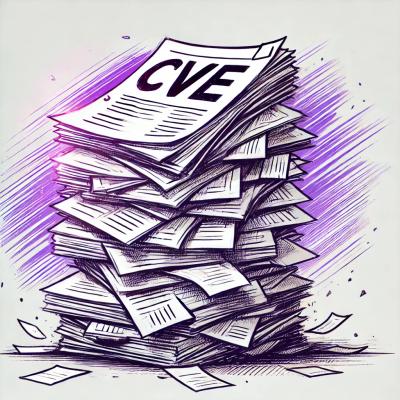
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
bloc-nodejs
Advanced tools
A Nodejs API wrapper for Bloc banking services written in typescript
Node v16 and higher is required. To make sure you have them available on your machine, try running the following command.
node -v
To get started with this SDK, create an account on Bloc if you haven't already. You can then retrieve your API keys from your Bloc dashboard.
This SDK can be installed with npm or yarn or pnpm.
# using npm
npm install bloc-nodejs
# using yarn
yarn install bloc-nodejs
# using pnpm
pnpm add bloc-nodejs
Import and Initialize the library
// use modules
import { Bloc } from 'bloc-nodejs';
// use cjs
const { Bloc } = require('bloc-nodejs')
// Instantiate the bloc class
const bloc = new Bloc('SECRET_KEY, PUBLIC_KEY');
Accounts API operations
// import the accounts interfaces from the sdk
import type { ICreateFixedAccountRequest, IAccountResponse } from 'bloc-nodejs';
const payload: ICreateFixedAccountRequest = {
// payload data
}
const response = await bloc.createFixedAccount(payload)
console.log(response) // IAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { ICreateCollectionAccountRequest, ICreateCollectionAccountResponse } from 'bloc-nodejs';
const payload: ICreateCollectionAccountRequest = {
// payload data
}
const response = await bloc.createCollectionAccount(payload)
console.log(response) // ICreateCollectionAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IGetAccountsResponse } from 'bloc-nodejs';
const response = await bloc.getAccounts(payload)
console.log(response) // IGetAccountsResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IAccountResponse } from 'bloc-nodejs';
const response = await bloc.getAccountById('account-id')
console.log(response) // IAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IGetCollectionAccountResponse } from 'bloc-nodejs';
const response = await bloc.getCollectionAccount()
console.log(response) // IGetCollectionAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IAccountResponse } from 'bloc-nodejs';
const response = await bloc.getAccountByAccountNumber('account-number')
console.log(response) // IAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IGetAccountsResponse } from 'bloc-nodejs';
const response = await bloc.getCustomerAccounts('customer-id')
console.log(response) // IGetAccountsResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IGetOrganisationDefaultAccountsResponse } from 'bloc-nodejs';
const response = await bloc.getOrganisationDefaultAccounts()
console.log(response) // IGetOrganisationDefaultAccountsResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IUpdateAccountRequest, IUpdateAccountResponse } from 'bloc-nodejs';
const payload: IUpdateAccountRequest = {
// payload data
}
const response = await bloc.freezeAccount('account-id', data)
console.log(response) // IUpdateAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IUpdateAccountRequest, IUpdateAccountResponse } from 'bloc-nodejs';
const payload: IUpdateAccountRequest = {
// payload data
}
const response = await bloc.unfreezeAccount('account-id', data)
console.log(response) // IUpdateAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IUpdateAccountRequest, IUpdateAccountResponse } from 'bloc-nodejs';
const payload: IUpdateAccountRequest = {
// payload data
}
const response = await bloc.closeAccount('account-id', data)
console.log(response) // IUpdateAccountResponse
Find more details about the parameters and response for the above method here
// import the accounts interfaces from the sdk
import type { IUpdateAccountRequest, IUpdateAccountResponse } from 'bloc-nodejs';
const payload: IUpdateAccountRequest = {
// payload data
}
const response = await bloc.reopenAccount('account-id', data)
console.log(response) // IUpdateAccountResponse
Find more details about the parameters and response for the above method here
Wallets API operations
// import the wallet interfaces from the sdk
import type { IWallet, IWalletResponse } from 'bloc-nodejs';
const payload: IWallet = {
// payload to create wallet
}
const response = await bloc.createWallet(payload)
console.log(response) // IWalletResponse
Find more details about the parameters and response for the above method here
// import the wallet interfaces from the sdk
import type { IGetWalletResponse } from 'bloc-nodejs';
const response = await bloc.getWallets()
console.log(response) // IGetWalletResponse
