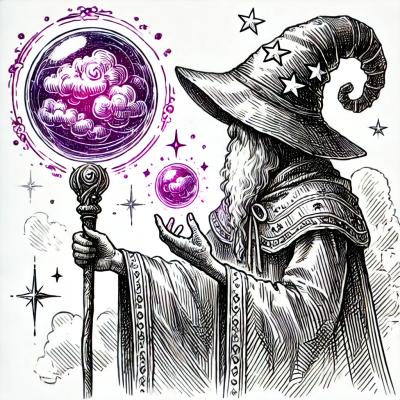
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
bass-clarinet
Advanced tools
SAX based evented streaming JSON parser in Typescript (browser and node)
bass-clarinet
is a JSON parser.
It was forked from clarinet
but the API has been changed significantly.
In addition to the port to TypeScript, the following changes have been made:
onopenobject
no longer includes the first keyJSONTestSuite
is added to the test set. All tests pass.trim
and normalize
options have been dropped. This can be handled by the consumer in the onsimplevalue
callbackcreateStackedDataSubscriber
which pairs onopenobject
/oncloseobject
and onopenarray
/onclosearray
events in a callbackattachStictJSONValidator
to the parser):
spaces_per_tab
bass-clarinet
is a sax-like streaming parser for JSON. works in the browser and node.js. just like you shouldn't use sax
when you need dom
you shouldn't use bass-clarinet
when you need JSON.parse
.
Clear reasons to use bass-clarinet
over the built-in JSON.parse
:
options
belowbass-clarinet
is very much like yajl but written in TypeScript:
npm install bass-clarinet
.ts
file: import * as bc from "bass-clarinet"
//a simple pretty printer
import * as bc from "bass-clarinet"
import * as fs from "fs"
const [, , path] = process.argv
if (path === undefined) {
console.error("missing path")
process.exit(1)
}
const data = fs.readFileSync(path, {encoding: "utf-8"})
export function createValuesPrettyPrinter(indentation: string, writer: (str: string) => void): bc.ValueHandler {
return {
array: beginMetaData => {
writer(beginMetaData.openCharacter)
return {
element: () => createValuesPrettyPrinter(`${indentation}\t`, writer),
end: endMetaData => {
writer(`${indentation}${endMetaData.range}`)
},
}
},
object: metaData => {
writer(metaData.openCharacter)
return {
property: (key, _keyRange) => {
writer(`${indentation}\t"${key}": `)
return createValuesPrettyPrinter(`${indentation}\t`, writer)
},
end: endMetaData => {
writer(`${indentation}${endMetaData.range}`)
},
}
},
simpleValue: (value, metaData) => {
if (metaData.quote !== null) {
writer(`${JSON.stringify(value)}`)
} else {
writer(`${value}`)
}
},
taggedUnion: (option, _metaData) => {
writer(`| "${option}" `)
return createValuesPrettyPrinter(`${indentation}`, writer)
},
}
}
export function attachPrettyPrinter(parser: bc.Parser, indentation: string, writer: (str: string) => void) {
const datasubscriber = bc.createStackedDataSubscriber(
createValuesPrettyPrinter(indentation, writer),
error => {
console.error("FOUND STACKED DATA ERROR", error.message)
},
_comments => {
//onEnd
}
)
parser.ondata.subscribe(datasubscriber)
parser.onschemadata.subscribe(datasubscriber)
}
const prsr = new bc.Parser(
err => { console.error("FOUND PARSER ERROR", err) },
)
attachPrettyPrinter(prsr, "\r\n", str => process.stdout.write(str))
bc.tokenizeString(
prsr,
err => { console.error("FOUND TOKENIZER ERROR", err) },
data
)
import * as bc from "bass-clarinet"
import * as fs from "fs"
const [, , path] = process.argv
if (path === undefined) {
console.error("missing path")
process.exit(1)
}
const data = fs.readFileSync(path, { encoding: "utf-8" })
const parser = new bc.Parser(
err => { console.error("FOUND PARSER ERROR", err) },
)
parser.ondata.subscribe({
onComma: () => {
//place your code here
},
onColon: () => {
//place your code here
},
onLineComment: (_comment, _range) => {
//place your code here
},
onBlockComment: (_comment, _range) => {
//
},
onString: (_value, _metaData) => {
//place your code here
//in strict JSON, the value is a string, a number, null, true or false
},
onOpenTaggedUnion: _range => {
//place your code here
},
onOpenArray: _metaData => {
//place your code here
},
onCloseArray: _metaData => {
//place your code here
},
onOpenObject: _metaData => {
//place your code here
},
onCloseObject: _metaData => {
//place your code here
},
onEnd: () => {
//place your code here
},
onNewLine: () => {
//
},
onWhitespace: () => {
//
},
})
bc.tokenizeString(
parser,
err => { console.error("FOUND TOKENIZER ERROR", err) },
data,
)
import * as bc from "bass-clarinet"
import * as fs from "fs"
const [, , path] = process.argv
