=n,this._blockSize=e,this._len=0,this._s=0}function o(t,e){return null==e?t.byteLength||t.length:"ascii"==e||"binary"==e?t.length:"hex"==e?t.length/2:"base64"==e?t.length/3:void 0}return e.prototype.init=function(){this._s=0,this._len=0},e.prototype.update=function(e,n){var i,s=this._blockSize;n||"string"!=typeof e||(n="utf8"),n?("utf-8"===n&&(n="utf8"),("base64"===n||"utf8"===n)&&(e=new t(e,n),n=null),i=o(e,n)):i=e.byteLength||e.length;for(var u=this._len+=i,a=this._s=this._s||0,c=0,f=this._block;u>a;){var l=Math.min(i,c+s-a%s);r(f,e,n,a%s,c,l);var h=l-c;a+=h,c+=h,a%s||this._update(f)}return this._s=a,this},e.prototype.digest=function(t){var e=this._blockSize,r=this._finalSize,o=8*this._len,s=this._block,u=o%(8*e);s[this._len%e]=128,i(this._block,this._len%e+1),u>=8*r&&(this._update(this._block),n.zeroFill(this._block,0)),s.writeInt32BE(o,r+4);var a=this._update(this._block)||this._hash();return null==t?a:a.toString(t)},e.prototype._update=function(){throw new Error("_update must be implemented by subclass")},e}},{"./util":26}],23:[function(t,e,n){var n=e.exports=function(t){var e=n[t];if(!e)throw new Error(t+" is not supported (we accept pull requests)");return new e},r=t("buffer").Buffer,i=t("./hash")(r);n.sha=n.sha1=t("./sha1")(r,i),n.sha256=t("./sha256")(r,i)},{"./hash":22,"./sha1":24,"./sha256":25,buffer:13}],24:[function(t,e){e.exports=function(e,n){function r(){return g.length?g.pop().init():this instanceof r?(this._w=d,n.call(this,64,56),this._h=null,void this.init()):new r}function i(t,e,n,r){return 20>t?e&n|~e&r:40>t?e^n^r:60>t?e&n|e&r|n&r:e^n^r}function o(t){return 20>t?1518500249:40>t?1859775393:60>t?-1894007588:-899497514}function s(t,e){return t+e|0}function u(t,e){return t<<e|t>>>32-e}var a=t("util").inherits;a(r,n);var c=0,f=4,l=8,h=12,p=16,d=new Int32Array(80),g=[];r.prototype.init=function(){return this._a=1732584193,this._b=4023233417,this._c=2562383102,this._d=271733878,this._e=3285377520,n.prototype.init.call(this),this},r.prototype._POOL=g;new e(1)instanceof DataView;return r.prototype._update=function(){{var t,e,n,r,a,c,f,l,h,p,d=this._block;this._h}t=c=this._a,e=f=this._b,n=l=this._c,r=h=this._d,a=p=this._e;for(var g=this._w,y=0;80>y;y++){var b=g[y]=16>y?d.readInt32BE(4*y):u(g[y-3]^g[y-8]^g[y-14]^g[y-16],1),v=s(s(u(t,5),i(y,e,n,r)),s(s(a,b),o(y)));a=r,r=n,n=u(e,30),e=t,t=v}this._a=s(t,c),this._b=s(e,f),this._c=s(n,l),this._d=s(r,h),this._e=s(a,p)},r.prototype._hash=function(){g.length<100&&g.push(this);var t=new e(20);return t.writeInt32BE(0|this._a,c),t.writeInt32BE(0|this._b,f),t.writeInt32BE(0|this._c,l),t.writeInt32BE(0|this._d,h),t.writeInt32BE(0|this._e,p),t},r}},{util:32}],25:[function(t,e){{var n=t("util").inherits;t("./util")}e.exports=function(t,e){function r(){d.length,this.init(),this._w=p,e.call(this,64,56)}function i(t,e){return t>>>e|t<<32-e}function o(t,e){return t>>>e}function s(t,e,n){return t&e^~t&n}function u(t,e,n){return t&e^t&n^e&n}function a(t){return i(t,2)^i(t,13)^i(t,22)}function c(t){return i(t,6)^i(t,11)^i(t,25)}function f(t){return i(t,7)^i(t,18)^o(t,3)}function l(t){return i(t,17)^i(t,19)^o(t,10)}var h=[1116352408,1899447441,3049323471,3921009573,961987163,1508970993,2453635748,2870763221,3624381080,310598401,607225278,1426881987,1925078388,2162078206,2614888103,3248222580,3835390401,4022224774,264347078,604807628,770255983,1249150122,1555081692,1996064986,2554220882,2821834349,2952996808,3210313671,3336571891,3584528711,113926993,338241895,666307205,773529912,1294757372,1396182291,1695183700,1986661051,2177026350,2456956037,2730485921,2820302411,3259730800,3345764771,3516065817,3600352804,4094571909,275423344,430227734,506948616,659060556,883997877,958139571,1322822218,1537002063,1747873779,1955562222,2024104815,2227730452,2361852424,2428436474,2756734187,3204031479,3329325298];n(r,e);var p=new Array(64),d=[];r.prototype.init=function(){return this._a=1779033703,this._b=-1150833019,this._c=1013904242,this._d=-1521486534,this._e=1359893119,this._f=-1694144372,this._g=528734635,this._h=1541459225,this._len=this._s=0,this};return r.prototype._update=function(){var t,e,n,r,i,o,p,d,g,y,b=this._block,v=this._w;t=0|this._a,e=0|this._b,n=0|this._c,r=0|this._d,i=0|this._e,o=0|this._f,p=0|this._g,d=0|this._h;for(var w=0;64>w;w++){var m=v[w]=16>w?b.readInt32BE(4*w):l(v[w-2])+v[w-7]+f(v[w-15])+v[w-16];g=d+c(i)+s(i,o,p)+h[w]+m,y=a(t)+u(t,e,n),d=p,p=o,o=i,i=r+g,r=n,n=e,e=t,t=g+y}this._a=t+this._a|0,this._b=e+this._b|0,this._c=n+this._c|0,this._d=r+this._d|0,this._e=i+this._e|0,this._f=o+this._f|0,this._g=p+this._g|0,this._h=d+this._h|0},r.prototype._hash=function(){d.length<10&&d.push(this);var e=new t(32);return e.writeInt32BE(this._a,0),e.writeInt32BE(this._b,4),e.writeInt32BE(this._c,8),e.writeInt32BE(this._d,12),e.writeInt32BE(this._e,16),e.writeInt32BE(this._f,20),e.writeInt32BE(this._g,24),e.writeInt32BE(this._h,28),e},r}},{"./util":26,util:32}],26:[function(t,e,n){function r(t,e,n,r,i,o){var s=o-i;if("ascii"===n||"binary"===n)for(var u=0;s>u;u++)t[r+u]=e.charCodeAt(u+i);else if(null==n)for(var u=0;s>u;u++)t[r+u]=e[u+i];else{if("hex"!==n)throw new Error("base64"===n?"base64 encoding not yet supported":n+" encoding not yet supported");for(var u=0;s>u;u++){var a=i+u;t[r+u]=parseInt(e[2*a]+e[2*a+1],16)}}}function i(t,e){for(var n=e;n<t.length;n++)t[n]=0}n.write=r,n.zeroFill=i,n.toString=toString},{}],27:[function(t,e){(function(t){var n=64,r=new t(n);r.fill(0),e.exports=function(e,i){return i=i||{},i.pbkdf2=function(t,e,n,r,o){if("function"!=typeof o)throw new Error("No callback provided to pbkdf2");setTimeout(function(){o(null,i.pbkdf2Sync(t,e,n,r))})},i.pbkdf2Sync=function(i,o,s,u){if("number"!=typeof s)throw new TypeError("Iterations not a number");if(0>s)throw new TypeError("Bad iterations");if("number"!=typeof u)throw new TypeError("Key length not a number");if(0>u)throw new TypeError("Bad key length");var i=t.isBuffer(i)?i:new t(i);i.length>n?i=createHash(alg).update(i).digest():i.length<n&&(i=t.concat([i,r],n));var a,c,f,l=0,h=1,p=new t(4),d=new t(u);for(d.fill(0);u;){c=u>20?20:u,p[0]=h>>24&255,p[1]=h>>16&255,p[2]=h>>8&255,p[3]=255&h,a=e("sha1",i),a.update(o),a.update(p),f=a.digest(),f.copy(d,l,0,c);for(var g=1;s>g;g++){a=e("sha1",i),a.update(f),f=a.digest();for(var y=0;c>y;y++)d[y]^=f[y]}u-=c,h++,l+=c}return d},i}}).call(this,t("buffer").Buffer)},{buffer:13}],28:[function(t,e){(function(t){!function(){e.exports=function(e){var n=new t(e);return crypto.getRandomValues(n),n}}()}).call(this,t("buffer").Buffer)},{buffer:13}],29:[function(t,e){e.exports="function"==typeof Object.create?function(t,e){t.super_=e,t.prototype=Object.create(e.prototype,{constructor:{value:t,enumerable:!1,writable:!0,configurable:!0}})}:function(t,e){t.super_=e;var n=function(){};n.prototype=e.prototype,t.prototype=new n,t.prototype.constructor=t}},{}],30:[function(t,e){function n(){}var r=e.exports={};r.nextTick=function(){var t="undefined"!=typeof window&&window.setImmediate,e="undefined"!=typeof window&&window.postMessage&&window.addEventListener;if(t)return function(t){return window.setImmediate(t)};if(e){var n=[];return window.addEventListener("message",function(t){var e=t.source;if((e===window||null===e)&&"process-tick"===t.data&&(t.stopPropagation(),n.length>0)){var r=n.shift();r()}},!0),function(t){n.push(t),window.postMessage("process-tick","*")}}return function(t){setTimeout(t,0)}}(),r.title="browser",r.browser=!0,r.env={},r.argv=[],r.on=n,r.addListener=n,r.once=n,r.off=n,r.removeListener=n,r.removeAllListeners=n,r.emit=n,r.binding=function(){throw new Error("process.binding is not supported")},r.cwd=function(){return"/"},r.chdir=function(){throw new Error("process.chdir is not supported")}},{}],31:[function(t,e){e.exports=function(t){return t&&"object"==typeof t&&"function"==typeof t.copy&&"function"==typeof t.fill&&"function"==typeof t.readUInt8}},{}],32:[function(t,e,n){(function(e,r){function i(t,e){var r={seen:[],stylize:s};return arguments.length>=3&&(r.depth=arguments[2]),arguments.length>=4&&(r.colors=arguments[3]),g(e)?r.showHidden=e:e&&n._extend(r,e),_(r.showHidden)&&(r.showHidden=!1),_(r.depth)&&(r.depth=2),_(r.colors)&&(r.colors=!1),_(r.customInspect)&&(r.customInspect=!0),r.colors&&(r.stylize=o),a(r,t,r.depth)}function o(t,e){var n=i.styles[e];return n?"["+i.colors[n][0]+"m"+t+"["+i.colors[n][1]+"m":t}function s(t){return t}function u(t){var e={};return t.forEach(function(t){e[t]=!0}),e}function a(t,e,r){if(t.customInspect&&e&&x(e.inspect)&&e.inspect!==n.inspect&&(!e.constructor||e.constructor.prototype!==e)){var i=e.inspect(r,t);return w(i)||(i=a(t,i,r)),i}var o=c(t,e);if(o)return o;var s=Object.keys(e),g=u(s);if(t.showHidden&&(s=Object.getOwnPropertyNames(e)),I(e)&&(s.indexOf("message")>=0||s.indexOf("description")>=0))return f(e);if(0===s.length){if(x(e)){var y=e.name?": "+e.name:"";return t.stylize("[Function"+y+"]","special")}if(E(e))return t.stylize(RegExp.prototype.toString.call(e),"regexp");if(k(e))return t.stylize(Date.prototype.toString.call(e),"date");if(I(e))return f(e)}var b="",v=!1,m=["{","}"];if(d(e)&&(v=!0,m=["[","]"]),x(e)){var _=e.name?": "+e.name:"";b=" [Function"+_+"]"}if(E(e)&&(b=" "+RegExp.prototype.toString.call(e)),k(e)&&(b=" "+Date.prototype.toUTCString.call(e)),I(e)&&(b=" "+f(e)),0===s.length&&(!v||0==e.length))return m[0]+b+m[1];if(0>r)return E(e)?t.stylize(RegExp.prototype.toString.call(e),"regexp"):t.stylize("[Object]","special");t.seen.push(e);var B;return B=v?l(t,e,r,g,s):s.map(function(n){return h(t,e,r,g,n,v)}),t.seen.pop(),p(B,b,m)}function c(t,e){if(_(e))return t.stylize("undefined","undefined");if(w(e)){var n="'"+JSON.stringify(e).replace(/^"|"$/g,"").replace(/'/g,"\\'").replace(/\\"/g,'"')+"'";return t.stylize(n,"string")}return v(e)?t.stylize(""+e,"number"):g(e)?t.stylize(""+e,"boolean"):y(e)?t.stylize("null","null"):void 0}function f(t){return"["+Error.prototype.toString.call(t)+"]"}function l(t,e,n,r,i){for(var o=[],s=0,u=e.length;u>s;++s)o.push(j(e,String(s))?h(t,e,n,r,String(s),!0):"");return i.forEach(function(i){i.match(/^\d+$/)||o.push(h(t,e,n,r,i,!0))}),o}function h(t,e,n,r,i,o){var s,u,c;if(c=Object.getOwnPropertyDescriptor(e,i)||{value:e[i]},c.get?u=c.set?t.stylize("[Getter/Setter]","special"):t.stylize("[Getter]","special"):c.set&&(u=t.stylize("[Setter]","special")),j(r,i)||(s="["+i+"]"),u||(t.seen.indexOf(c.value)<0?