Find more details about the parameters and response for the above method here
// import the wallet interfaces from the sdk
import type { IWalletResponse } from 'bloc-nodejs';
const response = await bloc.getWalletById('wallet-id')
console.log(response) // IWalletResponse
Find more details about the parameters and response for the above method here
// import the wallet interfaces from the sdk
import type { IGetCustomerWalletResponse } from 'bloc-nodejs';
const response = await bloc.getCustomerWallets('customer-id')
console.log(response) // IGetCustomerWalletResponse
Find more details about the parameters and response for the above method here
// import the wallet interfaces from the sdk
import type { IDebitWallet, IGetCustomerWalletResponse } from 'bloc-nodejs';
const payload: IDebitWallet = {
// payload to debit wallet
}
const response = await bloc.debitWallet(payload)
console.log(response) // IGetCustomerWalletResponse
Find more details about the parameters and response for the above method here
Bills Payments API operations
// import the bills-payments interfaces from the sdk
import type { ISupportedBillsResponse } from 'bloc-nodejs';
const response = await bloc.getSupportedBills()
console.log(response) // ISupportedBillsResponse
Find more details about the parameters and response for the above method here
// import the bills-payments interfaces from the sdk
import type { ISupportedOperatorsResponse } from 'bloc-nodejs';
const response = await bloc.getSupportedOperators()
console.log(response) // ISupportedOperatorsResponse
Find more details about the parameters and response for the above method here
// import the bills-payments interfaces from the sdk
import type { IOperatorProductsResponse } from 'bloc-nodejs';
const response = await bloc.getOperatorProducts('operator-id')
console.log(response) // IOperatorProductsResponse
Find more details about the parameters and response for the above method here
// import the bills-payments interfaces from the sdk
import type { IGetCustomerWalletResponse } from 'bloc-nodejs';
const response = await bloc.customerDeviceValidation('operator-id')
console.log(response) // IGetCustomerWalletResponse
Find more details about the parameters and response for the above method here
// import the bills-payment interfaces from the sdk
import type { IMakePaymentResponse, IMakePaymentResponse } from 'bloc-nodejs';
const payload: IMakePaymentResponse = {
// payload to make payment
}
const response = await bloc.makePayment(payload)
console.log(response) // IMakePaymentResponse
Find more details about the parameters and response for the above method here
Disputes API operations
// import the disputes interfaces from the sdk
import type { IGetCardDisputeReasonsResponse } from 'bloc-nodejs';
const response = await bloc.getCardDisputeReasons()
console.log(response) // IGetCardDisputeReasonsResponse
Find more details about the parameters and response for the above method here
// import the disputes interfaces from the sdk
import type { ICreateCardDisputeRequest, ICardDisputeResponse } from 'bloc-nodejs';
const payload: ICreateCardDisputeRequest = {
// payload to create card dispute
}
const response = await bloc.createCardDispute(payload)
console.log(response) // ICardDisputeResponse
Find more details about the parameters and response for the above method here
// import the disputes interfaces from the sdk
import type { IGetCardDisputesResponse } from 'bloc-nodejs';
const response = await bloc.getCardDisputes()
console.log(response) // IGetCardDisputesResponse
Find more details about the parameters and response for the above method here
// import the disputes interfaces from the sdk
import type { ICardDisputeResponse } from 'bloc-nodejs';
const response = await bloc.getCardDisputeById('dispute-id')
console.log(response) // ICardDisputeResponse
Find more details about the parameters and response for the above method here
// import the disputes interfaces from the sdk
import type { IUpdateCardDisputeRequest, ICardDisputeResponse } from 'bloc-nodejs';
const payload: IUpdateCardDisputeRequest = {
// payload to update card dispute
}
const response = await bloc.updateCardDispute('dispute-id', payload)
console.log(response) // ICardDisputeResponse
Find more details about the parameters and response for the above method here
Cards API operations
// import the cards interfaces from the sdk
import type { IIssueCardRequest, ICardResponse } from 'bloc-nodejs';
