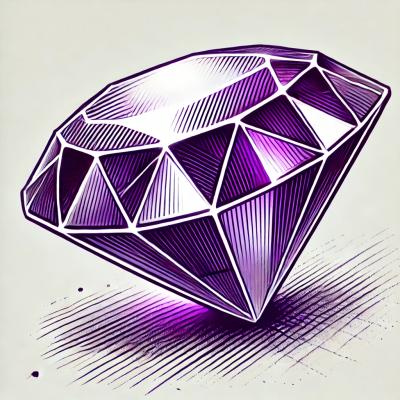
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
The borsh npm package is a JavaScript implementation of the Binary Object Representation Serializer for Hashing (BORSH) serialization format. It is used to serialize and deserialize complex data structures in a compact binary format, which is particularly useful in blockchain and other performance-critical applications.
Serialization
This feature allows you to serialize JavaScript objects into a binary format. The code sample demonstrates how to define a schema for a class and serialize an instance of that class.
const borsh = require('borsh');
class Greeting {
constructor({ message }) {
this.message = message;
}
}
const schema = new Map([
[Greeting, { kind: 'struct', fields: [['message', 'string']] }]
]);
const greeting = new Greeting({ message: 'Hello, world!' });
const serialized = borsh.serialize(schema, greeting);
console.log(serialized);
Deserialization
This feature allows you to deserialize binary data back into JavaScript objects. The code sample shows how to deserialize a binary array into an instance of a class using a predefined schema.
const borsh = require('borsh');
class Greeting {
constructor({ message }) {
this.message = message;
}
}
const schema = new Map([
[Greeting, { kind: 'struct', fields: [['message', 'string']] }]
]);
const serialized = new Uint8Array([10, 0, 0, 0, 72, 101, 108, 108, 111, 44, 32, 119, 111, 114, 108, 100, 33]);
const deserialized = borsh.deserialize(schema, Greeting, serialized);
console.log(deserialized);
protobufjs is a JavaScript implementation of Protocol Buffers, a language-neutral, platform-neutral, extensible mechanism for serializing structured data. It is similar to borsh in that it provides efficient serialization and deserialization of complex data structures, but it uses a different format and is more widely adopted in various industries.
msgpack-lite is a JavaScript implementation of the MessagePack serialization format. Like borsh, it provides a compact binary format for serializing and deserializing data. However, MessagePack is a more general-purpose format and is used in a variety of applications beyond blockchain.
avsc is a JavaScript library for working with Avro, a data serialization system. Avro is similar to borsh in that it provides a compact binary format for data serialization, but it also includes features for schema evolution and is widely used in big data applications.
Borsh JS is an implementation of the Borsh binary serialization format for JavaScript and TypeScript projects.
Borsh stands for Binary Object Representation Serializer for Hashing. It is meant to be used in security-critical projects as it prioritizes consistency, safety, speed, and comes with a strict specification.
import * as borsh from 'borsh';
const encodedU16 = borsh.serialize('u16', 2);
const decodedU16 = borsh.deserialize('u16', encodedU16);
const encodedStr = borsh.serialize('string', 'testing');
const decodedStr = borsh.deserialize('string', encodedStr);
import * as borsh from 'borsh';
const value = {x: 255, y: BigInt(20), z: '123', arr: [1, 2, 3]};
const schema = { struct: { x: 'u8', y: 'u64', 'z': 'string', 'arr': { array: { type: 'u8' }}}};
const encoded = borsh.serialize(schema, value);
const decoded = borsh.deserialize(schema, encoded);
The package exposes the following functions:
serialize(schema: Schema, obj: any): Uint8Array
- serializes an object obj
according to the schema schema
.deserialize(schema: Schema, buffer: Uint8Array, class?: Class): any
- deserializes an object according to the schema schema
from the buffer buffer
. If the optional parameter class
is present, the deserialized object will be an of class
.Schemas are used to describe the structure of the data being serialized or deserialized. They are used to validate the data and to determine the order of the fields in the serialized data.
NOTE: You can find examples of valid in the test folder.
Basic types are described by a string. The following types are supported:
u8
, u16
, u32
, u64
, u128
- unsigned integers of 8, 16, 32, 64, and 128 bits respectively.i8
, i16
, i32
, i64
, i128
- signed integers of 8, 16, 32, 64, and 128 bits respectively.f32
, f64
- IEEE 754 floating point numbers of 32 and 64 bits respectively.bool
- boolean value.string
- UTF-8 string.More complex objects are described by a JSON object. The following types are supported:
{ array: { type: Schema, len?: number } }
- an array of objects of the same type. The type of the array elements is described by the type
field. If the field len
is present, the array is fixed-size and the length of the array is len
. Otherwise, the array is dynamic-sized and the length of the array is serialized before the elements.{ option: Schema }
- an optional object. The type of the object is described by the type
field.{ map: { key: Schema, value: Schema }}
- a map. The type of the keys and values are described by the key
and value
fields respectively.{ set: Schema }
- a set. The type of the elements is described by the type
field.{ enum: [{ className1: { struct: {...} } }, { className2: { struct: {...} } }, ... ] }
- an enum. The variants of the enum are described by the className1
, className2
, etc. fields. The variants are structs.{ struct: { field1: Schema1, field2: Schema2, ... } }
- a struct. The fields of the struct are described by the field1
, field2
, etc. fields.Javascript | Borsh |
---|---|
number | u8 u16 u32 i8 i16 i32 |
bigint | u64 u128 i64 i128 |
number | f32 f64 |
number | f32 f64 |
boolean | bool |
string | UTF-8 string |
type[] | fixed-size byte array |
type[] | dynamic sized array |
object | enum |
Map | HashMap |
Set | HashSet |
null or type | Option |
Install dependencies:
yarn install
Continuously build with:
yarn dev
Run tests:
yarn test
Run linter
yarn lint
Prepare dist
version by running:
yarn build
When publishing to npm use np.
This repository is distributed under the terms of both the MIT license and the Apache License (Version 2.0). See LICENSE-MIT and LICENSE-APACHE for details.
FAQs
Binary Object Representation Serializer for Hashing
The npm package borsh receives a total of 214,655 weekly downloads. As such, borsh popularity was classified as popular.
We found that borsh demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.