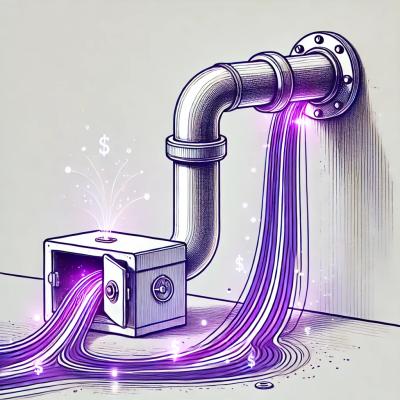
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
babel-preset-minify
Advanced tools
babel-preset-minify is a Babel preset that allows you to minify your JavaScript code. It includes a collection of Babel plugins that perform various code optimizations and transformations to reduce the size of your JavaScript files.
Remove Console Statements
This feature removes all console statements from your code, which can help reduce the size of your JavaScript files and improve performance.
const code = `console.log('Hello World');`; // After minification: ''
Dead Code Elimination
This feature eliminates dead code that will never be executed, further reducing the size of your JavaScript files.
const code = `if (false) { console.log('This will be removed'); }`; // After minification: ''
Mangle Variable Names
This feature shortens variable and function names to single characters, which can significantly reduce the size of your JavaScript files.
const code = `function add(a, b) { return a + b; }`; // After minification: 'function a(b,c){return b+c}'
UglifyJS is a JavaScript parser, minifier, compressor, and beautifier toolkit. It is one of the most popular tools for minifying JavaScript and offers a wide range of options for code optimization. Compared to babel-preset-minify, UglifyJS is more mature and widely used, but it may not integrate as seamlessly with Babel.
Terser is a JavaScript parser and mangler/compressor toolkit for ES6+. It is a fork of UglifyJS that aims to retain API and CLI compatibility with UglifyJS while improving support for modern JavaScript features. Terser is often preferred over UglifyJS for projects using ES6+ syntax. Compared to babel-preset-minify, Terser offers more advanced minification options and better support for modern JavaScript.
Google Closure Compiler is a tool for making JavaScript download and run faster. It parses your JavaScript, analyzes it, removes dead code, and rewrites and minimizes what's left. It also checks syntax, variable references, and types. Compared to babel-preset-minify, Closure Compiler offers more aggressive optimizations and advanced features like type checking, but it can be more complex to configure and use.
Babel preset for all minify plugins.
npm install babel-preset-minify --save-dev
.babelrc
(Recommended).babelrc
{
"presets": ["minify"]
}
or pass in options -
{
"presets": [["minify", {
"mangle": {
"exclude": ["MyCustomError"]
},
"unsafe": {
"typeConstructors": false
},
"keepFnName": true
}]]
}
babel script.js --presets minify
const babel = require("@babel/core");
const fs = require("fs");
const code = fs.readFileSync("./input.js").toString();
const minified = babel.transform(code, {
presets: ["minify"]
});
Two types of options:
false
- disable plugintrue
- enable plugin{ ...pluginOpts }
- enable plugin and pass pluginOpts to pluginOptionName | Plugin | DefaultValue |
---|---|---|
booleans | transform-minify-booleans | true |
builtIns | minify-builtins | true |
consecutiveAdds | transform-inline-consecutive-adds | true |
deadcode | minify-dead-code-elimination | true |
evaluate | minify-constant-folding | true |
flipComparisons | minify-flip-comparisons | true |
guards | minify-guarded-expressions | true |
infinity | minify-infinity | true |
mangle | minify-mangle-names | true |
memberExpressions | transform-member-expression-literals | true |
mergeVars | transform-merge-sibling-variables | true |
numericLiterals | minify-numeric-literals | true |
propertyLiterals | transform-property-literals | true |
regexpConstructors | transform-regexp-constructors | true |
removeConsole | transform-remove-console | false |
removeDebugger | transform-remove-debugger | false |
removeUndefined | transform-remove-undefined | true |
replace | minify-replace | true |
simplify | minify-simplify | true |
simplifyComparisons | transform-simplify-comparison-operators | true |
typeConstructors | minify-type-constructors | true |
undefinedToVoid | transform-undefined-to-void | true |
OptionName | Plugins |
---|---|
keepFnName | Passed to mangle & deadcode |
keepClassName | Passed to mangle & deadcode |
tdz | Passed to builtIns, evaluate, deadcode, removeUndefined |
Examples
{
"presets": [["minify", {
"evaluate": false,
"mangle": true
}]]
}
{
"presets": [["minify", {
"mangle": {
"exclude": ["ParserError", "NetworkError"]
}
}]]
}
{
"presets": [["minify", {
"keepFnName": true
}]]
}
// is the same as
{
"presets": [["minify", {
"mangle": {
"keepFnName": true
},
"deadcode": {
"keepFnName": true
}
}]]
}
FAQs
Babel preset for all minify plugins.
The npm package babel-preset-minify receives a total of 394,643 weekly downloads. As such, babel-preset-minify popularity was classified as popular.
We found that babel-preset-minify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.