const payload: IIssueCardRequest = {
// payload to issue card
}
const response = await bloc.issueCard(payload)
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { IGetCardsResponse } from 'bloc-nodejs';
const response = await bloc.getCards()
console.log(response) // IGetCardsResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { ICardResponse } from 'bloc-nodejs';
const response = await bloc.getCardById('card-id')
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { IGetCardsResponse } from 'bloc-nodejs';
const response = await bloc.getCustomerCards('customer-id')
console.log(response) // IGetCardsResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { ICardSecureDataResponse } from 'bloc-nodejs';
const response = await bloc.getCardSecureData('card-id')
console.log(response) // ICardSecureDataResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { IChangeCardPinRequest, ICardResponse } from 'bloc-nodejs';
const payload: IChangeCardPinRequest = {
// payload to change card pin
}
const response = await bloc.changeCardPIN('card-id', payload)
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { ICardResponse } from 'bloc-nodejs';
const response = await bloc.freezeCard('card-id')
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { ICardResponse } from 'bloc-nodejs';
const response = await bloc.unfreezeCard('card-id')
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { IBlockCardRequest, ICardResponse } from 'bloc-nodejs';
const payload: IBlockCardRequest = {
// payload to block card
}
const response = await bloc.blockCard('card-id', payload)
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { ILinkCardwithFixedAccountRequest, ICardResponse } from 'bloc-nodejs';
const payload: ILinkCardwithFixedAccountRequest = {
// payload to link card with fixed account
}
const response = await bloc.linkCardwithFixedAccount(payload)
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { ICardResponse } from 'bloc-nodejs';
const response = await bloc.unlinkCardwithFixedAccount('card-id')
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { IFundCardRequest, ICardResponse } from 'bloc-nodejs';
const payload: IFundCardRequest = {
// payload to fund card
}
const response = await bloc.fundCard('card-id', payload)
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
// import the cards interfaces from the sdk
import type { IWithdrawFromCardRequest, ICardResponse } from 'bloc-nodejs';
const payload: IWithdrawFromCardRequest = {
// payload to withdraw from card
}
const response = await bloc.withdrawFromCard('card-id', payload)
console.log(response) // ICardResponse
Find more details about the parameters and response for the above method here
Checkout API operations
// import the create checkout interfaces from the sdk
import type { ICreateCheckout, ICheckoutResponse } from 'bloc-nodejs';
const payload: ICreateCheckout = {
// payload to create checkout
}
const response = await bloc.createCheckout(payload)
console.log(response) // ICheckoutResponse
Find more details about the parameters and response for the above method here
Customer API operations
// import the customer interfaces from the sdk
import type { ICreateCustomer, ICreateCustomerResponse } from 'bloc-nodejs';
const payload: ICreateCustomer = {
// payload to create checkout
}
const response = await bloc.createCustomer(payload)
console.log(response) // ICreateCustomerResponse
Find more details about the parameters and response for the above method here
// import the message interfaces from the sdk
import type { IGetCustomerResponse } from 'bloc-nodejs';
const response = await bloc.getCustomers()
console.log(response) // IGetCustomerResponse
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IUpgradeCustomerToKYCT1, IUpgradeCustomerToKYCTierResponse } from 'bloc-nodejs';
const payload: IUpgradeCustomerToKYCT1 = {
// payload data
}
const response = await bloc.upgradeCustomerToKYCT1('customer-id', payload)
console.log(response) // IUpgradeCustomerToKYCTierResponse
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IUpgradeCustomerToKYCT2, IUpgradeCustomerToKYCTierResponse } from 'bloc-nodejs';
const payload: IUpgradeCustomerToKYCT2 = {
// payload data
}
const response = await bloc.upgradeCustomerToKYCT2('customer-id', payload)
console.log(response) // IUpgradeCustomerToKYCTierResponse
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IUpgradeCustomerToKYCT3, IUpgradeCustomerToKYCTierResponse } from 'bloc-nodejs';
