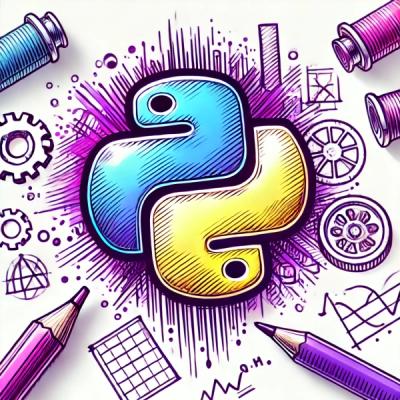
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
beautify-console-log
Advanced tools
This is a further beautification and encapsulation of the 'console' object. You can use it like using "console. log", "console. info", "console. warn", "console. error", and it can display the code line information where the log is printed.
Due to most log beautification plugins not being able to locate the code line, I implemented this library myself.
Please check the effect on the console.
npm i beautify-console-log --save
or
yarn add beautify-console-log
import BeautifyConsole from "beautify-console-log";
const log = BeautifyConsole.getInstance();
//The usage is consistent with the normal console.info()
Log.info(1234, '4', [3, 5]);
or
import BeautifyConsole from "beautify-console-log";
const log = new BeautifyConsole();
log.info(111111);
or
const log = new BeautifyConsole();
//The usage is consistent with the normal console.info()
Log.info(1234, '4', [3, 5]);
Or directly use the dist/index. js
file
<script src="./dist/index.js">
<script>
const log = BeautifyConsole.default.getInstance()
log.info(1234, '4', [3, 5])
// OR
const log2 = new BeautifyConsole.default()
log2.error(111)
</script>
const log = BeautifyConsole.default.getInstance()
log.info(1234, '4', [3, 5])
log.log(1234)
log.close().warn('no show')
log.open().log('show log')
log.error(1234)
log.setPadStartText({
title: "hello world ->",
logType: LogType.info,
}).log(1234)
const log = BeautifyConsole.getInstance();
import { LogType } from 'beautify-console-log/lib/beautify-console/model';
log.config({
title: 'example pad start text', // Log header content filled on the left
type: ['error', 'warn'], // Display partial log types, only display logs of "error" and "warn" types
})
log.info(1234, '4', [3, 5]);
log.log(1234, '4', [3, 5]);
log.warn(1234, '4', [3, 5]);
log.error(1234, '4', [3, 5]);
const log = BeautifyConsole.getInstance();
Log.info(1234, '4', [3, 5]);
Log.log(1234, '4', [3, 5]);
Log.warn(1234, '4', [3, 5]);
Log.error(1234, '4', [3, 5]);
const log = BeautifyConsole.getInstance();
log.setPadStartText({
title: "hello world ->",
logType: LogType.info,
}).info(1234,'4 ', [3, 5])
// ...
const log = BeautifyConsole.getInstance();
log.close(LogType.info);
log.close(LogType.log);
log.close(LogType.warn);
log.close(LogType.error);
log.close();
log.close().open(LogType.error);
// or
log.open(LogType.error).open(LogType.log).open(LogType.warn).open(LogType.info);
// or
log.close(LogType.error).info('closed error');
log.close(LogType.error).error('closed error');
// or
log.close(LogType.error).open(LogType.info);
log.close(LogType.error).open(LogType.info).info('info...');
// ...
const log = BeautifyConsole.getInstance();
log.open(LogType.info);
log.open(LogType.log);
log.open(LogType.warn);
log.open(LogType.error);
log.open();
log.open().close(LogType.info);
//or
log.open(LogType.error).open(LogType.log).open(LogType.warn).open(LogType.info);
// or
log.open().info('closed error');
log.open(LogType.error).error('closed error');
// or
log.close(LogType.error).open(LogType.info);
log.close(LogType.error).open(LogType.info).info('info...');
param | type | description |
---|---|---|
param | BaseConfig | |
├──title | String? | Custom log header, do not display custom log header when the value is empty |
└──type | LogType[] | ('info' 、 'log' 、 'warn' 、 'error')[] | The type of log displayed, set to only display the corresponding log type(LogType.info 、LogType.log 、LogType.warn 、LogType.error 、"info" 、"log" 、"warn" 、"error" ) |
import BeautifyConsole from "beautify-console-log";
import { LogType } from 'beautify-console-log/lib/beautify-console/model';
const log = BeautifyConsole.getInstance();
log.config({
title: 'custom title',
type: [LogType.info, LogType.error, 'log']
})
// The usage method is consistent with the normal console.info
log.info(1234, '4', [3, 5]);
// OR
const log2 = new BeautifyConsole();
log2.info(111111);
The usage method is consistent with the normal console.log
import BeautifyConsole from "beautify-console-log";
const log = BeautifyConsole.getInstance();
log.log(1234, '4', [3, 5]);
log.log({
"name": "chengzan"
});
The usage method is consistent with the normal console.info
import BeautifyConsole from "beautify-console-log";
const log = BeautifyConsole.getInstance();
log.info(1234, '4', [3, 5]);
log.info({
"name": "chengzan"
});
The usage method is consistent with the normal console.warn
import BeautifyConsole from "beautify-console-log";
const log = BeautifyConsole.getInstance();
log.warn(1234, '4', [3, 5]);
log.warn('warn');
The usage method is consistent with the normal console.error
import BeautifyConsole from "beautify-console-log";
const log = BeautifyConsole.getInstance();
log.error(1234, '4', [3, 5]);
log.error('warn');
After using log.close()
to close the log, you can use log.open()
to open the corresponding log type. When opening all types of logs, no parameters are passed (support chain calling).
