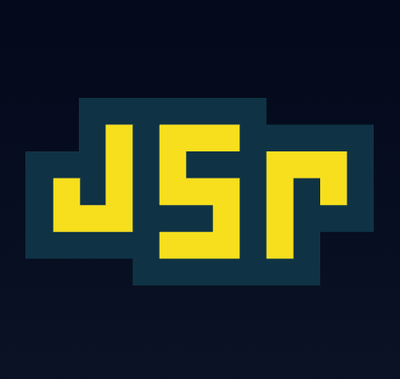
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The cac npm package is a simple yet powerful framework for building command-line applications. It allows developers to parse arguments, generate help messages, and create commands with options and sub-commands.
Command Parsing
This feature allows you to define commands with required and optional arguments. The code sample demonstrates how to create a command 'init' that requires a project name and accepts an optional type argument.
{"const cac = require('cac');\nconst cli = cac();\ncli.command('init <name>', 'Initialize a project')\n .option('--type <type>', 'Project type')\n .action((name, options) => {\n console.log(`Initializing project: ${name} with type: ${options.type}`);\n });\ncli.help();\ncli.parse();"}
Help Generation
Automatically generates help information for the defined commands and options. The code sample shows how to define a 'build' command and an option to minify the output, with automatic help generation.
{"const cac = require('cac');\nconst cli = cac();\ncli.command('build', 'Build the project')\n .option('--minify', 'Minify the output')\ncli.help();\ncli.parse();"}
Sub-commands
Supports the creation of sub-commands for more complex CLI structures. The code sample illustrates how to create a 'deploy' command with sub-commands for different deployment providers like AWS and Azure.
{"const cac = require('cac');\nconst cli = cac();\nconst deploy = cli.command('deploy <provider>', 'Deploy your project');\ndeploy.command('aws', 'Deploy to AWS')\n .action(() => {\n console.log('Deploying to AWS...');\n });\ndeploy.command('azure', 'Deploy to Azure')\n .action(() => {\n console.log('Deploying to Azure...');\n });\ncli.help();\ncli.parse();"}
Commander is one of the most popular npm packages for building command-line interfaces. It offers similar functionalities to cac, such as command parsing, option management, and automatic help generation. Commander has a more extensive feature set and a larger community, but cac is known for its simplicity and ease of use.
Yargs is another well-known package for building command-line tools. It provides a rich API for argument parsing, command chaining, and validation. Yargs is more verbose and configurable compared to cac, which might be preferred for more complex CLI applications.
Meow is a lightweight CLI helper with a minimalistic approach. It is less feature-rich than cac but is very straightforward to use for simple command-line applications. Meow is a good choice for those who need something simpler than cac and do not require complex command structures.
Command And Conquer, the queen living in your command line, is a minimalistic but pluggable CLI framework.
yarn add cac
Use ./examples/simple.js
as example:
const cac = require('cac')
const cli = cac()
// Add a default command
const defaultCommand = cli.command('*', {
desc: 'The default command'
}, (input, flags) => {
if (flags.age) {
console.log(`${input[0]} is ${flags.age} years old`)
}
})
defaultCommand.option('age', {
desc: 'tell me the age'
})
// Add a sub command
cli.command('bob', {
desc: 'Command for bob'
}, () => {
console.log('This is a command dedicated to bob!')
})
// Bootstrap the CLI app
cli.parse()
Then run it:
And the Help Documentation is ready out of the box:
Projects that use CAC:
Register an option globally, i.e. for all commands
string
option nameobject
string
string
descriptionstring
Array<string>
option name aliasstring
option type, valid values: boolean
string
any
option default valueboolean
mark option as requiredArray<any>
limit valid values for the optionstring
object
string
(string
is used as desc
)
string
descriptionstring
Array<string>
command name aliasArray<string>
command examplesfunction
command handler
Array<string>
cli argumentsobject
cli flagsconst command = cli.command('init', 'init a new project', (input, flags) => {
const folderName = input[0]
console.log(`init project in folder ${folderName}`)
})
cli.command
returns a command instance.
Same as cli.option but it adds options for specified command.
Array<string>
Defaults to process.argv.slice(2)
boolean
Defaults to true
Run command after parsed argv.Display cli helps, must be called after cli.parse()
Plugin
Array<Plugin>
Apply a plugin to cli instance:
cli.use(plugin(options))
function plugin(options) {
return cli => {
// do something...
}
}
Type: string
The filename of executed file.
e.g. It's cli.js
when you run node ./cli.js
.
A getter which simply returns cli.parse(null, { run: false })
Add extra help messages to the bottom of help.
Type: string
object
The help
could be a string
or in { title, body }
format.
Error handler for errors in your command handler:
cli.on('error', err => {
console.error('command failed:', err)
process.exit(1)
})
Emit after CAC parsed cli arguments:
cli.on('parsed', (command, input, flags) => {
// command might be undefined
})
Emit after CAC executed commands or outputed help / version number:
cli.on('executed', (command, input, flags) => {
// command might be undefined
})
commander.js
yargs
caporal.js
or meow
?CAC
is simpler and less opinionated comparing to commander.js
yargs
caporal.js
.
Commander.js does not support chaining option which is a feature I like a lot. It's not really actively maintained at the time of writing either.
Yargs has a powerful API, but it's so massive that my brain trembles. Meow is simple and elegant but I have to manully construct the help message, which will be annoying. And I want it to support sub-command too.
And none of them are pluggable.
So why creating a new thing instead of pull request?
I would ask me myself why there's preact
instead of PR to react
, and why yarn
instead of PR to npm
? It's obvious.
CAC is kind of like a combination of the simplicity of Meow and the powerful features of the rest. And our help log is inspired by Caporal.js, I guess it might be the most elegant one out there?
CAC, not Cac or cac, pronounced C-A-C
.
And this project is dedicated to our lovely C.C. sama. Maybe CAC stands for C&C as well :P
git checkout -b my-new-feature
git commit -am 'Add some feature'
git push origin my-new-feature
cac © egoist, Released under the MIT License.
Authored and maintained by egoist with help from contributors (list).
egoist.moe · GitHub @egoist · Twitter @_egoistlily
FAQs
Simple yet powerful framework for building command-line apps.
The npm package cac receives a total of 6,059,803 weekly downloads. As such, cac popularity was classified as popular.
We found that cac demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.