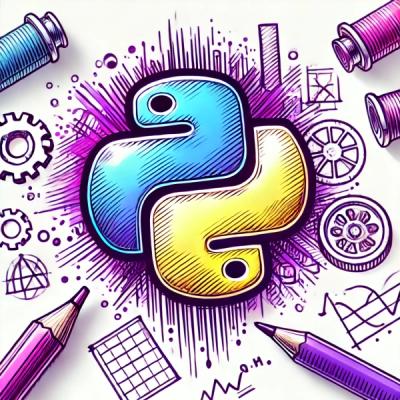
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
battlenet-api
Advanced tools
A Node JS wrapper for the Battle.net API
Add battlenet-api
to your application's package.json
file and run:
npm install
Alternatively:
npm install battlenet-api --save
Simply require()
the Battle.net API within your application:
var bnet = require('battlenet-api');
And then access the API methods to request data:
bnet.wow.character.profile(obj, callback);
Your private Battle.net API key is input with the BATTLENET_API_KEY
environment variable. This must be present in order to get a proper response. There are a variety of ways to set this variable but the easiest is to run your node server with the variable from the command line.
$ sudo BATTLENET_API_KEY=[your_api_key] node server.js
Each API method receives a parameters object for the request, and a callback function to execute once the request has completed. The available request parameters are explained for each method below.
callback
takes three arguments: error
, response
, and body
.
The World of Warcraft API methods are available through the wow
object of the Battle.net API.
var wow = bnet.wow;
ALL API methods take origin
as one of the parameters. This indicates which regional API endpoint to use. The possible values are us
, eu
, kr
, tw
. The China API is unavailable at this time.
Parameters
origin
[us
, eu
, kr
, tw
]
id
the unique achievement ID.
Usage
bnet.wow.achievement({origin: 'us', id: 2144}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
realm
the slugified realm name.
Usage
bnet.wow.auction({origin: 'us', realm: 'proudmoore'}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique ID of the battle pet ability.
Usage
bnet.wow.battlePet.ability({origin: 'us', id: 640}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique ID of the battle pet species.
Usage
bnet.wow.battlePet.species({origin: 'us', id: 258}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique ID of the battle pet species.
fields
an object containing the battle pet level
, breedId
, and qualityId
Usage
bnet.wow.battlePet.stats({origin: 'us', id: 258, fields: { level: 25, breedId: 5, qualityId: 4 }}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
realm
the slugified realm name.
Usage
bnet.wow.challenge.realmLeaderboard({origin: 'us', realm: 'proudmoore'}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
Usage
bnet.wow.challenge.regionLeaderboard({origin: 'us'}, callback);
All character requests require the following parameters:
origin
[us
, eu
, kr
, tw
].
realm
the slugified realm of the character.
name
the name of the character.
Returns basic profile data about the character.
Usage
bnet.wow.character.profile({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the achievement data of the character.
Usage
bnet.wow.character.achievements({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the appearance data of the character.
Usage
bnet.wow.character.appearance({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the guild data of the character.
Usage
bnet.wow.character.guild({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the hunter pet data of the character (where applicable).
Usage
bnet.wow.character.hunterPets({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the item data of the character.
Usage
bnet.wow.character.items({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the mount data of the character.
Usage
bnet.wow.character.mounts({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the pet data of the character.
Usage
bnet.wow.character.pets({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the pet slots data of the character.
Usage
bnet.wow.character.petSlots({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the progression data of the character.
Usage
bnet.wow.character.progression({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the PVP data of the character.
Usage
bnet.wow.character.pvp({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the quest data of the character.
Usage
bnet.wow.character.quests({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the reputation data of the character.
Usage
bnet.wow.character.reputation({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the statistics data of the character.
Usage
bnet.wow.character.stats({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the talent data of the character.
Usage
bnet.wow.character.talents({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the title data of the character.
Usage
bnet.wow.character.titles({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns an audit of the character's equipment.
Usage
bnet.wow.character.audit({origin: 'us', realm: 'proudmoore', name: 'charni'}, callback);
Returns the specified character fields aggregated in a single request.
Parameters
fields
an array of one or more character fields.
Usage
bnet.wow.character.aggregate({origin: 'us', realm: 'proudmoore', name: 'charni', fields: ['pets', 'petSlots']}, callback);
Returns the item data of the specified item id.
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique item id.
Usage
bnet.wow.item.item({origin: 'us', id: 18803}, callback);
Returns the item set data of the specified set id.
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique item set id.
Usage
bnet.wow.item.item({origin: 'us', id: 1060}, callback);
All guild requests require the following parameters:
origin
[us
, eu
, kr
, tw
].
realm
the slugified realm of the guild.
name
the name of the guild.
Returns basic profile data of the guild.
Usage
bnet.wow.guild.profile({origin: 'us', realm: 'proudmoore', name: 'black wolf mercenaries'}, callback);
Returns the members data of the guild.
Usage
bnet.wow.guild.members({origin: 'us', realm: 'proudmoore', name: 'black wolf mercenaries'}, callback);
Returns the achievement data of the guild.
Usage
bnet.wow.guild.achievements({origin: 'us', realm: 'proudmoore', name: 'black wolf mercenaries'}, callback);
Returns the news data of the guild.
Usage
bnet.wow.guild.news({origin: 'us', realm: 'proudmoore', name: 'black wolf mercenaries'}, callback);
Returns the challenge data of the guild.
Usage
bnet.wow.guild.challenge({origin: 'us', realm: 'proudmoore', name: 'black wolf mercenaries'}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
bracket
[2v2
, 3v3
, 5v5
, rbg
]
Usage
bnet.wow.pvp({origin: 'us', bracket: '2v2'}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique quest id.
Usage
bnet.wow.quest({origin: 'us', quest: 13146}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
fields
[optional] an array of one or more realms to limit.
Usage
All realms
bnet.wow.realmStatus({origin: 'us']}, callback);
Selected realms
bnet.wow.realmStatus({origin: 'us', realms: ['proudmoore', 'blackrock', 'frostmourne']]}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique recipe id.
Usage
All realms
bnet.wow.recipe({origin: 'us', id: 33994]}, callback);
Parameters
origin
[us
, eu
, kr
, tw
].
id
the unique spell id.
Usage
All realms
bnet.wow.spell({origin: 'us', id: 8056]}, callback);
FAQs
A Node.JS library for the Battle.net Community Platform API
We found that battlenet-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.