What is btoa-lite?
The btoa-lite npm package is a lightweight utility for encoding data in base64 format. It is a simplified version of the native btoa function available in browsers, designed to work in Node.js environments.
What are btoa-lite's main functionalities?
Base64 Encoding
This feature allows you to encode a string into base64 format. The code sample demonstrates how to use the btoa-lite package to encode the string 'Hello, World!' into its base64 representation.
const btoa = require('btoa-lite');
const encoded = btoa('Hello, World!');
console.log(encoded); // Outputs: 'SGVsbG8sIFdvcmxkIQ=='
Other packages similar to btoa-lite
js-base64
The js-base64 package provides similar functionality for encoding and decoding base64 strings. It is a more comprehensive library that works both in Node.js and browser environments. Compared to btoa-lite, js-base64 offers additional methods like decoding base64 strings back to their original form.
base-64
The base-64 package is another alternative for base64 encoding and decoding. It is lightweight and works in both Node.js and browser environments. It provides a straightforward API for encoding and decoding base64 strings, similar to btoa-lite, but with additional support for decoding.
buffer
The buffer package is a core Node.js module that provides a way to handle binary data. It includes methods for base64 encoding and decoding. While it is more versatile and powerful than btoa-lite, it is also more complex and may be overkill for simple base64 encoding tasks.
btoa-lite

Smallest/simplest possible means of using btoa with both Node and browserify.
In the browser, encoding base64 strings is done using:
var encoded = btoa(decoded)
However in Node, it's done like so:
var encoded = new Buffer(decoded).toString('base64')
You can easily check if Buffer
exists and switch between the approaches
accordingly, but using Buffer
anywhere in your browser source will pull
in browserify's Buffer
shim which is pretty hefty. This package uses
the main
and browser
fields in its package.json
to perform this
check at build time and avoid pulling Buffer
in unnecessarily.
Usage
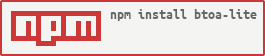
encoded = btoa(decoded)
Returns the base64-encoded value of a string.
License
MIT. See LICENSE.md for details.