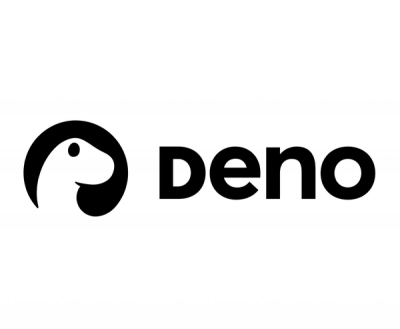
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
broccoli-plugin
Advanced tools
The broccoli-plugin npm package is a base class for Broccoli plugins. Broccoli is a JavaScript build tool, and this package allows developers to create custom plugins for their build pipelines. It provides a structured way to define input and output nodes, manage file transformations, and handle build errors.
Creating a Basic Plugin
This code demonstrates how to create a basic Broccoli plugin that reads a file, transforms its content to uppercase, and writes the result to the output directory.
const Plugin = require('broccoli-plugin');
const fs = require('fs');
class MyPlugin extends Plugin {
constructor(inputNodes, options) {
super(inputNodes, options);
}
build() {
const inputPath = this.inputPaths[0];
const outputPath = this.outputPath;
const inputFile = `${inputPath}/input.txt`;
const outputFile = `${outputPath}/output.txt`;
const content = fs.readFileSync(inputFile, 'utf8');
const transformedContent = content.toUpperCase();
fs.writeFileSync(outputFile, transformedContent);
}
}
module.exports = MyPlugin;
Handling Multiple Input Nodes
This example shows how to handle multiple input nodes in a Broccoli plugin. It reads files from two different input directories, combines their contents, and writes the result to the output directory.
const Plugin = require('broccoli-plugin');
const fs = require('fs');
class MultiInputPlugin extends Plugin {
constructor(inputNodes, options) {
super(inputNodes, options);
}
build() {
const inputPath1 = this.inputPaths[0];
const inputPath2 = this.inputPaths[1];
const outputPath = this.outputPath;
const content1 = fs.readFileSync(`${inputPath1}/file1.txt`, 'utf8');
const content2 = fs.readFileSync(`${inputPath2}/file2.txt`, 'utf8');
const combinedContent = content1 + '\n' + content2;
fs.writeFileSync(`${outputPath}/combined.txt`, combinedContent);
}
}
module.exports = MultiInputPlugin;
Error Handling in Plugins
This code demonstrates how to handle errors in a Broccoli plugin. It checks if the input file exists and throws an error if it does not. Any errors encountered during the build process are caught and logged.
const Plugin = require('broccoli-plugin');
const fs = require('fs');
class ErrorHandlingPlugin extends Plugin {
constructor(inputNodes, options) {
super(inputNodes, options);
}
build() {
try {
const inputPath = this.inputPaths[0];
const outputPath = this.outputPath;
const inputFile = `${inputPath}/input.txt`;
const outputFile = `${outputPath}/output.txt`;
if (!fs.existsSync(inputFile)) {
throw new Error('Input file does not exist');
}
const content = fs.readFileSync(inputFile, 'utf8');
const transformedContent = content.toUpperCase();
fs.writeFileSync(outputFile, transformedContent);
} catch (error) {
console.error('Build error:', error);
}
}
}
module.exports = ErrorHandlingPlugin;
Gulp is a toolkit for automating painful or time-consuming tasks in your development workflow. It uses a code-over-configuration approach and streams to handle file transformations. Compared to Broccoli, Gulp is more flexible and has a larger ecosystem of plugins, but it can be more complex to set up and use.
Webpack is a module bundler that takes modules with dependencies and generates static assets representing those modules. It is highly configurable and supports a wide range of plugins and loaders. Webpack is more focused on bundling JavaScript applications, whereas Broccoli is more general-purpose for file transformations.
Rollup is a module bundler for JavaScript that compiles small pieces of code into something larger and more complex, such as a library or application. It is known for its tree-shaking capabilities, which help to remove unused code. Rollup is more specialized for JavaScript bundling, while Broccoli is a more general build tool.
var Plugin = require('broccoli-plugin');
var path = require('path');
// Create a subclass MyPlugin derived from Plugin
MyPlugin.prototype = Object.create(Plugin.prototype);
MyPlugin.prototype.constructor = MyPlugin;
function MyPlugin(inputNodes, options) {
options = options || {};
Plugin.call(this, inputNodes, {
annotation: options.annotation
});
this.options = options;
}
MyPlugin.prototype.build = function() {
// Read files from this.inputPaths, and write files to this.outputPath.
// Silly example:
// Read 'foo.txt' from the third input node
var inputBuffer = fs.readFileSync(path.join(this.inputPaths[2], 'foo.txt'));
var outputBuffer = someCompiler(inputBuffer);
// Write to 'bar.txt' in this node's output
fs.writeFileSync(path.join(this.outputPath, 'bar.txt'), outputBuffer);
};
new Plugin(inputNodes, options)
Call this base class constructor from your subclass constructor.
inputNodes
: An array of node objects that this plugin will read from.
Nodes are usually other plugin instances; they were formerly known as
"trees".
options
name
: The name of this plugin class. Defaults to this.constructor.name
.annotation
: A descriptive annotation. Useful for debugging, to tell
multiple instances of the same plugin apart.persistentOutput
: If true, the output directory is not automatically
emptied between builds.Plugin.prototype.build()
Override this method in your subclass. It will be called on each (re-)build.
This function will typically access the following read-only properties:
this.inputPaths
: An array of paths on disk corresponding to each node in
inputNodes
. Your plugin will read files from these paths.
this.outputPath
: The path on disk corresponding to this plugin instance
(this node). Your plugin will write files to this path. This directory is
emptied by Broccoli before each build, unless the persistentOutput
options
is true.
this.cachePath
: The path on disk to an auxiliary cache directory. Use this
to store files that you want preserved between builds. This directory will
only be deleted when Broccoli exits.
All paths stay the same between builds.
To perform asynchronous work, return a promise. The promise's eventual value
is ignored (typically null
).
To report a compile error, throw
it or return a rejected promise. Also see
section "Error Objects" below.
Plugin.prototype.getCallbackObject()
Advanced usage only.
Return the object on which Broccoli will call obj.build()
. Called once after
instantiation. By default, returns this
. Plugins do not usually need to
override this, but it can be useful for base classes that other plugins in turn
derive from, such as
broccoli-caching-writer.
For example, to intercept .build()
calls, you might
return { build: this.buildWrapper.bind(this) }
.
Or, to hand off the plugin implementation to a completely separate object:
return new MyPluginWorker(this.inputPaths, this.outputPath, this.cachePath)
,
where MyPluginWorker
provides a .build
method.
To help with displaying clear error messages for build errors, error objects
may have the following optional properties in addition to the standard
message
property:
file
: Path of the file in which the error occurred, relative to one of the
inputPaths
directoriestreeDir
: The path that file
is relative to. Must be an element of
this.inputPaths
. (The name treeDir
is for historical reasons.)line
: Line in which the error occurred (one-indexed)column
: Column in which the error occurred (zero-indexed)1.2.1
FAQs
Base class for all Broccoli plugins
The npm package broccoli-plugin receives a total of 1,054,525 weekly downloads. As such, broccoli-plugin popularity was classified as popular.
We found that broccoli-plugin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.