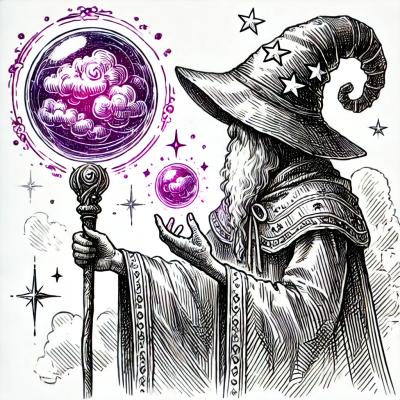
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
better-sqlite3
Advanced tools
Simple and expressive SQLite3 bindings for Node.js, with full transaction support.
better-sqlite3 is a fast and simple SQLite3 library for Node.js applications. It provides a synchronous API for interacting with SQLite databases, making it easier to write and maintain code. The library is designed to be efficient and easy to use, with a focus on performance and simplicity.
Database Connection
This feature allows you to establish a connection to an SQLite database. The `Database` constructor takes the path to the database file as an argument.
const Database = require('better-sqlite3');
const db = new Database('my-database.db');
Executing SQL Statements
This feature allows you to prepare and execute SQL statements. The `prepare` method creates a prepared statement, and the `all` method executes the statement and returns all matching rows.
const stmt = db.prepare('SELECT * FROM users WHERE age > ?');
const users = stmt.all(18);
Inserting Data
This feature allows you to insert data into the database. The `run` method executes the prepared statement with the provided parameters.
const insert = db.prepare('INSERT INTO users (name, age) VALUES (?, ?)');
const info = insert.run('John Doe', 30);
Transaction Management
This feature allows you to manage transactions. The `transaction` method creates a transaction that can execute multiple statements atomically.
const insert = db.prepare('INSERT INTO users (name, age) VALUES (?, ?)');
const insertMany = db.transaction((users) => {
for (const user of users) insert.run(user.name, user.age);
});
insertMany([{ name: 'Alice', age: 25 }, { name: 'Bob', age: 35 }]);
Custom Functions
This feature allows you to define custom SQL functions. The `function` method registers a new function that can be used in SQL statements.
db.function('add', (a, b) => a + b);
const result = db.prepare('SELECT add(2, 3)').get();
The `sqlite3` package is another popular SQLite library for Node.js. Unlike better-sqlite3, it provides an asynchronous API, which can be beneficial for non-blocking operations. However, it can be more complex to use due to the asynchronous nature of its API.
The `node-sqlite3` package is similar to `sqlite3` and provides an asynchronous API for SQLite. It is widely used and well-documented, but like `sqlite3`, it can be more challenging to work with compared to the synchronous API of better-sqlite3.
The `sql.js` package is a JavaScript library that runs SQLite in the browser using Emscripten. It is useful for web applications that need to use SQLite in a client-side environment. However, it is not designed for Node.js server-side applications like better-sqlite3.
You want Node?
You want Sqlite?
You want your life to be easy?
npm install --save better-sqlite3
var Database = require('better-sqlite3');
var db = new Database('foobar.db', {memory: true, wal: false});
FAQs
The fastest and simplest library for SQLite3 in Node.js.
The npm package better-sqlite3 receives a total of 489,042 weekly downloads. As such, better-sqlite3 popularity was classified as popular.
We found that better-sqlite3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.