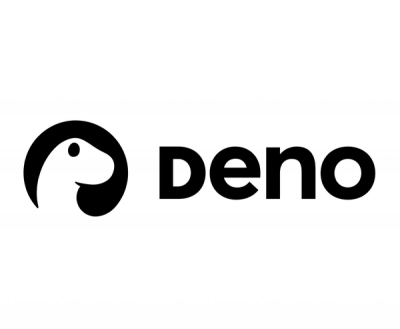
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
provides simplified promise error handling
Just npm install baseball
With Promises:
var baseball = require('baseball')();
var throwAPitch = new Promise(function (resolve, reject) {
reject(new Error("sports are hard"));
});
// automatically catches and logs to console.error
// Error: sports are hard
//
throwAPitch.catch(baseball);
With options (messagePrefix):
var baseball = require('baseball')("PREPEND ME: ");
var throwAPitch = new Promise(function (resolve, reject) {
reject(new Error("everything is awful"));
});
// automatically catches and logs to console.error
// PREPEND ME: Error: everything is awful
//
throwAPitch.catch(baseball);
With options (logger):
var baseball = require('baseball')(bunyan.error);
var throwAPitch = new Promise(function (resolve, reject) {
reject(new Error("everything is awful"));
});
// automatically catches and logs to bunyan
// Error: everything is awful
//
throwAPitch.catch(baseball);
With explicit options:
var baseball = require('baseball')({messagePrefix: "PREPEND ME: ", logger: bunyan.error});
var throwAPitch = new Promise(function (resolve, reject) {
reject(new Error("everything is awful"));
});
// automatically catches and logs to bunyan
// PREPEND ME: Error: everything is awful
//
throwAPitch.catch(baseball);
Why not - sometimes even the simple things could be simplier. Ya know, for lazy people.
MIT LICENSE
FAQs
simplified runtime requirements
The npm package baseball receives a total of 2 weekly downloads. As such, baseball popularity was classified as not popular.
We found that baseball demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.