type | description |
---|---|
LogType | "info" | "log" | "warn" | "error" | LogType.info 、LogType.log 、LogType.warn 、LogType.error 、"info" 、"log" 、"warn" 、"error" , Or not transmitted |
import BeautifyConsole from "beautify-console-log";
import { LogType } from 'beautify-console-log/lib/beautify-console/model';
const log = BeautifyConsole.getInstance();
log.open() // Open all types of logs
// OR
log.open(LogType.info) // Open the info log
// OR
log.open(LogType.info).open('error') // Open the info log
Closing logs allows you to close all logs or a certain type of log.
type | description |
---|---|
LogType | "info" | "log" | "warn" | "error" | LogType.info 、LogType.log 、LogType.warn 、LogType.error 、"info" 、"log" 、"warn" 、"error" , Or not transmitted |
import BeautifyConsole from "beautify-console-log";
import { LogType } from 'beautify-console-log/lib/beautify-console/model';
const log = BeautifyConsole.getInstance();
log.close() // Close all types of logs
// OR
log.close(LogType.info) // Close the info log
// OR
log.close(LogType.info).open(LogType.log)
Set the text content and style of the log header
param | type | description |
---|---|---|
param | PadStartText | |
├──title | String | Custom log header |
├──logType | LogType | "info" | "log" | "warn" | "error" | LogType.info 、LogType.log 、LogType.warn 、LogType.error 、"info" 、"log" 、"warn" 、"error" |
└──style | PadStartStyle | |
├──color (ColorType | 'black' | 'red' | 'green' | 'yellow' | 'blue' | 'purple' | 'cyan' | 'white') | ColorType.black ,ColorType.red ,ColorType.green ,ColorType.yellow ,ColorType.blue ,ColorType.purple ,ColorType.cyan ,ColorType.white | |
└──bgColor (ColorType | 'black' | 'red' | 'green' | 'yellow' | 'blue' | 'purple' | 'cyan' | 'white') | ColorType.black ,ColorType.red ,ColorType.green ,ColorType.yellow ,ColorType.blue ,ColorType.purple ,ColorType.cyan ,ColorType.white |
import BeautifyConsole from "beautify-console-log";
import { LogType, ColorType } from 'beautify-console-log/lib/beautify-console/model';
const log = BeautifyConsole.getInstance();
log.close() // Close all types of logs
// OR
log.close(LogType.info) // Close the info log
// OR
log.close(LogType.info).open(LogType.log)
log.setPadStartText({
title: "hello world ->",
logType: LogType.info,
style: {
color: ColorType.yellow,
bgColor: ColorType.purple,
}
}).log(1234)
// OR
log.setPadStartText({
title: "hello world ->",
logType: LogType.info,
style: {
color: 'black',
bgColor: 'purple',
}
}).log(1234)
After setting custom log headers or closing some logs, you can reset them through log.reset()
.
import BeautifyConsole from "beautify-console-log";
import { LogType } from 'beautify-console-log/lib/beautify-console/model';
const log = BeautifyConsole.getInstance();
log.config({
title: 'custom title',
type: [LogType.info, LogType.error]
})
log.reset() // 打开所有类型日志
log.info('reset log')
{
type?: LogType[] | ('info' | 'log' | 'warn' | 'error')[]
title?: string
}
{
color?: ColorType | 'black' | 'red' | 'green' | 'yellow' | 'blue' | 'purple' | 'cyan' | 'white';
bgColor?: ColorType | 'black' | 'red' | 'green' | 'yellow' | 'blue' | 'purple' | 'cyan' | 'white';
}
{
color?: ColorType | 'black' | 'red' | 'green' | 'yellow' | 'blue' | 'purple' | 'cyan' | 'white';
bgColor?: ColorType | 'black' | 'red' | 'green' | 'yellow' | 'blue' | 'purple' | 'cyan' | 'white';
}
{
title: string;
logType: LogType | 'info' | 'log' | 'warn' | 'error';
style?: PadStartStyle;
}
{
black = 90,
red = 91,
green = 92,
yellow = 93,
blue = 94,
purple = 95,
cyan = 96,
white = 97,
}
{
info = "info",
warn = "warn",
error = "error",
log = "log",
}
import BeautifyConsole from "beautify-console-log";
import { formatConsoleStr } from 'beautify-console-log/lib/utils';
// node.js
log.info(formatConsoleStr('string=%s number=%d', 'string', 1).join(''))
// browser
log.info(...formatConsoleStr('string=%s number=%d', 'string', 1))
Fork warehouse
Create a new Feat_Xxx branch
Submit Code
Create a new Pull Request
FAQs
This is a further beautification and encapsulation of the 'console' object. You can use it like using "console. log", "console. info", "console. warn", "console. error", and it can display the code line information where the log is printed.
The npm package beautify-console-log receives a total of 504 weekly downloads. As such, beautify-console-log popularity was classified as not popular.
We found that beautify-console-log demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.