if (path === undefined) {
console.error("missing path")
process.exit(1)
}
const data = fs.readFileSync(path, { encoding: "utf-8" })
const parser = new bc.Parser(
err => { console.error("FOUND PARSER ERROR", err) },
)
const ec = new bc.ExpectContext(
(_message, _range) => {
throw new Error("encounterd error")
},
(_message, _range) => {
throw new Error("encounterd warning")
},
() => bc.createDummyArrayHandler(),
() => bc.createDummyObjectHandler(),
() => bc.createDummyValueHandler(),
() => bc.createDummyValueHandler(),
)
/**
* expect an object/type with 2 properties, 'prop a' and 'prop b', both numbers
*/
parser.ondata.subscribe(bc.createStackedDataSubscriber(
ec.expectType(
(_range, _comments) => {
//prepare code here
},
{
"prop a": {
onExists: _propertyMetaData => ec.expectNumber((_value, _metaData) => {
//handle 'prop a'
}),
onNotExists: null,
},
"prop b": {
onExists: () => ec.expectNumber(_value => {
//handle 'prop b'
}),
onNotExists: null,
},
},
(_hasErrors, _range, _comments) => {
//wrap up the object
}
),
error => {
if (error.context[0] === "range") {
throw new bc.RangeError(error.message, error.context[1])
} else {
throw new bc.LocationError(error.message, error.context[1])
}
},
_comments => {
//wrap up the document
}
))
bc.tokenizeString(
parser,
err => { console.error("FOUND TOKENIZER ERROR", err) },
data
)
pass the following argument to the tokenizer function:
spaces_per_tab
- number. needed for proper column info.: Rationale: without knowing how many spaces per tab base-clarinet
is not able to determine the colomn of a character. Default is 4
(ofcourse)pass the following arguments to the parser function. all are optional.
opt
- object bag of settings.
write
- write bytes to the tokenizer. you don't have to do this all at
once. you can keep writing as much as you want.
end
- ends the stream. once ended, no more data may be written, it signals the onend
event.
the parser supports the following additional (to JSON) features
}
or the ]
. Rationale: for serializers it is easier to write a comma for every property/element instead of keeping a state that tracks if a property/element is the first one.//
and block comments /* */
. Rationale: when using JSON-like documents for editing, it is often useful to add comments'
in place of "
. Rationale: In an editor this is less intrusive (although only slightly)<
and >
in place of [
and ]
. Rationale: a semantic distinction can be made between fixed length arrays (ArrayType
) and variable length arrays (lists
)(
and )
in place of {
and }
. Rationale: a semantic distinction can be made between objctes with known properties (Type
) and objects with dynamic keys (dictionary
)!
followed by a value (object
, string
etc), followed by an optional #
(indicating compact
).
compact
is an indicator for a processor (code that uses bass-clarinet
's API) that the data is compact
. base-clarinet
only sends the compact
flag but does not change any other behaviour. Rationale: If a schema is known, the keys of a Type
are known at design time. these types can therefor be converted to ArrayTypes
and thus omit the keys without losing information. This trades in readability in favor of size. This option indicates that this happened in this document. The file can only be properly interpreted by a processor in combination with the schema.| "the chosen option" { "my data": "foo" }
. The same information can ofcourse also be written in strict JSON with an array with 2 elements of which the first element is a string.onerror
(passed as argument to the constructor) - indication that something bad happened. The parser will continue as good as it can
the data subscriber can be seen in the example code above:
check issues
everyone is welcome to contribute. patches, bug-fixes, new features
bass-clarinet
git checkout -b my_branch
git push origin my_branch
git clone git://github.com/corno/bass-clarinet.git
FAQs
Unknown package
The npm package bass-clarinet receives a total of 0 weekly downloads. As such, bass-clarinet popularity was classified as not popular.
We found that bass-clarinet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.