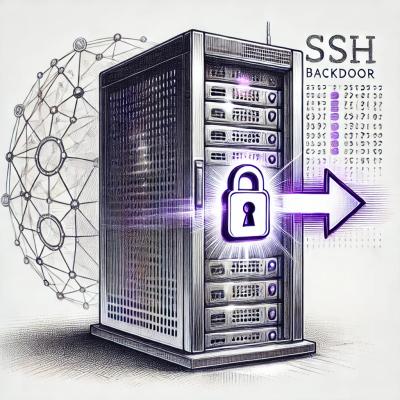
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
babe-project
Advanced tools
Basic Architecture for Browser-based experiments (https://github.com/babe-project/babe-project)
basic architecture for browser-based experiments
version 0.0.11
Table of contents
Download the .zip from this repository
Unzip and move _babe.full.min.js
, _babe.min.js
and _babe-styles.css
in the libraries/
folder of your experiment.
Your experiment's structure should look like this:
_babe.full.min.js
includes the dependencies that _babe uses (jQuery, Mustache and csv-js). There is no need to install and import jQuery, Mustache and csv-js.
_babe.min.js
includes only the _babe package, the dependencies should be installed separately for _babe to work.
_babe-styles.css
includes styles for _babe experiments.
index.html
the full version or no-dependencies version:
<script src="libraries/_babe.full.min.js></script>
or <script src="libraries/_babe.min.js></script>
and _babe-styles:
<link rel="stylesheet" type="text/css" href="libraries/_babe-styles.css">
# clone the repo
git clone https://github.com/babe-project/babe-project.git
# move to the project's folder
cd babe-project
# install the dependencies with npm
npm install babe-project --save
You need to have npm installed in your machine. Install npm.
_babe dependencies:
Once you have installed _babe, you can start using _babe funcitons to create your experiment. You can use:
Use babeInit({..})
to create a _babe experiment.
babeInit
takes an object as a parameter with the following properties:
views_seq
- an array of view objects in the sequence you want them to appear in your experiment. more infodeploy
- an object with information about the deploy methods of your experiment. more infoprogress_bar
- an object with information about the progress bars in the views of your experiment. more infoSample babeInit
call:
$("document").ready(function() {
babeInit({
views_seq: [
intro,
instructions,
practice,
main,
thanks
],
deploy: {
"experimentID": "4",
"serverAppURL": "https://babe-demo.herokuapp.com/api/submit_experiment/",
"deployMethod": "debug",
"contact_email": "YOUREMAIL@wherelifeisgreat.you",
"prolificURL": "https://app.prolific.ac/submissions/complete?cc=ABCD1234"
},
progress_bar: {
in: [
"practice",
"main"
],
style: "default",
width: 150
}
});
});
_babe views get inserted in a html element with id main
, you need to have an html tag (preferrably div
or main
)
with id="main"
Sample index.html
<!DOCTYPE html>
<html>
<head>
...
...
...
</head>
<body>
<-- ask the participants to enable JavaScript in their browser -->
<noscript>This task requires JavaScript. Please enable JavaScript in your browser and reload the page. For more information on how to do that, please refer to
<a href='https://enable-javascript.com' target='_blank'>enable-javascript.com</a>
</noscript>
<!-- views are inserted in here -->
<main id='main'>
Loading...
</main>
</body>
</html>
_babe provides several ready-made views which you can access form the babeViews
object.
trial type views:
babeViews.forcedChoice
- binary forced-choice taskbabeViews.sliderRating
- slider rating taskbabeViews.textboxInput
textbox input taskbabeViews.dropdownMenu
- dropdown menu taskbabeViews.ratingScale
- Likert-scale rating taskbabeViews.sentenceSelection
- text selection taskbabeViews.imageSelection
- click-on-a-picture taskbabeViews.keyPress
- press a button taskother views:
babeViews.intro
- introduction viewbabeViews.instructions
- instructions viewbabeViews.begin
- begin experiment view; can be used between the practice and the main viewbabeViews.postTest
- post-experiment questionnairebabeViews.thanks
- the last view that handles the submission of the results of creates a table with the results in 'Debug Mode'Each _babe view function takes an object as a parameter with the following properties:
trials: int
- the number of trials this view will appear
name: string
trial type views also have:
trial_type: string
- the name of the trial type that will be in the final data (for example 'main binary choice');data: array
- an array of trial objectsother views also have:
title: string
- the title in the viewtext: string
- the text in the viewbuttonText: string
- the text on the button that takes the user to the newxt viewSample use of _babe views:
// your_js_file.js
const intro = babeViews.intro({
title: 'Welcome!',
text: 'This is an experiment!',
buttonText: 'Begin the experiment',
trials: 1
});
const instructions = babeViews.instructions({
title: 'Instructions',
text: 'Choose an answer',
buttonText: 'Next',
trials: 1
});
const main = babeViews.forcedChoice({
trial_type: 'main',
data: main_trials,
trials: 4
});
const thanks = babeViews.thanks({
title: 'Thank you for taking part in this experiment!',
trials: 1
});
$("document").ready(function() {
babeInit({
views_seq: [
intro,
instructions,
main,
thanks
],
deploy: {
"experimentID": "4",
"serverAppURL": "https://babe-demo.herokuapp.com/api/submit_experiment/",
"deployMethod": "debug",
"contact_email": "YOUREMAIL@wherelifeisgreat.you",
"prolificURL": "https://app.prolific.ac/submissions/complete?cc=ABCD1234"
},
progress_bar: {
in: [
"main"
],
style: "default",
width: 100
}
});
});
You can also create your own views.
