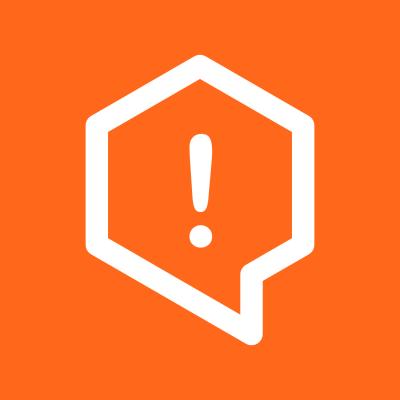
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
bison-types
Advanced tools
If you answered yes to any of the above questions, then bison-types
is for you
bison-types
allows you to define custom types.
With these custom types you can build up a message definition
Pass these types and the buffer to bison-types and it will do the rest.
For example:
var bison = require('bison-types');
var types = bison.preCompile({
'timeline': [
{ count: 'uint16' },
{ messages: 'message[count]' }
],
'message': [
{ id: 'uint8' },
{ timestamp: 'uint16' },
{ length: 'uint16' },
{ text: 'utf-8(length)' }
]
});
// time to read!
var buf = Buffer.from([0x04, 0x92, 0x04, 0x3b, 0xf4, 0x2c]);
var reader = new bison.Reader(buf, types, { bigEndian: false });
var json = reader.read('timeline');
// or write !
var buf = Buffer.alloc(1024);
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('timeline', {
count: 1,
messages: [
{
id: 3,
date: new Date().getTime(),
length: 11,
text: 'hello world'
}
]
});
Note: bison-types uses clever-buffer under the hood for all buffer manipulation.
The following types can be declared as a string, for example: {timestamp: 'uint16'}
.
uint8
- unsigned 8 bit integeruint16
- unsigned 16 bit integeruint32
- unsigned 32 bit integeruint64
- unsigned 64 bit integerint8
- signed 8 bit integerint16
- signed 16 bit integerint32
- signed 32 bit integerint64
- signed 64 bit integerutf-8
- utf-8 encoded stringlatin1
- latin1 encoded stringbool
- boolean (stored as 8 bit integer)skip
- will skip specified bytesThere is also an enumeration
type, which can be used to represent enums from arrays of objects:
will store the index in the array
var levelIndex = bison.enumeration('uint8', ['admin', 'reader', 'writer']);
// will store the value in the object
var levelCode = bison.enumeration('uint16', {
'admin': 0xb8a3,
'reader': 0xb90a,
'writer': 0xf23c
});
bison.preCompile({
'levelIndex': levelIndex,
'levelCode': levelCode,
'user': [
{ id: 'uint8' },
{ levelX: levelIndex },
{ levelY: levelCode }
]
});
There are 2 different ways that you can define a custom type
var types = bison.preCompile({
'my-other-type': [
{ a: 'uint8' },
{ b: 'uint16' }
],
'my-type': [
{ c: 'my-other-type' },
{ d: 'uint8' }
]
});
would create an object like
var myType = {c: {a: 12, b: 123}, d: 1234}
We expose the underlying clever-buffer as @buffer.
You can call any of its methods
var types = bison.preCompile({
multiply: {
_read: function(multiplier) {
return this.buffer.getUint8() * multiplier;
},
_write: function(val, multiplier) {
return this.buffer.writeUInt8(val * multiplier);
}
}
});
would multiply the value read from the buffer before returning it when reading and multiply the value to be written when writing
You need to pass in a buffer to read from, and any custom types that you may have.
You can also pass in options, look at clever-buffer for a full list of options
var bison = require('bison-types');
var buf = Buffer.from([0x01, 0x02, 0x03, 0x04]);
var types = bison.preCompile({
'my-type': [
{ a: 'uint8' },
{ b: 'uint8' },
{ c: 'uint8' },
{ d: 'uint8' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
var bison = require('bison-types');
var buf = Buffer.from([0x48, 0x45, 0x4C, 0x4C, 0x4F]);
var types = bison.preCompile({
'my-type': [
{ a: 'utf-8(5)' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
var bison = require('bison-types');
var buf = Buffer.from([0x48, 0xC9, 0x4C, 0x4C, 0x4F]);
var types = bison.preCompile({
'my-type': [
{ a: 'latin1(5)' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
var bison = require('bison-types');
var buf = Buffer.from([0x48, 0xC3, 0x89, 0x4C, 0x4C, 0x4F]);
var types = bison.preCompile({
'my-type': [
{ a: 'utf-8(6)' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
// myType = { a: 'HÉLLO' }
The power of bison-types is evident as you define more complex types
var bison = require('bison-types');
var buf = Buffer.from([0x01, 0x03, 0x04]);
var types = bison.preCompile({
'my-type': [
{ a: 'uint8' },
{ b: 'my-other-type' }
],
'my-other-type': [
{ c: 'uint8' },
{ d: 'uint8' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
You can use previously resolved values as parameters to types The power of bison-types is evident as you define more complex types
var bison = require('bison-types');
var buf = Buffer.from([0x04, 0x02]);
var types = bison.preCompile({
mult: {
_read: function(val) {
return this.buffer.getUInt8() * val;
}
},
'my-type': [
{ a: 'uint8' },
{ b: 'mult(a)' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
You can specify arrays in a similar matter
var bison = require('bison-types');
var buf = Buffer.from([0x03, 0x01, 0x02, 0x03]);
var types = bison.preCompile({
object: [
{ c: 'uint8' }
],
'my-type': [
{ a: 'uint8' },
{ b: 'object[a]' }
]
});
var reader = new bison.Reader(buf, types, { bigEndian: false });
var myType = reader.read('my-type');
// myType = { a: 3, b:[{c:1},{c:2},{c:3}] }
You need to pass in a buffer to write to, and any custom types that you may have.
