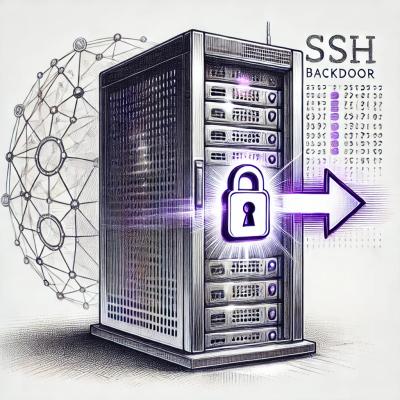
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
babe-project
Advanced tools
Basic Architecture for Browser-based experiments (https://github.com/babe-project)
basic architecture for browser-based experiments
You need npm installed on your machine. Here is more information on how to install npm
If you have npm installed, run the following command from your experiment's directory
npm install babe-project --save
Alternatively you can get the package by cloning the repo in your experiment's directory (?)
To initialise the experiment import the babe babeInit
function in your_js_file.js
// your_js_file.js
import { babeInit } from './link_to_your_libraries/babe-project/babe-init.js';
...
...
...
babeInit
takes an object as a parameter with the following properties:
views_seq
- a list of view objects in the sequence you want the to appear in your experimentdeploy
- an object with information about the deploy methods of your experimentprogress_bar
- an object with information about the progress bars in the views of your experimentSample babeInit
call:
import { babeInit } from './link_to_your_libraries/babe-project/babe-init.js';
$("document").ready(function() {
babeInit({
views_seq: [
intro,
instructions,
practice,
main,
thanks
],
deploy: {
"experimentID": "4",
"serverAppURL": "https://babe-demo.herokuapp.com/api/submit_experiment/",
"deployMethod": "debug",
"contact_email": "YOUREMAIL@wherelifeisgreat.you",
"prolificURL": "https://app.prolific.ac/submissions/complete?cc=ABCD1234"
},
progress_bar: {
in: [
"practice",
"main"
],
style: "default",
width: 150
}
});
});
For _babe views to render, you need to have an html tag (preferrably div
or main
)
with id="main"
_babe provides several ready-made views which you can use by importing them in your_js_file.js
.
trial type views:
forcedChoice
- binary forced-choice tasktextboxInput
textbox input tasksliderRating
- slider rating taskdropdownMenu
- dropdown menu taskratingScale
- Likert-scale rating tasksentenceSelection
- text selection taskimageSelection
- click-on-a-picture taskkeyPress
- press a button taskother views:
Each _babe view function takes an object as a parameter with the following properties:
trials
- the number of trials this view will appear
trial type views also have:
trial_type
- the name of the trial type that will be in the final data (for example 'main binary choice');data
- a list of trialsother views also have:
title
- the title in the viewtext
- the text in the viewbuttonText
- the text on the button that takes the user to the newxt viewSample use of _babe views:
// your_js_file.js
import { babeInit } from './link_to_your_libraries/babe-project/babe-init.js';
import { intro, instructions, forcedChoice, thanks } from './link_to_your_libraries/babe-project/babe-views.js';
const myIntro = intro({
title: 'Welcome!',
text: 'This is an experiment!',
buttonText: 'Begin the experiment',
trials: 1
});
const myInstructions = instructions({
title: 'Instructions',
text: 'Choose an answer',
buttonText: 'Next',
trials: 1
});
const main = forcedChoice({
trial_type: 'main',
data: main_trials,
trials: 4
});
const thankYou = thanks({
title: 'Thank you for taking part in this experiment!',
trials: 1
});
$("document").ready(function() {
babeInit({
views_seq: [
myIntro,
myInstructions,
main,
thankYou
],
deploy: {
"experimentID": "4",
"serverAppURL": "https://babe-demo.herokuapp.com/api/submit_experiment/",
"deployMethod": "debug",
"contact_email": "YOUREMAIL@wherelifeisgreat.you",
"prolificURL": "https://app.prolific.ac/submissions/complete?cc=ABCD1234"
},
progress_bar: {
in: [
"practice",
"main"
],
style: "default",
width: 150
}
});
});
You can also create your own views. Here is what you need to know:
The view is an object that has the following properties:
trials: number
- the number of trials this view appearsCT: 0
- current trial, always starts from 0, ??name: string
- the name of the view ??render: function
- a function that renders the viewfor the trial type views
CT
as a parameter to render()Sample custom view:
// your_js_file.js
import { babeInit } from './link_to_your_libraries/babe-project/babe-init.js';
const sayHello = function(info) {
const sayHello = {
name: info.name,
title: info.title,
text: info.text,
render() {
const viewTemplate =
`<div class='view'>
{{# title }}
<h1 class="title">{{ title }}</h1>
{{/ title }}
{{# text }}
<section class="text-container">
<p class="text">{{ text }}</p>
</section>
{{/ text }}
<button id="hello-back">Hello to you, too!</button>
<button id="next" class="nodisplay">Bye!</button>
</div>`;
$("#main").html(
Mustache.render(viewTemplate, {
title: this.title,
text: this.text
})
);
$('#hello-back').on('click', function(e) {
$('#next').removeClass('nodisplay');
$('.text').addClass('nodisplay');
e.target.classList.add('nodisplay');
});
$('#next').on('click', function() {
findNextView();
})
},
CT: 0,
trials: info.trials
};
return sayHello;
};
const mySayHello = sayHello({
name: 'sayHello',
title: 'Hello',
text: 'I am here just to say Hello..',
trials: 1
});
$("document").ready(function() {
babeInit({
...
views_seq: [
...
mySayHello,
...
],
...
});
});
The deploy config has the following properties:
experimentID
- the experimentID is needed to recover data from the babe server app. You receive the experimentID when you create the experiment using the babe server appserverAppURL
- if you use the _babe server app, specify its URL heredeployMethod
- use one of 'debug', 'localServer', 'MTurk', 'MTurkSandbox', 'Prolific', 'directLink'contact_email
- who to contact in case of troubleprolificURL
- the prolific completion URL if the deploy method is "Prolific"_babe provides the option to include progress bars in the views specified in the progress_bar.in
list passed to babeInit
.
You can use one of the following 3 styles (include pictues)
separate
- have separate progress bars in each views declared in progress_bar.in
default
- have one progress bar throughout the whole experimentchunks
- have a separate progress chunk for each view in progress_bar.in
Use progress_bar.width
to set the width (in pixels) of the progress bar or chunk
Sample progress bar
$("document").ready(function() {
babeInit({
...
progress_bar: {
in: [
"practice",
"main"
], // only the practice and the main view will have progress bars in this experiment
style: "chunks", // there will be two chunks - one for the practice and one for the main view
width: 100 // each one of the two chunks will be 100 pixels long
}
});
});
FAQs
Basic Architecture for Browser-based experiments (https://github.com/babe-project/babe-project)
The npm package babe-project receives a total of 3 weekly downloads. As such, babe-project popularity was classified as not popular.
We found that babe-project demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.