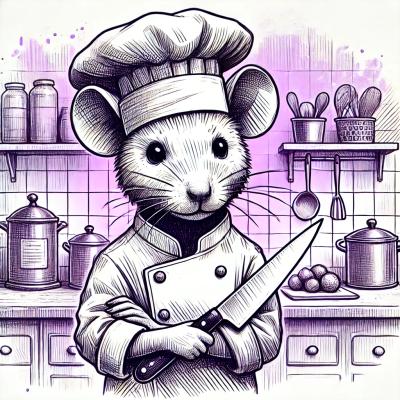
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
brave-cache
Advanced tools
Brave Cache is a simple caching library manager for Nodejs.
NodeJs Ecosystem already has lots of caching libraries and this is not one of them, but they are not merge-able and consists of different apis. Brave Cache supports adding multiple cache providers/drivers while keeping same api.
npm install brave-cache
# OR
yarn add brave-cache
const BraveCache = require("brave-cache");
// Create Cache, it will use the default provider
const cache = new BraveCache();
cache.set("pet", "cat");
console.log(cache.get("pet")); // "cat"
// You can also access the provider
cache.provider.client.set("direct", "direct");
console.log(cache.get("direct")); // => direct
console.log(cache.provider.client.get("direct")); // => direct
Out of the box, BraveCache provides supports for the following cache libraries:
lru-cache
)node-cache
)The default Provider is ObjectCacheProvider
which uses object-collection
to store the cache data in an object.
It has been registered by default.
The registerProvider
method can be used to register a custom provider.
it takes two arguments:
Argument | Type | Description |
---|---|---|
provider | object | The provider object i.e. instance of BraveCacheProvider |
as | string | Custom name of the provider (optional) |
const BraveCache = require("brave-cache");
const LRUCacheProvider = require("brave-cache/providers/lru-cache");
// default name is `lru-cache`
BraveCache.registerProvider(LRUCacheProvider());
// custom name as `my-cache`
BraveCache.registerProvider(LRUCacheProvider(), "my-cache");
// custom name as `my-cache-2` and max size of 50
BraveCache.registerProvider(LRUCacheProvider({ max: 50 }), "my-cache-2");
const cache = new BraveCache("lru-cache");
const cache2 = new BraveCache("my-cache");
const cache3 = new BraveCache("my-cache-2");
Providers are registered and accessed by their names.
Brave Cache adds prefix support to the cache keys without needing the provider to do it.
on get
and set
methods the prefix will be added.
The example below is how brave cache works without prefix.
// will use default provider (ObjectCacheProvider)
const cache1 = new BraveCache();
const cache2 = new BraveCache();
// cache1 and cache2 will have same data
cache1.set("test", "from cache1");
// this will overwrite the value for `test` in cache1
cache2.set("test", "from cache2");
cache1.get("test"); // => from cache2
cache2.get("test"); // => from cache2
cache1
and cache2
will have same data because they are both using the same provider and without prefix you are referring to the same key in one provider.
The example below is how brave cache works with prefix.
// will use default provider (ObjectCacheProvider)
const cache1 = new BraveCache({ prefix: "cache1" });
const cache2 = new BraveCache({ prefix: "cache2" });
const cache3 = new BraveCache(); // no prefix
cache1.set("test", "from cache1");
cache2.set("test", "from cache2");
cache2.set("test2", "another value from cache2");
cache1.get("test"); // => from cache1
cache1.get("test2"); // => undefined
cache2.get("test"); // => from cache
cache2.get("test2"); // => another value from cache2
cache1.size(); // => 1
cache2.size(); // => 2
cache3.size(); // => 3
cache1
and cache2
will not have same date because they are using different prefix. Meanwhile cache3
will have cache1 + cache2
data because they all reference the same provider instance.
These are the functions available on the cache instance. Unlike other cache packages, all instance methods come in both sync
and async
versions.
Note: Async
methods will only work if your provider supports async operations. most cache libraries do not support async operations.
Example of a cache instance.
const cache = new BraveCache();
// or specify a provider
const cache = new BraveCache("object-cache");
Get a value from the cache.
cache.set("key", "value");
cache.get("key"); // => "value"
cache.get("non existent key"); // => undefined
// cache with default value
cache.get("non existent key", "default value"); // => "default value"
// Or with default value computed from a function
cache.get("non existent key", () => "default value"); // => "default value"
Async version of get()
, Includes all the functionalities of get()
with extra capability to resolve promises or functions that return promises.