(u=y(n)?a(t,c.value,null):a(t,c.value,n-1),u.indexOf("\n")>-1&&(u=o?u.split("\n").map(function(t){return" "+t}).join("\n").substr(2):"\n"+u.split("\n").map(function(t){return" "+t}).join("\n"))):u=t.stylize("[Circular]","special")),_(s)){if(o&&i.match(/^\d+$/))return u;s=JSON.stringify(""+i),s.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/)?(s=s.substr(1,s.length-2),s=t.stylize(s,"name")):(s=s.replace(/'/g,"\\'").replace(/\\"/g,'"').replace(/(^"|"$)/g,"'"),s=t.stylize(s,"string"))}return s+": "+u}function p(t,e,n){var r=0,i=t.reduce(function(t,e){return r++,e.indexOf("\n")>=0&&r++,t+e.replace(/\u001b\[\d\d?m/g,"").length+1},0);return i>60?n[0]+(""===e?"":e+"\n ")+" "+t.join(",\n ")+" "+n[1]:n[0]+e+" "+t.join(", ")+" "+n[1]}function d(t){return Array.isArray(t)}function g(t){return"boolean"==typeof t}function y(t){return null===t}function b(t){return null==t}function v(t){return"number"==typeof t}function w(t){return"string"==typeof t}function m(t){return"symbol"==typeof t}function _(t){return void 0===t}function E(t){return B(t)&&"[object RegExp]"===A(t)}function B(t){return"object"==typeof t&&null!==t}function k(t){return B(t)&&"[object Date]"===A(t)}function I(t){return B(t)&&("[object Error]"===A(t)||t instanceof Error)}function x(t){return"function"==typeof t}function S(t){return null===t||"boolean"==typeof t||"number"==typeof t||"string"==typeof t||"symbol"==typeof t||"undefined"==typeof t}function A(t){return Object.prototype.toString.call(t)}function T(t){return 10>t?"0"+t.toString(10):t.toString(10)}function L(){var t=new Date,e=[T(t.getHours()),T(t.getMinutes()),T(t.getSeconds())].join(":");return[t.getDate(),C[t.getMonth()],e].join(" ")}function j(t,e){return Object.prototype.hasOwnProperty.call(t,e)}var U=/%[sdj%]/g;n.format=function(t){if(!w(t)){for(var e=[],n=0;n<arguments.length;n++)e.push(i(arguments[n]));return e.join(" ")}for(var n=1,r=arguments,o=r.length,s=String(t).replace(U,function(t){if("%%"===t)return"%";if(n>=o)return t;switch(t){case"%s":return String(r[n++]);case"%d":return Number(r[n++]);case"%j":try{return JSON.stringify(r[n++])}catch(e){return"[Circular]"}default:return t}}),u=r[n];o>n;u=r[++n])s+=y(u)||!B(u)?" "+u:" "+i(u);return s},n.deprecate=function(t,i){function o(){if(!s){if(e.throwDeprecation)throw new Error(i);e.traceDeprecation?console.trace(i):console.error(i),s=!0}return t.apply(this,arguments)}if(_(r.process))return function(){return n.deprecate(t,i).apply(this,arguments)};if(e.noDeprecation===!0)return t;var s=!1;return o};var O,P={};n.debuglog=function(t){if(_(O)&&(O=e.env.NODE_DEBUG||""),t=t.toUpperCase(),!P[t])if(new RegExp("\\b"+t+"\\b","i").test(O)){var r=e.pid;P[t]=function(){var e=n.format.apply(n,arguments);console.error("%s %d: %s",t,r,e)}}else P[t]=function(){};return P[t]},n.inspect=i,i.colors={bold:[1,22],italic:[3,23],underline:[4,24],inverse:[7,27],white:[37,39],grey:[90,39],black:[30,39],blue:[34,39],cyan:[36,39],green:[32,39],magenta:[35,39],red:[31,39],yellow:[33,39]},i.styles={special:"cyan",number:"yellow","boolean":"yellow",undefined:"grey","null":"bold",string:"green",date:"magenta",regexp:"red"},n.isArray=d,n.isBoolean=g,n.isNull=y,n.isNullOrUndefined=b,n.isNumber=v,n.isString=w,n.isSymbol=m,n.isUndefined=_,n.isRegExp=E,n.isObject=B,n.isDate=k,n.isError=I,n.isFunction=x,n.isPrimitive=S,n.isBuffer=t("./support/isBuffer");var C=["Jan","Feb","Mar","Apr","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec"];n.log=function(){console.log("%s - %s",L(),n.format.apply(n,arguments))},n.inherits=t("inherits"),n._extend=function(t,e){if(!e||!B(e))return t;for(var n=Object.keys(e),r=n.length;r--;)t[n[r]]=e[n[r]];return t}}).call(this,t("_process"),"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{"./support/isBuffer":31,_process:30,inherits:29}],33:[function(t,e,n){"use strict";var r=t("./promise/promise").Promise,i=t("./promise/polyfill").polyfill;n.Promise=r,n.polyfill=i},{"./promise/polyfill":37,"./promise/promise":38}],34:[function(t,e,n){"use strict";function r(t){var e=this;if(!i(t))throw new TypeError("You must pass an array to all.");return new e(function(e,n){function r(t){return function(e){i(t,e)}}function i(t,n){u[t]=n,0===--a&&e(u)}var s,u=[],a=t.length;0===a&&e([]);for(var c=0;c<t.length;c++)s=t[c],s&&o(s.then)?s.then(r(c),n):i(c,s)})}var i=t("./utils").isArray,o=t("./utils").isFunction;n.all=r},{"./utils":42}],35:[function(t,e,n){(function(t,e){"use strict";function r(){return function(){t.nextTick(s)}}function i(){var t=0,e=new f(s),n=document.createTextNode("");return e.observe(n,{characterData:!0}),function(){n.data=t=++t%2}}function o(){return function(){l.setTimeout(s,1)}}function s(){for(var t=0;t<h.length;t++){var e=h[t],n=e[0],r=e[1];n(r)}h=[]}function u(t,e){var n=h.push([t,e]);1===n&&a()}var a,c="undefined"!=typeof window?window:{},f=c.MutationObserver||c.WebKitMutationObserver,l="undefined"!=typeof e?e:void 0===this?window:this,h=[];a="undefined"!=typeof t&&"[object process]"==={}.toString.call(t)?r():f?i():o(),n.asap=u}).call(this,t("_process"),"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{_process:30}],36:[function(t,e,n){"use strict";function r(t,e){return 2!==arguments.length?i[t]:void(i[t]=e)}var i={instrument:!1};n.config=i,n.configure=r},{}],37:[function(t,e,n){(function(e){"use strict";function r(){var t;t="undefined"!=typeof e?e:"undefined"!=typeof window&&window.document?window:self;var n="Promise"in t&&"resolve"in t.Promise&&"reject"in t.Promise&&"all"in t.Promise&&"race"in t.Promise&&function(){var e;return new t.Promise(function(t){e=t}),o(e)}();n||(t.Promise=i)}var i=t("./promise").Promise,o=t("./utils").isFunction;n.polyfill=r}).call(this,"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{"./promise":38,"./utils":42}],38:[function(t,e,n){"use strict";function r(t){if(!y(t))throw new TypeError("You must pass a resolver function as the first argument to the promise constructor");if(!(this instanceof r))throw new TypeError("Failed to construct 'Promise': Please use the 'new' operator, this object constructor cannot be called as a function.");this._subscribers=[],i(t,this)}function i(t,e){function n(t){c(e,t)}function r(t){l(e,t)}try{t(n,r)}catch(i){r(i)}}function o(t,e,n,r){var i,o,s,u,f=y(n);if(f)try{i=n(r),s=!0}catch(h){u=!0,o=h}else i=r,s=!0;a(e,i)||(f&&s?c(e,i):u?l(e,o):t===k?c(e,i):t===I&&l(e,i))}function s(t,e,n,r){var i=t._subscribers,o=i.length;i[o]=e,i[o+k]=n,i[o+I]=r}function u(t,e){for(var n,r,i=t._subscribers,s=t._detail,u=0;u<i.length;u+=3)n=i[u],r=i[u+e],o(e,n,r,s);t._subscribers=null}function a(t,e){var n,r=null;try{if(t===e)throw new TypeError("A promises callback cannot return that same promise.");if(g(e)&&(r=e.then,y(r)))return r.call(e,function(r){return n?!0:(n=!0,void(e!==r?c(t,r):f(t,r)))},function(e){return n?!0:(n=!0,void l(t,e))}),!0}catch(i){return n?!0:(l(t,i),!0)}return!1}function c(t,e){t===e?f(t,e):a(t,e)||f(t,e)}function f(t,e){t._state===E&&(t._state=B,t._detail=e,d.async(h,t))}function l(t,e){t._state===E&&(t._state=B,t._detail=e,d.async(p,t))}function h(t){u(t,t._state=k)}function p(t){u(t,t._state=I)}var d=t("./config").config,g=(t("./config").configure,t("./utils").objectOrFunction),y=t("./utils").isFunction,b=(t("./utils").now,t("./all").all),v=t("./race").race,w=t("./resolve").resolve,m=t("./reject").reject,_=t("./asap").asap;d.async=_;var E=void 0,B=0,k=1,I=2;r.prototype={constructor:r,_state:void 0,_detail:void 0,_subscribers:void 0,then:function(t,e){var n=this,r=new this.constructor(function(){});if(this._state){var i=arguments;d.async(function(){o(n._state,r,i[n._state-1],n._detail)})}else s(this,r,t,e);return r},"catch":function(t){return this.then(null,t)}},r.all=b,r.race=v,r.resolve=w,r.reject=m,n.Promise=r},{"./all":34,"./asap":35,"./config":36,"./race":39,"./reject":40,"./resolve":41,"./utils":42}],39:[function(t,e,n){"use strict";function r(t){var e=this;if(!i(t))throw new TypeError("You must pass an array to race.");return new e(function(e,n){for(var r,i=0;i<t.length;i++)r=t[i],r&&"function"==typeof r.then?r.then(e,n):e(r)})}var i=t("./utils").isArray;n.race=r},{"./utils":42}],40:[function(t,e,n){"use strict";function r(t){var e=this;return new e(function(e,n){n(t)})}n.reject=r},{}],41:[function(t,e,n){"use strict";function r(t){if(t&&"object"==typeof t&&t.constructor===this)return t;var e=this;return new e(function(e){e(t)})}n.resolve=r},{}],42:[function(t,e,n){"use strict";function r(t){return i(t)||"object"==typeof t&&null!==t}function i(t){return"function"==typeof t}function o(t){return"[object Array]"===Object.prototype.toString.call(t)}var s=Date.now||function(){return(new Date).getTime()};n.objectOrFunction=r,n.isFunction=i,n.isArray=o,n.now=s},{}],43:[function(e,n){(function(r){(function(){function i(t,e,n){var r=e&&n||0,i=0;for(e=e||[],t.toLowerCase().replace(/[0-9a-f]{2}/g,function(t){16>i&&(e[r+i++]=y[t])});16>i;)e[r+i++]=0;return e}function o(t,e){var n=e||0,r=g;return r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+"-"+r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]+r[t[n++]]}function s(t,e,n){var r=e&&n||0,i=e||[];t=t||{};var s=null!