
Bot Framework Web Chat

This repository contains code for the Bot Framework Web Chat component. The Bot Framework Web Chat component is a highly-customizable web-based client for the Bot Framework v4 SDK. The Bot Framework SDK v4 enables developers to model conversation and build sophisticated bot applications.
This repository is part of the Microsoft Bot Framework - a comprehensive framework for building enterprise-grade conversational AI experiences.
Web Chat supports Content Security Policy (CSP). Web developers are recommended to enable CSP to improve security and protect conversations. You can read more about CSP in this article.
Version notes
This section points out important version notes. For further information, please see the related links and check the CHANGELOG.md
Notes: web developers are advised to use ~
(tilde range) to select minor versions, which contains new features and/or fixes. Use ^
(caret range) to select major versions, which may contains breaking changes.
4.18.0 notable changes
In this release, we are focusing on performance improvements, including memory and load time optimizations.
4.17.0 notable changes
Support livestreaming response
Bots can now livestream their responses. Before Bot Framework SDK support this feature, bot authors can follow the details in this pull request to construct the livestream responses.
Debut of ES Modules
Web Chat now exports as ES Modules (named exports) along with CommonJS (named and unnamed exports).
Improvement to file upload experience
End-user can now add a message and confirm before uploading their file to the bot. To opt-out of the new experience, pass sendAttachmentOn: 'send'
in style options.
Theme pack support
We are excited to add theme pack support. Developers can now pack all their customization in a single package and publish it to NPM.
Experimental Fluent UI theme pack
We are excited to announce Fluent UI theme pack is in the work and is currently in experimental phase. This theme pack is designed for web developers who want to bring a native Copilot user experience to their customers.
We will continue to add new features and support both white-label experience and Fluent UI experience with the same level of parity.
You can wrap Web Chat with <FluentThemeProvider>
to try out the new experience.
import ReactWebChat from 'botframework-webchat';
import { FluentThemeProvider } from 'botframework-webchat-fluent-theme';
export default function MyComponent() {
return (
<FluentThemeProvider>
<ReactWebChat />
</FluentThemeProvider>
);
}
Support HTML-in-Markdown
Web Chat will now render HTML-in-Markdown. We have ported our sanitizer and accessibility fixer to work on HTML level. Both Markdown and HTML-in-Markdown will receive the same treatment and meet our security and accessibility requirements.
You can turn off this option by setting styleOptions.markdownRenderHTML
to false
.
4.16.1 notable changes
Web Chat now supports Adaptive Cards schema up to 1.6. Some features in Adaptive Cards are in preview or designed to use outside of Bot Framework. Web Chat does not support these features.
4.16.0 notable changes
Starting from 4.16.0, Internet Explorer is no longer supported. After more than a year of the Internet Explorer 11 officially retirement, we decided to stop supporting Internet Explorer. This will help us to bring new features to Web Chat. 4.15.9 is the last version which supports Internet Explorer in limited fashion.
4.12.1 patch: New style property adaptiveCardsParserMaxVersion
Web Chat 4.12.1 patch includes a new style property allowing developers to choose the max Adaptive Cards schema version. See PR #3778 for code changes.
To specify a different max version, you can adjust the style options, shown below:
window.WebChat.renderWebChat(
{
directLine,
store,
styleOptions: {
adaptiveCardsParserMaxVersion: '1.2'
}
},
document.getElementById('webchat')
);
- Web Chat will apply the maximum schema available according to the Adaptive Cards version (as of this patch, schema 1.3) by default.
- An invalid version will revert to Web Chat's default.
Visual focus changes to transcript in Web Chat 4.12.0
A new accessibility update has been added to Web Chat from PR #3703. This change creates visual focus for the transcript (bold black border) and aria-activedescendent
focused activity (black dashed border) by default. Where applicable, transcriptVisualKeyboardIndicator...
values will also be applied to carousel (CarouselFilmStrip.js
) children. This is done in order to match current default focus styling for Adaptive Cards, which may be a child of a carousel.
To modify these styles, you can change the following props via styleOptions
:
transcriptActivityVisualKeyboardIndicatorColor: DEFAULT_SUBTLE,
transcriptActivityVisualKeyboardIndicatorStyle: 'dashed',
transcriptActivityVisualKeyboardIndicatorWidth: 1,
transcriptVisualKeyboardIndicatorColor: 'Black',
transcriptVisualKeyboardIndicatorStyle: 'solid',
transcriptVisualKeyboardIndicatorWidth: 2,
The above code shows the default values you will see in Web Chat.
API refactor into new package in Web Chat 4.11.0
The Web Chat API has been refactored into a separate package. To learn more, check out the API refactor summary.
