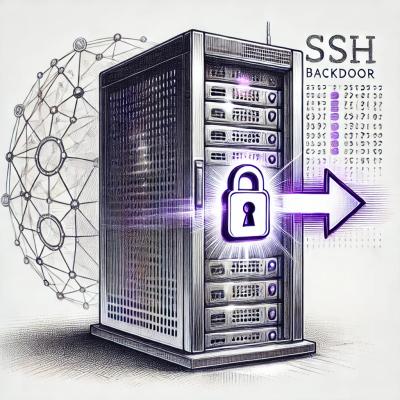
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
bitwise-operation
Advanced tools
bitwise-operation
is a JavaScript library that provides useful bitwise operation helpers without converting integer to an array.
Node.js npm install --save bitwise-operation
Require.js require(["bitwise"], ...
Require in Node var Bitwise = require('bitwise-operation');
Broser <script src="/node_modules/bitwise-operation/bitwise.js"></script>
new Bitwise(value)
Bitwise(value)
Create a bitwise object to chain operations.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0100)
.and(0b1100)
.or(0b0010)
.valueOf();
=> 0b0110
Bitwise([0, 0, 1, 0])
.valueOf();
=> 0b0100
Bitwise("1011")
.valueOf();
=> 0b1011
Bitwise.not(value) Bitwise(value).not()
Performs a logical NOT of this target bit set.
Truth table:
a | NOT a |
---|---|
0 | 1 |
1 | 0 |
Example:
var Bitwise = require('bitwise-operation')
Bitwise.not(0b1010);
Bitwise(0b1010)
.not()
.valueOf();
=> 0b0101
Bitwise.and(...values)
Bitwise(value).and(value)
Performs a logical AND of this target bit set with the argument bit set.
Truth table:
a | b | a AND b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Example:
var Bitwise = require('bitwise-operation')
Bitwise.and(0b0111, Bitwise(0b0101), 0b1100);
Bitwise(0b0111)
.and(Bitwise(0b0101))
.and(0b1100)
.valueOf();
=> 0b0100
Bitwise.nand(...values) Bitwise(value).nand(value)
Clears all of the bits in this BitSet whose corresponding bit is set in the specified BitSet.
Truth table:
a | b | a NAND b |
---|---|---|
0 | 0 | 1 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Example:
var Bitwise = require('bitwise-operation')
Bitwise.nand(0b0010, 0b0110);
Bitwise(0b0010)
.nand(0b0110)
.valueOf();
=> 0b1101
Alias: andNot()
Bitwise.or(...values)
Bitwise(value).or(value)
Performs a logical OR of this bit set with the bit set argument.
Truth table:
a | b | a OR b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Example:
var Bitwise = require('bitwise-operation')
Bitwise.or(0b0010, 0b0110);
Bitwise(0b0010)
.or(0b0110)
.valueOf();
=> 0b0110
### nor()
**Bitwise.nor(...values)**
**Bitwise(value).nor(value)**
Performs a logical **NOR** of this bit set with the bit set argument.
Truth table:
| a | b | a NOR b |
|---|---|---------|
| 0 | 0 | 1 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 0 |
Example:
```js
var Bitwise = require('bitwise-operation')
Bitwise.nor(0b0010, 0b0110);
Bitwise(0b0010)
.nor(0b0110)
.valueOf();
=> 0b1001
Bitwise.xor(...values)
Bitwise(value).xor(value)
Performs a logical XOR of this bit set with the bit set argument.
Truth table:
a | b | a XOR b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Example:
var Bitwise = require('bitwise-operation')
Bitwise.xor(0b0010, 0b0110);
Bitwise(0b0010)
.xor(0b0110)
.valueOf();
=> 0b0100
Bitwise.xnor(...values) Bitwise(value).xnor(value)
Performs a logical XNOR of this bit set with the bit set argument.
Truth table:
a | b | a XNOR b |
---|---|---|
0 | 0 | 1 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Example:
var Bitwise = require('bitwise-operation')
Bitwise.xor(0b0010, 0b0110);
Bitwise(0b0010)
.xor(0b0110)
.valueOf();
=> 0b1011
Alias: nxor()
Bitwise.mask([fromIndex= 0,] toIndex)
Bitwise(value).mask([fromIndex= 0,] toIndex)
Sets the bits not in the specified fromIndex (inclusive) to the specified toIndex (inclusive) to false.