const payload: IUpgradeCustomerToKYCT3 = {
// payload data
}
const response = await bloc.upgradeCustomerToKYCT3('customer-id', payload)
console.log(response) // IUpgradeCustomerToKYCTierResponse
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IUpdateCustomer, IUpdateCustomerResponse } from 'bloc-nodejs';
const payload: IUpdateCustomer = {
// payload data
}
const response = await bloc.updateCustomer('customer-id', payload)
console.log(response) // IUpdateCustomerResponse
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IGetCustomerByIdResponse } from 'bloc-nodejs';
const response = await bloc.getCustomerById('customer-id')
console.log(response) // IGetCustomerByIdResponse
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IMeansOfIdentification } from 'bloc-nodejs';
const response = await bloc.meansOfIdentification()
console.log(response) // IMeansOfIdentification
Find more details about the parameters and response for the above method here
// import the customer interfaces from the sdk
import type { IRevalidateCustomerKYCResponse } from 'bloc-nodejs';
const response = await bloc.revalidateCustomerKYC('customer-id')
console.log(response) // IRevalidateCustomerKYCResponse
Find more details about the parameters and response for the above method here
Miscellaneous API operations
// import the miscellaneous interfaces from the sdk
import type { IListOfBanksResponse } from 'bloc-nodejs';
const response = await bloc.getListOfBanks()
console.log(response) // IListOfBanksResponse
Find more details about the parameters and response for the above method here
// import the miscellaneous interfaces from the sdk
import type { IResolveAccountResponse } from 'bloc-nodejs';
const response = await bloc.resolveAccount()
console.log(response) // IResolveAccountResponse
Find more details about the parameters and response for the above method here
// import the miscellaneous interfaces from the sdk
import type { IGetExchangeRateResponse } from 'bloc-nodejs';
const response = await bloc.getExchangeRate('NGN-USD')
console.log(response) // IGetExchangeRateResponse
Find more details about the parameters and response for the above method here
Simulation API operations
// import the simulation interfaces from the sdk
import type { ISimulationAccount, ICreditAccountResponse } from 'bloc-nodejs';
const payload: ISimulationAccount = {
// payload data
}
const response = await bloc.creditAccount(payload)
console.log(response) // ICreditAccountResponse
Find more details about the parameters and response for the above method here
// import the simulation interfaces from the sdk
import type { ISimulationAccount, IDebitAccountResponse } from 'bloc-nodejs';
const payload: ISimulationAccount = {
// payload data
}
const response = await bloc.debitAccount(payload)
console.log(response) // IDebitAccountResponse
Find more details about the parameters and response for the above method here
Transactions API operations
// import the transactions interfaces from the sdk
import type { ITransactionResponse } from 'bloc-nodejs';
const response = await bloc.getAllTransactions()
console.log(response) // ITransactionResponse
Find more details about the parameters and response for the above method here
// import the transactions interfaces from the sdk
import type { ITransactionByReferenceResponse } from 'bloc-nodejs';
const response = await bloc.getTransactionByReference('ref_num')
console.log(response) // ITransactionByReferenceResponse
Find more details about the parameters and response for the above method here
Transfers API operations
// import the transfers interfaces from the sdk
import type { ITransferFromAFixedAccountRequest, ITransferResponse } from 'bloc-nodejs';
const payload: ITransferFromAFixedAccountRequest = {
// payload data
}
const response = await bloc.transferFromAFixedAccount(payload)
console.log(response) // ITransferResponse
Find more details about the parameters and response for the above method here
// import the transfers interfaces from the sdk
import type { ITransferFromOrganizationBalance, ITransferResponse } from 'bloc-nodejs';
const payload: ITransferFromOrganizationBalance = {
// payload data
}
const response = await bloc.transferFromOrganizationBalance(payload)
console.log(response) // ITransferResponse
Find more details about the parameters and response for the above method here
// import the transfers interfaces from the sdk
import type { IInternalTransferRequest, ITransferResponse } from 'bloc-nodejs';