Direct Line Speech support in Web Chat 4.7.0
Starting from Web Chat 4.7.0, Direct Line Speech is supported, and it is the preferred way to provide an integrated speech functionality in Web Chat. We are working on closing feature gaps between Direct Line Speech and Web Speech API (includes Cognitive Services and browser-provided speech functionality).
Upgrading to 4.6.0
Starting from Web Chat 4.6.0, Web Chat requires React 16.8.6 or up.
Although we recommend that you upgrade your host app at your earliest convenience, we understand that host app may need some time before its React dependencies are updated, especially in regards to huge applications.
If your app is not ready for React 16.8.6 yet, you can follow the hybrid React sample to dual-host React in your app.
Speech changes in Web Chat 4.5.0
There is a breaking change on behavior expectations regarding speech and input hint in Web Chat. Please refer to the section on input hint behavior before 4.5.0 for details.
Migrating from Web Chat v3 to v4
View migration docs to learn about migrating from Web Chat v3.
How to use
First, create a bot using Azure Bot Service.
Once the bot is created, you will need to obtain the bot's Web Chat secret in Azure Portal. Then use the secret to generate a token and pass it to your Web Chat.
Connect a client app to bot
Web Chat provides UI on top of the Direct Line and Direct Line Speech Channels. There are two ways to connect to your bot through HTTP calls from the client: by sending the Bot secret or generating a token via the secret.
We strongly recommend using the token API instead of providing the app with your secret. To learn more about why, see the authentication documentation on the token API and client security.
For further reading, please see the following links:
Integrate with JavaScript
Web Chat is designed to integrate with your existing website using JavaScript or React. Integrating with JavaScript will give you moderate styling and customizability options.
You can use the full, typical Web Chat package (called full-feature bundle) that contains the most typically used features.
Here is how how you can add Web Chat control to your website:
<!DOCTYPE html>
<html>
<head>
<script
crossorigin="anonymous"
src="https://cdn.botframework.com/botframework-webchat/latest/webchat.js"
></script>
<style>
html,
body {
height: 100%;
}
body {
margin: 0;
}
#webchat {
height: 100%;
width: 100%;
}
</style>
</head>
<body>
<div id="webchat" role="main"></div>
<script>
window.WebChat.renderWebChat(
{
directLine: window.WebChat.createDirectLine({
token: 'YOUR_DIRECT_LINE_TOKEN'
}),
userID: 'YOUR_USER_ID',
username: 'Web Chat User',
locale: 'en-US'
},
document.getElementById('webchat')
);
</script>
</body>
</html>
userID
, username
, and locale
are all optional parameters to pass into the renderWebChat
method. To learn more about Web Chat props, look at the Web Chat API Reference documentation.
Assigning userID
as a static value is not recommended since this will cause all users to share state. Please see the API userID entry
for more information.
More information on localization can be found in the Localization documentation.
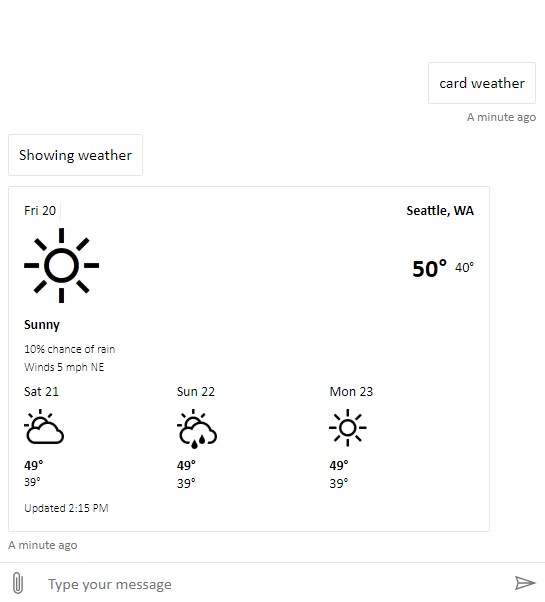
See the working sample of the full Web Chat bundle.
Integrate with React
For full customizability, you can use React to recompose components of Web Chat.
To install the production build from NPM, run npm install botframework-webchat
. See our version notes on how to select a version.
import React, { useMemo } from 'react';
import ReactWebChat, { createDirectLine } from 'botframework-webchat';
export default () => {
const directLine = useMemo(() => createDirectLine({ token: 'YOUR_DIRECT_LINE_TOKEN' }), []);
return <ReactWebChat directLine={directLine} userID="YOUR_USER_ID" />;
};
You can also run npm install botframework-webchat@main
to install a development build that is synced with Web Chat's GitHub main
branch.
See the working sample of Web Chat rendered via React.
