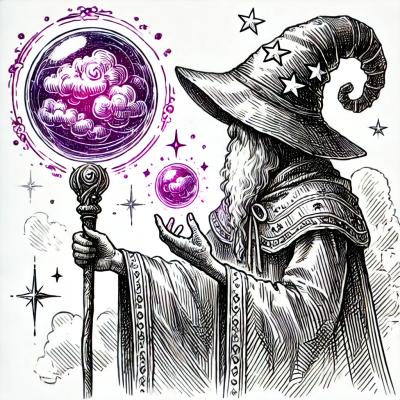
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
The blessed npm package is a high-level terminal interface library for Node.js that allows developers to create terminal applications with rich interactive UIs. It provides a way to render widgets like text boxes, lists, and forms in the terminal, handle user input, and manage layout and rendering.
Creating a basic window
This code creates a simple window (box) that is centered on the screen with a border and some styled text content. It also sets up a key listener to exit the application.
const blessed = require('blessed');
// Create a screen object.
const screen = blessed.screen({
smartCSR: true
});
// Create a box perfectly centered horizontally and vertically.
const box = blessed.box({
top: 'center',
left: 'center',
width: '50%',
height: '50%',
content: 'Hello {bold}world{/bold}!',
tags: true,
border: {
type: 'line'
},
style: {
fg: 'white',
bg: 'magenta',
border: {
fg: '#f0f0f0'
},
hover: {
bg: 'green'
}
}
});
// Append our box to the screen.
screen.append(box);
// Quit on Escape, q, or Control-C.
screen.key(['escape', 'q', 'C-c'], function(ch, key) {
return process.exit(0);
});
// Focus our element.
box.focus();
// Render the screen.
screen.render();
Handling input
This code creates an input box that allows the user to type in text. When the user submits the input, it is logged to the console, and the input box is cleared.
const blessed = require('blessed');
// Create a screen object.
const screen = blessed.screen();
// Create a box for input.
const inputBox = blessed.textbox({
top: 'center',
left: 'center',
width: '50%',
height: '10%',
inputOnFocus: true
});
// Append input box to the screen.
screen.append(inputBox);
// Focus the input box.
inputBox.focus();
// Handle submit event.
inputBox.on('submit', function(value) {
console.log(value);
inputBox.clearValue();
});
// Render the screen.
screen.render();
Creating a list
This code creates a list widget with three items. The user can navigate the list using the keyboard, and the selected item's text is logged to the console when selected.
const blessed = require('blessed');
// Create a screen object.
const screen = blessed.screen();
// Create a list.
const list = blessed.list({
items: ['Item 1', 'Item 2', 'Item 3'],
top: 'center',
left: 'center',
width: '50%',
height: '50%',
keys: true,
border: { type: 'line' },
selectedBg: 'green'
});
// Append list to the screen.
screen.append(list);
// Handle item select.
list.on('select', function(item) {
console.log(item.getText());
});
// Render the screen.
screen.render();
Inquirer.js is a common interactive command-line user interfaces library. It is more focused on asking questions and parsing input rather than creating full-fledged terminal applications with complex layouts like blessed.
Neovim is a project that seeks to aggressively refactor Vim. While not an npm package, it provides a terminal-based text editor with extensive plugin capabilities, which can be considered a more specialized application compared to the general-purpose UI toolkit that blessed offers.
Termui is a Go package for creating terminal UIs with a similar concept to blessed, but it is for the Go programming language. It provides widgets and layout management, but being in a different language ecosystem, it is not directly comparable in terms of npm package usage.
Terminal-kit is an npm package for terminal applications. It offers similar functionality to blessed, such as input handling, terminal graphics, and widgets, but with a different API design and additional features like true color support and terminal detection.
A curses-like library for node.js.
$ npm install blessed
This will render a box with line borders containing the text 'Hello world!'
