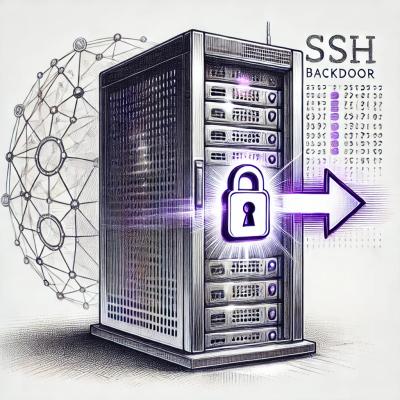
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Highly inspired by funkia/io and redux-saga, this library intends to wrap small pieces of impure code, orchestrates and tests them.
testHandler(logTwice('hello world'))
.matchIo(log('hello world'))
.matchIo(log('hello world'))
.run()
This piece of code is an assertion, an error will be throw if something go wrong :
npm install --save guillaumearm/handle-io.git
io is just a wrapper for functions and arguments. In some way, it transforms impure functions into pure functions
Conceptually, an io just could be :
const log = (...args) => [console.log, args];
but in handle-io
, it's not.
you can use io
to create one :
const { io } = require('handle-io');
const log = io(console.log);
call .run() after apply io to arguments :
log('Hello', 'World').run(); // print Hello World
keep it mind : piece of codes with .run()
cannot be tested properly.
All the idea of this library is to apply IO in structures called handlers.
A handler is a wrapped pure generator which just apply some IO and/or handlers.
e.g.
const { io, handler } = require('handle-io');
const log = io(console.log);
const logTwice = handler(function*(...args) {
yield log(...args);
yield log(...args);
});
Write a test for this handler is very simple (please see first example above).
But what about test a handler which apply IO and return values ?
There is a very simple way :
e.g.
const { io, handler } = require('handle-io');
const getEnv = io((v) => process.env[v]);
const addValues = handler(function*() {
const value1 = yield getEnv('VALUE1');
const value2 = yield getEnv('VALUE2');
return value1 + value2;
});
testHandler(addValues())
.matchIo(getEnv('VALUE1'), 32),
.matchIo(getEnv('VALUE2'), 10),
.shouldReturn(42)
.run()
Same as for IO, there is a .run() method :
addValues().run() // => 42
And same as for IO, don't use .run() everywhere in your codebase.
handlers are combinable together : you can yield a handler.
handle-io
support promises and allow you to create asynchronous IO.
e.g.
// async io
const sleep = io((ms) => new Promise(resolve => setTimeout(resolve, ms)));
// create an async combination
const sleepSecond = handler(function*(s) {
yield sleep(s * 1000);
return s;
});
// test this combination synchronously
testHander(sleepSecond(42))
.matchIo(sleep(42000))
.shouldReturn(42)
.run()
Please note sleep(n)
and sleepSecond(n)
will expose .run() method that return a promise.
e.g.
sleepSecond(1).run().then((n) => {
console.log(`${n} second(s) waited`);
})
The very simple way to handle errors with handle-io
is to use try/catch blocks.
As you can see in the example below, you can try/catch any errors inside a handler :
e.g.
const handler1 = handler(function*() {
throw new Error();
});
// Synchronous IO
const io1 = io(() => { throw new Error() });
// Asynchronous IO
const io2 = io(() => Promise.reject(new Error()));
// handler2 is safe, it can't throw because it handles errors
const handler2 = handler(function*() {
try {
yield io1();
yield io2();
yield handler1();
} catch (e) {
console.error(e);
}
});
FAQs
Fake repository for testing ci tools
We found that bidon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.