// Includes all the functionality of get()
// Or with default value as a promise
await cache.getAsync("non existent key", Promise.resolve("default value")); // => "default value"
// Or with default value computed from a function
await cache.getAsync("non existent key", () => "default value"); // => "default value"
// Or with default value computed from a function that returns a promise
await cache.getAsync("non existent key", () => Promise.resolve("default value")); // => "default value"
Get multiple values at once. keys that do not exist will be ignored.
// Set Many
cache.setMany([
["key1", "value1"],
["key2", "value2"],
["key3", "value3"]
]);
let test = cache.getMany(["key1", "key2", "key3", "key4"]);
console.log(test.key1); // => "value1";
console.log(test.key2); // => "value2";
console.log(test.key3); // => "value3";
console.log(test.key4); // => undefined;
Async version of getMany()
Set a value in the cache.
cache.set("key", "value"); // set key to value
Async version of set()
Set multiple values at once.
// using array of key value pairs
cache.setMany([
["key1", "value1"],
["key2", "value2", 500] // with TTL
]);
// using object
cache.setMany([
{ key: "key3", value: "value3" },
{ key: "key4", value: "value4", ttl: 500 } // or with TTL in seconds
]);
console.log(cache.keys()); // => ["key1", "key2", "key3", "key4"]
Async version of setMany()
Find a value in the cache. If it does not exist, set it to the default value.
cache.getOrSet("username", "joe"); // => "joe"
// cache should have username set to joe
cache.has("username"); // => true
// Trying again will return the value set in the cache
cache.getOrSet("username", "john"); // => "joe" because joe is still in the cache
// Functions can be used as default values
cache.getOrSet("email", () => "joe@example.com"); // => "joe@example.com"
Async version of getOrSet()
Check if key exists in the cache.
cache.set("pet", "cat");
cache.has("pet"); // => true
cache.has("pet2"); // => false
Async version of has()
Delete a key from the cache.
cache.set("pet", "dog");
cache.del("pet");
cache.has("pet"); // => false
Async version of del()
Alias for del()
Alias for delAsync()
Get all the keys in the cache.
cache.setMany([
["pet", "dog"],
["username", "joe"],
["email", "joe@example.com"]
]);
cache.keys(); // => ["pet", "username", "email"]
Async version of keys()
const cache = new BraveCache({ prefix: "a" });
cache.setMany([
["pet", "dog"],
["username", "joe"],
["email", "joe@example.com"]
]);
cache.keys(); // => ["pet", "username", "email"]
cache.keysWithPrefix(); // => ["a:pet", "a:username", "a:email"]
Async version of keysWithPrefix()
Empty the cache completely.
Async version of flush()
Get the number of items in the cache.
Async version of size()
Basically all nodejs cache
modules have similar api, so creating a custom provider is easy.
A custom provider is a function
that takes any necessary configuration and returns a BraveCacheProvider
class instance.
const BraveCacheProvider = require("brave-cache/src/BraveCacheProvider");
function SimpleCache() {
// Holds cache values
let SimpleCacheStore = {};
// Return provider instance
return new BraveCacheProvider({
name,
functions: {
get(key) {
return SimpleCacheStore[key];
},
set(key, value) {
SimpleCacheStore[key] = value;
},
has(key) {
return SimpleCacheStore.hasOwnProperty(key);
},
del(key) {
delete SimpleCacheStore[key];
},
flush() {
SimpleCacheStore = {};
},
keys() {
return Object.keys(SimpleCacheStore);
}
}
});
}
From the example above, the SimpleCache
provider is a simple implementation of how to create a cache Provider.
Notice that you have to implement all the functions that are required by the BraveCacheProvider
class.
These functions will be used when accessing the cache.
Functions to be implemented: get
, set
, has
, del
, flush
, keys
Optional functions: getMany
Note: if any optional function is not implemented, BraveCache in house function will be used instead.
A custom Provider function can include arguments that are related to the provider.
const BraveCache = require("brave-cache");
// Register Simple Cache Above
BraveCache.registerProvider(SimpleCache());
const cache = new BraveCache("simple-cache"); // `simple-cache` is the name of the provider
cache.set("test", "value");
console.log(cache.get("test")); // => value
FAQs
A flexible semantic Api for handling multiple node cache drivers.
The npm package brave-cache receives a total of 2 weekly downloads. As such, brave-cache popularity was classified as not popular.
We found that brave-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.