Example:
var Bitwise = require('bitwise-operation')
Bitwise.mask(1, 5); // => 0b00011110
Bitwise(0b1011011)
.mask(1, 5) // => 0b00011110
.valueOf();
// Equal to: Bitwise(0b1011011).and(Bitwise.mask(1, 5)).valueOf()
=> 0b00011010
Bitwise(value).clear([fromIndex= 0,] toIndex)
Sets the bits from the specified fromIndex (inclusive) to the specified toIndex (inclusive) to false.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b1011011)
.clear(1, 5)
.valueOf();
=> 0b1000001
Bitwise(value).length()
Returns the "logical size" of this Bitwise: the index of the highest set bit in the Bitwise plus one.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b1011011)
.length();
=> 7
Alias: size()
Bitwise(value).set(idx [, value = true])
Sets the bit at the specified index to the specified value (default true
)
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0001)
.set(2)
.valueOf();
=> 0b0101
Bitwise(value).set(idx)
Sets the bit at the specified index to false
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.unset(0)
.valueOf();
=> 0b0100
Bitwise(value).get(idx)
Returns the value of the bit with the specified index
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0001)
.get(0)
.valueOf();
=> true
Bitwise.toggle(value, ...idx) Bitwise(value).toggle(idx)
Sets the bit at the specified index to the complement of its current value.
Example:
var Bitwise = require('bitwise-operation')
Bitwise.toggle(0b0001, 1);
Bitwise(0b0001)
.toggle(1)
.valueOf();
=> 0b0011
Bitwise.toggle(value, ...[idx1, idx2]) Bitwise(value).swap(idx1, idx2)
Swap bits to index idx1
and idx2
Example:
var Bitwise = require('bitwise-operation')
Bitwise.swap(0b0101, [1, 2]);
Bitwise(0b0101)
.swap(1, 2)
.valueOf();
=> 0b0011
Bitwise(value).equals(value)
Compares this object against the specified object
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.equals(0b0111);
=> false
Bitwise(value).setValue(value)
Replaces the current value of the object with the new value
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.setValue(0b0111)
.valueOf();
=> 0b0111
Bitwise(value).setRange(fromIndex, toIndex )
Sets the bits from the specified fromIndex
(inclusive) to the specified toIndex
(inclusive) to true
.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.setRange(1, 2)
.valueOf();
=> 0b0111
Bitwise(value).unsetRange(fromIndex, toIndex )
Sets the bits from the specified fromIndex
(inclusive) to the specified toIndex
(inclusive) to false
.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.unsetRange(1, 2)
.valueOf();
=> 0b0001
Bitwise(value).toggleRange(fromIndex, toIndex )
Sets each bit from the specified fromIndex (inclusive) to the specified toIndex (inclusive) to the complement of its current value.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.toggleRange(1, 2)
.valueOf();
=> 0b0011
Bitwise(value).copy()
Cloning this Bitwise produces a new Bitwise that is equal to it.
Example:
var Bitwise = require('bitwise-operation')
var a = Bitwise(0b0001);
var b = a;
var c = a.copy();
a.toggle(1);
a.valueOf(); // => 0b0011
b.valueOf(); // => 0b0011
c.valueOf(); // => 0b0001
Alias: clone()
Bitwise(value).valueOf()
Return the current value of this Bitwise.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.valueOf();
=> 0b0101
Bitwise(value).toString()
Bitwise(value).toString(length, separator)
Returns a string representation of this Bitwise.
Example:
var Bitwise = require('bitwise-operation')
Bitwise(0b0101)
.toString();
=> "0101"
Bitwise(571)
.toString(4, " ");
=> "10 0011 1011"
Bitwise(value).toArray()
Returns a array representation of this Bitwise.
Example:
var Bitwise = require('bitwise-operation')
var bitwise = Bitwise(571);
bitwise.toString(4, " ");
=> "10 0011 1011"
bitwise.toArray();
=> [ 1, 1, 0, 1, 1, 1, 0, 0, 0, 1 ]
Bitwise(value).cardinality()
Returns the number of bits set to true in this Bitwise.
Example:
var Bitwise = require('bitwise-operation')
var bitwise = Bitwise(571);
bitwise.toString(4, " ");
=> "10 0011 1011"
bitwise.cardinality();
=> 6
FAQs
JavaScript's bitwise operation helper library.
The npm package bitwise-operation receives a total of 1 weekly downloads. As such, bitwise-operation popularity was classified as not popular.
We found that bitwise-operation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.