,
perfectly centered horizontally and vertically.
var blessed = require('blessed');
// Create a screen object.
var screen = blessed.screen();
// Create a box perfectly centered horizontally and vertically.
var box = blessed.box({
top: 'center',
left: 'center',
width: '50%',
height: '50%',
content: 'Hello {bold}world{/bold}!',
tags: true,
border: {
type: 'line'
},
style: {
fg: 'white',
bg: 'magenta',
border: {
fg: '#f0f0f0'
},
hover: {
bg: 'green'
}
}
});
// Append our box to the screen.
screen.append(box);
// If our box is clicked, change the content.
box.on('click', function(data) {
box.setContent('{center}Some different {red-fg}content{/red-fg}.{/center}');
screen.render();
});
// If box is focused, handle `enter`/`return` and give us some more content.
box.key('enter', function(ch, key) {
box.setContent('{right}Even different {black-fg}content{/black-fg}.{/right}\n');
box.setLine(1, 'bar');
box.insertLine(1, 'foo');
screen.render();
});
// Quit on Escape, q, or Control-C.
screen.key(['escape', 'q', 'C-c'], function(ch, key) {
return process.exit(0);
});
// Focus our element.
box.focus();
// Render the screen.
screen.render();
Currently there is no mouse
or resize
event support on Windows.
Windows users will need to explicitly set term
when creating a screen like so
(NOTE: This is no longer necessary as of the latest versions of blessed.
This is now handled automatically):
var screen = blessed.screen({ term: 'windows-ansi' });
Blessed comes with a number of high-level widgets so you can avoid all the nasty low-level terminal stuff.
The base node which everything inherits from.
box
).i
.The screen on which every other node renders.
smartCSR
, but may cause flickering depending on
what is on each side of the element.back_color_erase
optimizations for terminals
that support it. it will also work with terminals that don't support it, but
only on lines with the default background color. as it stands with the current
implementation, it's uncertain how much terminal performance this adds at the
cost of overhead within node.log
method.log
option if set as a boolean.debug
method.C-c
) to ignore
when keys are locked. Useful for creating a key that will always exit no
matter whether the keys are locked.tput: true
to the Program constructor.)program.cols
).program.rows
).screen.width
.screen.height
.debug
option was set.screen.focused = el
).The base element.
{
fg: 'blue',
bg: 'black',
border: {
fg: 'blue'
},
scrollbar: {
bg: 'blue'
},
focus: {
bg: 'red'
},
hover: {
bg: 'red'
}
}
left
, center
, or right
.top
, middle
, or bottom
.left
, right
, top
, and bottom
.0-100%
), or keyword (half
or shrink
).0-100%
), or keyword (center
).
right
and bottom
do not accept keywords.
).line
or bg
). bg
by default.bg
type, default is space.fg/bg/underline
). see above.scrollable
option is enabled, Element inherits all methods
from ScrollableBox.el.on('screen', ...)
except this
will automatically cleanup listeners after the element is detached.Methods for dealing with text content, line by line. Useful for writing a text editor, irc client, etc.
Note: all of these methods deal with pre-aligned, pre-wrapped text. If you use deleteTop() on a box with a wrapped line at the top, it may remove 3-4 "real" lines (rows) depending on how long the original line was.
The lines
parameter can be a string or an array of strings. The line
parameter must be a string.
el.content
.
assume the above formatting.setContent
, but ignore tags and remove escape
codes.getContent
, but return content with tags and
escape codes removed.A box element which draws a simple box containing content
or other elements.
An element similar to Box, but geared towards rendering simple text elements.
left
, center
, or right
.Inherits all options, properties, events, and methods from Element.
A simple line which can be line
or bg
styled.
vertical
or horizontal
.style
).Inherits all options, properties, events, and methods from Box.
DEPRECATED - Use Box with the scrollable
option instead.
A box with scrollable content.
Infinity
.childOffset
. this
in turn causes the childBase to change every time the element is scrolled.ch
, fg
, and bg
properties.scrollTo
.DEPRECATED - Use Box with the scrollable
and alwaysScroll
options
instead.
A scrollable text box which can display and scroll text, as well as handle pre-existing newlines and escape codes.
keys
option.A scrollable list which can display selectable items.
style.selected.fg
).style.selected.bold
).style.item.fg
).style.item.bold
).keys
option.vi
mode is enabled and the key /
is pressed. This function accepts a callback function which should be called with the search string. The search string is then used to jump to an item that is found in items
.esc
is pressed with the keys
option).A form which can contain form elements.
A form input.
A box which allows multiline text input.
i
or enter
for insert, e
for editor,
C-e
for editor while inserting).readInput()
when the element is focused.
automatically unfocus.submit
).cancel
).$EDITOR
, read the output from
the resulting file. takes a callback which receives the final value.this.value
, for now.A box which allows text input.
