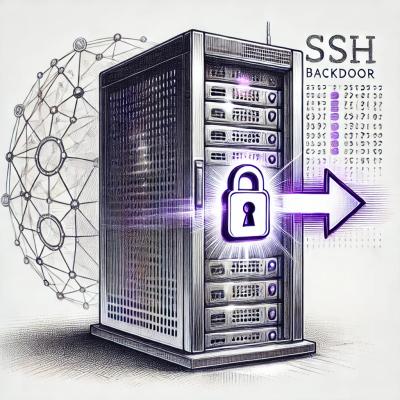
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Utilities for working with Lightning Network BOLT 07
Sample code for working with bolt07 utility functions:
x
delimited format designed to be readable.const {chanFormat} = require('bolt07');
const id = '15fbe70000260000';
const number = '1584113681139367936';
try {
const fromNumber = chanFormat({number}).channel;
// fromNumber === '1440743x38x0'
} catch (err) {
// valid channel, no error
}
try {
const fromId = chanFormat({id}).channel;
// fromId === '1440743x38x0'
} catch (err) {
// valid id, no error
}
const {chanNumber} = require('bolt07');
const channel = '1440743x38x0';
const id = '15fbe70000260000';
try {
const fromChannel = chanNumber({channel}).number;
// fromChannel === '1584113681139367936'
} catch (err) {
// valid channel, no error
}
try {
const fromId = chanNumber({id}).number;
// fromId === '1584113681139367936'
} catch (err) {
// valid id, no error
}
Returns components of a channel id or channel number.
const {decodeChanId} = require('bolt07');
const channel = '1440743x38x0';
const id = '15fbe70000260000';
const number = '1584113681139367936';
try {
const fromChannel = decodeChanId({channel});
// fromChannel.block_height === 1440743
// fromChannel.block_index === 38
// fromChannel.output_index === 0
} catch (err) {
// valid channel, no error
}
try {
const fromId = decodeChanId({id});
// fromId.block_height === 1440743
// fromId.block_index === 38
// fromId.output_index === 0
} catch (err) {
// valid id, no error
}
try {
const fromNumber = decodeChanId({channel});
// fromNumber.block_height === 1440743
// fromNumber.block_index === 38
// fromNumber.output_index === 0
} catch (err) {
// valid number, no error
}
Returns channel id when components are specified
const {encodeChanId} = require('bolt07');
try {
const encoded = encodeChanId({
block_height: 1440743,
block_index: 38,
output_index: 0,
});
// encoded.channel === '1440743x38x0'
// encoded.id === '15fbe70000260000'
// encoded.number === '1584113681139367936'
} catch (err) {
// valid components, no error
}
Returns a raw channel id in numeric format.
const {rawChanId} = require('bolt07');
const channel = '1440743x38x0';
const number = '1584113681139367936';
try {
const idFromNumber = rawChanId({number}).id;
// idFromNumber === '15fbe70000260000'
} catch (err) {
// valid number, no error
}
try {
const idFromChannel = rawChanId({channel}).id;
// idFromChannel === '15fbe70000260000'
} catch (err) {
// valid channel, no error
}
Get channel components formatted string
{
[id]: <Raw Channel Id String>
[number]: <Number Format Channel Id String>
}
@throws
<Error>
@returns
{
channel: <Components Channel Format String>
}
Channel id in numeric format
{
[channel]: <Channel Components String>
[id]: <Channel Id Hex String>
}
@throws
<ExpectedChannelIdOrComponentsToConvertToNumber Error>
@returns
{
number: <Channel Id Number String>
}
Decode a short channel id into components
{
[channel]: <Channel Components String>
[id]: <Channel Id Hex String>
[number]: <Channel Id Number Format String>
}
@throws
<ExpectedShortChannelIdToDecode Error>
<UnexpectedErrorDecodingChannelIdNumber Error>
<UnexpectedLengthOfShortChannelId Error>
@returns
{
block_height: <Channel Funding Transaction Inclusion Block Height Number>
block_index: <Channel Funding Transaction Inclusion Block Position Number>
output_index: <Channel Funding Transaction Output Index Number>
}
Encode a short channel id from components
{
block_height: <Channel Funding Transaction Inclusion Block Height Number>
block_index: <Channel Funding Transaction Inclusion Block Position Number>
output_index: <Channel Funding Transaction Output Index Number>
}
@throws
<ExpectedBlockHeightForChannelId Error>
<ExpectedBlockIndexForChannelId Error>
