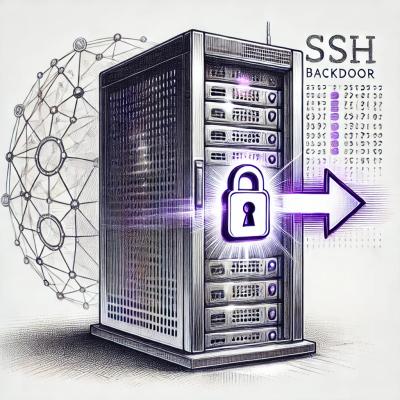
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
benchmark-tester
Advanced tools
Benchmark test runner with package loading and result printing functions.
Benchmark test runner with package loading and result printing functions.
$ npm install -D benchmark-tester benchmark lodash platform
var BenchmarkTester = require('benchmark-tester');
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title></title>
<script src="node_modules/lodash/lodash.min.js"></script>
<script src="node_modules/platform/platform.js"></script>
<script src="node_modules/benchmark/benchmark.js"></script>
<script src="node_modules/benchmark-tester/web/benchmark-tester.min.js"></script>
<script src="node_modules/benchmark-tester/web/markdown-table.js"></script>
<script src="./load-packages.js"></script><!-- Creates in "Usage" below -->
<body>
<script src="./browser-test.js"></script><!-- Creates in "Usage" below -->
</body>
</html>
Add test functions for each package and run the test.
// test.js
var BenchmarkTester = require('benchmark-tester');
new BenchmarkTester()
.addTest('lodash', function(lodash, data) { // Loads `lodash` automatically
return lodash.trim(data);
})
.runTest('Trim', ' abc ');
The result of running the above test is:
$ node test.js
Trim:
lodash x 5,919,517 ops/sec ±2.04% (79 runs sampled)
Output the result as a Markdown table.
// test.js
var BenchmarkTester = require('benchmark-tester');
new BenchmarkTester()
.addTest('lodash', function(lodash, data) {
return lodash.trim(data);
})
.runTest('Trim', ' abc ')
.print();
The result of running the above test is:
$ node test/readme-example.test.js
Trim:
lodash x 6,036,712 ops/sec ±1.59% (82 runs sampled)
| | lodash(4.17.11) |
|:-----|------------------:|
| Trim | 6,036,712 ops/sec |
- Platform: Node.js 10.8.0 on Darwin 64-bit
- Machine: Intel(R) Core(TM) i7-2620M CPU @ 2.70GHz, 16GB
A test function can be verified as follows:
var BenchmarkTester = require('benchmark-tester');
var assert = require('assert');
var inputData = ' abc ';
var expectedData = 'abc';
new BenchmarkTester()
.addTest('lodash', function(lodash, inputData) {
return lodash.trim(inputData);
})
.verifyTest('lodash', inputData, expectedData)
.verifyTest('lodash', function(testFn, lodash) {
assert.equal(testFn(lodash, inputData), expectedData);
})
.runTest('Trim', inputData)
.print();
A package to be loaded can be added manually.
var BenchmarkTester = require('benchmark-tester');
var assert = require('assert');
var inputData = ' abc ';
var expectedData = 'abc';
new BenchmarkTester()
.addPackage('String API', String.prototype)
.addTest('lodash', function(lodash, inputData) {
return lodash.trim(inputData);
})
.addTest('String API', function(proto, inputData) {
return proto.trim.call(inputData);
})
.runTest('Trim', inputData)
.print();
The result of running the above test is:
$ node test/readme-example.test.js
Trim:
lodash x 5,962,477 ops/sec ±2.06% (81 runs sampled)
String API x 23,272,338 ops/sec ±1.55% (85 runs sampled)
| | String API | lodash(4.17.11) |
|:-----|-------------------:|------------------:|
| Trim | 23,272,338 ops/sec | 5,962,477 ops/sec |
- Platform: Node.js 10.8.0 on Darwin 64-bit
- Machine: Intel(R) Core(TM) i7-2620M CPU @ 2.70GHz, 16GB
A package can be configure as follows:
// test.js
var BenchmarkTester = require('benchmark-tester');
new BenchmarkTester()
.addTest('lodash', function(lodash, data) {
return lodash.trim(data);
})
.configPackage('lodash', function(lodash, version) {
...