=t.clockseq?t.clockseq:m,u=null!=t.msecs?t.msecs:(new Date).getTime(),a=null!=t.nsecs?t.nsecs:E+1,c=u-_+(a-E)/1e4;if(0>c&&null==t.clockseq&&(s=s+1&16383),(0>c||u>_)&&null==t.nsecs&&(a=0),a>=1e4)throw new Error("uuid.v1(): Can't create more than 10M uuids/sec");_=u,E=a,m=s,u+=122192928e5;var f=(1e4*(268435455&u)+a)%4294967296;i[r++]=f>>>24&255,i[r++]=f>>>16&255,i[r++]=f>>>8&255,i[r++]=255&f;var l=u/4294967296*1e4&268435455;i[r++]=l>>>8&255,i[r++]=255&l,i[r++]=l>>>24&15|16,i[r++]=l>>>16&255,i[r++]=s>>>8|128,i[r++]=255&s;for(var h=t.node||w,p=0;6>p;p++)i[r+p]=h[p];return e?e:o(i)}function u(t,e,n){var r=e&&n||0;"string"==typeof t&&(e="binary"==t?new d(16):null,t=null),t=t||{};var i=t.random||(t.rng||a)();if(i[6]=15&i[6]|64,i[8]=63&i[8]|128,e)for(var s=0;16>s;s++)e[r+s]=i[s];return e||o(i)}var a,c=this;if("function"==typeof e)try{var f=e("crypto").randomBytes;a=f&&function(){return f(16)}}catch(l){}if(!a&&c.crypto&&crypto.getRandomValues){var h=new Uint8Array(16);a=function(){return crypto.getRandomValues(h),h}}if(!a){var p=new Array(16);a=function(){for(var t,e=0;16>e;e++)0===(3&e)&&(t=4294967296*Math.random()),p[e]=t>>>((3&e)<<3)&255;return p}}for(var d="function"==typeof r?r:Array,g=[],y={},b=0;256>b;b++)g[b]=(b+256).toString(16).substr(1),y[g[b]]=b;var v=a(),w=[1|v[0],v[1],v[2],v[3],v[4],v[5]],m=16383&(v[6]<<8|v[7]),_=0,E=0,B=u;if(B.v1=s,B.v4=u,B.parse=i,B.unparse=o,B.BufferClass=d,"function"==typeof t&&t.amd)t(function(){return B});else if("undefined"!=typeof n&&n.exports)n.exports=B;else{var k=c.uuid;B.noConflict=function(){return c.uuid=k,B},c.uuid=B}}).call(this)}).call(this,e("buffer").Buffer)},{buffer:13,crypto:19}],44:[function(e,n,r){!function(e,i){"use strict";"object"==typeof r&&n?n.exports=i():"function"==typeof t&&t.amd?t(i):e.PubSub=i()}("object"==typeof window&&window||this,function(){"use strict";function t(t){var e;for(e in t)if(t.hasOwnProperty(e))return!0;return!1}function e(t){return function(){throw t}}function n(t,n,r){try{t(n,r)}catch(i){setTimeout(e(i),0)}}function r(t,e,n){t(e,n)}function i(t,e,i,o){var s,u=c[e],a=o?r:n;if(c.hasOwnProperty(e))for(s in u)u.hasOwnProperty(s)&&a(u[s],t,i)}function o(t,e,n){return function(){var r=String(t),o=r.lastIndexOf(".");for(i(t,t,e,n);-1!==o;)r=r.substr(0,o),o=r.lastIndexOf("."),i(t,r,e)}}function s(e){for(var n=String(e),r=Boolean(c.hasOwnProperty(n)&&t(c[n])),i=n.lastIndexOf(".");!r&&-1!==i;)n=n.substr(0,i),i=n.lastIndexOf("."),r=Boolean(c.hasOwnProperty(n)&&t(c[n]));return r}function u(t,e,n,r){var i=o(t,e,r),u=s(t);return u?(n===!0?i():setTimeout(i,0),!0):!1}var a={},c={},f=-1;return a.publish=function(t,e){return u(t,e,!1,a.immediateExceptions)},a.publishSync=function(t,e){return u(t,e,!0,a.immediateExceptions)},a.subscribe=function(t,e){if("function"!=typeof e)return!1;c.hasOwnProperty(t)||(c[t]={});var n="uid_"+String(++f);return c[t][n]=e,n},a.unsubscribe=function(t){var e,n,r,i="string"==typeof t,o=!1;for(e in c)if(c.hasOwnProperty(e)){if(n=c[e],i&&n[t]){delete n[t],o=t;break}if(!i)for(r in n)n.hasOwnProperty(r)&&n[r]===t&&(delete n[r],o=!0)}return o},a})},{}]},{},[1])(1)}); | ||
//# sourceMappingURL=./babble.min.map |
@@ -6,3 +6,4 @@ var babble = require('../index'); | ||
emma.listen('what is your age?') | ||
// listen for messages containing either 'age' or 'how old' | ||
emma.listen(/age|how old/) | ||
.tell(function () { | ||
@@ -9,0 +10,0 @@ return 25; |
@@ -72,4 +72,4 @@ var babble = require('../index'); | ||
function decideToStart (response) { | ||
return (response == 'ok') ? 'start': 'cancel'; | ||
function canStart (response) { | ||
return (response == 'ok'); | ||
} | ||
@@ -112,6 +112,7 @@ | ||
jack.ask('emma', 'lets play guess the number') | ||
.decide(decideToStart, { | ||
start: babble.then(start).tell(guess).listen().then(checkGuess), | ||
cancel: babble.then(whine) | ||
}); | ||
.iif( | ||
canStart, // condition | ||
babble.then(start).tell(guess).listen().then(checkGuess), // trueBlock | ||
babble.then(whine) // falseBlock | ||
); | ||
@@ -118,0 +119,0 @@ })(); |
@@ -7,3 +7,6 @@ var babble = require('../index'); | ||
emma.listen('hi') | ||
.listen(printMessage) | ||
.listen(function (message, context) { | ||
console.log(context.from + ': ' + message); | ||
return message; | ||
}) | ||
.decide(function (message, context) { | ||
@@ -24,7 +27,4 @@ return (message.indexOf('age') != -1) ? 'age' : 'name'; | ||
}) | ||
.listen(printMessage); | ||
function printMessage (message, context) { | ||
console.log(context.from + ': ' + message); | ||
return message; | ||
} | ||
.listen(function (message, context) { | ||
console.log(context.from + ': ' + message); | ||
}); |
@@ -5,2 +5,13 @@ # babble history | ||
## 2014-08-07, version 0.8.0 | ||
- Completely reworked the library internally to support asynchronous flows | ||
using promises. | ||
- Callbacks can now return promises to resolve callbacks for decisions, | ||
conditions, and responses asynchronously. | ||
- Implemented a new block: `IIf(condition, trueBlock, falseBlock)`. | ||
- `Babbler.listen` now has a start condition instead of a fixed string. | ||
Condition can be a function, regexp, or any value. (Uses `iif` under the hood). | ||
## 2014-08-01, version 0.7.0 | ||
@@ -7,0 +18,0 @@ |
@@ -9,2 +9,3 @@ 'use strict'; | ||
var Decision = require('./block/Decision'); | ||
var IIf = require('./block/IIf'); | ||
@@ -33,7 +34,3 @@ /** | ||
exports.tell = function (message) { | ||
var callback = (typeof message === 'function') ? message : function () { | ||
return message; | ||
}; | ||
return new Tell(callback); | ||
return new Tell(message); | ||
}; | ||
@@ -96,3 +93,7 @@ | ||
/** | ||
* Listen for a message | ||
* Listen for a message. | ||
* | ||
* Optionally a callback function can be provided, which is equivalent of | ||
* doing `listen().then(callback)`. | ||
* | ||
* @param {Function} [callback] Invoked as callback(message, context), | ||
@@ -106,3 +107,7 @@ * where `message` is the just received message, | ||
exports.listen = function(callback) { | ||
return new Listen(callback); | ||
var block = new Listen(); | ||
if (callback) { | ||
return block.then(callback); | ||
} | ||
return block; | ||
}; | ||
@@ -122,2 +127,39 @@ | ||
/** | ||
* IIf | ||
* Create an iif block, which checks a condition and continues either with | ||
* the trueBlock or the falseBlock. The input message is passed to the next | ||
* block in the flow. | ||
* | ||
* Can be used as follows: | ||
* - When `condition` evaluates true: | ||
* - when `trueBlock` is provided, the flow continues with `trueBlock` | ||
* - else, when there is a block connected to the IIf block, the flow continues | ||
* with that block. | ||
* - When `condition` evaluates false: | ||
* - when `falseBlock` is provided, the flow continues with `falseBlock` | ||
* | ||
* Syntax: | ||
* | ||
* new IIf(condition, trueBlock) | ||
* new IIf(condition, trueBlock [, falseBlock]) | ||
* new IIf(condition).then(...) | ||
* | ||
* @param {Function | RegExp | *} condition A condition returning true or false | ||
* In case of a function, | ||
* the function is invoked as | ||
* `condition(message, context)` and | ||
* must return a boolean. In case of | ||
* a RegExp, condition will be tested | ||
* to return true. In other cases, | ||
* non-strict equality is tested on | ||
* the input. | ||
* @param {Block} [trueBlock] | ||
* @param {Block} [falseBlock] | ||
* @returns {Block} | ||
*/ | ||
exports.iif = function (condition, trueBlock, falseBlock) { | ||
return new IIf(condition, trueBlock, falseBlock); | ||
}; | ||
// export the babbler prototype | ||
@@ -131,2 +173,3 @@ exports.Babbler = Babbler; | ||
Decision: require('./block/Decision'), | ||
IIf: require('./block/IIf'), | ||
Listen: require('./block/Listen'), | ||
@@ -196,8 +239,13 @@ Tell: require('./block/Tell') | ||
var onMessageOriginal = actor.hasOwnProperty(onMessageName) ? actor[onMessageName] : null; | ||
actor[onMessageName] = function (from, message) { | ||
var handled = babbler._onMessage(message); | ||
if (!handled) { | ||
if (onMessageOriginal) { | ||
actor[onMessageName] = function (from, message) { | ||
babbler._onMessage(message); | ||
onMessageOriginal.call(actor, from, message); | ||
} | ||
}; | ||
}; | ||
} | ||
else { | ||
actor[onMessageName] = function (from, message) { | ||
babbler._onMessage(message); | ||
}; | ||
} | ||
@@ -204,0 +252,0 @@ // attach send function to the babbler |
@@ -7,2 +7,3 @@ 'use strict'; | ||
var messagers = require('./messagers'); | ||
var Conversation = require('./Conversation'); | ||
var Block = require('./block/Block'); | ||
@@ -13,2 +14,4 @@ var Then = require('./block/Then'); | ||
require('./block/IIf'); // append iif function to Block | ||
/** | ||
@@ -29,4 +32,4 @@ * Babbler | ||
this.id = id; | ||
this.listeners = {}; | ||
this.conversations = {}; | ||
this.listeners = []; // Array.<Listen> | ||
this.conversations = {}; // Array.<Array.<Conversation>> all open conversations | ||
@@ -78,3 +81,3 @@ this.connect(); // automatically connect to the local message bus | ||
// the need to await the promise to resolve on the next tick. | ||
var _resolve; | ||
var _resolve = null; | ||
var connected = new Promise(function (resolve, reject) { | ||
@@ -103,40 +106,60 @@ _resolve = resolve; | ||
* @param {{id: string, from: string, to: string, message: string}} envelope | ||
