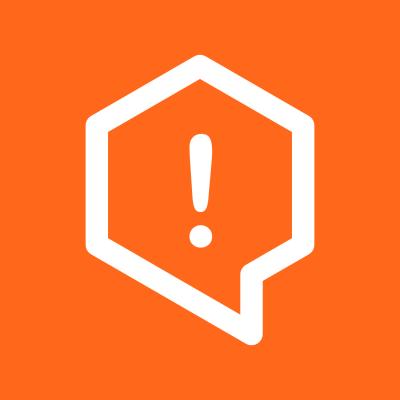
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
cache-element
Advanced tools
Cache a bel element. Makes rendering elements very fast™. Analogous to
React's .shouldComponentUpdate()
method, but only using native DOM methods.
Here we take a regular element, and cache it so re-renders are fast. We compare
the arguments on each run passing it to the compare
function:
var cache = require('cache-element')
var html = require('bel')
var element = Element()
let el = element('Tubi', 12) // creates new element
let el = element('Tubi', 12) // returns cached element (proxy)
let el = element('Babs', 12) // creates new element
function Element () {
return cache(function (name, age) {
return html`
<section>
<p>The person's name is ${name}</p>
<p>The person's age is ${age}</p>
</section>
`
})
}
Here we take a widget (e.g. d3, gmaps) and wrap it so it return a DOM node once, and then only has to worry about managing its lifecycle:
var widget = require('cache-element/widget')
var morph = require('nanomorph')
var html = require('bel')
var element = Element()
var el = element('Tubi', 12) // creates new element
var el = element('Tubi', 12) // returns cached element (proxy)
var el = element('Babs', 25) // returns cached element (proxy)
function Element () {
return widget({
onupdate: function (el, name, age) {
var newEl = html`<p>Name is ${name} and age is ${age}</p>`
morph(el, newEl)
},
render: function (name, age) {
return html`<p>Name is ${name} and age is ${age}</p>`
}
})
}
Cache an element. The compare
function is optional, and defaults to:
function compare (args1, args2) {
var length = args1.length
if (length !== args2.length) return false
for (var i = 0; i < length; i++) {
if (args1[i] !== args2[i]) return false
}
return true
}
Render a widget. Takes a render
function which exposes an update
function
which takes an onupdate
function which is passed arguments whenever arguments
are passed into the tree. Unlike cache
, widget
takes no compare
function
as it will always return a proxy
element. If you want to prevent any updates
from happening, run a comparison inside onupdate
.
Render a widget. Takes an object with the following methods:
Render an element, passing it arbitrary arguments. The arguments are passed to
the element's compare
function (onupdate
for widget
).
Make sure you're running a diffing engine that checks for .isSameNode()
, if
it doesn't you'll end up with super weird results because proxy nodes will
probably be rendered which is not what should happen. Probably make sure you're
using morphdom. Seriously.
It's a node that overloads Node.isSameNode()
to compare it to another node.
This is needed because a given DOM node can only exist in one DOM tree at the
time, so we need a way to reference mounted nodes in the tree without actually
using them. Hence the proxy pattern, and the recently added support for it in
certain diffing engines:
var html = require('bel')
var el1 = html`<div>pink is the best</div>`
var el2 = html`<div>blue is the best</div>`
// let's proxy el1
var proxy = html`<div></div>`
proxy.isSameNode = function (targetNode) {
return (targetNode === el1)
}
el1.isSameNode(el1) // true
el1.isSameNode(el2) // false
proxy.isSameNode(el1) // true
proxy.isSameNode(el2) // false
Morphdom is a diffing engine that diffs real DOM trees. It runs a series of checks between nodes to see if they should either be replaced, removed, updated or reordered. This is done using a series of property checks on the nodes.
Since v2.1.0 morphdom
also runs Node.isSameNode(otherNode)
. This
allows us to override the function and replace it with a custom function that
proxies an existing node. Check out the code to see how it works. The result is
that if every element in our tree uses cache-element
, only elements that have
changed will be recomputed and rerendered making things very fast.
cache
return a proxy node if the arguments were the same. If arguments
change, it'll rerender and return a new node.widget
will always return a proxy node. It also listens for the node to be
unmounted from the DOM so it can clean up internal references, making it more
expensive to use.$ npm install cache-element
FAQs
Memoize a bel element
We found that cache-element demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.