Experimental support for Redux DevTools
Web Chat internally use Redux for state management. Redux DevTools is enabled in the NPM build as an opt-in feature.
This is for glancing into how Web Chat works. This is not an API explorer and is not an endorsement of using the Redux store to programmatically access the UI. The hooks API should be used instead.
To use Redux DevTools, use the createStoreWithDevTools
function for creating a Redux DevTools-enabled store.
import React, { useMemo } from 'react';
- import ReactWebChat, { createDirectLine, createStore } from 'botframework-webchat';
+ import ReactWebChat, { createDirectLine, createStoreWithDevTools } from 'botframework-webchat';
export default () => {
const directLine = useMemo(() => createDirectLine({ token: 'YOUR_DIRECT_LINE_TOKEN' }), []);
- const store = useMemo(() => createStore(), []);
+ const store = useMemo(() => createStoreWithDevTools(), []);
return <ReactWebChat directLine={directLine} store={store} userID="YOUR_USER_ID" />;
};
There are some limitations when using the Redux DevTools:
- The Redux store uses side-effects via
redux-saga
. Time-traveling may break the UI.
- Many UI states are stored in React context and state. They are not exposed in the Redux store.
- Some time-sensitive UIs are based on real-time clock and not affected by time-traveling.
- Dispatching actions are not officially supported. Please use hooks API instead.
- Actions and reducers may move in and out of Redux store across versions. Hooks API is the official API for accessing the UI.
Customizing the Web Chat UI
Web Chat is designed to be customizable without forking the source code. The table below outlines what kind of customizations you can achieve when you are importing Web Chat in different ways. This list is not exhaustive.
Change colors | ✔ | ✔ |
Change sizes | ✔ | ✔ |
Update/replace CSS styles | ✔ | ✔ |
Listen to events | ✔ | ✔ |
Interact with hosting webpage | ✔ | ✔ |
Custom render activities | | ✔ |
Custom render attachments | | ✔ |
Add new UI components | | ✔ |
Recompose the whole UI | | ✔ |
See more about customizing Web Chat to learn more on customization.
Supported Activity Types on the Web Chat Client
Bot Framework has many activity types, but not all are supported in Web Chat. View activity types docs to learn more.
Samples list
View the complete list of Web Chat samples for more ideas on customizing Web Chat.
Further reading
API Reference
View the API documentation for implementing Web Chat.
Browser compatibility
Web Chat supports the latest 2 versions of modern browsers like Chrome, Microsoft Edge, and FireFox.
If you need Web Chat in Internet Explorer 11, please see the ES5 bundle demo.
Please note, however:
- Web Chat does not support Internet Explorer older than version 11
- Customization as shown in non-ES5 samples are not supported for Internet Explorer. Because IE11 is a non-modern browser, it does not support ES6, and many samples that use arrow functions and modern promises would need to be manually converted to ES5. If you are in need of heavy customization for your app, we strongly recommend developing your app for a modern browser like Google Chrome or Microsoft Edge.
- Web Chat has no plan to support samples for IE11 (ES5).
- For customers who wish to manually rewrite our other samples to work in IE11, we recommend looking into converting code from ES6+ to ES5 using polyfills and transpilers like
babel
.
Accessibility
View the accessibility documentation.
Localization
View the localization documentation for implementing in Web Chat.
Notifications
View the notification documentation for implementing in Web Chat.
Telemetry
View the telemetry documentation for implementing in Web Chat.
Technical Support Guide
View the Technical Support Guide to get guidance and help on troubleshooting in the Web Chat repo for more information before filing a new issue.
Speech
Web Chat supports a wide-range of speech engines for a natural chat experience with a bot. This section outlines the different engines that are supported:
Integrate with Direct Line Speech
Direct Line Speech is the preferred way to add speech functionality in Web Chat. Please refer to the Direct Line Speech documentation for details.
Integrate with Cognitive Services Speech Services
You can use Cognitive Services Speech Services to add speech functionality to Web Chat. Please refer to the Cognitive Services Speech Services documentation for details.
Browser-provided engine or other engines
You can also use any speech engines which support W3C Web Speech API standard. Some browsers support the Speech Recognition API and the Speech Synthesis API. You can mix-and-match different engines - including Cognitive Services Speech Services - to provide best user experience.
How to test with Web Chat's latest bits
Web Chat latest bits are available on the Web Chat daily releases page.
Dailies will be released after 3:00AM Pacific Standard Time when changes have been committed to the main branch.
Contributing
See our Contributing page for details on how to build the project and our repository guidelines for Pull Requests.
See our CODE OF CONDUCT page for details about the Microsoft Code of Conduct.
Reporting Security Issues
View the security documentation to learn more about reporting security issues.