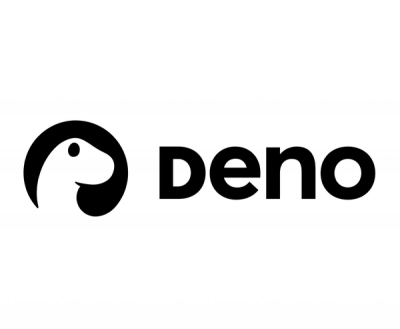
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This module provides calls to the Bitly API for Nodejs. For more information on the API request and responses visit the Bitly API docs
To install via NPM type the following: npm install bitlyapi
You can also install via git by cloning: git clone https://github.com/kishanmadhesiya/bitly.git /path/to/bitly
This library uses the API provided by bitly and requires an OAuth token to use. To get your access token, visit https://bitly.com/a/oauth_apps (under Generic Access Token)
See http://dev.bitly.com/ for format of returned objects from the API
// For ES2015/ES6
import Bitly from 'bitlyapi';
let bitly = new Bitly('<YOUR ACCESS TOKEN>');
bitly.shorten('http://nodejs.org', (response) => {
console.log(response);
}, (error) => {
console.log(error);
});
// For ES5
var Bitly = require('bitlyapi');
var bitly = new Bitly('<YOUR ACCESS TOKEN>');
bitly.shorten('https://github.com/kishanmadhesiya/bitly')
.then(function(response) {
var short_url = response.data.url
// Do something with data
}, function(error) {
throw error;
});
To run tests type npm test
. For coverage type npm run coverage
This module is limited to the following API methods:
FAQs
Bitly api
We found that bitlyapi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.