*
).*
).A button which can be focused and allows key and mouse input.
press
.A progress bar allowing various styles. This can also be used as a form input.
horizontal
or vertical
.style
: e.g. style.bar.fg
).filled
.A very simple file manager for selecting files.
readdir
on cwd
and update the list items).A checkbox which can be used in a form element.
check.text = ''
).checked
.An element wrapping RadioButtons. RadioButtons within this element will be mutually exclusive with each other.
A radio button which can be used in a form element.
Offsets may be a number, a percentage (e.g. 50%
), or a keyword (e.g.
center
).
Dimensions may be a number, or a percentage (e.g. 50%
).
Positions are treated almost exactly the same as they are in CSS/CSSOM when
an element has the position: absolute
CSS property.
When an element is created, it can be given coordinates in its constructor:
var box = blessed.box({
left: 'center',
top: 'center',
bg: 'yellow',
width: '50%',
height: '50%'
});
This tells blessed to create a box, perfectly centered relative to its parent, 50% as wide and 50% as tall as its parent.
To access the calculated offsets, relative to the parent:
console.log(box.rleft);
console.log(box.rtop);
To access the calculated offsets, absolute (relative to the screen):
console.log(box.left);
console.log(box.top);
This still needs to be tested a bit, but it should work.
Every element can have text content via setContent
. If tags: true
was
passed to the element's constructor, the content can contain tags. For example:
box.setContent('hello {red-fg}{green-bg}{bold}world{/bold}{/green-bg}{/red-fg}');
To make this more concise {/}
cancels all character attributes.
box.setContent('hello {red-fg}{green-bg}{bold}world{/}');
Newlines and alignment are also possible in content.
box.setContent('hello\n'
+ '{right}world{/right}\n'
+ '{center}foo{/center}');
This will produce a box that looks like:
| hello |
| world |
| foo |
Content can also handle SGR escape codes. This means if you got output from a
program, say git log
for example, you can feed it directly to an element's
content and the colors will be parsed appropriately.
This means that while {red-fg}foo{/red-fg}
produces ^[[31mfoo^[[39m
, you
could just feed ^[[31mfoo^[[39m
directly to the content.
Events can bubble in blessed. For example:
Receiving all click events for box
:
box.on('click', function(mouse) {
box.setContent('You clicked ' + mouse.x + ', ' + mouse.y + '.');
screen.render();
});
Receiving all click events for box
, as well as all of its children:
box.on('element click', function(el, mouse) {
box.setContent('You clicked '
+ el.type + ' at ' + mouse.x + ', ' + mouse.y + '.');
screen.render();
if (el === box) {
return false; // Cancel propagation.
}
});
el
gets passed in as the first argument. It refers to the target element the
event occurred on. Returning false
will cancel propagation up the tree.
To actually render the screen buffer, you must call render
.
box.setContent('Hello world.');
screen.render();
Elements are rendered with the lower elements in the children array being painted first. In terms of the painter's algorithm, the lowest indicies in the array are the furthest away, just like in the DOM.
test/widget.js
.test/widget-pos.js
.This will actually parse the xterm terminfo and compile every string capability to a javascript function:
var blessed = require('blessed')
, tput = blessed.tput('xterm-256color');
console.log(tput.setaf(4) + 'hello' + tput.sgr0());
To play around with it on the command line, it works just like tput:
$ tput.js setaf 2
$ tput.js sgr0
$ echo "$(tput.js setaf 2)hello world$(tput.js sgr0)"
The main functionality is exposed in the main blessed
module:
var blessed = require('blessed')
, program = blessed.program();
program.key('q', function(ch, key) {
program.clear();
program.disableMouse();
program.showCursor();
program.normalBuffer();
process.exit(0);
});
program.on('mouse', function(data) {
if (data.action === 'mousemove') {
program.move(data.x, data.y);
program.bg('red');
program.write('x');
program.bg('!red');
}
});
program.alternateBuffer();
program.enableMouse();
program.hideCursor();
program.clear();
program.move(1, 1);
program.bg('black');
program.write('Hello world', 'blue fg');
program.setx((program.cols / 2 | 0) - 4);
program.down(5);
program.write('Hi again!');
program.bg('!black');
program.feed();
If you contribute code to this project, you are implicitly allowing your code
to be distributed under the MIT license. You are also implicitly verifying that
all code is your original work. </legalese>
Copyright (c) 2013, Christopher Jeffrey. (MIT License)
See LICENSE for more info.
FAQs
A high-level terminal interface library for node.js.
The npm package blessed receives a total of 1,351,381 weekly downloads. As such, blessed popularity was classified as popular.
We found that blessed demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.