* @returns {boolean} Returns true when a message has been handled, else returns | ||
* false | ||
* @private | ||
*/ | ||
Babbler.prototype._onMessage = function (envelope) { | ||
var conversation; | ||
var trigger; | ||
var message = envelope.message; | ||
// ignore when envelope does not contain an id and message | ||
if (!envelope || !('id' in envelope) || !('message' in envelope)) { | ||
return; | ||
} | ||
//console.log('message', this.id, envelope); // TODO: cleanup | ||
// console.log('_onMessage', envelope) // TODO: cleanup | ||
// check the open conversations | ||
conversation = this.conversations[envelope.id]; | ||
if (conversation) { | ||
this._run(conversation, message); | ||
return true; | ||
var me = this; | ||
var id = envelope.id; | ||
var conversations = this.conversations[id]; | ||
if (conversations && conversations.length) { | ||
// directly deliver to all open conversations with this id | ||
conversations.forEach(function (conversation) { | ||
conversation.deliver(envelope); | ||
}) | ||
} | ||
else { | ||
// check the listeners to start a new conversation | ||
trigger = this.listeners[message]; | ||
if (trigger) { | ||
//console.log('message create a new conversation', trigger, trigger.callback); // TODO: cleanup | ||
// start new conversations at each of the listeners | ||
if (!conversations) { | ||
conversations = []; | ||
} | ||
this.conversations[id] = conversations; | ||
this.listeners.forEach(function (block) { | ||
// create a new conversation | ||
conversation = { | ||
id: envelope.id, | ||
peer: envelope.from, | ||
next: trigger, | ||
var conversation = new Conversation({ | ||
id: id, | ||
self: me.id, | ||
other: envelope.from, | ||
context: { | ||
from: envelope.from | ||
} | ||
}; | ||
}, | ||
send: me.send | ||
}); | ||
this._run(conversation, message); | ||
return true; | ||
} | ||
// append this conversation to the list with conversations | ||
conversations.push(conversation); | ||
// deliver the first message to the new conversation | ||
conversation.deliver(envelope); | ||
// process the conversation | ||
return me._process(block, conversation) | ||
.then(function() { | ||
// remove the conversation from the list again | ||
var index = conversations.indexOf(conversation); | ||
if (index !== -1) { | ||
conversations.splice(index, 1); | ||
} | ||
if (conversations.length === 0) { | ||
delete me.conversations[id]; | ||
} | ||
}); | ||
}); | ||
} | ||
return false; | ||
}; | ||
@@ -154,6 +177,6 @@ | ||
* Send a message | ||
* @param {String} id | ||
* @param {*} message | ||
* @param {String} to Id of a babbler | ||
* @param {*} message Any message. Message must be a stringifiable JSON object. | ||
*/ | ||
Babbler.prototype.send = function (id, message) { | ||
Babbler.prototype.send = function (to, message) { | ||
// send is overridden when running connect | ||
@@ -165,3 +188,10 @@ throw new Error('Cannot send: not connected'); | ||
* Listen for a specific event | ||
* @param {String} message | ||
* | ||
* Providing a condition will only start the flow when condition is met, | ||
* this is equivalent of doing `listen().iif(condition)` | ||
* | ||
* Providing a callback function is equivalent of doing either | ||
* `listen(message).then(callback)` or `listen().iif(message).then(callback)`. | ||
* | ||
* @param {function | RegExp | String | *} [condition] | ||
* @param {Function} [callback] Invoked as callback(message, context), | ||
@@ -174,15 +204,14 @@ * where `message` is the just received message, | ||
*/ | ||
// TODO: remove callback from listen | ||
Babbler.prototype.listen = function (message, callback) { | ||
if (typeof message !== 'string') { | ||
throw new TypeError('Parameter message must be a string'); | ||
Babbler.prototype.listen = function (condition, callback) { | ||
var listen = new Listen(); | ||
this.listeners.push(listen); | ||
var block = listen; | ||
if (condition) { | ||
block = listen.iif(condition); | ||
} | ||
// TODO: add support for a string as callback | ||
var listen = new Listen(callback); | ||
this.listeners[message] = listen; | ||
return listen; | ||
if (callback) { | ||
block = listen.then(callback); | ||
} | ||
return block; | ||
}; | ||
@@ -193,52 +222,33 @@ | ||
* Creates a block Tell, and runs the block immediately. | ||
* @param {String} id Babbler id | ||
* @param {String} to Babbler id | ||
* @param {Function | *} message | ||
* @return {Block} block Last block in the created control flow | ||
*/ | ||
Babbler.prototype.tell = function (id, message) { | ||
Babbler.prototype.tell = function (to, message) { | ||
var me = this; | ||
var cid = uuid.v4(); // create an id for this conversation | ||
var block = new Tell(); | ||
// create a new conversation | ||
var conversation = { | ||
var conversation = new Conversation({ | ||
id: cid, | ||
peer: id, | ||
next: block, | ||
self: this.id, | ||
other: to, | ||
context: { | ||
from: id | ||
} | ||
}; | ||
from: to | ||
}, | ||
send: me.send | ||
}); | ||
this.conversations[cid] = [conversation]; | ||
// override the `then` function, so we can immediately execute chained blocks | ||
// until we encounter a Listen block. | ||
var me = this; | ||
block.then = function then (next) { | ||
// turn a callback function into a Then block | ||
if (typeof next === 'function') { | ||
next = new Then(next); | ||
} | ||
var block = new Tell(message); | ||
if (!(next instanceof Block)) { | ||
throw new TypeError('Parameter next must be a Block or function'); | ||
} | ||
// run the Tell block on the next tick, when the conversation flow is created | ||
setTimeout(function () { | ||
me._process(block, conversation) | ||
.then(function () { | ||
// cleanup the conversation | ||
delete me.conversations[cid]; | ||
}) | ||
}, 0); | ||
conversation.next = next; | ||
if (next instanceof Listen) { | ||
// add to the conversations queue | ||
me.conversations[cid] = conversation; | ||
} | ||
else { | ||
// execute immediately | ||
message = me._run(conversation, message); | ||
next.then = then; | ||
} | ||
return next; | ||
}; | ||
// run the Tell block immediately | ||
message = this._run(conversation, message); | ||
return block; | ||
@@ -248,21 +258,7 @@ }; | ||
/** | ||
* Create a control flow starting with a Then block | ||
* @param {Function} callback Invoked as callback(message, context), | ||
* where `message` is the output from the previous | ||
* block in the chain, and `context` is an object | ||
* where state can be stored during a conversation. | ||
* @return {Then} then | ||
*/ | ||
/* TODO: implement Babbler.then | ||
Babbler.prototype.then = function (callback) { | ||
}; | ||
*/ | ||
/** | ||
* Send a question, listen for a response. | ||
* Creates two blocks: Tell and Listen, and runs them immediately. | ||
* This is equivalent of doing `Babbler.tell(id, message).listen(callback)` | ||
* @param {String} id Babbler id | ||
* @param {* | Function} message | ||
* This is equivalent of doing `Babbler.tell(to, message).listen(callback)` | ||
* @param {String} to Babbler id | ||
* @param {* | Function} message A message or a callback returning a message. | ||
* @param {Function} [callback] Invoked as callback(message, context), | ||
@@ -275,5 +271,5 @@ * where `message` is the just received message, | ||
*/ | ||
Babbler.prototype.ask = function (id, message, callback) { | ||
Babbler.prototype.ask = function (to, message, callback) { | ||
return this | ||
.tell(id, message) | ||
.tell(to, message) | ||
.listen(callback); | ||
@@ -283,44 +279,37 @@ }; | ||
/** | ||
* Run next block for given conversation | ||
* @param {Object} conversation | ||
* @param {*} [message] | ||
* @return {*} message Response returned by the last executed block | ||
* Process a flow starting with `block`, given a conversation | ||
* @param {Block} block | ||
* @param {Conversation} conversation | ||
* @return {Promise.<Conversation>} Resolves when the conversation is finished | ||
* @private | ||
*/ | ||
Babbler.prototype._run = function (conversation, message) { | ||
// console.log('_run', conversation, message); // TODO: cleanup | ||
Babbler.prototype._process = function (block, conversation) { | ||
return new Promise(function (resolve, reject) { | ||
/** | ||
* Process a block, given the conversation and a message which is chained | ||
* from block to block. | ||
* @param {Block} block | ||
* @param {*} [message] | ||
*/ | ||
function process(block, message) { | ||
//console.log('process', conversation.self, conversation.id, block.constructor.name, message) // TODO: cleanup | ||
// remove the conversation from the queue | ||
delete this.conversations[conversation.id]; | ||
var block = conversation.next; | ||
do { | ||
var next = block.execute(message, conversation.context); | ||
message = next.result; | ||
if (block.next instanceof Listen) { | ||
// wait for a next incoming message | ||
// Note: we add this listener *before* we send a message, | ||
// this is needed in case the reply is send synchronously | ||
conversation.next = block.next; | ||
this.conversations[conversation.id] = conversation; | ||
block.execute(conversation, message) | ||
.then(function (next) { | ||
if (next.block) { | ||
// recursively evaluate the next block in the conversation flow | ||
process(next.block, next.result); | ||
} | ||
else { | ||
// we are done, this is the end of the conversation | ||
resolve(conversation); | ||
} | ||
}); | ||
} | ||
if (block instanceof Tell) { | ||
// send a response back to the other peer | ||
this.send(conversation.peer, { | ||
id: conversation.id, | ||
from: this.id, | ||
to: conversation.peer, | ||
message: message | ||
}); | ||
} | ||
