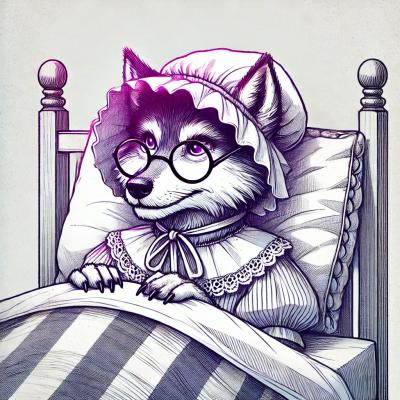
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Lightweight App extensibility and hookable middleware customization.
broadway
is designed to be the littlest possible extensibility for server applications. It is does not take any other external dependencies besides those to expose basic "start middleware".
Additional functionality may be added through .mixin(base, redefine)
:
var express = require('express'),
App = require('broadway');
//
// Create a new base App.
//
var app = new App({ http: 8080 });
//
// Then mixin `express` functionality later on. This
// can be called multiple times. By default: it will
// only define a single property on your app once.
//
app.mixin(express());
//
// Do anything you want asynchronously before
// the application starts.
//
app.preboot(function (app, options, next) {
console.log('Starting up...');
next();
});
//
// Start listening on HTTP port passed in to
// App when it was created above.
//
app.start(function (err) {
if (err) {
console.error('Error on startup: %s', err.message);
return process.exit(1);
}
console.log('Listening over HTTP on port %s', this.given.http);
});
Because broadway
exposes a generic hook mechanism from understudy it is possible to write hooks into your middleware easily. Consider the following example that defines hookable "auth" handlers into its existing authorization middleware:
var express = require('express'),
basicAuth = require('basic-auth'),
App = require('broadway');
//
// Create an app with default http options,
// mixin all express functions
//
var app = new App({ http: 8080 }, express());
//
// Define a simple "auth" middleware that only
// performs Basic Auth.
//
app.use(function auth(req, res, next) {
//
// Before checking and/or parsing the Basic Auth
// header allow others to attempt their auth methods.
//
app.perform('auth', req, res, function (done) {
var creds = basicAuth(req);
if (req.authed) {
return done();
}
else if (!creds || creds.name !== 'bob' || creds.pass !== 'secret') {
res.writeHead(401, { 'WWW-Authenticate': 'Basic realm="example"' });
res.end('Unauthorized\n');
return;
}
done();
}, next);
});
//
// Hook into the new "auth middleware" defined above
// to add support for the `X-AUTH-TOKEN` header.
//
app.before('auth', function (req, res, next) {
var bearerToken = req.headers['x-auth-token'];
if (bearerToken === 'golden-ticket') {
req.authed = true;
}
next();
});
// ... continue starting the app as usual.
All tests are written with mocha and should be run with npm
:
$ npm test
FAQs
Lightweight App extensibility and hookable middleware customization.
We found that broadway demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.