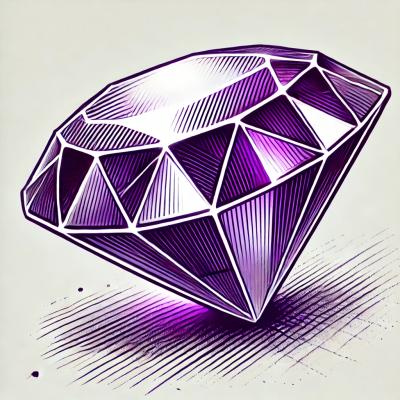
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
botbuilder
Advanced tools
Bot Builder is a framework for building rich bots on virtually any platform.
The botbuilder npm package is a comprehensive framework for building conversational AI experiences using Microsoft Bot Framework. It provides tools and libraries to create, test, and deploy chatbots across various platforms such as Microsoft Teams, Slack, and Facebook Messenger.
Creating a Simple Echo Bot
This code sample demonstrates how to create a simple echo bot using the botbuilder package. The bot listens for incoming messages and echoes back the text received.
const { ActivityHandler, BotFrameworkAdapter } = require('botbuilder');
const restify = require('restify');
const server = restify.createServer();
server.listen(process.env.port || process.env.PORT || 3978, () => {
console.log(`\n${server.name} listening to ${server.url}`);
});
const adapter = new BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
const bot = new ActivityHandler();
bot.onMessage(async (context, next) => {
await context.sendActivity(`You said '${context.activity.text}'`);
await next();
});
server.post('/api/messages', (req, res) => {
adapter.processActivity(req, res, async (context) => {
await bot.run(context);
});
});
Adding Dialogs
This code sample demonstrates how to add dialogs to a bot using the botbuilder package. The bot prompts the user for their name and then responds with a greeting.
const { ActivityHandler, BotFrameworkAdapter, DialogSet, WaterfallDialog, TextPrompt, MemoryStorage, ConversationState } = require('botbuilder');
const restify = require('restify');
const server = restify.createServer();
server.listen(process.env.port || process.env.PORT || 3978, () => {
console.log(`\n${server.name} listening to ${server.url}`);
});
const adapter = new BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
const memoryStorage = new MemoryStorage();
const conversationState = new ConversationState(memoryStorage);
const dialogState = conversationState.createProperty('dialogState');
const dialogs = new DialogSet(dialogState);
dialogs.add(new TextPrompt('textPrompt'));
dialogs.add(new WaterfallDialog('mainDialog', [
async (step) => {
return await step.prompt('textPrompt', 'What is your name?');
},
async (step) => {
await step.context.sendActivity(`Hello, ${step.result}!`);
return await step.endDialog();
}
]));
const bot = new ActivityHandler();
bot.onMessage(async (context, next) => {
const dialogContext = await dialogs.createContext(context);
const results = await dialogContext.continueDialog();
if (results.status === 'empty') {
await dialogContext.beginDialog('mainDialog');
}
await next();
});
server.post('/api/messages', (req, res) => {
adapter.processActivity(req, res, async (context) => {
await bot.run(context);
});
});
Handling Different Activity Types
This code sample demonstrates how to handle different activity types, such as messages and members being added to a conversation, using the botbuilder package.
const { ActivityHandler, BotFrameworkAdapter } = require('botbuilder');
const restify = require('restify');
const server = restify.createServer();
server.listen(process.env.port || process.env.PORT || 3978, () => {
console.log(`\n${server.name} listening to ${server.url}`);
});
const adapter = new BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
const bot = new ActivityHandler();
bot.onMessage(async (context, next) => {
await context.sendActivity(`You said '${context.activity.text}'`);
await next();
});
bot.onMembersAdded(async (context, next) => {
const membersAdded = context.activity.membersAdded;
for (let member of membersAdded) {
if (member.id !== context.activity.recipient.id) {
await context.sendActivity('Welcome to the bot!');
}
}
await next();
});
server.post('/api/messages', (req, res) => {
adapter.processActivity(req, res, async (context) => {
await bot.run(context);
});
});
Botpress is an open-source conversational AI platform that allows developers to build, deploy, and manage chatbots. It offers a visual interface for designing conversation flows and supports multiple messaging channels. Compared to botbuilder, Botpress provides a more user-friendly interface and is highly customizable.
