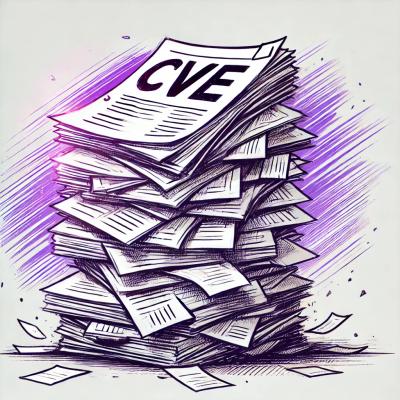
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
bloc-react
Advanced tools
[](https://codecov.io/gh/jsnanigans/bloc-react) [](https://opensource.org/licenses/MIT) [![
TypeScript BLoC pattern implementation for react using RxJS and heavily inspired by flutter_react - https://bloclibrary.dev
The BLoC Pattern (Business Logic Component) is a battle-tested design pattern for state management coming from Flutter and Dart. It tries to separate business logic from UI as much as possible while still being simple and flexible.
Everything revolves around subjects which are native to Dart, for JS there is a solid implementation by RxJS.
// CounterCubit.ts
export default class CounterCubit extends Cubit<number> {
increment = (): void => { this.emit(this.state + 1); };}
useBloc
from it// state.ts
const state = new BlocReact([new CounterCubit(0)]);
export const { useBloc } = state;
// CounterButton.tsx
import { useBloc } from "../state";
export default function CounterButton() {
const [count, { increment }] = useBloc(CounterCubit); return <button onClick={() => increment()}>count is: {count}</button>; }
[TODO]
The BlocReact
class handles the global state and manages all communication between individual BLoCs.
When initializing pass all the BLoCs for the global state in an array as first parameter.
const state = new BlocReact([new MyBloc(), new MyCubit()]);
You can add an observer to all state changes global and local
state.observer = new BlocObserver({
// onChange is called for all changes (Cubits and Blocs)
onChange: (bloc, event) => console.log({bloc, event}),
// onTransition is called only when Blocs transition from one state to another,
// it is not called for Cubits
onTransition: (bloc, event) => console.log({bloc, event}),
});
A Cubit is a simplofied version Bloc
class. Create your custom Cubit by extending the Cubit
class, pass the initial state to the super
constructor.
The Cubits' state is updated by calling the this.emit
method with the new state.
export default class CounterCubit extends Cubit<number> {
constructor() {
super(0);
}
increment = (): void => {
this.emit(this.state + 1);
};
decrement = (): void => {
this.emit(this.state - 1);
};
}
Most of the time the Cubit
class will be the easiest way to manage a peace of state but for the more critical parts of your application where there can be various reasons why the state changes to the same value, for example user authentication. It might be nice to know if the user got logged out because an error occured, the token expired or if they just clickd on the logout button.
This is especially helpful when debugging some unexpected behaviour.
in the BlocObserver
you can then use the onTransition
to see why the state changes, it will pass the previous state, the event itself and the next state.
export enum AuthEvent {
unknown = "unknown",
authenticated = "authenticated",
unauthenticated = "unauthenticated",
}
export default class AuthBloc extends Bloc<AuthEvent, boolean> {
constructor() {
super(false)
this.mapEventToState = (event) => {
switch (event) {
case AuthEvent.unknown:
return false;
case AuthEvent.unauthenticated:
return false;
case AuthEvent.authenticated:
return true;
}
};
}
In your app you can then update the state by "adding" an event. Use the useBloc
hook to get access to the BLoC class and add an event.
const Component: FC = (): ReactElement => {
const [state, bloc] = useBloc<AuthBloc>(AuthBloc);
return <>
{state === true && <Button onClick={() => bloc.add(AuthEvent.unauthenticated)}>Logout</Button>}
</>
}
[TODO: Add docs for hook]
[TODO: Add docs for hook alternative for class components]
[TODO: Add docs for local state]
FAQs
Unknown package
The npm package bloc-react receives a total of 7 weekly downloads. As such, bloc-react popularity was classified as not popular.
We found that bloc-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.