block = next.block; | ||
} | ||
while (block && !(block instanceof Listen)); | ||
return message; | ||
// process the first block | ||
process(block); | ||
}); | ||
}; | ||
module.exports = Babbler; |
@@ -14,7 +14,7 @@ 'use strict'; | ||
* Execute the block | ||
* @param {Conversation} conversation | ||
* @param {*} message | ||
* @param {Object} context | ||
* @return {{result: *, block: Block}} next | ||
* @return {Promise.<{result: *, block: Block}, Error>} next | ||
*/ | ||
Block.prototype.execute = function (message, context) { | ||
Block.prototype.execute = function (conversation, message) { | ||
throw new Error('Cannot run an abstract Block'); | ||
@@ -21,0 +21,0 @@ }; |
'use strict'; | ||
var Promise = require('es6-promise').Promise; | ||
var Block = require('./Block'); | ||
var isPromise =require('../util').isPromise; | ||
require('./Then'); // extend Block with function then | ||
/** | ||
@@ -33,4 +37,4 @@ * Decision | ||
* | ||
* @param arg1 Can be {function} decision or {Object} choices | ||
* @param [arg2] {Object} choices | ||
* @param arg1 | ||
* @param arg2 | ||
* @constructor | ||
@@ -61,3 +65,3 @@ * @extends {Block} | ||
else { | ||
decision = function (message) { | ||
decision = function (message, context) { | ||
return message; | ||
@@ -87,23 +91,23 @@ } | ||
* Execute the block | ||
* @param {Conversation} conversation | ||
* @param {*} message | ||
* @param {Object} context | ||
* @return {{result: *, block: Block}} next | ||
* @return {Promise.<{result: *, block: Block}, Error>} next | ||
*/ | ||
Decision.prototype.execute = function (message, context) { | ||
var id = this.decision(message, context); | ||
Decision.prototype.execute = function (conversation, message) { | ||
var me = this; | ||
var id = this.decision(message, conversation.context); | ||
if (typeof id !== 'string') { | ||
throw new TypeError('Decision function must return a string containing ' + | ||
'the id of the next block'); | ||
} | ||
var resolve = isPromise(id) ? id : Promise.resolve(id); | ||
return resolve.then(function (id) { | ||
var next = me.choices[id]; | ||
var next = this.choices[id]; | ||
if (!next) { | ||
throw new Error('Block with id "' + id + '" not found'); | ||
} | ||
if (!next) { | ||
throw new Error('Block with id "' + id + '" not found'); | ||
} | ||
return { | ||
result: message, | ||
block: next | ||
}; | ||
return { | ||
result: message, | ||
block: next | ||
}; | ||
}); | ||
}; | ||
@@ -167,3 +171,2 @@ | ||
Block.prototype.decide = function (arg1, arg2) { | ||
// TODO: test arguments.length > 2 | ||
var decision = new Decision(arg1, arg2); | ||
@@ -170,0 +173,0 @@ |
'use strict'; | ||
var Promise = require('es6-promise').Promise; | ||
var Block = require('./Block'); | ||
var Then = require('./Then'); | ||
@@ -9,41 +11,32 @@ /** | ||
* with the next block in the control flow. | ||
* @param {Function} [callback] Invoked as callback(message, context), | ||
* where `message` is the just received message, | ||
* and `context` is an object where state can be | ||
* stored during a conversation. | ||
* If callback is provided, the returned result of | ||
* the callback function is passed to the next | ||
* block, else, the received message is passed. | ||
* | ||
* @constructor | ||
* @extends {Block} | ||
*/ | ||
function Listen (callback) { | ||
function Listen () { | ||
if (!(this instanceof Listen)) { | ||
throw new SyntaxError('Constructor must be called with the new operator'); | ||
} | ||
if (callback && !(typeof callback === 'function')) { | ||
throw new TypeError('Parameter callback must be a Function'); | ||
} | ||
this.callback = callback || function (message) { | ||
return message; | ||
}; | ||
} | ||
Listen.prototype = Object.create(Block.prototype); | ||
Listen.prototype.constructor = Listen; | ||
/** | ||
* Execute the block | ||
* @param {*} message | ||
* @param {Object} context | ||
* @return {{result: *, block: Block}} next | ||
* @param {Conversation} conversation | ||
* @param {*} [message] Message is ignored by Listen blocks | ||
* @return {Promise.<{result: *, block: Block}, Error>} next | ||
*/ | ||
Listen.prototype.execute = function (message, context) { | ||
var result = this.callback(message, context); | ||
Listen.prototype.execute = function (conversation, message) { | ||
var me = this; | ||
return { | ||
result: result, | ||
block: this.next | ||
}; | ||
// wait until a message is received | ||
return conversation.receive() | ||
.then(function (message) { | ||
return { | ||
result: message, | ||
block: me.next | ||
} | ||
}); | ||
}; | ||
@@ -54,2 +47,6 @@ | ||
* Returns the first block in the chain. | ||
* | ||
* Optionally a callback function can be provided, which is equivalent of | ||
* doing `listen().then(callback)`. | ||
* | ||
* @param {Function} [callback] Executed as callback(message: *, context: Object) | ||
@@ -60,7 +57,10 @@ * Must return a result | ||
Block.prototype.listen = function (callback) { | ||
var block = new Listen(callback); | ||
return this.then(block); | ||
var listen = new Listen(); | ||
var block = this.then(listen); | ||
if (callback) { | ||
block = block.then(callback); | ||
} | ||
return block; | ||
}; | ||
module.exports = Listen; |
'use strict'; | ||
var Promise = require('es6-promise').Promise; | ||
var Block = require('./Block'); | ||
var isPromise = require('../util').isPromise; | ||
require('./Then'); // extend Block with function then | ||
require('./Listen'); // extend Block with function listen | ||
/** | ||
* Tell | ||
* Send a message to the other peer. | ||
* @param {Function} [callback] Invoked as callback(message, context), | ||
* where `message` is the output from the previous | ||
* block in the chain, and `context` is an object | ||
* where state can be stored during a conversation. | ||
* The result returned by the function will be | ||
* send back to the sender. | ||
* If no callback is provided, the block will send | ||
* the output from previous block in the chain as | ||
* message. | ||
* @param {* | Function} message A static message or callback function | ||
* returning a message dynamically. | ||
* When `message` is a function, it will be | ||
* invoked as callback(message, context), | ||
* where `message` is the output from the | ||
* previous block in the chain, and `context` is | ||
* an object where state can be stored during a | ||
* conversation. | ||
* @constructor | ||
* @extends {Block} | ||
*/ | ||
function Tell (callback) { | ||
function Tell (message) { | ||
if (!(this instanceof Tell)) { | ||
@@ -25,26 +29,38 @@ throw new SyntaxError('Constructor must be called with the new operator'); | ||
if (callback && !(typeof callback === 'function')) { | ||
throw new TypeError('Parameter callback must be a Function'); | ||
} | ||
this.callback = callback || function (message) { | ||
return message; | ||
}; | ||
this.message = message; | ||
} | ||
Tell.prototype = Object.create(Block.prototype); | ||
Tell.prototype.constructor = Tell; | ||
/** | ||
* Execute the block | ||
* @param {*} message | ||
* @param {Object} context | ||
* @return {{result: *, block: Block}} next | ||
* @param {Conversation} conversation | ||
* @param {*} [message] A message is ignored by the Tell block | ||
* @return {Promise.<{result: *, block: Block}, Error>} next | ||
*/ | ||
Tell.prototype.execute = function (message, context) { | ||
var result = this.callback(message, context); | ||
Tell.prototype.execute = function (conversation, message) { | ||
// resolve the message | ||
var me = this; | ||
var resolve; | ||
if (typeof this.message === 'function') { | ||
var result = this.message(message, conversation.context); | ||
resolve = isPromise(result) ? result : Promise.resolve(result); | ||
} | ||
else { | ||
resolve = Promise.resolve(this.message); // static string or value | ||
} | ||
return { | ||
result: result, | ||
block: this.next | ||
}; | ||
return resolve | ||
.then(function (result) { | ||
var res = conversation.send(result); | ||
var done = isPromise(res) ? res : Promise.resolve(res); | ||
return done.then(function () { | ||
return { | ||
result: result, | ||
block: me.next | ||
}; | ||
}); | ||
}); | ||
}; | ||
@@ -66,8 +82,4 @@ | ||
Block.prototype.tell = function (message) { | ||
var callback = (typeof message === 'function') ? message : function () { | ||
return message; | ||
}; | ||
var block = new Tell(message); | ||
var block = new Tell(callback); | ||
return this.then(block); | ||
@@ -74,0 +86,0 @@ }; |
'use strict'; | ||
var Promise = require('es6-promise').Promise; | ||
var Block = require('./Block'); | ||
var isPromise = require('../util').isPromise; | ||
@@ -28,16 +30,22 @@ /** | ||
Then.prototype = Object.create(Block.prototype); | ||
Then.prototype.constructor = Then; | ||
/** | ||
* Execute the block | ||
* @param {Conversation} conversation | ||
* @param {*} message | ||
* @param {Object} context | ||
* @return {{result: *, block: Block}} next | ||
* @return {Promise.<{result: *, block: Block}, Error>} next | ||
*/ | ||
Then.prototype.execute = function (message, context) { | ||
var result = this.callback(message, context); | ||
Then.prototype.execute = function (conversation, message) { | ||
var me = this; | ||
var result = this.callback(message, conversation.context); | ||
return { | ||
result: result, | ||
block: this.next | ||
}; | ||
var resolve = isPromise(result) ? result : Promise.resolve(result); | ||
return resolve.then(function (result) { | ||
return { | ||
result: result, | ||
block: me.next | ||
} | ||
}); | ||
}; | ||
@@ -44,0 +52,0 @@ |
'use strict'; | ||
var Promise = require('es6-promise').Promise; | ||
