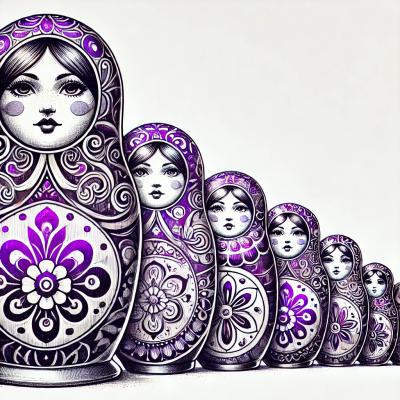
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
bind-event-listener
Advanced tools
A utility to make using
addEventListener
easier. I seem to write this again with every new project, so I made it a library
import { bind } from 'bind-event-listener';
const unbind = bind(button, {
type: 'click',
listener: onClick,
});
// when your are all done:
unbind();
import { bindAll } from 'bind-event-listener';
const unbind = bind(button, [
{
type: 'click',
listener: onClick,
options: { capture: true },
},
{
type: 'mouseover',
listener: onMouseOver,
},
]);
// when your are all done:
unbind();
When using addEventListener()
you need to remember to manually call removeEventListener()
correctly in order to unbind the event.
target.addEventListener('click', onClick, options);
// You need to remember to call removeEventListener to unbind the event
target.removeEventListener('click', onClick, options);
You need to pass in the same listener reference (onClick
), otherwise the original function will not unbind.
target.addEventListener(
'click',
function onClick() {
console.log('clicked');
},
options,
);
// This will not unbind as you have not passed in the same 'onClick' function reference
target.removeEventListener(
'click',
function onClick() {
console.log('clicked');
},
options,
);
This means you can also never unbind listeners that are arrow functions
target.addEventListener('click', () => console.log('i will never unbind'), options);
// This will not unbind as you have not passed in the same function reference
target.removeEventListener('click', () => console.log('i will never unbind'), options);
You also need to remember to pass in the same capture
value for the third argument to addEventListener
: (boolean | AddEventListenerOption
) or the event will not be unbound
// add a listener
target.addEventListener('click', onClick, { capture: true });
// forget the third value: not unbound
target.addEventListener('click', onClick);
// different capture value: not unbound
target.addEventListener('click', onClick, { capture: false });
// You need to pass in the same capture value when using the boolean capture format as well
target.addEventListener('click', onClick, true /* shorthand for {capture: true} */);
// not unbound
target.addEventListener('click', onClick);
// not unbound
target.addEventListener('click', onClick, false);
bind-event-listener
solves these problems!
bind
: basicimport { bind } from 'bind-event-listener';
const unbind = bind(button, {
type: 'click',
listener: onClick,
});
// when your are all done:
unbind();
bind
: with optionsimport { bind } from 'bind-event-listener';
const unbind = bind(button, {
type: 'click',
listener: onClick,
options: { capture: true, passive: false },
});
// when your are all done:
unbind();
bindAll
: basicimport { bindAll } from 'bind-event-listener';
const unbind = bindAll(button, [
{
type: 'click',
listener: onClick,
},
]);
// when your are all done:
unbind();
bindAll
: with optionsimport { bindAll } from 'bind-event-listener';
const unbind = bindAll(button, [
{
type: 'click',
listener: onClick,
options: { passive: true },
},
// default options that are applied to all bindings
{ capture: false },
]);
// when your are all done:
unbind();
When using defaultOptions
for bindAll
, there defaultOptions
are merged with the options
on each binding. Options on the individual bindings will take predicdent. You can think of it like this:
const merged = {
...defaultOptions,
...options,
};
Note: it is a little bit more complicated than just spreading as the library will also behave correctly when passing in a
boolean
capture argument. An options value can be a boolean (which is shorthand for{ capture: value}
FAQs
Making binding and unbinding DOM events easier
The npm package bind-event-listener receives a total of 190,029 weekly downloads. As such, bind-event-listener popularity was classified as popular.
We found that bind-event-listener demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.