You can also pass in options, look at clever-buffer for a full list of options
var bison = require('bison-types');
var buf = Buffer.alloc(4);
var types = bison.preCompile({
'my-type': [
{ a: 'uint8' },
{ b: 'uint8' },
{ c: 'uint8' },
{ d: 'uint8' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', { a: 1, b: 2, c: 3, d: 4 });
// buf will equal [ 0x01, 0x02, 0x03, 0x04 ]
var bison = require('bison-types');
var buf = Buffer.alloc(5);
var types = bison.preCompile({
'my-type': [
{ a: 'utf-8' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', { a: 'HELLO' });
// buf will equal [0x48, 0x45, 0x4C, 0x4C, 0x4F]
var bison = require('bison-types');
var buf = Buffer.alloc(5);
var types = bison.preCompile({
'my-type': [
{ a: 'latin1' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', { a: 'HÉLLO' });
// buf will equal [0x48, 0xC9, 0x4C, 0x4C, 0x4F]
var bison = require('bison-types');
var buf = Buffer.alloc(10);
var types = bison.preCompile({
'my-type': [
{ a: 'utf-8(5)' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', { a: 'HELLOWORLD' });
// buf will equal [0x48, 0x45, 0x4C, 0x4C, 0x4F, 0x00, 0x00, 0x00, 0x00, 0x00]
var bison = require('bison-types');
var buf = Buffer.alloc(6);
var types = bison.preCompile({
'my-type': [
{ a: 'utf-8' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', { a: 'HÉLLO' });
// buf will equal [0x48, 0xC3, 0x89, 0x4C, 0x4C, 0x4F]
The power of bison-types is evident as you define more complex types
var bison = require('bison-types');
var buf = Buffer.alloc(4);
var types = bison.preCompile({
'my-type': [
{ a: 'uint8' },
{ b: 'my-other-type' }
],
'my-other-type': [
{ c: 'uint8' },
{ d: 'uint8' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', {
a: 1,
b: { c: 3, d: 4 }
});
// buf will equal [ 0x01, 0x03, 0x04 ]
You can use other values as parameters to types The power of bison-types is evident as you define more complex types
var bison = require('bison-types');
var buf = Buffer.alloc(2);
var types = bison.preCompile({
div: {
_write: function(val, divider) {
return this.buffer.writeUInt8(val / divider);
}
},
'my-type': [
{ a: 'uint8' },
{ b: 'div(a)' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', { a: 4, b: 8 });
// buf will equal [ 0x04, 0x02 ]
You can specify a specific value using the following syntax
var bison = require('bison-types');
var buf = Buffer.alloc(2);
var types = bison.preCompile({
'my-type': [
{ a: 'uint8=1' },
{ b: 'uint8=2' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', {});
// buf will equal [ 0x01, 0x02 ]
You can specify arrays in a similar matter
var bison = require('bison-types');
var buf = Buffer.alloc(4);
var types = bison.preCompile({
object: [
{ c: 'uint8' }
],
'my-type': [
{ a: 'uint8' },
{ b: 'object[a]' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', {
a: 3,
b: [
{ c: 1 },
{ c: 2 },
{ c: 3 }
]
});
// buf will equal [ 0x03, 0x01, 0x02, 0x03 ]
This is a shorthand of the above example
var bison = require('bison-types');
var buf = Buffer.alloc(4);
var types = bison.preCompile({
object: [
{ c: 'uint8' }
],
'my-type': [
{ a: 'uint8=b.length' },
{ b: 'object[b.length]' }
]
});
var writer = new bison.Writer(buf, types, { bigEndian: false });
writer.write('my-type', {
b: [
{ c: 1 },
{ c: 2 },
{ c: 3 }
]
});
// buf will equal [ 0x03, 0x01, 0x02, 0x03 ]
npm test
FAQs
Convert between json and binary
We found that bison-types demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.