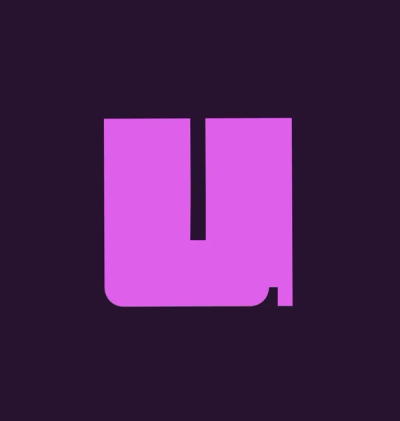
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
bottender
Advanced tools
1.0.0 / 2019-12-05
create-bottender-app
. You can use following command to create your new bot:npx create-bottender-app my-app
bottender start
cli. It finds index.js
entry and bottender.config.js
config file then executes accordingly:bottender start
To enable console mode:
bottender start --console
bottender dev
cli:bottender dev
bottender dev --console
The bot server will be restarted after changing the files.
Action:
async function SayHi(context) {
await context.sendText('hi');
}
Pass Props to Action:
const { withProps } = require('bottender');
async function SayHi(context, { name }) {
await context.sendText(`hi! ${name}.`);
}
async function App() {
return withProps(SayHi, { name: 'John' });
}
Router:
const { router, text } = require('bottender/router');
async function SayHi(context) {
await context.sendText('Hi!');
}
async function SayHello(context) {
await context.sendText('Hello!');
}
async function App() {
return router([
text('hi', SayHi), // return SayHi when receiving hi text message
text('hello', SayHello), // return SayHello when receiving hello text message
]);
}
Chain:
const { chain } = require('bottender');
function RuleBased(context, props) {
if (context.event.text === 'hi') {
// discontinue and return SayHi
return SayHi;
}
// continue to next
return props.next;
}
function MachineLearningBased(context, props) {
/* ...skip */
}
function HumanAgent(context, props) {
/* ...skip */
}
function App() {
return chain([
// will execute in following order
RuleBased,
MachineLearningBased,
HumanAgent,
]);
}
_error.js
entry support for error handling:// _error.js
module.exports = async function HandleError(context, props) {
await context.sendText(
'There are some unexpected errors happened. Please try again later, sorry for the inconvenience.'
);
console.error(props.error);
if (process.env.NODE_ENV === 'production') {
// send your error to the error tracker, for example: Sentry
}
if (process.env.NODE_ENV === 'development') {
await context.sendText(props.error.stack);
}
};
middleware
and Handlers has been moved to @bottender/handlers
package. You can install it from registry:npm install @bottender/handlers
// or using yarn:
yarn add @bottender/handlers
And import them like this:
const {
middleware,
Handler,
MessengerHandler,
LineHandler,
SlackHandler,
TelegramHandler,
ViberHandler,
} = require('@bottender/handlers');
Messenger -
context.sendGenericTemplate([
{
title: "Welcome to Peter's Hats",
imageUrl: 'https://petersfancybrownhats.com/company_image.png',
subtitle: "We've got the right hat for everyone.",
defaultAction: {
type: 'web_url',
url: 'https://peterssendreceiveapp.ngrok.io/view?item=103',
messengerExtensions: true,
webviewHeightRatio: 'tall',
fallbackUrl: 'https://peterssendreceiveapp.ngrok.io/',
},
buttons: [
{
type: 'postback',
title: 'Start Chatting',
payload: 'DEVELOPER_DEFINED_PAYLOAD',
},
],
},
]);
Slack -
context.postMessage({
blocks: [
{
type: 'section',
text: {
type: 'plain_text',
text: 'You updated the modal!',
},
},
{
type: 'image',
imageUrl: 'https://media.giphy.com/media/SVZGEcYt7brkFUyU90/giphy.gif',
altText: 'Yay! The modal was updated',
},
],
});
Telegram -
context.sendMessage('hi', {
disableWebPagePreview: true,
disableNotification: true,
});
Viber -
context.sendFile({
media: 'http://www.images.com/file.doc',
size: 10000,
fileName: 'name_of_file.doc',
});
context.event.rawEvent; // all keys is camelcase in this object
[breaking] rename skipProfile
to skipLegacyProfile
, and set to true by default
[breaking] unify requestContext (#541)
[deps] update messaging-apis
to v1
[examples] Rewrite all examples for Bottender v1
[docs] A brand-new website with new docs - https://bottender.js.org?new
pageId
config to automatically add subscribe app in bottender messenger webhook set
.get-started
, greeting
, persistent-menu
, whitelisted-domains
cli subcommands has been removed. Use profile
instead:bottender messenger profile get
bottender messenger profile set
bottender messenger profile delete
context.sendAirlineFlightUpdateTemplate()
.context.getMessageContent()
. You can use it to get received media content:async function App(context) {
if (context.event.isImage || context.event.isVideo || context.event.isAudio) {
const buffer = await context.getMessageContent();
}
}
sendMethod
to reply
and shouldBatch
to true
by default.menu
cli subcommand has been removed.context.postMessage({
blocks: [
{
type: 'section',
text: {
type: 'plain_text',
text: 'You updated the modal!',
},
},
{
type: 'image',
imageUrl: 'https://media.giphy.com/media/SVZGEcYt7brkFUyU90/giphy.gif',
altText: 'Yay! The modal was updated',
},
],
});
token
in payload when received a JSON string payload.context.sendAnimation()
:context.sendAnimation('xxx.mp4');
context.sendPoll()
const options = ['a', 'b'];
context.sendPoll(question, options);
context.editMessageText('<MESSAGE_ID>', text);
context.editMessageCaption('<MESSAGE_ID>', caption);
context.editMessageReplyMarkup('<MESSAGE_ID>', replyMarkup);
context.editMessageLiveLocation('<MESSAGE_ID>', location);
context.stopMessageLiveLocation('<MESSAGE_ID>');
FAQs
A framework for building conversational user interfaces.
The npm package bottender receives a total of 317 weekly downloads. As such, bottender popularity was classified as not popular.
We found that bottender demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.