<ExpectedTransactionOutputIndexForChannelId Error>
@returns
{
channel: <Channel Components Format String>
id: <Channel Id Hex String>
number: <Channel Number String>
}
Raw channel id
{
[channel]: <Channel Components String>
[number]: <Channel Id In Number Format String>
}
@throws
<Error>
@returns
{
id: <Raw Channel Id Hex String>
}
Get a route from a sequence of channels
Either next hop destination in channels or final destination is required
{
channels: [{
capacity: <Maximum Tokens Number>
[destination]: <Next Node Public Key Hex String>
id: <Standard Format Channel Id String>
policies: [{
base_fee_mtokens: <Base Fee Millitokens String>
cltv_delta: <Locktime Delta Number>
fee_rate: <Fees Charged in Millitokens Per Million Number>
is_disabled: <Channel Is Disabled Bool>
max_htlc_mtokens: <Maximum HTLC Millitokens Value String>
min_htlc_mtokens: <Minimum HTLC Millitokens Value String>
public_key: <Node Public Key String>
}]
}]
[cltv_delta]: <Final CLTV Delta Number>
[destination]: <Destination Public Key Hex String>
height: <Current Block Height Number>
[messages]: [{
type: <Message Type Number String>
value: <Message Raw Value Hex Encoded String>
}]
mtokens: <Millitokens To Send String>
[payment]: <Payment Identification Value Hex String>
[total_mtokens]: <Sum of Shards Millitokens String>
}
@throws
<Error>
@returns
{
route: {
fee: <Total Fee Tokens To Pay Number>
fee_mtokens: <Total Fee Millitokens To Pay String>
hops: [{
channel: <Standard Format Channel Id String>
channel_capacity: <Channel Capacity Tokens Number>
fee: <Fee Number>
fee_mtokens: <Fee Millitokens String>
forward: <Forward Tokens Number>
forward_mtokens: <Forward Millitokens String>
[public_key]: <Public Key Hex String>
timeout: <Timeout Block Height Number>
}]
[messages]: [{
type: <Message Type Number String>
value: <Message Raw Value Hex Encoded String>
}]
mtokens: <Total Fee-Inclusive Millitokens String>
[payment]: <Payment Identification Value Hex String>
timeout: <Timeout Block Height Number>
tokens: <Total Fee-Inclusive Tokens Number>
[total_mtokens]: <Sum of Shards Millitokens String>
}
}
Given hops to a destination, construct a payable route
{
[cltv_delta]: <Final Cltv Delta Number>
height: <Current Block Height Number>
hops: [{
base_fee_mtokens: <Base Fee Millitokens String>
channel: <Standard Format Channel Id String>
[channel_capacity]: <Channel Capacity Tokens Number>
cltv_delta: <CLTV Delta Number>
fee_rate: <Fee Rate In Millitokens Per Million Number>
public_key: <Next Hop Public Key Hex String>
}]
initial_cltv: <Initial CLTV Delta Number>
[messages]: [{
type: <Message Type Number String>
value: <Message Raw Value Hex Encoded String>
}]
mtokens: <Millitokens To Send String>
[payment]: <Payment Identification Value Hex String>
[total_mtokens]: <Total Millitokens For Sharded Payments String>
}
@throws
<Error>
@returns
{
fee: <Route Fee Tokens Number>
fee_mtokens: <Route Fee Millitokens String>
hops: [{
channel: <Standard Format Channel Id String>
channel_capacity: <Channel Capacity Tokens Number>
fee: <Fee Number>
fee_mtokens: <Fee Millitokens String>
forward: <Forward Tokens Number>
forward_mtokens: <Forward Millitokens String>
[public_key]: <Public Key Hex String>
timeout: <Timeout Block Height Number>
}]
[messages]: [{
type: <Message Type Number String>
value: <Message Raw Value Hex Encoded String>
}]
mtokens: <Total Fee-Inclusive Millitokens String>
[payment]: <Payment Identification Value Hex String>
timeout: <Timeout Block Height Number>
tokens: <Total Fee-Inclusive Tokens Number>
[total_mtokens]: <Sharded Payments Total Millitokens String>
}
FAQs
Utilities for working with bolt07 data formats
The npm package bolt07 receives a total of 5,644 weekly downloads. As such, bolt07 popularity was classified as popular.
We found that bolt07 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.