const payload: IInternalTransferRequest = {
// payload data
}
const response = await bloc.internalTransfer(payload)
console.log(response) // ITransferResponse
Find more details about the parameters and response for the above method here
// import the transfers interfaces from the sdk
import type { IBulkTransferRequest, ITransferResponse } from 'bloc-nodejs';
const payload: IBulkTransferRequest = {
// payload data
}
const response = await bloc.bulkTransfer(payload)
console.log(response) // ITransferResponse
Find more details about the parameters and response for the above method here
Webhook API operations
// import the webhook interfaces from the sdk
import type { ISetWebhook, IWebhookResponse } from 'bloc-nodejs';
const payload: ISetWebhook = {
// payload data
}
const response = await bloc.setWebhook(payload)
console.log(response) // IWebhookResponse
Find more details about the parameters and response for the above method here
// import the webhook interfaces from the sdk
import type { IWebhookResponse } from 'bloc-nodejs';
const response = await bloc.getWebhook()
console.log(response) // IWebhookResponse
Find more details about the parameters and response for the above method here
Payment Link API operations
// import the payment-links interfaces from the sdk
import type { ICreatePaymentLinkRequest, IPaymentLinkResponse } from 'bloc-nodejs';
const payload: ICreatePaymentLinkRequest = {
// payload data
}
const response = await bloc.createPaymentLink(payload)
console.log(response) // IPaymentLinkResponse
Find more details about the parameters and response for the above method here
// import the payment-links interfaces from the sdk
import type { IGetPaymentLinksResponse } from 'bloc-nodejs';
const response = await bloc.getPaymentLinks()
console.log(response) // IGetPaymentLinksResponse
Find more details about the parameters and response for the above method here
// import the payment-links interfaces from the sdk
import type { IPaymentLinkResponse } from 'bloc-nodejs';
const response = await bloc.getPaymentLinkById('link-id')
console.log(response) // IPaymentLinkResponse
Find more details about the parameters and response for the above method here
// import the payment-links interfaces from the sdk
import type { IEditPaymentLinkRequest, IPaymentLinkResponse } from 'bloc-nodejs';
const payload: IEditPaymentLinkRequest = {
// payload data
}
const response = await bloc.editPaymentLink('link-id', payload)
console.log(response) // IPaymentLinkResponse
Find more details about the parameters and response for the above method here
// import the payment-links interfaces from the sdk
import type { IDeletePaymentLinksRequest, IPaymentLinkResponse } from 'bloc-nodejs';
const payload: IDeletePaymentLinksRequest = {
// payload data
}
const response = await bloc.deletePaymentLink(payload)
console.log(response) // IPaymentLinkResponse
Find more details about the parameters and response for the above method here
Beneficiaries API operations
// import the beneficiary interfaces from the sdk
import type { ICreateBeneficiary, IBeneficiaryResponse } from 'bloc-nodejs';
const payload: ICreateBeneficiary = {
// payload data
}
const response = await bloc.createBeneficiary(payload)
console.log(response) // IBeneficiaryResponse
Find more details about the parameters and response for the above method here
// import the beneficiary interfaces from the sdk
import type { IBeneficiaryResponse } from 'bloc-nodejs';
const response = await bloc.getBeneficiaryById('beneficiary-id')
console.log(response) // IBeneficiaryResponse
Find more details about the parameters and response for the above method here
// import the beneficiary interfaces from the sdk
import type { IUpdateBeneficiary, IBeneficiaryResponse } from 'bloc-nodejs';
const payload: ICreateBeneficiary = {
// payload data
}
const response = await bloc.updateBeneficiary('beneficiary-id', payload)
console.log(response) // IBeneficiaryResponse
Find more details about the parameters and response for the above method here
const response = await bloc.deleteBeneficiary('beneficiary-id')
console.log(response) // any
Find more details about the parameters and response for the above method here
FAQs
Nodejs SDK wrapper for Bloc API written with Typescript support
The npm package bloc-nodejs receives a total of 5 weekly downloads. As such, bloc-nodejs popularity was classified as not popular.
We found that bloc-nodejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.