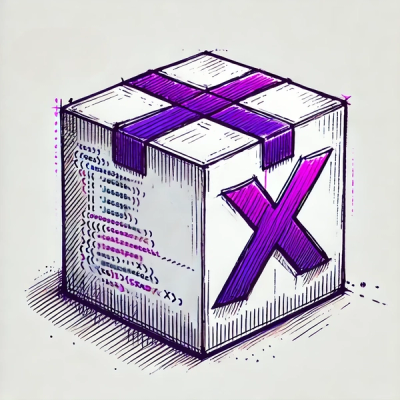
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
bnc-assist
Advanced tools
Takes care of onboarding your users, keeping them informed about transaction status and comprehensive usage analytics with minimal setup. Supports web3 versions 0.20 and 1.0.
☝️ A collection of Assist's UI elements.
👇 Assist's transaction notifications in action.
To integrate assist.js
into your dapp, you'll need to do 4 things:
onboard
Our widget is currently hosted on S3.
The library uses semantic versioning.
The current version 0.2.0.
There are minified and non-minified versions.
Put this script at the top of your <head>
<script src="https://s3.amazonaws.com/bnc-assist/0-2-0/assist.js"
integrity="sha384-wtXu8HYQaAoqqmGxbJa799ue405EZi/uNhmblOFpOQOAcL0Tk5wUCYfbELA4OvP6"
crossorigin="anonymous"></script>
<!-- OR... -->
<script src="https://s3.amazonaws.com/bnc-assist/0-2-0/assist.min.js"
integrity="sha384-1pabeMrnax5diGU6TvH3LqKx5EOLvgHy7gv0Kyh7l+qMr/YsQ82W2eXWXcAW6gfz"
crossorigin="anonymous"></script>
A module will be available on NPM in the coming weeks.
Full documentation of the config
object is below, but the minimum required config
is as follows:
var bncAssistConfig = {
dappId: dappId, // [String] The dapp ID supplied to you by Blocknative when you sign up for an account
networkId: networkId // [Integer] The network ID of the Ethereum network your dapp is deployd on.
// See below for instructions on how to setup for local blockchains.
};
var assistInstance = assist.init(bncAssistConfig);
onboard
At some point in your dapp's workflow, you'll want to ensure users have the proper environment.
This is done by calling onboard
. Some dapps will call onboard
immediately upon any page
load. Others may wait until loading certain pages or until a certain button is clicked.
In any event, it is as simple as calling:
assistInstance.onboard()
.then(function(success) {
// User has been successfully onboarded and is ready to transact
})
.catch(function(error) {
// The user exited onboarding before completion
// Will let you know what stage of onboarding the user was up to when they exited
console.log(error.msg);
})
The first three steps in the getting started flow will get your users onboarded. This step adds transaction support in order to help guide your users through a series of issues that can arise when signing transactions.
Using our decorated contracts will also enable some transaction-level metrics tracking to give you insights into things like:
Decorating your contracts is simple:
var myContract = new web3.eth.Contract(abi, address);
var myDecoratedContract = assistInstance.Contract(myContract)
// and then replace `myContract` with `myDecoratedContract`
// throughout your app
// ...
You can then use myDecoratedContract
instead of myContract
.
To speed things up, you can replace the contract after saving the original:
var myContract = new web3.eth.Contract(abi, address);
var originalMyContract = myContract;
myContract = assistInstance.Contract(myContract)
A JavaScript Object that is passed to the init
function.
