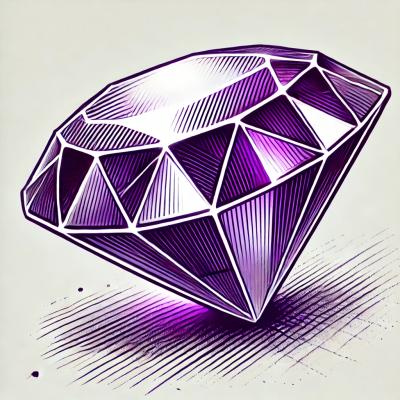
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
babel-plugin-transform-cssobj
Advanced tools
Babel plugin to transform css into cssobj (CSS in JS engine), map class names into cssobj localized names
Babel plugin to transform css into cssobj (CSS in JS solution), map class names into cssobj localized names
npm install --save-dev babel-plugin-transform-cssobj
.babelrc
:{
"plugins": ["transform-cssobj"]
}
Write CSS as normal, Wrap JSX in result.mapClass()
const result = CSSOBJ`
---
# YAML as config
plugins:
- default-unit: px
- flexbox
---
// support inline comment in style
body { color: red; font-size:12; }
.container {
display: flex;
height: ${ getWindowHeight() };
// nest selector
.item {
flex: 1; width: 100; height: ${ v=> v.prev ? v.prev + 1 : 200 };
a {
color: red;
&:hover { color: blue; }
}
}
}
`
const html = result.mapClass(
<div class='container'>
<div class='item'>
<a class='!news item active'>link text</a></div></div>
)
Which transform into below code:
import cssobj from 'cssobj';
import cssobj_plugin_default_unit from 'cssobj-plugin-default-unit';
import cssobj_plugin_flexbox from 'cssobj-plugin-flexbox';
const result = cssobj({
body: {
color: 'red',
fontSize: 12
},
'.container': {
display: 'flex',
height: getWindowHeight(),
'.item': {
flex: 1,
width: 100,
height: v => v.prev ? v.prev + 1 : 200,
a: {
color: 'red',
'&:hover': {
color: 'blue'
}
}
}
}
}, {
plugins: [cssobj_plugin_default_unit('px'), cssobj_plugin_flexbox()]
});
const html = <div class={result.mapClass('container')}>
<div class={result.mapClass('item')}>
<a class={result.mapClass('!news item active')}>link text</a></div></div>;
Note: According to cssobj mapClass
rule, the !news
will become news
and not localized (aka keep AS IS).
This plugin transform the below formats:
result.mapClass(JSX)
result.mapName(JSX) (alias of result.mapClass)
mapName(JSX) (function reference of result.mapClass)
If your existing code already has the form, .e.g:
// existing code, you don't want below to transform
myObj.mapClass(<div className='abc'>should not be transformed</div>)
You have two way to escape the transform
Change the original method call as myObj['mapClass']
, that way this plugin don't touch it
Pass plugin option mapName
to use other name rather than mapClass
{
"plugins": [ ["transform-cssobj", {"mapName": "makeLocal"}] ]
}
Then you can use makeLocal
instead of mapClass
, as a alias property of cssobj result
Notice: makeLocal
must not exists in result object to avoid conflict
// below will be transformed, using alias property
style.makeLocal( <div className='nav'></div> )
// <div className={ style.mapClass('nav') }></div>
// your existing code keep untouched
myObj.mapClass( <div className='abc'> )
If you discard the cssobj result part, then the mapName
is not alias, it's a real function
Notice: makeLocal
must exists in your scope, it will be kept as real function
// makeLocal is not alias, it's have to be assigned
const makeLocal = style.mapClass
// will inject to className, shorter code
makeLocal( <div className='nav'></div> )
// <div className={ makeLocal('nav') }></div>
See, all the className have a shorter code, that reduced the bundle size and have better pref
Normally the default import name of import cssobj from 'cssobj'
declear should be ok, but if the cssobj
have conflicts, pass below option in .babelrc
{
"plugins": [ ["transform-cssobj",
{
"mapName": "makeLocal",
"tag": "MYTAG",
"format": "less",
"names": {"cssobj": {"name": "_cssobj", "path": "./cssobj"}}
} ] ]
}
FAQs
Babel plugin to transform css into cssobj (CSS in JS engine), map class names into cssobj localized names
We found that babel-plugin-transform-cssobj demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.