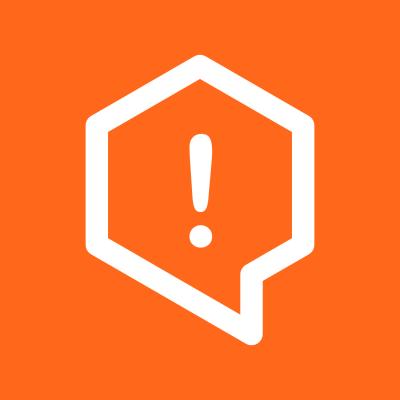
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
bbcookiemanager
Advanced tools
A simple component that implements the 2B Advice Cookie Consent Frameworks.
The Cookie Consent Manager from 2B Advice is a Processing Activity for the PrIME Data Privacy Software system. Cookie Consent Manager provides comprehensive management of website cookies in compliance with applicable privacy laws. As a part of that compliance, the Consent Manager provides three JavaScript snippets that must be integrated into the website.
Visit 2B Advice website.
Just install the component by NPM
npm install bbcookiemanager
Read cookie manager documentation.
Description
This function appends a DOM element with the “src” attribute into the parent node as the last child and sets the cookie category attribute. The appended DOM element is disabled (e.g. script DOM element has attribute “text/plain” and the iframe DOM element does not have the “src” attribute). To enable appended DOM elements, the API function “runSrcTags” must be called.
appendSrcTag(tag: HTMLBaseElement, categoryIdentifier: string, parentNode: HTMLBaseElement)
Parameters
Example
On the website page there is a button and after a click on this button two script elements and one iframe element are inserted into the website. The second script element sets the cookies which are classified as “marketing” cookies.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
var script1 = document.createElement('script');
script1.type = 'text/javascript';
script1.src = 'script1.js';
document.head.appendChild(script1);
var iframe = document.createElement('iframe');
iframe.src = 'video'
document.body.appendChild(iframe);
var script2 = document.createElement('script');
script2.type = 'text/javascript';
script2.src = 'script2.js';
var marketingContainer = document.getElementById('marketingCon tainer');
bbCookieComponent.appendSrcTag(script2[0], 'marketing', marketingContainer[0]);
}
}
Description
This function executes the callback function according to the web site visitor’s consent. The call back function is only executed if the visitor has consented to the category specified in the parameter “categoryIdentifier”.
callbackByCategory(categoryIdentifier: string, callbackFunction: Function)
Parameters
Example
On the website there is a javascript file that contains multiple functions. Some of them should only be executed if the categories “marketing” and “statistic” are enabled.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
bbCookieComponent.callbackByCategory('marketing', this.showAdvertisment);
bbCookieComponent.callbackByCategory('statistic', this.makeStatistic);
}
makeStatistic() {
document.cookie = 'StatisticCookie=valueStatisticCookie;expi res=Thu, 18 Dec 2030 12:00:00 UTC; path=/'
}
showAdvertisment() {
var script = document.createElement('script');
script.src = 'script.js'
document.head.appendChild(script[0]);
var iframe = document.createElement('iframe');
iframe.src = 'video'
document.body.appendChild(iframe);
}
}
Description
This function registers a callback function for the cookie consent manager. Multiple bindings for the same cookie consent event is allowed.
registerEvent(eventName: CookieEvents, eventCallback: Function)
Parameters
Example
On a website it is necessary to check the current cookie consent when visitor clicks on the button “Accept”. And if the category “statistic” is enabled then a new cookie is set for a website with geolocation.
import { Component } from '@angular/core';
import { BbCookieManagerComponent, CookieEvents } from 'bbcookiemanager';
declare let BBCookieManager: any;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
bbCookieComponent.registerEvent(CookieEvents.onAccept, function (e) {
if (BBCookieManager.consent.statistic) {
document.cookie = 'visitorGeo=EU;expires=Thu, 18 Dec 2030 12:00:00 UTC;path=/'
}
});
}
}
Description
The API function renders the cookie declaration as a last child of the parent node. The render mode must be set.