})
.runTest('Trim', ' abc ')
.print();
A package test data can be converted before each test as follows:
// test.js
var BenchmarkTester = require('benchmark-tester');
new BenchmarkTester()
.addTest('lodash', function(lodash, data) {
return lodash.trim(data);
})
.setConverter('lodash', function(data, module) {
return '\t' + data + '\t';
})
.runTest('Trim', ' abc ')
.print();
Creates load-packages.js
file in the above.
var packages = {
lodash: { name: 'lodash', module: _, version: '4.17.11' },
};
BenchmarkTester.prototype._getPackage = function(name) {
return packages[name];
};
Creates browser-test.js
file in the above.
var inputData = ' abc ';
var expectedData = 'abc';
new BenchmarkTester()
.addTest('lodash', function(lodash, inputData) {
return lodash.trim(inputData);
})
.verifyTest('lodash', inputData, expectedData)
.verifyTest('String API', inputData, expectedData)
.runTest('Trim', inputData)
.print();
The result of running the above test is:
(Page's HTML fragment)
<pre id="r1540722940278">
Trim:
String API x 21,569,892 ops/sec ±2.43% (56 runs sampled)
lodash x 5,380,868 ops/sec ±2.19% (55 runs sampled)
</pre>
<pre id="r1540722952219">
| | String API | lodash(4.17.11) |
|:--------------|-------------------:|------------------:|
| Trim | 21,569,892 ops/sec | 5,380,868 ops/sec |
- Platform: Chrome 69.0.3497.100 on OS X 10.13.6 64-bit
</pre>
(Web Develper Tool)
Trim:
String API x 21,569,892 ops/sec ±2.43% (56 runs sampled)
lodash x 5,380,868 ops/sec ±2.19% (55 runs sampled)
Creates an instance of BenchmarkTester.
Runs a benchmark test about package modules added by .addTest
method.
Parameter:
Parameter | Type | Description |
---|---|---|
testTitle | string | The test title to be output. |
inputData | any | The input data to be used in a test. |
Prints a result text. In default, this program prints a result as a Markdown table.
Adds a package and a test function for it.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
testFunc | function | The test function for the package. |
The API of testFunc is as follows:
Parameter:
Parameter | Type | Description |
---|---|---|
module | object / function | The module of the package. |
inputData | any | The input data to be passed to the module. |
Verifys a test function.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
inputData | any | The input data to be passed to the module. |
expectedData | any | The expected data of the test function. |
Verifys a test function.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
verifyFunc | function | The function to verify the test function. |
The API of testFunc is as follows:
Parameter:
Parameter | Type | Description |
---|---|---|
testFunc | function | The test function for the package. |
module | object / function | The module of the package. |
Add a package module be loaded manually.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
modle | object/function | The module be loaded. |
version | string | The version of the package. |
Execute configFunc to configure a package module.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
configFunc | function | The function to configure the package module. |
The API of * configFunc* is as follows:
Parameter:
Parameter | Type | Description |
---|---|---|
modle | object/function | The package module. |
version | string | The version of the package. |
Set a test data converter. convertFunc is executed a test data before a test.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
convertFunc | function | The function to convert a test data. |
The API of convertFunc is as follows:
Parameter:
Parameter | Type | Description |
---|---|---|
testData | any | Test data passed by .runTest or .verifyTest . |
modle | object/function | The package module. |
For customizing
Is called before starting a benchmark test.
Parameter:
Parameter | Type | Description |
---|---|---|
testInfo | object | The benchmark test information. |
The properties of testInfo is as follows:
Properties:
Name | Type | Description |
---|---|---|
title | object | The test title. |
data | any | The input data for the package test function. |
Is called after ending a benchmark test.
Parameter:
Parameter | Type | Description |
---|---|---|
testInfo | object | The benchmark test information. |
The properties of testInfo is same with ._beforeTest
method.
Is called after executing each package test function.
Parameter:
Parameter | Type | Description |
---|---|---|
cycleInfo | object | The event.target of benchmark. |
testInfo | object | The benchmark test information. |
The properties of testInfo is same with ._beforeTest
method.
Formats the result text for a package test function.
Parameter:
Parameter | Type | Description |
---|---|---|
cycleInfo | object | The event.target of benchmark. |
Loads a package module and returns it.
In Web browser, this method is needed to be overriden like load-package.js
file in the example above.
The package name of the current project can be specified.
This program will find up package.json
file and load the package module automatically.
Parameter:
Parameter | Type | Description |
---|---|---|
packageName | string | The package name. |
Copyright (C) 2018 Takayuki Sato.
This program is free software under MIT License. See the file LICENSE in this distribution for more details.
FAQs
Benchmark test runner with package loading and result printing functions.
The npm package benchmark-tester receives a total of 0 weekly downloads. As such, benchmark-tester popularity was classified as not popular.
We found that benchmark-tester demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.