// built-in messaging interfaces | ||
@@ -29,4 +31,4 @@ | ||
send: function (id, message) { | ||
PubSub.publish(id, message); | ||
send: function (to, message) { | ||
PubSub.publish(to, message); | ||
} | ||
@@ -72,7 +74,10 @@ } | ||
send: function (id, message) { | ||
pubnub.publish({ | ||
channel: id, | ||
message: message | ||
}); | ||
send: function (to, message) { | ||
return new Promise(function (resolve, reject) { | ||
pubnub.publish({ | ||
channel: to, | ||
message: message, | ||
callback: resolve | ||
}); | ||
}) | ||
} | ||
@@ -79,0 +84,0 @@ } |
{ | ||
"name": "babble", | ||
"version": "0.7.0", | ||
"version": "0.8.0", | ||
"description": "Dynamic communication flows between message based actors.", | ||
@@ -27,4 +27,3 @@ "author": "Jos de Jong <wjosdejong@gmail.com> (https://github.com/josdejong)", | ||
"scripts": { | ||
"build": "browserify ./index.js -o ./dist/babble.js -s babble -x pubnub", | ||
"minify": "npm run build; uglifyjs ./dist/babble.js --output ./dist/babble.min.js --source-map ./dist/babble.min.map --source-map-url ./babble.min.map --compress --mangle --comments", | ||
"build": "browserify ./index.js -o ./dist/babble.js -s babble -x pubnub; uglifyjs ./dist/babble.js --output ./dist/babble.min.js --source-map ./dist/babble.map --source-map-url ./babble.min.map --compress --mangle --comments", | ||
"test": "mocha test --recursive --reporter spec" | ||
@@ -31,0 +30,0 @@ }, |
118
README.md
@@ -7,3 +7,3 @@ # Babble | ||
is modeled as a control flow diagram containing blocks `ask`, `tell`, `listen`, | ||
`decide`, and `then`. Each block can link to a next block in the | ||
`iif`, `decide`, and `then`. Each block can link to a next block in the | ||
control flow. Conversations are dynamic: a scenario is build programmatically, | ||
@@ -60,3 +60,4 @@ and the blocks can dynamically determine the next block in the scenario. | ||
emma.listen('what is your age?') | ||
// listen for messages containing either 'age' or 'how old' | ||
emma.listen(/age|how old/) | ||
.tell(function () { | ||
@@ -88,3 +89,3 @@ return 25; | ||
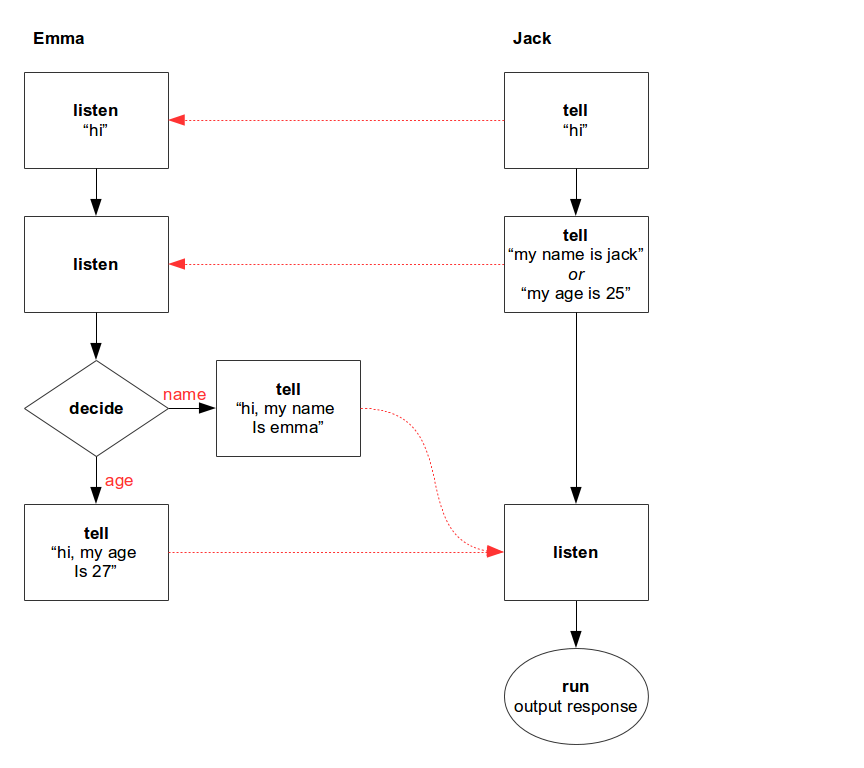 | ||
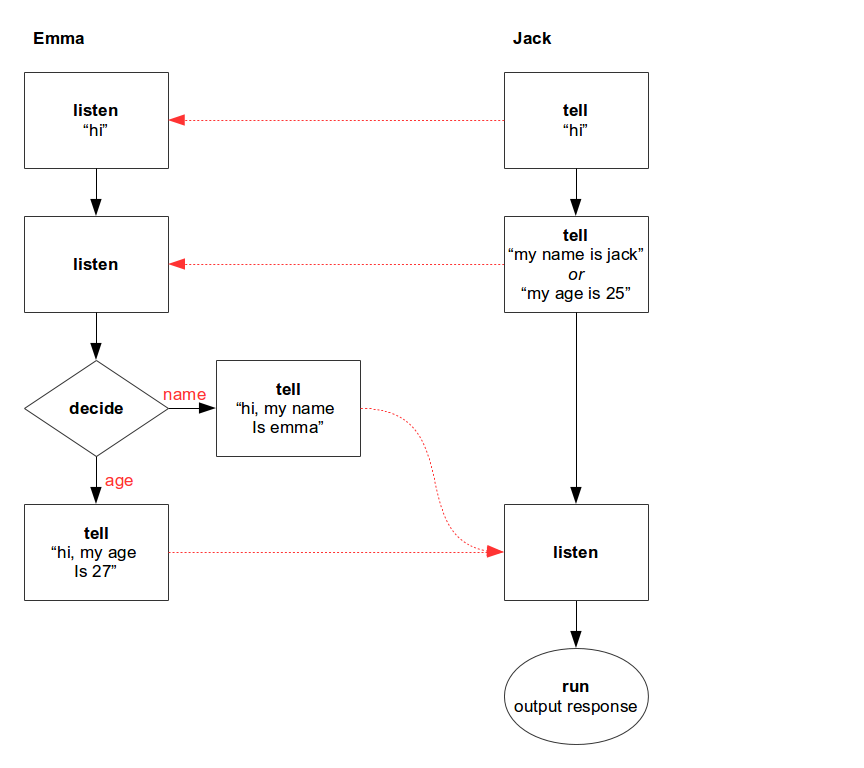 | ||
@@ -99,9 +100,7 @@ The scenario can be programmed as: | ||
function printMessage (message, context) { | ||
console.log(context.from + ': ' + message); | ||
return message; | ||
} | ||
emma.listen('hi') | ||
.listen(printMessage) | ||
.listen(function (message, context) { | ||
console.log(context.from + ': ' + message); | ||
return message; | ||
}) | ||
.decide(function (message, context) { | ||
@@ -122,3 +121,5 @@ return (message.indexOf('age') != -1) ? 'age' : 'name'; | ||
}) | ||
.listen(printMessage); | ||
.listen(function (message, context) { | ||
console.log(context.from + ': ' + message); | ||
}); | ||
``` | ||
@@ -195,3 +196,2 @@ | ||
}); | ||
``` | ||
@@ -204,6 +204,6 @@ | ||
- `babble.ask(message: String [, callback: Function]) : Block` | ||
- `babble.ask(message: String | Function [, callback: Function]) : Block` | ||
Send a question and listen for a reply. | ||
This is equivalent of doing `tell(message).listen([callback])`. | ||
This is equivalent of doing `tell(message).listen([callback])`. | ||
- `babble.babbler(id: String) : Babbler` | ||
@@ -245,2 +245,4 @@ Factory function to create a new Babbler. | ||
block in the control flow, which must be available in the provided `options`. | ||
The function `decision` can also return a Promise resolving with an id for the | ||
next block. | ||
If `decision` is not provided, the next block will be mapped directly from the | ||
@@ -251,5 +253,18 @@ `response`. Parameter `choices` is a map with the possible next blocks in the | ||
- `babble.iif(condition: function | RegExp | * [, trueBlock : Block] [, falseBlock : Block]) : Block` | ||
Create a control flow starting with an `IIf` block. | ||
When the condition is a function, it can either return a boolean or a Promise | ||
resolving with a boolean value. | ||
When the condition evaluates `true`, `trueBlock` is executed. If no `trueBlock` | ||
is provided, the next block in the chain will be executed. | ||
When the condition evaluates `true`, `falseBlock` is executed. | ||
- `babble.listen([callback: Function])` | ||
Wait for a message. The provided callback function is called as | ||
`callback(response, context)`, where `response` is the just received message. | ||
When the callback returns a promise, babble will wait with execution of the | ||
next block until the promise is resolved. The result returned by the callback | ||
is passed to the next block in the chain. | ||
Providing a callback function is equivalent of doing | ||
`babble.listen().then(callback)`. | ||
@@ -260,4 +275,6 @@ - `babble.tell(message: Function | *) : Block` | ||
is called as `callback(response, context)`, where `response` is the latest | ||
received message, and must return a result. | ||
received message, and must return a result. | ||
The returned result is send to the connected peer. | ||
When the callback returns a Promise, the value returned when the promise | ||
resolves will be send to the connected peer. | ||
@@ -268,5 +285,8 @@ - `babble.then(next: Block | function) : Block` | ||
is called as `callback(response, context)`, where `response` is the latest | ||
received message, and must return a result. The returned result is passed to | ||
the next block in the chain. | ||
received message, and must return a result. | ||
When the callback returns a promise, babble will wait with execution of the | ||
next block until the promise is resolved. The result returned by the callback | ||
is passed to the next block in the chain. | ||
- `babble.unbabblify(actor: Object) : Object` | ||
@@ -281,2 +301,3 @@ Unbabblify an actor. Returns the unbabblified actor. | ||
- `babble.block.Decision` | ||
- `babble.block.IIf` | ||
- `babble.block.Listen` | ||
@@ -295,5 +316,4 @@ - `babble.block.Tell` | ||
- `ask(id: String, message: String [, callback: Function]) : Block` | ||
Send a question to another peer and listen for a reply. | ||
This is equivalent of doing `tell(id, message).listen([callback])`. | ||
- `ask(to: String, message: * | Function [, callback: Function]) : Block` | ||
This is equivalent of doing `tell(to, message).listen([callback])`. | ||
Other blocks can be chained to the returned block. | ||
@@ -321,11 +341,24 @@ | ||
- `listen(message: String [, callback: Function]) : Block` | ||
Listen for incoming messages. If there is a match, the returned control flow | ||
block will be executed. Other blocks can be chained to the returned block. | ||
- `listen([condition: Function | RegExp | * [, callback: Function]]) : Block` | ||
Listen for incoming messages and start the conversation flow. | ||
Other blocks can be chained to the returned block. | ||
- `send(id: String, message: *)` | ||
Providing a condition will only start the flow when condition is met, | ||
this is equivalent of doing `listen().iif(condition)`. | ||
Providing a callback function is equivalent of doing either | ||
`listen(message).then(callback)` or `listen().iif(message).then(callback)`. | ||
The callback is invoked as `callback(message, context)`, and must return | ||
either a result or a Promise resolving with a result. The result will be | ||
passed to the next block in the chain. | ||
- `send(to: String, message: *)` | ||
Send a message to another peer. | ||
- `tell(id: String, message: Function | *)` | ||
- `tell(to: String, message: Function | *)` | ||
Send a notification to another peer. | ||
`message` can be a static value or a callback function. When `message` is | ||
a function, it is invoked as `callback(message, context)`, and must return | ||
either a result or a Promise resolving with a result. The result will be | ||
sent to the other peer, and will be passed to the next block in the chain. | ||
@@ -335,3 +368,3 @@ ### Block | ||
Blocks can be created via the factory functions available in `babble` | ||
(`tell`, `decide`, `then`, `listen`), or in a Babbler (`listen`, `tell`, | ||
(`tell`, `iif`, `decide`, `then`, `listen`), or in a Babbler (`listen`, `tell`, | ||
`ask`). Blocks can be chained together, resulting in a control flow. The results | ||
@@ -346,11 +379,22 @@ returned by blocks are used as input argument for the next block in the chain. | ||
Returns the first block in the chain. | ||
- `decide([decision: function, ] choices: Object<String, Block>) : Block` | ||
Append a `Decision` block to the control flow. Returns the first block in the | ||
chain. | ||
- `tell(message: *) : Block` | ||
- `iif(condition: function | RegExp | * [, trueBlock : Block] [, falseBlock : Block]) : Block` | ||
Append an `IIf` block to the control flow. Returns the first block in the | ||
chain. When the condition evaluates `true`, `trueBlock` is executed. | ||
If no `trueBlock` is provided, the next block in the chain will be executed. | ||
When the condition evaluates `true`, `falseBlock` is executed. | ||
- `listen([callback: Function]) : Block` | ||
Append a `Listen` block to the control flow. Returns the first block in the | ||
chain. Providing a callback function is equivalent of doing | ||
`listen().then(callback)`. | ||
- `tell(message: * | Function) : Block` | ||
Append a `Tell` block to the control flow. Parameter `message` can be callback | ||
function or an object or value. Returns the first block in the chain. | ||
- `listen([callback: Function]) : Block` | ||
Append a `Listen` block to the control flow. Returns the first block in the | ||
chain. | ||
- `then(block : Block | function) : Block` | ||