var config = {
networkId: Number, // The network id of the Ethereum network your Dapp is working with (REQUIRED)
dappId: String, // The api key supplied to you by Blocknative (REQUIRED)
web3: Object, // The instantiated version of web3 that the Dapp is using
mobileBlocked: Boolean, // Defines if the Dapp works on mobile
minimumBalance: String, // Defines the minimum balance in Wei that a user needs to have to use the Dapp
messages: {
// See custom transaction messages section below for more details
txPending: Function, // Transaction is pending and awaiting confirmation
txSent: Function, // Transaction has been sent to the network
txSendFail: Function, // Transaction failed to be sent to the network
txStall: Function, // Transaction was sent but not received in the mempool after 30 secs
txFailed: Function, // Transaction failed
nsfFail: Function, // User doesn't have enough funds to complete transaction
txRepeat: Function, // Warning to user that they might be repeating a transaction
txAwaitingApproval: Function, // Warning to user that they have a previous transaction awaiting approval
txConfirmReminder: Function, // A warning to the user that their transaction is still awaiting approval
txConfirmed: Function // Transaction is confirmed
},
images: {
welcome: {
src: String, // Image URL for welcome onboard modal
srcset: String // Image URL(s) for welcome onboard modal
},
complete: {
src: String, // Image URL for complete onboard modal
srcset: String // Image URL(s) for complete onboard modal
}
}
}
The functions provided to the messages
object in the config, will be
called with the following object so that a custom string can be returned:
{
transaction: {
to: String, // The address the transaction was going to
gas: String, // Gas (wei)
gasPrice: String, // Gas price (wei)
hash: String, // The transaction hash
nonce: Number, // The transaction nonce
value: String // The value of the transaction (wei)
},
contract: {
methodName: String, // The name of the method that was called
parameters: Array // The parameters that the method was called with
}
}
var config = {
//...
messages: {
txConfirmed: function(data) {
if (data.contract.methodName === 'contribute') {
return 'Congratulations! You are now a contributor to the campaign'
}
}
}
}
The available ids for the networkId
property of the config object:
1
: mainnet3
: ropsten testnet4
: rinkeby testnetThe kovan testnet is not supported.
When you are running locally (e.g. using ganache), set networkId
to 0
.
This represents a local network.
All errors are called with eventCode
and msg
properties
{
eventCode: 'initFail',
msg: 'A api key is required to run assist'
}
init(config)
config
- Object
: Config object to configure and setup assist (Required)
The initialized version of the assist library
var assistLib = assist.init(assistConfig)
onboard()
Promise
da.onboard()
.then(function(success) {
// User has been successfully onboarded and is ready to transact
})
.catch(function(error) {
// The user exited onboarding before completion
console.log(error.msg) // Will let you know what stage of onboarding the user was up to when they exited
})
Contract(contractInstance)
contractInstance
- Object
: Instantiated web3 contract
object (Required)
A decorated contract
to be used instead of the original instance
const myContract = new web3.eth.Contract(abi, address)
const myDecoratedContract = da.Contract(myContract)
mydecoratedContract.myMethod().call()
Transaction(txObject [, callback])
txObject
- Object
: Transaction object (Required)
callback
- Function
: Optional error first style callback if you don't want to use promises
Promise
or PromiEvent
(web3.js 1.0
)
da.Transaction(txObject)
.then(txHash => {
// Transaction has been sent to the network
})
.catch(error => {
console.log(error.msg) // => 'User has insufficient funds'
})
getState()
Promise
state = {
config: Object,
legacyWeb3: Boolean,
modernWeb3: Boolean,
web3Version: String,
web3: Object,
userAgent: String,
validKey: Boolean,
newUser: Boolean,
userWelcomed: Boolean,
currentProvider: String,
validBrowser: Boolean,
web3Wallet: Boolean,
legacyWallet: Boolean,
modernWallet: Boolean,
accessToAccounts: Boolean,
walletLoggedIn: Boolean,
walletEnabled: Boolean,
walletEnableCalled: Boolean,
walletEnableCanceled: Boolean,
correctNetwork: Boolean,
userCurrentNetworkId: Number,
minimumBalance: String,
socket: Object,
socketConnection: Boolean,
accountAddress: String,
accountBalance: String,
transactionQueue: Array,
transactionAwaitingApproval: Boolean,
iframe: Object,
iframeDocument: Object,
iframeWindow: Object
}
da.getState()
.then(function(state) {
if (state.validBrowser) {
console.log('valid browser')
}
})
npm i
yarn
npm test
yarn test
npm run build
yarn build
FAQs
Blocknative Assist js library for Dapp developers
The npm package bnc-assist receives a total of 14 weekly downloads. As such, bnc-assist popularity was classified as not popular.
We found that bnc-assist demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.