renderCookieDeclaration(parentNode: HTMLBaseElement)
Parameters
Example
The cookie declaration script is used in the HTML head tag. The cookie declaration must be rendered on a special location on the website.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
var _bbCookieComponent: BbCookieManagerComponent;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
_bbCookieComponent = bbCookieComponent;
}
insert() {
var cookieContainer = document.getElementById('cookies');
_bbCookieComponent.renderCookieDeclaration(cookieContainer[0]);
}
}
Description
The API function renders the policy declaration as a last child of the parent node. The render mode must be set
renderPolicyDeclaration(parentNode: HTMLBaseElement)
Parameters
Example
The policy declaration script is used in HTML head tag. The policy declaration must be rendered on a special location on the website.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
var _bbCookieComponent: BbCookieManagerComponent;
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
_bbCookieComponent = bbCookieComponent;
}
insert() {
var policyContainer = document.getElementById('policies');
_bbCookieComponent.renderPolicyDeclaration(policyContainer[0]);
}
}
Description
This function detects all tags of type=“text/plain” and enables/executes the tags according to the visitor’s consent. If script tags or any other tags with “src” attribute are not present in the website during the parsing process but there are added dynamically (e.g. by button click or in context of a SPA), then the cookie manager cannot process those elements. In this case, the scripts and src tags can be inserted to the website using the API function “appendSrcTag” or manually by setting type=“text/plain” and the appropriate category specified in the “data-bbcc” attribute. When the API function “runSrcTags” is called, it detects all tags of type “text/plain” and enables them according to the current consent.
runcSrcTags()
Example
On the website page there is a button and after a click on this button the script element and the iframe element are inserted into the website by the API function “appendSrcTag”. Those elements are disabled and to execute them the API function “runSrcTags” must be called. After calling the API function “runSrcTags” the script element is executed in case the “statistic” cookie category is enabled and the iframe element in case the “marketing” cookie category is enabled.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
var script = document.createElement('script');
script.src = 'script.js';
var iframe = document.createElement('iframe');
iframe.src = 'video';
// append DOM elements by cookie API
bbCookieComponent.appendSrcTag(script[0], 'statistic', document.head[0]);
bbCookieComponent.appendSrcTag(iframe[0], 'marketing', document.getElementById('videoContainer')[0]);
// run appended elements
bbCookieComponent.runSrcTags();
}
}
Description
The API function sets a cookie for the website. If the cookie category for the cookie is disabled, then the cookie is not set.
setCookie(name: string, value: string, hours: number)
Parameters
Example
On a website a new cookie must be set.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
bbCookieComponent.setCookie('cookieName', 'cookieValue', 24);
}
}
Description
This API function displays the cookie consent banner.
showBanner()
Example
On a website there is a button and after a click on this button the cookie consent banner appears.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
bbCookieComponent.showBanner();
}
}
Description
This API function displays the cookie consent dialog with the category selection.
showSettings()
Example
On a website page there is a button and after click on this button the cookie consent dialog appears.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
bbCookieComponent.showSettings();
}
}
Description
This API function disables all script HTML elements and HTML elements with the “src” attribute and removes website cookies which belong to categories which are not set as mandatory categories.
withdraw()
Example
On the website page there is a button and after click on this button all website cookies which are in non-mandatory categories are removed and all script HTML elements and HTML elements with “src” attribute are disabled.
import { Component } from '@angular/core';
import { BbCookieManagerComponent } from 'bbcookiemanager';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
})
export class HomeComponent {
constructor(bbCookieComponent: BbCookieManagerComponent) {
bbCookieComponent.withdraw();
}
}
Copyright 2020 2B Advice - the privacy benchmark
Permission to use, copy, modify, and/or distribute this software for any purpose with or without fee is hereby granted, provided that the above copyright notice and this permission notice appear in all copies.
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
FAQs
./README.md
The npm package bbcookiemanager receives a total of 209 weekly downloads. As such, bbcookiemanager popularity was classified as not popular.
We found that bbcookiemanager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.