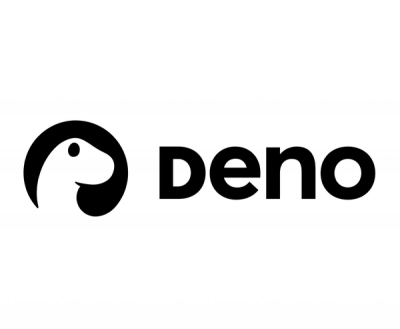
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
blooket.js
Advanced tools
modules/socket.js
, there is a typo on line 83
, causing some WebSocket issues. (Patched in Version 1.2)Run npm install blooket.js
to install Blooket.JS
To import the package, use
const Blooket = require("blooket.js")
const game = new Blooket()
The Blooket()
class can have options with it. The options you can use are below
Option | Description | Type | Values | Default Value |
---|---|---|---|---|
repeat | Once the client answers all questions, will it start over | Boolean | true/false | true |
blookcash | (Factory Mode Only) How much cash each block should give. | Integer | Any number | 100 |
blooktime | (Factory Mode Only) How long between the books generating cash | Integer (milliseconds) | Any Number | 1000 |
answertime | (Battle Royale Mode Only) How long it took the client to answer | Integer (milliseconds 1 to 20000) | Any number 1 to 20000 | 1 |
cafebonus | (Cafe Mode Only) The amount of cash to give the client after every answer. | Integer | Any number | 100 |
towerbonus | (Tower Defense Mode Only) The amount of cash to give the client after every answer. | Integer | Any number | 1 |
The options should be formatted as a JSON object like such
const game = new Blooket({
option: value
})
To join a game, run the following command
game.join(pin,name,animal)
This join function is the main function run by the user, It returns the events listed in the events section
The connect function is run by join() function, as shown in the blooket.js
file. It connected the user to the socket and game.
The startquestion function is used after NextQuestion
is emited (see NextQuestion
in Events
and NextQuestion
in Examples
), it is used to start the next question.
The answer function is used to answer the question. the a
variable can be a number 1 through 4. The answer function is called by the user after QuestionStart
is emitted. (See QuestionStart
in Events
and answer
in Examples
).
The autocorrect
function is a function to be used in place of the a
variable within the game.answer
function. So, instead of running game.answer(1,2,3 or 4)
, run game.answer(game.autocorrect())
(Only in Gold Quest) The getgold function is used to collect your prize. The p
variable can by a number 1 through 3. The prizes you can get are randomly selected (see modules/goldchance.js
). This function is run when the user handles the GetGold
event. (See GetGold
in Events
).
(Only in Gold Quest) The swap function is used to swap with a player. The player
variable should be a name of a player in the game, or a Object.keys()
function like Object.keys({variable})[0]
replacing 0
with a number 0-through the amount of players subtracted by 1 (ex: 20 players means number 0 through 19). It is used while handling the Swap
event (see Swap
in Events
and Swap and Steal
in Examples
).
(Only in Gold Quest) The rob function is used to steal from a player. The player
variable should be a name of a player in the game, or a Object.keys()
function like Object.keys({variable})[0]
replacing 0
with a number 0-through the amount of players subtracted by 1 (ex: 20 players means number 0 through 19). It is used while handling the Steal
event (see Steal
in Events
and Swap and Steal
in Examples
).
Using the BotSpam function will unleash a army of even robots to destroy the world:boom: :scream: :boom:! No it will not... yet... The BotSpam function takes all the normal join arguments (pin
, name
, animal
), and one extra, n
. This variable should be positive number. It is the number of bots to spam the game with. (The bots will not answer questions but might in the future). The BotSpam
function should be the ONLY function in the script when used. The correct way to call this function to use the following snippet
const Blooket = require("blooket.js")
const game = new Blooket()
game.BotSpam(PIN,Name,Animal, Number)
The Bot's names will be as such "Name0", "Name1", "Name2"...
The Blooket.JS class emits events at different times. Below is a brief explanation of the events
This is emitted when the client joins the game
The GameStart event is emitted when the game begins, this event does not work in Battle Royale Mode.
The QuestionStart event is emitted when a question begins. While handling this event, it is required to run the game.answer()
function.
The Correct event is emitted when a question is answered correctly.
The GetGold event is emitted when a player gets a question correct, and they are playing in gold quest mode. When handling this event, it is required to run the game.getgold()
function.
The Swap event is emitted when the game.getgold()
function returns swap
as the prize. for more prize details, see the modules/goldchance.js
file. When handling this event, it is required to run the game.swap()
function. Returns a list of players
The Steal event is emitted similarly to the Swap event, except instead running game.swap()
, it is required to run game.rob()
. Returns a list of players
The NextQuestion event is emitted when the question has ended, and the prizes (gold, cash, blooks, etc.) have been processed, when handling this event, this is required to run the game.startquestion()
function.
game.on("QuestionStart", function() {
game.answer(1) // can be 1,2,3,4
})
game.on("GetGold", function() {
game.getgold(1) // can be 1,2,3
})
game.on("Swap", function(p) {
game.swap(Object.keys(p)[0])
})
// Or
game.on("Steal", function(p) {
game.rob(Object.keys(p)[0])
})
game.on("NextQuestion", function() {
game.startquestion()
})
Powerups/Glitch
support in Factory ModeSome other projects I have created and worked on:
The First Transcontinental Railroad - Website, Repo
A website with information on the First Transcontinental Railroad, also good for a website example. (Uses examples from w3schools.)
Similar to this project, but online, it is still buggy.
EmbedCode in a website that allows to embed your source code into an iframe, with syntax highlighting and line numbering. Check the repo for more info.
FAQs
Blooket.js has been taken down per request of Blooket
The npm package blooket.js receives a total of 2 weekly downloads. As such, blooket.js popularity was classified as not popular.
We found that blooket.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.