@@ -369,11 +413,10 @@ Append an arbitrary block to the control flow. When a callback function is | ||
To build the library `./dist/babble.js`, run: | ||
To build the library, run: | ||
npm run build | ||
To build and minify the library `./dist/babble.min.js`, run: | ||
This generates the files `./dist/babble.js`, `./dist/babble.min.js`, and | ||
`./dist/babble.min.map`. | ||
npm run minify | ||
## Test | ||
@@ -392,9 +435,6 @@ | ||
- Implement mixin pattern, enrich any object (like an actor) with babbler functionality. | ||
- Implement an `if` function which can test a function, regexp, or 'string', and continue | ||
with one block when condition is met or another block when not matched. | ||
- Implement a `filter` function, which only continues a chain when a condition is met. | ||
- Implement error handling. | ||
- Implement support for returning promises from callbacks, to allow async callback functions. | ||
- Implement error handling and timeout conditions. | ||
- Implement support for returning promises from callbacks, allowing async | ||
callback functions. | ||
- Store message history in the context. | ||
- Implement conversations with multiple peers at the same time. |
@@ -8,2 +8,3 @@ var assert = require('assert'); | ||
var Decision = require('../lib/block/Decision'); | ||
var IIf = require('../lib/block/IIf'); | ||
var Then = require('../lib/block/Then'); | ||
@@ -43,2 +44,7 @@ | ||
it('should create a flow starting with an iif block', function() { | ||
var block = babble.iif(function () {}); | ||
assert.ok(block instanceof IIf); | ||
}); | ||
it('should create a flow starting with a Then block', function() { | ||
@@ -45,0 +51,0 @@ var block = babble.then(function () {}); |
@@ -52,7 +52,2 @@ var assert = require('assert'); | ||
it ('should throw an error when calling listen wrongly', function () { | ||
assert.throws(function () {emma.listen({'a': 'not a string'})}); | ||
assert.throws(function () {emma.listen()}); | ||
}); | ||
}); | ||
@@ -71,2 +66,13 @@ | ||
it('should tell a function as message', function(done) { | ||
emma.listen('test', function (response) { | ||
assert.equal(response, 'test'); | ||
done(); | ||
}); | ||
jack.tell('emma', function () { | ||
return 'test'; | ||
}); | ||
}); | ||
it('should tell two messages subsequently', function(done) { | ||
@@ -120,3 +126,3 @@ emma.listen('foo') | ||
it ('should send a message, listen, and send a reply', function(done) { | ||
it('should send a message, listen, and send a reply', function(done) { | ||
emma.listen('count', function () { | ||
@@ -139,3 +145,3 @@ return 0; | ||
it ('should send an object as reply', function(done) { | ||
it('should send an object as reply', function(done) { | ||
emma.listen('test') | ||
@@ -152,3 +158,3 @@ .tell(function (response) { | ||
it ('should send an object as reply (2)', function(done) { | ||
it('should send an object as reply (2)', function(done) { | ||
emma.listen('test') | ||
@@ -163,3 +169,3 @@ .tell({a: 2, b: 3}); | ||
it ('should invoke the callback provided with listener', function(done) { | ||
it('should invoke the callback provided with listener', function(done) { | ||
emma.listen('what is you age?', function (response) { | ||
@@ -173,3 +179,3 @@ assert.equal(response, 'what is you age?'); | ||
it ('should invoke the callback provided with ask', function(done) { | ||
it('should invoke the callback provided with ask', function(done) { | ||
emma.listen('age') | ||
@@ -218,2 +224,31 @@ .tell(function () { | ||
it('should make a decision with an iif block', function(done) { | ||
emma.listen('are you available?') | ||
.tell(function (response) { | ||
return 'yes'; | ||
}); | ||
jack.ask('emma', 'are you available?') | ||
.iif('yes', new Then(function (message) { | ||
assert.equal(message, 'yes'); | ||
done(); | ||
}), new Then(function (message) { | ||
assert.ok(false, 'should not execute falseBlock') | ||
})); | ||
}); | ||
it('should make a decision with an inline iif block', function(done) { | ||
emma.listen('are you available?') | ||
.tell(function (response) { | ||
return 'yes'; | ||
}); | ||
jack.ask('emma', 'are you available?') | ||
.iif('yes') | ||
.then(function (message) { | ||
assert.equal(message, 'yes'); | ||
done(); | ||
}); | ||
}); | ||
it('should run then blocks', function(done) { | ||
@@ -245,3 +280,3 @@ var logs = []; | ||
it ('should keep state in the context during the conversation', function(done) { | ||
it('should keep state in the context during the conversation', function(done) { | ||
emma.listen('question', function () { | ||
@@ -248,0 +283,0 @@ return 'a'; |
var assert = require('assert'); | ||
var Promise = require('es6-promise').Promise; | ||
var Conversation = require('../../lib/Conversation'); | ||
var Block = require('../../lib/block/Block'); | ||
@@ -85,18 +87,22 @@ var Decision = require('../../lib/block/Decision'); | ||
var context = {}; | ||
var next = decision.execute('yes', context); | ||
assert.deepEqual(next, { | ||
result: 'yes', | ||
block: yes | ||
var conversation = new Conversation(); | ||
return decision.execute(conversation, 'yes').then(function (next) { | ||
assert.deepEqual(next, { | ||
result: 'yes', | ||
block: yes | ||
}) | ||
}) | ||
}); | ||
it('should execute a decision without arguments', function () { | ||
it('should execute a decision function returning a Promise', function () { | ||
var yes = new Block(); | ||
var no = new Block(); | ||
var decision = new Decision(function (response, context) { | ||
assert.strictEqual(response, 'message'); | ||
assert.strictEqual(context, undefined); | ||
return 'yes'; | ||
}, { | ||
var fn = function () { | ||
return new Promise(function (resolve, reject) { | ||
setTimeout(function () { | ||
resolve('yes'); | ||
}, 10); | ||
}); | ||
}; | ||
var decision = new Decision(fn, { | ||
yes: yes, | ||
@@ -106,6 +112,8 @@ no: no | ||
var next = decision.execute('message'); | ||
assert.deepEqual(next, { | ||
result: 'message', | ||
block: yes | ||
var conversation = new Conversation(); | ||
return decision.execute(conversation, 'yes').then(function (next) { | ||
assert.deepEqual(next, { | ||
result: 'yes', | ||
block: yes | ||
}) | ||
}) | ||
@@ -116,3 +124,2 @@ }); | ||
var yes = new Block(); | ||
var context = {a: 2}; | ||
var decision = new Decision(function (response, context) { | ||
@@ -126,7 +133,11 @@ assert.strictEqual(response, 'message'); | ||
var next = decision.execute('message', context); | ||
assert.deepEqual(next, { | ||
result: 'message', | ||
block: yes | ||
}) | ||
var conversation = new Conversation({ | ||
context: {a: 2} | ||
}); | ||
return decision.execute(conversation, 'message').then(function (next) { | ||
assert.deepEqual(next, { | ||
result: 'message', | ||
block: yes | ||
}) | ||
}); | ||
}); | ||
@@ -136,3 +147,2 @@ | ||
var yes = new Block(); | ||
var context = {a: 2}; | ||
var decision = new Decision(function (response, context) { | ||
@@ -146,9 +156,13 @@ assert.strictEqual(response, 'hello world'); | ||
var next = decision.execute('hello world', context); | ||
assert.deepEqual(next, { | ||
result: 'hello world', | ||
block: yes | ||
}) | ||
var conversation = new Conversation({ | ||
context: {a: 2} | ||
}); | ||
return decision.execute(conversation, 'hello world').then(function (next) { | ||
assert.deepEqual(next, { | ||
result: 'hello world', | ||
block: yes | ||
}) | ||
}); | ||
}); | ||
}); |
var assert = require('assert'); | ||
var Promise = require('es6-promise').Promise; | ||
var Conversation = require('../../lib/Conversation'); | ||
var Block = require('../../lib/block/Block'); | ||
var Listen = require('../../lib/block/Listen'); | ||
var IIf = require('../../lib/block/IIf'); | ||
var Then = require('../../lib/block/Then'); | ||
@@ -16,70 +21,73 @@ describe('Listen', function() { | ||
assert.throws(function () { Listen(function () {}) }, SyntaxError); | ||
assert.throws(function () { new Listen('bla')}, TypeError); | ||
}); | ||
it('should execute a listener without arguments', function () { | ||
var listener = new Listen(function (response, context) { | ||
assert.strictEqual(response, undefined); | ||
assert.strictEqual(context, undefined); | ||
return 'foo'; | ||
}); | ||
it('should execute a listener', function () { | ||
var listener = new Listen(); | ||
var next = listener.execute(); | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
}); | ||
var conversation = new Conversation(); | ||
conversation.deliver({message: 'foo'}); | ||
it('should execute a listener with context', function () { | ||
var context = {a: 2}; | ||
var listener = new Listen(function (response, context) { | ||
assert.strictEqual(response, undefined); | ||
assert.deepEqual(context, {a: 2}); | ||
return 'foo'; | ||
return listener.execute(conversation).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
}); | ||
var next = listener.execute(undefined, context); | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
}); | ||
it('should execute a listener with context and argument', function () { | ||
var context = {a: 2}; | ||
var listener = new Listen(function (response, context) { | ||
assert.strictEqual(response, 'hello world'); | ||
assert.deepEqual(context, {a: 2}); | ||
return 'foo' | ||
it('should execute a listener with delay in receiving a message', function () { | ||
var listener = new Listen(); | ||
var conversation = new Conversation(); | ||
setTimeout(function () { | ||
conversation.deliver({message: 'foo'}); | ||
}, 10); | ||
return listener.execute(conversation).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
}); | ||
var next = listener.execute('hello world', context); | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