Rasa is an open-source machine learning framework for building contextual AI assistants and chatbots. It provides tools for natural language understanding (NLU) and dialogue management. Rasa is more focused on machine learning and offers advanced capabilities for training custom models, making it a good choice for complex conversational AI applications compared to botbuilder.
Dialogflow, developed by Google, is a natural language understanding platform used to design and integrate conversational user interfaces into mobile apps, web applications, devices, and bots. It offers pre-built agents and supports multiple languages. Dialogflow is easier to get started with and integrates well with Google Cloud services, but it may not offer the same level of customization as botbuilder.
Bot Builder for Node.js is a powerful framework for constructing bots that can handle both freeform interactions and more guided ones where the possibilities are explicitly shown to the user. It is easy to use and models frameworks like Express & Restify to provide developers with a familiar way to write Bots.
To add the latest version of this package to your bot:
npm install --save botbuilder
To get access to the daily builds of this library, configure npm to use the MyGet feed before installing.
npm config set registry https://botbuilder.myget.org/F/botbuilder-v4-js-daily/npm/
To reset the registry in order to get the latest published version, run:
npm config set registry https://registry.npmjs.org/
Bot Builder has everything you need to run a bot on almost any bot platform like the Microsoft Bot Framework, Skype, and Slack. The core library will get your bot online and chatting. Then, extend and connect your Bot Builder app with these plugins:
Plugin | Description |
---|---|
botbuilder-dialogs | Powerful dialog system with dialogs that are isolated and composable, and built-in prompts for simple things like Yes/No, strings, numbers, enumerations. |
botbuilder-ai | Utilize powerful AI frameworks like LUIS and QnA Maker. |
botbuilder-azure | Incorporate Azure services like Cosmos DB and Blob Storage into your bot. |
Read the quickstart guide that will walk you through setting up your Bot Builder app so that you've got a well structured project and all of the tools necessary to develop and extend your bot.
Create a folder for your bot, cd into it, and run npm init.
npm init
Get the BotBuilder and Restify modules using npm.
npm install --save botbuilder
npm install --save restify
Create a file named bot.js and get your bot online with a few lines of code.
const restify = require('restify');
const botbuilder = require('botbuilder');
// Create bot adapter, which defines how the bot sends and receives messages.
var adapter = new botbuilder.BotFrameworkAdapter({
appId: process.env.MicrosoftAppId,
appPassword: process.env.MicrosoftAppPassword
});
// Create HTTP server.
let server = restify.createServer();
server.listen(process.env.port || process.env.PORT || 3978, function () {
console.log(`\n${server.name} listening to ${server.url}`);
console.log(`\nGet Bot Framework Emulator: https://aka.ms/botframework-emulator`);
});
// Listen for incoming requests at /api/messages.
server.post('/api/messages', (req, res) => {
// Use the adapter to process the incoming web request into a TurnContext object.
adapter.processActivity(req, res, async (turnContext) => {
// Do something with this incoming activity!
if (turnContext.activity.type === 'message') {
// Get the user's text
const utterance = turnContext.activity.text;
// send a reply
await turnContext.sendActivity(`I heard you say ${ utterance }`);
}
});
});
Use the Bot Framework Emulator to test your bot on localhost.
Install the emulator from here and then start your bot in a console window.
Start the emulator and say "hello" to your bot.
Deploy your bot to the cloud and then register it with the Azure Bot Service.
Learn how to build great bots.
FAQs
Bot Builder is a framework for building rich bots on virtually any platform.
We found that botbuilder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.