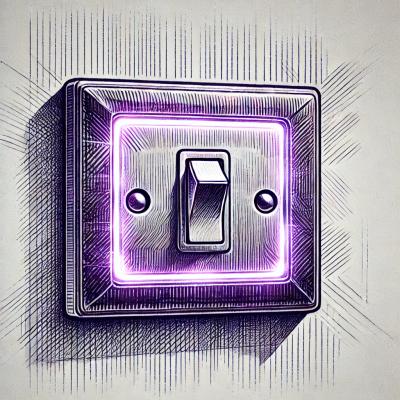
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Functional Reactive array filtering and aggregation with a MongoDB inspired syntax.
Functional Reactive array filtering and aggregation with a MongoDB inspired syntax.
For normal usage arrays are returned with the results.
var Bloc = require('bloc');
var data = [
{
id: 1,
region: 'us-east-1'
},
{
id: 2,
region: 'us-west-1'
}
];
var filter = {
region: {
$eq: 'us-east-1'
}
};
Bloc.filter(data, filter).then(function(results) {
// Results contains all items in the `us-east-1` region.
}, function(reason) {
// Something went wrong
});
If desired, a stream can be returned instead.
var Bloc = require('bloc');
var data = [
{
id: 1,
region: 'us-east-1'
},
{
id: 2,
region: 'us-west-1'
}
];
var filter = {
region: {
$eq: 'us-east-1'
}
};
Bloc.filter(data, filter, { stream: true }).then(function(stream) {
stream.subscribe(function(item) {
// Stream will eventually contain all items in the `us-east-1` region.
});
}, function(reason) {
// Something went wrong
});
Below is a list of all the supported query selectors.
Used for the comparison of different values for filters.
Matches all values that are equal to a specified value.
{
<field>: {
$eq: <value>
}
}
Matches all values that are not equal to a specified value.
{
<field>: {
$ne: <value>
}
}
Matches values that are greater than a specified value.
{
<field>: {
$gt: <value>
}
}
Matches values that are greater than or equal to a specified value.
{
<field>: {
$gte: <value>
}
}
Matches values that are less than a specified value.
{
<field>: {
$lt: <value>
}
}
Matches values that are less than or equal to a specified value.
{
<field>: {
$lte: <value>
}
}
Matches any of the values specified in an array.
{
<field>: {
$in: [ <value1>, <value2>, ... <valueN> ]
}
}
Matches none of the values specified in an array.
{
<field>: {
$nin: [ <value1>, <value2>, ... <valueN> ]
}
}
Performs a modulo operation on the value of a field and selects documents with a specified result.
{
<field>: {
$mod: [
divisor,
remainder
]
}
}
Selects documents where values match a specified regular expression.
{
<field>: {
$regex: <regular expression>
}
}
Matches documents that satisfy a JavaScript function.
{
<field>: {
$where: <function>
}
}
Used for grouping together filter clauses.
Joins query clauses with a logical OR returns all documents that match the conditions of either clause.
{
$or: [
{
<expression1>
},
{
<expression2>
},
...,
{
<expressionN>
}
]
}
Joins query clauses with a logical AND returns all documents that match the conditions of both clauses.
{
$and: [
{
<expression1>
},
{
<expression2>
},
...,
{
<expressionN>
}
]
}
Inverts the effect of a query expression and returns documents that do not match the query expression.
{
<field>: {
$not: {
<operator>: <value>
}
}
}
Joins query clauses with a logical NOR returns all documents that fail to match both clauses.
{
$nor: [
{
<expression1>
},
{
<expression2>
},
...,
{
<expressionN>
}
]
}
Operators to use when filtering arrays.
Matches arrays that contain all elements specified in the query.
{
<field>: {
$all: [
<value1>,
<value2>,
...,
<valueN>
]
}
}
Selects documents if element in the array field matches all the specified conditions.
{
<field>: {
$elemMatch: {
<query1>,
<query2>,
...,
<queryN>
}
}
}
Selects documents if the array field is a specified size.
{
<field>: {
$size: <number>
}
}
FAQs
Functional Reactive array filtering and aggregation with a MongoDB inspired syntax.
The npm package bloc receives a total of 3 weekly downloads. As such, bloc popularity was classified as not popular.
We found that bloc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.