The views are functions that return an object with the following properties:
trials: number
- the number of trials this view appearsCT: 0
- current trial, always starts from 0name: string
- the name of the view (the progress bar uses the name)render: function
- a function that renders the view
CT
and _babe
as parameters to render()Add the data gathered from your custom trial type views to _babe.trial_data
Sample custom trial type view:
babeViews.pressTheButton = function(config) {
const _pressTheButton = {
name: config.name,
title: config.title,
render(CT, _babe) {
let startTime = Date.now();
const viewTemplate =
`<div class='view'>
{{# title }}
<h1 class="title">{{ title }}</h1>
{{/ title }}
<button id="the-button">Press me!</button>
</div>`;
$("#main").html(
Mustache.render(viewTemplate, {
title: this.title
})
);
$('#the-button').on('click', function(e) {
_babe.trial_data.push({
trial_type: config.trial_type,
trial_number: CT+1,
RT: Date.now() - startTime
});
_babe.findNextView();
});
},
CT: 0,
trials: config.trials
};
return _pressTheButton;
};
const mainTrial = babeViews.pressTheButton({
name: 'buttonPress',
title: 'How quickly can you press this button?',
trial_type: 'main',
trials: 1
});
$("document").ready(function() {
babeInit({
...
views_seq: [
...
mainTrial,
...
],
...
});
});
Sample custom info view:
babeViews.sayHello = function(config) {
const _sayHello = {
name: config.name,
title: config.title,
render(CT, _babe) {
const viewTemplate =
`<div class='view'>
{{# title }}
<h1 class="title">{{ title }}</h1>
{{/ title }}
<button id="hello-button">Hello back!</button>
</div>`;
$("#main").html(
Mustache.render(viewTemplate, {
title: this.title
})
);
$('#hello-button').on('click', function(e) {
_babe.findNextView();
});
},
CT: 0,
trials: config.trials
};
return _sayHello;
};
const hello = babeViews.sayHello({
name: 'buttonPress',
title: 'Hello!?',
trials: 1
});
$("document").ready(function() {
babeInit({
...
views_seq: [
...
hello,
...
],
...
});
});
The deploy config expects the following properties:
experimentID: string
- the experimentID is needed to recover data from the babe server app. You receive the experimentID when you create the experiment using the babe server appserverAppURL: string
- if you use the _babe server app, specify its URL heredeployMethod: string
- use one of 'debug', 'localServer', 'MTurk', 'MTurkSandbox', 'Prolific', 'directLink'contact_email: string
- who to contact in case of troubleprolificURL: string
- the prolific completion URL if the deploy method is "Prolific"prolificURL is only needed if the experiment runs on Prolific.
_babe provides the option to include progress bars in the views specified in the progress_bar.in
list passed to babeInit
. Use the names of the views in progress_bar.in
.
You can use one of the following 3 styles (include pictues)
separate
- have separate progress bars in each type of views declared in progress_bar.in
default
- have one progress bar throughout the whole experimentchunks
- have a separate progress chunk for each type of view in progress_bar.in
Use progress_bar.width
to set the width (in pixels) of the progress bar or chunk
Sample progress bar
$("document").ready(function() {
babeInit({
...
progress_bar: {
in: [
"practice",
"main"
], // only the practice and the main view will have progress bars in this experiment
style: "chunks", // there will be two chunks - one for the practice and one for the main view
width: 100 // each one of the two chunks will be 100 pixels long
}
});
});
Here you can find a sample forced-choice experiment created with _babe.
To get the development version of the _babe package, clone this repository and install the dependencies by running npm install
in the terminal
Dependencies
Development dependencies
You can find the development files in the src/
folder
Code format
...
\_babe.min.js
includes only the babe project package
_babe.full.min.js
includes the babe project package + jQuery, Mustache and csv-js
You can use uglify-es to minify the files.
npm install -g uglify-es
Instead of running the below commands manually, you can also just run npm run uglify
to produce those two files.
Mac OS and Linux
uglifyjs src/babe-errors.js src/babe-init.js src/babe-progress-bar.js src/babe-submit.js src/babe-utils.js src/babe-views.js -o _babe.min.js
Windows
uglifyjs src\babe-errors.js src\babe-init.js src\babe-progress-bar.js src\babe-submit.js src\babe-utils.js src\babe-views.js -o _babe.min.js
Mac OS and Linux
uglifyjs _babe.min.js node_modules/jquery/dist/jquery.min.js node_modules/mustache/mustache.min.js node_modules/csv-js/csv.js -o _babe.full.min.js
Windows
uglifyjs _babe.min.js node_modules\jquery\dist\jquery.min.js node_modules\mustache\mustache.min.js node_modules\csv-js\csv.js -o _babe.full.min.js
FAQs
Basic Architecture for Browser-based experiments (https://github.com/babe-project/babe-project)
The npm package babe-project receives a total of 3 weekly downloads. As such, babe-project popularity was classified as not popular.
We found that babe-project demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.