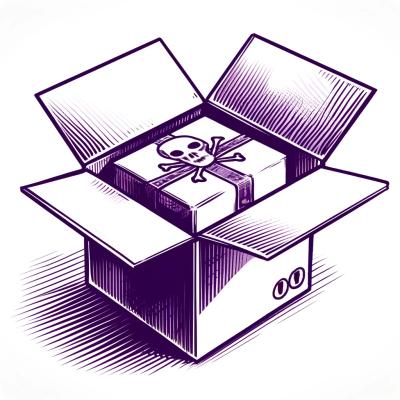
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
call-signature
Advanced tools
Parse / Generate Method Signatures
$ npm install --save call-signature
var signature = require('call-signature');
// parse a call signature definition
var parsed = signature.parse('t.equal(expected, actual, [message])');
console.log(parsed);
/* =>
{
callee: {
type: 'MemberExpression',
object: 't',
member: 'equal'
},
args: [
{
name: 'actual',
optional: false
},
{
name: 'expected',
optional: false
},
{
name: 'message',
optional: true
}
]
}
*/
// Create signature definitions from the parsed object.
signature.generate(parsed);
//=> "t.equal(expected, actual, [message])"
Type: string
A string that matches the call signature spec:
object.member(required_arg1, required_arg2, [optional_arg1])
name(required_arg1, required_arg2, [optional_arg1])
object
, member
and name
can be any identifiers, but currently the callee must be a MemberExpression
or an Identifier
(that requirement may loosen in the future).
You can have any number of arguments. Optional arguments are denoted by placing the argument name between square [
brackets]
.
A simple JS Object with three properties callee
and args
.
callee
will be an object containing type
property and its corresponding properties.
when matched against MemberExpression
like foo.bar(baz)
, object
and member
will be strings.
callee: {
type: 'MemberExpression',
object: 'foo',
member: 'bar'
}
when matched against Identifier
like foo(baz)
, name
will be string.
callee: {
type: 'Identifier',
name: 'foo'
}
args
will be an array. Each item of the array will have two properties name
, and optional
.
name
will be the string
name of the arg. optional
will be a boolean value.
Type: Object
Must have the same definition as the return value from the parse
method.
A string
signature definition that will parse to exactly the provided input.
MIT © James Talmage
FAQs
Parse / Generate Method Signatures
The npm package call-signature receives a total of 152,574 weekly downloads. As such, call-signature popularity was classified as popular.
We found that call-signature demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.