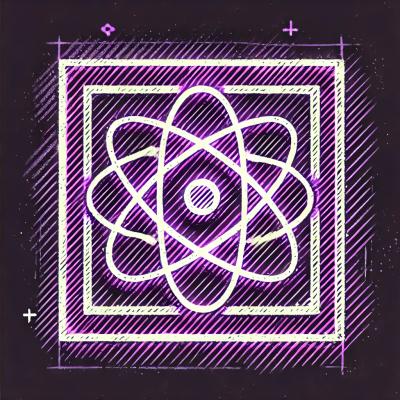
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Simple typescript base45 (charset of Qr codes, alphanumeric mode) encoder/decoder.
This library exports an ES module, together with the required type annotations.
import * as b45 from "base45-ts";
const buffer : Uint8Array = new TextEncoder().encode('Hello!')
const e : string = b45.encode(buffer);
console.log(e); // Will output %69 VD92EX0"
const d = b45.decode('%69 VD92EX0')
console.log(d); // will output 'Uint8Array(7) [72, 101, 108, 108, 111, 33, 33]'
const d = b45.decodeToUtf8String('%69 VD92EX0')
console.log(d); // will output 'Hello!'
decode(utf8StringArg: string): Uint8Array
Decode a base45-encoded string
string
: A base45-encoded stringAn Uint8Array
containing the decoded data
decodeToUtf8String(utf8StringArg: string): string
Same as decode, but returns a string instead of a typed array. If the base45-encoded data was not valid UTF-8, throws an error.
string
: base45-encoded string representing an utf8 stringthe decoded string
encode(byteArrayArg: Uint8Array | number[]): string
Encode binary data to base45
Uint8Array | number[]
An array of bytes to encodea base45-encoded string
This library doesn't have any dependency.
npm run build
npm test
FAQs
type-safe base45 encoder and decoder
We found that base45-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.