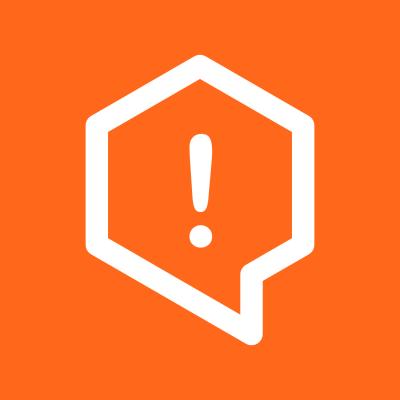
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Big-Schema is a TypeScript library designed for robust schema-based data validation. Using Big-Schema, you can easily define what your data should look like and ensure that it fits the mold. It is perfect for validating API requests, user inputs, configuration files, or any other structured data.
npm install big-schema
import { str, num, bool, obj, arr, date, union, validate } from "big-schema";
const userSchema = obj({
name: str({ required: true }),
age: num({ min: 0 }),
isAdmin: bool(),
});
const result = validate(userSchema, {
name: "John",
age: 30,
isAdmin: true
});
if (result.ok) {
console.log("Valid data: ", result.value);
} else {
console.log("Validation errors: ", result.errors);
}
StringSchema
required
: Indicates if the field is required.min
: Minimum length of the string.max
: Maximum length of the string.length
: Exact length of the string.pattern
: Regular expression the string must match.email
: Indicates the string should be a valid email.uri
: Indicates the string should be a valid URI.trim
: Indicates that the string should be trimmed.lowercase
: Indicates the string should be in lowercase.uppercase
: Indicates the string should be in uppercase.NumberSchema
required
: Indicates if the field is required.min
: Minimum value of the number.max
: Maximum value of the number.positive
: Indicates the number should be positive.negative
: Indicates the number should be negative.integer
: Indicates the number should be an integer.greater
: Specifies that the number should be greater than this value.less
: Specifies that the number should be less than this value.BooleanSchema
required
: Indicates if the field is required.oneOf
: Specifies a whitelist of boolean values.ArraySchema
required
: Indicates if the field is required.min
: Minimum number of items in the array.max
: Maximum number of items in the array.length
: Exact number of items in the array.unique
: Indicates that all items in the array should be unique.of
: Schema for validating items within the array.ObjectSchema
required
: Indicates if the field is required.keys
: Schema for validating keys within the object.unknown
: Allows properties not defined in keys
.DateSchema
required
: Indicates if the field is required.min
: Minimum date value.max
: Maximum date value.greater
: Specifies that the date should be greater than this value.less
: Specifies that the date should be less than this value.UnionSchema
of
: An array of schemas for validating the union.const productSchema = obj({
id: num({ required: true }),
name: str({ required: true, min: 1 }),
tags: arr(str({ min: 1 }), { min: 1 }),
manufacturer: obj({
name: str({ required: true }),
location: str(),
}),
});
const productData = {
id: 1,
name: "Laptop",
tags: ["Electronics", "Portable"],
manufacturer: {
name: "TechCorp",
location: "USA",
},
};
const result = validate(productSchema, productData);
const petSchema = union([
obj({
type: str({ required: true, pattern: /^Dog$/ }),
breed: str({ required: true }),
}),
obj({
type: str({ required: true, pattern: /^Cat$/ }),
livesLeft: num({ min: 0, max: 9 }),
}),
]);
const petData = {
type: "Cat",
livesLeft: 7,
};
const result = validate(petSchema, petData);
If you find any bugs or have a feature request, please open an issue. Contributions are welcome.
FAQs
A data validation library with support for complex schemas.
We found that big-schema demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.