}); | ||
it('should execute a listener with next block', function () { | ||
var listener = new Listen(function () {return 'foo'}); | ||
var nextListener = new Listen (function () {return 'foo'}); | ||
var listener = new Listen(); | ||
var nextListener = new Listen (); | ||
listener.then(nextListener); | ||
var next = listener.execute(); | ||
assert.strictEqual(next.result, 'foo'); | ||
assert.strictEqual(next.block, nextListener); | ||
var conversation = new Conversation(); | ||
conversation.deliver({message: 'foo'}); | ||
return listener.execute(conversation).then(function(next) { | ||
assert.strictEqual(next.result, 'foo'); | ||
assert.strictEqual(next.block, nextListener); | ||
}); | ||
}); | ||
it('should pass the result from and to callback when executing', function () { | ||
var action = new Listen(function (response, context) { | ||
assert.equal(response, 'in'); | ||
return 'out'; | ||
it('should create a listen+iif block from function .listen', function () { | ||
var block = new Block(); | ||
var callback = function (message) { | ||
return message + 'bar'; | ||
}; | ||
var listen = block.listen(callback); | ||
assert(block.next instanceof Listen); | ||
assert(listen.next.next instanceof Then); | ||
var conversation = new Conversation(); | ||
conversation.deliver({message: 'foo'}); | ||
return block.next.execute(conversation).then(function(next) { | ||
assert(next.block instanceof Then); | ||
assert.strictEqual(next.result, 'foo'); | ||
return next.block.execute(conversation, next.result); | ||
}).then(function(next) { | ||
assert.equal(next.block, null); | ||
assert.strictEqual(next.result, 'foobar'); | ||
}); | ||
var next = action.execute('in'); | ||
assert.strictEqual(next.result, 'out'); | ||
assert.strictEqual(next.block, undefined); | ||
}); | ||
}); |
var assert = require('assert'); | ||
var Promise = require('es6-promise').Promise; | ||
var Conversation = require('../../lib/Conversation'); | ||
var Tell = require('../../lib/block/Tell'); | ||
@@ -6,2 +8,12 @@ | ||
var sent = []; | ||
var send = function (from, message) { | ||
sent.push([from, message]); | ||
return Promise.resolve(); | ||
}; | ||
beforeEach(function () { | ||
sent = []; | ||
}); | ||
it('should create a tell', function () { | ||
@@ -17,21 +29,71 @@ var tell1 = new Tell(); | ||
assert.throws(function () { Tell(function () {}) }, SyntaxError); | ||
assert.throws(function () { new Tell('hello')}, TypeError); | ||
}); | ||
it('should execute a tell without arguments', function () { | ||
it('should execute a tell with static message', function () { | ||
var tell = new Tell('foo'); | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send | ||
}); | ||
return tell.execute(conversation).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
it('should execute a tell with callback function', function () { | ||
var tell = new Tell(function (response, context) { | ||
assert.strictEqual(response, undefined); | ||
assert.strictEqual(context, undefined); | ||
assert.strictEqual(response, 'bar'); | ||
assert.strictEqual(context, conversation.context); | ||
return 'foo'; | ||
}); | ||
var next = tell.execute(); | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send | ||
}); | ||
return tell.execute(conversation, 'bar').then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
it('should execute a tell with callback function returning a Promise', function () { | ||
var tell = new Tell(function (response, context) { | ||
assert.strictEqual(response, undefined); | ||
assert.strictEqual(context, conversation.context); | ||
return new Promise(function (resolve, reject) { | ||
setTimeout(function () { | ||
resolve('foo'); | ||
}, 10) | ||
}); | ||
}); | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send | ||
}); | ||
return tell.execute(conversation).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
it('should execute a tell with context', function () { | ||
var context = {a: 2}; | ||
var tell = new Tell(function (response, context) { | ||
@@ -43,11 +105,19 @@ assert.strictEqual(response, undefined); | ||
var next = tell.execute(undefined, context); | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send, | ||
context: {a: 2} | ||
}); | ||
return tell.execute(conversation, undefined).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
it('should execute a tell with context and argument', function () { | ||
var context = {a: 2}; | ||
var tell = new Tell(function (response, context) { | ||
@@ -59,7 +129,16 @@ assert.strictEqual(response, 'hello world'); | ||
var next = tell.execute('hello world', context); | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}) | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send, | ||
context: {a: 2} | ||
}); | ||
return tell.execute(conversation, 'hello world').then(function(next) { | ||
assert.deepEqual(next, { | ||
result: 'foo', | ||
block: undefined | ||
}); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
@@ -72,7 +151,41 @@ | ||
var next = tell.execute(); | ||
assert.strictEqual(next.result, 'foo'); | ||
assert.strictEqual(next.block, nextTell); | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send | ||
}); | ||
return tell.execute(conversation).then(function(next) { | ||
assert.strictEqual(next.result, 'foo'); | ||
assert.strictEqual(next.block, nextTell); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
it('should execute a tell with an async send method', function () { | ||
var action = new Tell('foo'); | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: function (from, message) { | ||
return new Promise(function (resolve, reject) { | ||
setTimeout(function () { | ||
sent.push([from, message]); | ||
resolve(); | ||
}, 10); | ||
}); | ||
} | ||
}); | ||
return action.execute(conversation, 'in').then(function(next) { | ||
assert.strictEqual(next.result, 'foo'); | ||
assert.strictEqual(next.block, undefined); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'foo'}]]); | ||
}); | ||
}); | ||
it('should pass the result from and to callback when executing', function () { | ||
@@ -84,7 +197,16 @@ var action = new Tell(function (response, context) { | ||
var next = action.execute('in'); | ||
assert.strictEqual(next.result, 'out'); | ||
assert.strictEqual(next.block, undefined); | ||
var conversation = new Conversation({ | ||
id: 1, | ||
self: 'peer1', | ||
other: 'peer2', | ||
send: send | ||
}); | ||
return action.execute(conversation, 'in').then(function(next) { | ||
assert.strictEqual(next.result, 'out'); | ||
assert.strictEqual(next.block, undefined); | ||
assert.deepEqual(sent, [['peer2', {id:1, from: 'peer1', to: 'peer2', message: 'out'}]]); | ||
}); | ||
}); | ||
}); |
var assert = require('assert'); | ||
var Conversation = require('../../lib/Conversation'); | ||
var Then = require('../../lib/block/Then'); | ||
@@ -17,17 +18,21 @@ | ||
it('should execute a Then block without arguments', function () { | ||
it('should execute a Then block without message', function () { | ||
var action = new Then(function (response, context) { | ||
assert.strictEqual(response, undefined); | ||
assert.strictEqual(context, undefined); | ||
assert.strictEqual(context, conversation.context); | ||
}); | ||
var next = action.execute(); | ||
assert.deepEqual(next, { | ||
result: undefined, | ||
block: undefined | ||
}) | ||
var conversation = new Conversation(); | ||
return action.execute(conversation).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: undefined, | ||
block: undefined | ||
}) | ||
}); | ||
}); | ||
it('should execute a Then block with context', function () { | ||
var context = {a: 2}; | ||
var conversation = new Conversation({ | ||
context: {a: 2} | ||
}); | ||
var action = new Then(function (response, context) { | ||
@@ -38,11 +43,14 @@ assert.strictEqual(response, undefined); | ||
var next = action.execute(undefined, context); | ||
assert.deepEqual(next, { | ||
result: undefined, | ||
block: undefined | ||
}) | ||
return action.execute(conversation, undefined).then(function(next) { | ||
assert.deepEqual(next, { | ||
result: undefined, | ||
block: undefined | ||
}) | ||
}); | ||
}); | ||
it('should execute a Then block with context and argument', function () { | ||
var context = {a: 2}; | ||
var conversation = new Conversation({ | ||
context: {a: 2} | ||
}); | ||
var action = new Then(function (response, context) { | ||
@@ -53,7 +61,8 @@ assert.strictEqual(response, 'hello world'); | ||
var next = action.execute('hello world', context); | ||
assert.deepEqual(next, { | ||
result: undefined, | ||
block: undefined | ||
}) | ||
return action.execute(conversation, 'hello world').then(function(next) { | ||
assert.deepEqual(next, { | ||
result: undefined, | ||
block: undefined | ||
}) | ||
}); | ||
}); | ||
@@ -66,5 +75,7 @@ | ||
var next = action.execute(); | ||
assert.strictEqual(next.result, undefined); | ||
assert.strictEqual(next.block, nextThen); | ||
var conversation = new Conversation(); | ||
return action.execute(conversation).then(function(next) { | ||
assert.strictEqual(next.result, undefined); | ||
assert.strictEqual(next.block, nextThen); | ||
}); | ||
}); | ||
@@ -78,7 +89,9 @@ | ||
var next = action.execute('in'); | ||
assert.strictEqual(next.result, 'out'); | ||
assert.strictEqual(next.block, undefined); | ||
var conversation = new Conversation(); | ||
return action.execute(conversation, 'in').then(function(next) { | ||
assert.strictEqual(next.result, 'out'); | ||
assert.strictEqual(next.block, undefined); | ||
}); | ||
}); | ||
}); |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
566721
46
7711
423